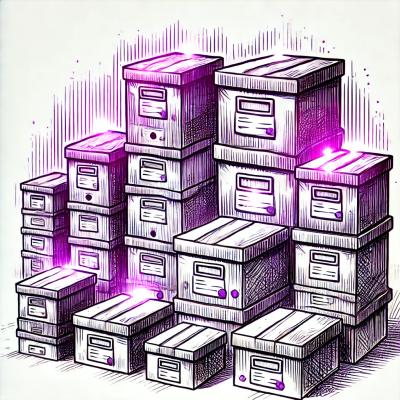
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
The mnemonist package is a collection of data structures for JavaScript and TypeScript. It provides a variety of advanced data structures that are not available in the standard JavaScript library, allowing developers to handle complex data manipulation tasks more efficiently.
FibonacciHeap
FibonacciHeap is a heap data structure that allows for efficient retrieval and modification of the minimum element. It is particularly efficient for mergeable heap operations.
const { FibonacciHeap } = require('mnemonist');
const heap = new FibonacciHeap();
heap.push(3, 'three');
heap.push(1, 'one');
heap.pop(); // -> 'one'
Trie
Trie is a tree-like data structure that is used to store a dynamic set or associative array where the keys are usually strings. It is useful for tasks like autocomplete where prefix-based search is needed.
const { Trie } = require('mnemonist');
const trie = new Trie();
trie.add('cat');
trie.add('can');
trie.has('cat'); // -> true
SuffixArray
SuffixArray is a data structure that provides an efficient way to search for substrings within a given string. It is useful for text processing and search applications.
const { SuffixArray } = require('mnemonist');
const suffixArray = new SuffixArray('banana');
suffixArray.search('ana'); // -> [1, 3]
BKTree
BKTree is a tree data structure for approximate string matching. It is useful for spell checking, fuzzy searching, and other applications where you need to find strings that are similar to a target string.
const { BKTree } = require('mnemonist');
const tree = new BKTree(levenshtein);
tree.add('book');
tree.add('back');
tree.search('bock', 2); // -> [{word: 'book', distance: 1}, {word: 'back', distance: 1}]
Immutable.js offers a wide range of persistent immutable data structures. Unlike mnemonist, which provides a mix of both mutable and immutable structures, Immutable.js focuses solely on immutable structures, which can be beneficial for certain functional programming paradigms.
Lodash is a utility library that provides a lot of the same functionality as mnemonist in terms of data manipulation, but it does not offer the same range of advanced data structures. Lodash is more focused on utility functions for common tasks.
Collect.js is a dependency-free wrapper for working with arrays and objects that mimics Laravel Collections. While it provides a fluent interface for manipulating collections of data, it does not offer the specialized data structures that mnemonist does.
Mnemonist is a curated collection of data structures for the JavaScript language.
It gathers classic data structures (think heap, trie etc.) as well as more exotic ones such as Buckhard-Keller trees etc.
It strives at being:
npm install --save mnemonist
Full documentation for the library can be found here.
Classics
Low-level & structures for very specific use cases
Information retrieval & Natural language processing
Space & time indexation
Metric space indexation
Probabilistic & succinct data structures
Utility classes
Note that this list does not include a Graph
data structure, whose implementation is usually far too complex for the scope of this library.
However, we advise the reader to take a look at the graphology
library instead.
Don't find the data structure you need? Maybe we can work it out together.
Contributions are obviously welcome. Be sure to lint the code & add relevant unit tests.
# Installing
git clone git@github.com:Yomguithereal/mnemonist.git
cd mnemonist
npm install
# Linting
npm run lint
# Running the unit tests
npm test
0.40.0
Set
operations CommonJS named export collision by renaming it to set
(@jerome-benoit).Uint8Vector
, Uint8ClampedVector
, Int8Vector
, Uint16Vector
, Int16Vector
, Uint32Vector
, Int32Vector
, Float32Vector
, Float64Vector
, PointerVector
CommonJS named exports (@jerome-benoit).PointerVector
TS exports (@jerome-benoit).ReadonlySet
in set
operations (@yoursunny).FAQs
Curated collection of data structures for the JavaScript/TypeScript.
The npm package mnemonist receives a total of 2,622,480 weekly downloads. As such, mnemonist popularity was classified as popular.
We found that mnemonist demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.