What is multistream?
The 'multistream' npm package allows you to combine multiple streams into a single stream. This can be useful for tasks such as concatenating files, streaming data from multiple sources, or handling multiple input streams in a unified manner.
What are multistream's main functionalities?
Combining Multiple Streams
This feature allows you to combine multiple file streams into a single output stream. In this example, the contents of 'file1.txt', 'file2.txt', and 'file3.txt' are concatenated and written to 'combined.txt'.
const MultiStream = require('multistream');
const fs = require('fs');
const streams = [
fs.createReadStream('file1.txt'),
fs.createReadStream('file2.txt'),
fs.createReadStream('file3.txt')
];
MultiStream(streams).pipe(fs.createWriteStream('combined.txt'));
Dynamic Stream Creation
This feature allows you to dynamically create streams based on a factory function. The factory function is called with an index and a callback, and it should call the callback with a new stream or null to indicate the end. In this example, streams for 'file1.txt', 'file2.txt', and 'file3.txt' are created dynamically and concatenated into 'combined.txt'.
const MultiStream = require('multistream');
const fs = require('fs');
const streamFactory = (i, callback) => {
if (i > 3) return callback(null, null);
callback(null, fs.createReadStream(`file${i}.txt`));
};
MultiStream(streamFactory).pipe(fs.createWriteStream('combined.txt'));
Other packages similar to multistream
concat-stream
The 'concat-stream' package is used to concatenate multiple streams into a single buffer or string. Unlike 'multistream', which can handle multiple streams sequentially, 'concat-stream' is more focused on collecting all data into a single output at once. It is useful for scenarios where you need the entire data set available at once rather than streaming it.
stream-combiner
The 'stream-combiner' package allows you to combine multiple streams into a single pipeline. It is similar to 'multistream' in that it can handle multiple streams, but it is more focused on creating a pipeline of streams that process data in sequence. This can be useful for tasks like transforming data through multiple stages.
merge-stream
The 'merge-stream' package allows you to merge multiple streams into a single stream. Unlike 'multistream', which concatenates streams sequentially, 'merge-stream' interleaves the data from multiple streams. This can be useful for scenarios where you need to handle data from multiple sources simultaneously.
multistream

A stream that emits multiple other streams one after another (streams2)

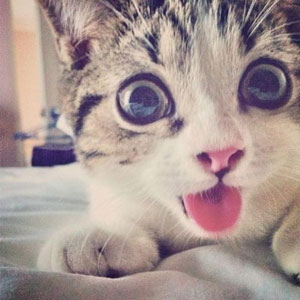
Simple, robust streams2 version of combined-stream. Allows you to combine multiple streams into a single stream. When the first stream ends, the next one starts, and so on, until all streams are consumed.
This module is used by WebTorrent, specifically create-torrent.
install
npm install multistream
usage
Use multistream
like this:
var MultiStream = require('multistream')
var fs = require('fs')
var streams = [
fs.createReadStream(__dirname + '/numbers/1.txt'),
fs.createReadStream(__dirname + '/numbers/2.txt'),
fs.createReadStream(__dirname + '/numbers/3.txt')
]
MultiStream(streams).pipe(process.stdout)
You can also create an object-mode stream with MultiStream.obj(streams)
.
To lazily create the streams, wrap them in a function:
var streams = [
fs.createReadStream(__dirname + '/numbers/1.txt'),
function () {
return fs.createReadStream(__dirname + '/numbers/2.txt')
},
function () {
return fs.createReadStream(__dirname + '/numbers/3.txt')
}
]
MultiStream(streams).pipe(process.stdout)
Alternatively, streams may be created by an asynchronous "factory" function:
var count = 0;
function factory (cb) {
if (count > 3) return cb(null, null)
count++
setTimeout(function () {
cb(null, fs.createReadStream(__dirname + '/numbers/' + count + '.txt'))
}, 100)
}
MultiStream(factory).pipe(process.stdout)
contributors
license
MIT. Copyright (c) Feross Aboukhadijeh.