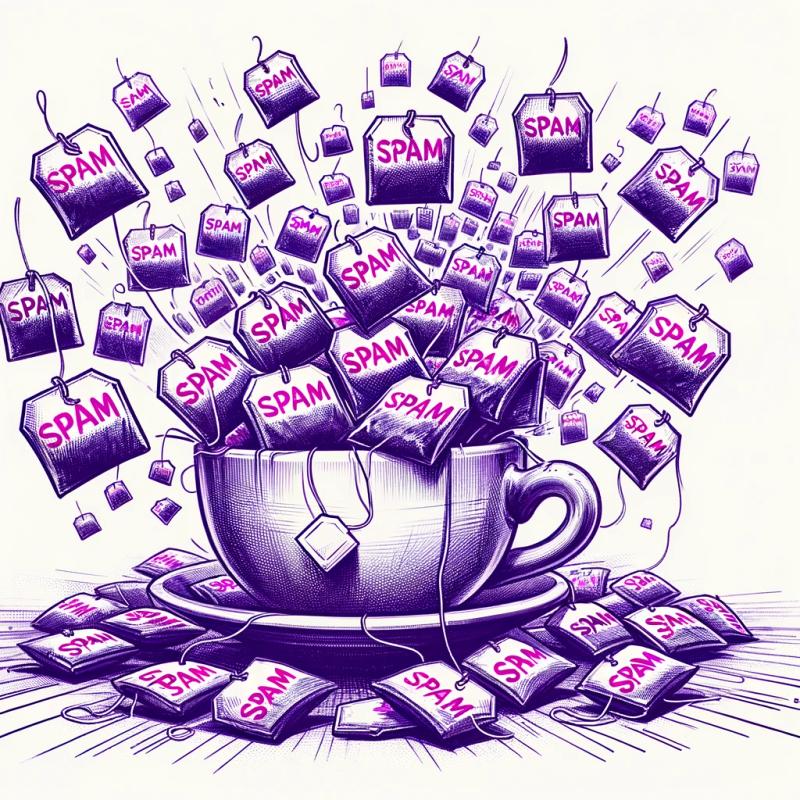
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
next-data-hooks
Advanced tools
Readme
Co-located, static data hooks in next.js
Writing one large query per page doesn't organize well. Asynchronous data fetching frameworks like apollo, relay, and react-query already allow you to write the queries closer to the component.
Why can't static data queries be written closer to the component too?
next-data-hooks
is a small and simple lib that lets you write React hooks for static data queries in next.js by lifting static props into React Context.
npm i next-data-hooks
or
yarn add next-data-hooks
_app.tsx
or _app.js
import { AppProps } from 'next/app';
import { NextDataHooksProvider } from 'next-data-hooks';
function App({ Component, pageProps }: AppProps) {
const { children, ...rest } = pageProps;
return (
<NextDataHooksProvider {...rest}>
<Component {...rest}>{children}</Component>
</NextDataHooksProvider>
);
}
At the root, add a .babelrc
file that contains the following:
{
"presets": ["next/babel"],
"plugins": ["next-data-hooks/babel"]
}
⚠️ Don't forget this step. This enables code elimination to eliminate server-side code in client code.
import { createDataHook } from 'next-data-hooks';
// this context is the GetStaticPropsContext from 'next'
// 👇
export const useBlogPost = createDataHook('BlogPost', async (context) => {
const slug = context.params?.slug as string;
// do something async to grab the data your component needs
const blogPost = /* ... */;
return blogPost;
});
import { createDataHooksProps } from 'next-data-hooks';
import { GetStaticPaths } from 'next';
import { useBlogPost } from '..';
import BlogPostComponent from '..';
export const getStaticPaths: GetStaticPaths = (context) => {
// return static paths...
};
export const getStaticProps = createDataHooksProps([useBlogPost]);
export default function BlogPostEntry() {
return (
<>
{/* Note: this component doesn't have to be a direct child of BlogPostEntry */}
<BlogPostComponent />
</>
);
}
import { useBlogPost } from '..';
function BlogPostComponent() {
const { title, content } = useBlogPost();
return (
<article>
<h1>{title}</h1>
<p>{content}</p>
</article>
);
}
createDataHook(key, getData)
Creates a data hook.
Params
Name | Type | Description |
---|---|---|
key | string | The key to uniquely identify this data hooks from other on the same page. |
getData | (variables: GetStaticPropsContext) => R | Promise<R> | An async for data that will be called via getStaticProps in next.js |
Return
A hook that can be used in any component within the page's React tree
(() => Unwrap<R>) & { getData: (variables: GetStaticPropsContext) => R | Promise<R>; key: string; }
createDataHooksProps(hooks)
Given an array of data hooks created with createDataHooks
, this function
returns a function that be used as a getStaticProps
implementation.
Params
Name | Type | Description |
---|---|---|
hooks | DataHook[] | an array of data hooks created with createDataHooks |
Return
a getStaticProps
implementation
(context: GetStaticPropsContext) => Promise<{ props: { nextDataHooks: any; }; }>
NextDataHooksProvider(nextDataHooks)
Injects the data from data hooks into React Context. Place this in _app
Params
Name | Type | Description |
---|---|---|
nextDataHooks | any |
Return
JSX.Element
FAQs
Use `getStaticProps` as react hooks
The npm package next-data-hooks receives a total of 25 weekly downloads. As such, next-data-hooks popularity was classified as not popular.
We found that next-data-hooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.