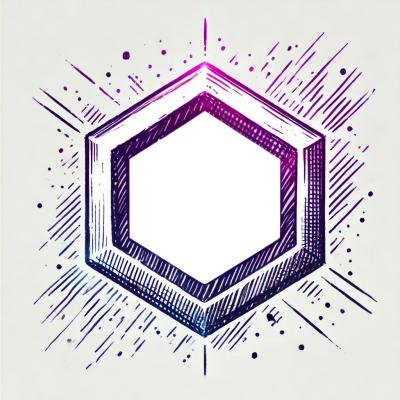
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Moonito is a simple but powerful real-time web traffic filtering service and analytics that not only helps you to track, analyse and understand your visitors but also protects your website from bot attacks, data scraping, and other unwanted activities.
Moonito is a Node.js and TypeScript module built for web protection, traffic filtering, and analytics. It safeguards your application by detecting and blocking potentially harmful traffic based on IP, user agents, and visitor behavior. With Moonito, you can shield your website from bots, competitors, and unwanted traffic, all while gaining actionable insights into your audience.
To use Moonito, you need an API key and a subscription to the Moonito service. For more details, review our Moonito documentation.
Install Moonito using npm:
npm install moonito
import express from 'express';
import { VisitorTrafficFiltering } from 'moonito';
// Create an Express application
const app = express();
const port = 3000;
/**
* Configuration object for VisitorTrafficFiltering
* This configuration is used to set up the AnalyticsHandler instance for handling visitor requests.
* To use the API, you need to set up your public and secret API keys.
*
* Visit https://moonito.net/api for more details.
*/
const config = {
isProtected: true, // Enable protection for the site (true) or disable it (false)
apiPublicKey: 'Your API Public Key', // Enter your API Public Key here
apiSecretKey: 'Your API Secret Key', // Enter your API Secret Key here
unwantedVisitorTo: 'https://google.com', // URL or HTTP error code for unwanted visitors based on `unwantedVisitorAction`
unwantedVisitorAction: 1 // Action to take for unwanted visitors: 1 = Redirect, 2 = Iframe, 3 = Load content
};
// Create an instance of VisitorTrafficFiltering with the provided configuration
const filter = new VisitorTrafficFiltering(config);
// Middleware function to handle visitor requests.
app.use(async (req, res, next) => {
try {
await filter.evaluateVisitor(req, res);
} catch (error) {
return next(error);
}
next(!res.headersSent ? undefined : null);
});
// Route handler for the root URL
app.get('/', (req, res) => {
res.send('Hello World!');
});
// Start the Express server and listen on the specified port
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
const { VisitorTrafficFiltering } = require('moonito');
/**
* Configuration object for VisitorTrafficFiltering
* This configuration is used to set up the AnalyticsHandler instance for handling visitor requests.
* To use the API, you need to set up your public and secret API keys.
*
* Visit https://moonito.net/api for more details.
*/
const config = {
isProtected: true, // Enable protection for the site (true) or disable it (false)
apiPublicKey: 'Your API Public Key', // Enter your API Public Key here
apiSecretKey: 'Your API Secret Key', // Enter your API Secret Key here
unwantedVisitorTo: 'https://google.com', // URL or HTTP error code for unwanted visitors based on `unwantedVisitorAction`
unwantedVisitorAction: 1 // Action to take for unwanted visitors: 1 = Redirect, 2 = Iframe, 3 = Load content
};
// Create an instance of VisitorTrafficFiltering with the provided configuration
const filter = new VisitorTrafficFiltering(config);
// Example visitor data
const userIP = '1.1.1.1'; // Replace with the visitor's IP
const userAgent = 'Mozilla/5.0 ...'; // Replace with the visitor's User-Agent
const event = 'page-view'; // Example event
const domain = 'example.com'; // Example domain
// Manually evaluate visitor
filter.evaluateVisitorManually(userIP, userAgent, event, domain)
.then(result => {
console.log('Visitor evaluation result:', result);
if (result.need_to_block) {
// You can handle blocked content here, e.g., displaying it on the page or redirecting
console.log('Blocked content:', result.content);
return;
}
console.log('Visitor is not blocked.');
})
.catch(error => {
console.error('Unexpected error:', error);
});
Replace Your API Public Key
and Your API Secret Key
with your actual Moonito API credentials in both methods.
We welcome contributions! For significant changes, please open an issue to discuss the proposed modifications. Make sure to update tests as necessary.
This project is licensed under the MIT license.
FAQs
Moonito is a simple but powerful real-time web traffic filtering service and analytics that not only helps you to track, analyse and understand your visitors but also protects your website from bot attacks, data scraping, and other unwanted activities.
The npm package moonito receives a total of 0 weekly downloads. As such, moonito popularity was classified as not popular.
We found that moonito demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.