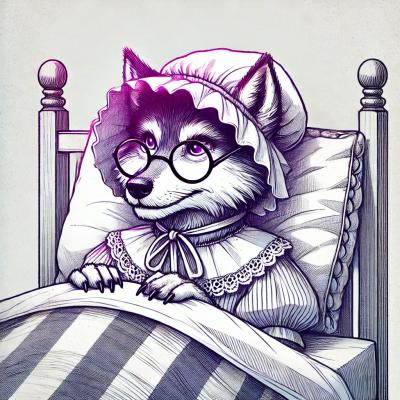
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The main idea is to help to organize models, views and controllers and to have a single point, where all the components are combined together. This could be treated as a global register of view mapped to a model class. This reduces the interdependency of some simple view in contrast to external views, but it then forcing the need oto manage this maps.
npm install mvc-pack
A recommended rules to follow to create a flexible MVC package:
Views are providing on demand by an application and the package wrapper is passing a reference to a model as an input argument.
mvcPack(
SomeModelClass,
{
text: vcPack(TextView),
table: vcPack(TableView, TableController)
}
);
const model = new SomeModelClass();
console.log(getView(model, 'table') instanceof TableView); // -> true
It is also possible to register view by providing own custom function for that. The only thing to keep in mind, that that function must return a view:
class CustomView {
...
}
class CustomController {
...
}
mvcPack(
SomeModelClass,
{
customView(model) {
const view = new CustomView(model, 'other arguments');
const controller = new CustomController(model, 'other arguments');
view.setController(controller);
return view;
}
}
);
It is recommended to create a base model class in scope of an application, in order to attach an event system to trigger and receive events. For example when some property is changing - update all connected views, or when a model is destructing - properly clean up the views.
class Model {
constructor() {
this._eventSystem = new EventTarget();
this.data = {};
}
destruct() {
this.data = null;
this.dispatchEvent('destruct');
}
setData(data) {
for (let key in data) {
this.data[key] = delta[key];
this.dispatchEvent(`update-${key}`);
}
this.dispatchEvent('update');
}
dispatchEvent(type) {
this._eventSystem.dispatchEvent(new CustomEvent(type));
}
addEventListener(type, listener) {
this._eventSystem.addEventListener(type, listener);
}
removeEventListener(type, listener) {
this._eventSystem.removeEventListener(type, listener);
}
}
In context of this package a "View" is a final chain between a model and the information representation. A view can be an HTML representation, so a View class in this context is a class that, for example creates all the needed HTML elements and or combines other Views to display some information.
An example to assotiate only a view with a model:
class TextView {
...
}
class TableView {
...
}
mvcPack(
SomeModelClass,
{
text: vcPack(TextView),
table: vcPack(TableView)
}
);
It also can work with other classes, that needs more arguments to be constructed or need to do some extra build steps:
class YourView {
...
}
mvcPack(
SomeModelClass,
{
customView(model) {
return new YourView(model, 'other', 'arguments');
}
}
);
Sometimes in between the model and view an additional data processing is needed (for example an aggregation, or an adaptation, or formatting, or something else), but the responsibilities for that is not really related to a model or a view. Or an application might require to reuse the same view component but with different data processors. A controller can help with it here. In order to make it work the following conditions must be met:
Under this conditions a controller between them can be easily inserted - a view must use a controller interface to get and display data, or use it to convert into raw format and set into the model..
class TextController {
...
}
mvcPack(
SomeModelClass,
{
text: vcPack(TextView, TextController)
}
);
class CustomController {
...
}
mvcPack(
SomeModelClass,
{
customView(model) {
const view = new CustomView(model, 'other arguments');
const controller = new CustomController(model, 'other arguments');
view.setController(controller);
return view;
}
}
);
FAQs
Organize MVC components into packs
The npm package mvc-pack receives a total of 2 weekly downloads. As such, mvc-pack popularity was classified as not popular.
We found that mvc-pack demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.