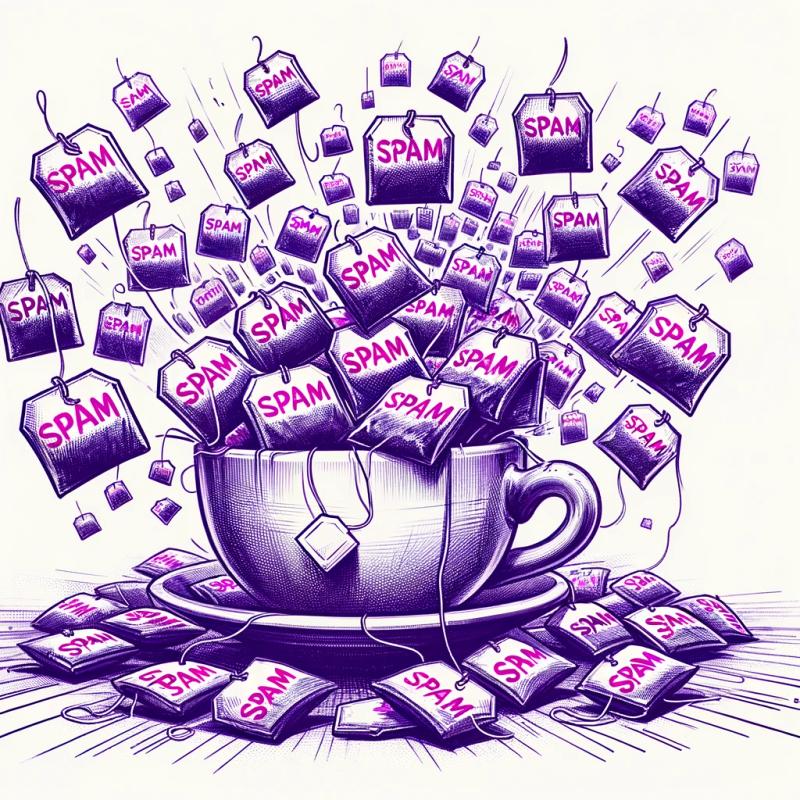
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
msafe-wallet
Advanced tools
Readme
MSafe Wallet SDK is used to integrate any dapp into msafe multi-sign wallet.
The frontend of dapp will run in an iframe under the msafe website, this SDK can be used for the interaction between dapp and msafe wallet.
This SDK wraps the msafe wallet and exposes a set of easy-to-use wallet interfaces. We are also working on integrating our SDK into aptos-wallet-adaptor, so that you can access our wallet through aptos-wallet-adaptor.
Two way to integrate msafe wallet to your dapp:
Installation of the npm package:
npm install --save msafe-wallet
To use msfe wallet, the dapp website must be running in an iframe under the msafe website. There are two way to detect if the dapp is running in msafe:
MSafeWallet.inMSafeWallet()
return true.msafe=true
.If the msafe wallet environment is detected, you can automatically connect to the msafe wallet at initialization.
Example:
import { MSafeWallet } from "msafe-wallet";
const inMSafeWallet = window.location.search.includes('msafe=true');
if(!MSafeWallet.inMSafeWallet()){ // check if the dapp is running in msafe
const url = MSafeWallet.getAppUrl('Testnet'); // get dapp url for msafe
window.location.href.replace(url); // redirect to msafe dapp
}
You should initialize it once and use it later.
To use msafe wallet, the dapp must run in msafe website.
Example:
import { MSafeWallet } from "msafe-wallet";
if(!MSafeWallet.inMSafeWallet()){ // check if the dapp is running in msafe
const url = MSafeWallet.getAppUrl('Testnet'); // get dapp url for msafe
window.location.href.replace(url); // redirect to msafe dapp
}
const msafe = await MSafeWallet.new('Testnet');
This method is used to connect to current account. It returns an account object containing the address
and publicKey
.
In the current implementation, accounts are always connected, and disconnect is not supported.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
const account = await msafe.connect(); // {addres:string, publicKey:string}
await msafe.isConnected(); // true
This method is used to get current network. It returns a string.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
const network:string = await msafe.network(); // 'Testnet'
This method is used to get current msafe account. It returns an account object containing the address
and publicKey
.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
const account:Account = await msafe.account();
console.log("address:", account.address);
console.log("public key:", account.publicKey);
This method is used to get current ChainId. It returns a number.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
const account = await msafe.chainId(); // 1
This method is used to sign the transaction and then submit it to the blockchain.
It takes two parameters:
payload
- mandatory parameter containing the transaction body.
vector<u8>
, you can pass in Uint8Array
.Uint8Array
), which ignores option.option
- optional parameter that overrides transaction parameters.In current implementation, this method call never returns. This is because when a transaction is initiated, the dapp page will be closed and then the msafe page will be entered to collect signatures. We will optimize it in future releases.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
const payload = {
type: "entry_function",
function: "0x1::coin::transfer",
type_arguments: ["0x1::aptos_coin::AptosCoin"],
arguments: ["0x997b38d2127711011462bc42e788a537eae77806404769188f20d3dc46d72750", 50]
};
// each field of option is optional.
const option = {
sender: account.address,
sequence_number: "1",
max_gas_amount: "4000",
gas_unit_price: "100",
// Unix timestamp, in seconds + 30 days
expiration_timestamp_secs: (Math.floor(Date.now() / 1000) + 30*24*3600).toString(),
}
await msafe.signAndSubmit(payload, option);
Not supported for now.
Not supported for now.
This method is used to register the callback function for the network change event.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
msafe.onChangeAccount((network:string)=>{
console.log("network change to:", network)
});
This method is used to register the callback function for the account change event.
Example:
import { MSafeWallet } from "msafe-wallet";
const msafe = await MSafeWallet.new('Testnet');
msafe.onChangeAccount((account:Account)=>{
console.log("address:", account.address);
console.log("public key:", account.publicKey);
});
The wallet adapter helps you to integrate many different wallets at once and use the same interface to interact with any supported wallet.
Here we give an example of using msafe wallet with the adaptor. For more details, see: https://github.com/hippospace/aptos-wallet-adapter.
npm install @manahippo/aptos-wallet-adapter
Example:
import {
WalletProvider,
MSafeWalletAdapter,
} from "@manahippo/aptos-wallet-adapter";
const wallets = [
new MSafeWalletAdapter('Testnet'),
];
Example:
import React from "react";
import {
WalletProvider,
MSafeWalletAdapter,
} from "@manahippo/aptos-wallet-adapter";
const wallets = [
new MSafeWalletAdapter('Testnet'),
];
const App: React.FC = () => {
return (
<WalletProvider
wallets={wallets}
onError={(error: Error) => {
console.log('Handle Error Message', error)
}}>
{/* your website */}
</WalletProvider>
);
};
export default App;
Example:
import { useWallet, MSafeWalletName } from "@manahippo/aptos-wallet-adapter";
const WalletName = MSafeWalletName;
export function DAPP() {
const {
account,
connected,
wallet,
network,
connect,
disconnect,
select,
signAndSubmitTransaction,
} = useWallet();
}
In current implementation, the function
signAndSubmitTransaction
never returns. This is because when a transaction is initiated, the dapp page will be closed and then the msafe page will be entered to collect signatures. We will optimize it in future releases.
Example:
import { useWallet, MSafeWalletName } from "@manahippo/aptos-wallet-adapter";
const WalletName = MSafeWalletName;
export function DAPP() {
const {
connect,
signAndSubmitTransaction,
} = useWallet();
return (
<>
<button onClick={()=>{connect(WalletName)}}> Connect </button>
<button onClick={()=>{
const payload = {
type: "entry_function",
function: "0x1::coin::transfer",
type_arguments: ["0x1::aptos_coin::AptosCoin"],
arguments: ["0x997b38d2127711011462bc42e788a537eae77806404769188f20d3dc46d72750", 50]
};
// each field of option is optional.
const option = {
sequence_number: "1",
max_gas_amount: "4000",
gas_unit_price: "100",
// Unix timestamp, in seconds + 30 days
expiration_timestamp_secs: (Math.floor(Date.now() / 1000) + 30*24*3600).toString(),
}
signAndSubmitTransaction(payload, option);
}}> SignAndSubmit </button>
</>
);
}
This section is for implementation on the msafe server side and is intended for use only by the msafe developers themselves.
Example:
import { Connector,MSafeServer } from "msafe-wallet";
// cleaner is a function that used to remove listener.
const cleaner = Connector.accepts(dappUrl, (connector:Connector) => {
})
Example:
import { Connector,MSafeServer,WalletAPI } from "msafe-wallet";
const connector:Connector = await Connector.accept(dappUrl);
const server = new MSafeServer(connector, {
async connect(): Promise<Account> {
// ...
return {address:'0x1', publicKey:'0x1234...'};
},
// ... signAndSubmit(),signTransaction()
async signMessage(
message: string | Uint8Array
): Promise<Uint8Array> {
throw Error("unsupport");
},
} as WalletAPI);
Example:
import { Connector,MSafeServer,WalletAPI } from "msafe-wallet";
const server = new MSafeServer(...);
await server.changeNetwork('Testnet');
Example:
import { Connector,MSafeServer,WalletAPI } from "msafe-wallet";
const server = new MSafeServer(...);
await server.changeAccount({address:'0x1234...', publicKey:'0xabce...'});
# Install dependencies
> npm install
# Build
> npm run build
# Test
> npm run test
# Publish
> npm publish
FAQs
appstore sdk of msafe wallet
The npm package msafe-wallet receives a total of 3,974 weekly downloads. As such, msafe-wallet popularity was classified as popular.
We found that msafe-wallet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.