next-redux-saga
Advanced tools
Comparing version 3.0.0 to 4.0.0
@@ -78,21 +78,2 @@ import _regeneratorRuntime from '@babel/runtime/regenerator'; | ||
function _objectSpread(target) { | ||
for (var i = 1; i < arguments.length; i++) { | ||
var source = arguments[i] != null ? arguments[i] : {}; | ||
var ownKeys = Object.keys(source); | ||
if (typeof Object.getOwnPropertySymbols === 'function') { | ||
ownKeys = ownKeys.concat(Object.getOwnPropertySymbols(source).filter(function (sym) { | ||
return Object.getOwnPropertyDescriptor(source, sym).enumerable; | ||
})); | ||
} | ||
ownKeys.forEach(function (key) { | ||
_defineProperty(target, key, source[key]); | ||
}); | ||
} | ||
return target; | ||
} | ||
function _inherits(subClass, superClass) { | ||
@@ -113,2 +94,9 @@ if (typeof superClass !== "function" && superClass !== null) { | ||
function _getPrototypeOf(o) { | ||
_getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { | ||
return o.__proto__ || Object.getPrototypeOf(o); | ||
}; | ||
return _getPrototypeOf(o); | ||
} | ||
function _setPrototypeOf(o, p) { | ||
@@ -139,105 +127,78 @@ _setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { | ||
function hoc(config) { | ||
return function (BaseComponent) { | ||
var WrappedComponent = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(WrappedComponent, _Component); | ||
function withReduxSaga(BaseComponent) { | ||
var WrappedComponent = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(WrappedComponent, _Component); | ||
function WrappedComponent() { | ||
_classCallCheck(this, WrappedComponent); | ||
function WrappedComponent() { | ||
_classCallCheck(this, WrappedComponent); | ||
return _possibleConstructorReturn(this, (WrappedComponent.__proto__ || Object.getPrototypeOf(WrappedComponent)).apply(this, arguments)); | ||
return _possibleConstructorReturn(this, _getPrototypeOf(WrappedComponent).apply(this, arguments)); | ||
} | ||
_createClass(WrappedComponent, [{ | ||
key: "render", | ||
value: function render() { | ||
return React.createElement(BaseComponent, this.props); | ||
} | ||
}], [{ | ||
key: "getInitialProps", | ||
value: function () { | ||
var _getInitialProps = _asyncToGenerator( | ||
/*#__PURE__*/ | ||
_regeneratorRuntime.mark(function _callee(props) { | ||
var _props$ctx, isServer, store, pageProps; | ||
_createClass(WrappedComponent, [{ | ||
key: "render", | ||
value: function render() { | ||
return React.createElement(BaseComponent, this.props); | ||
} | ||
}], [{ | ||
key: "getInitialProps", | ||
value: function () { | ||
var _getInitialProps = _asyncToGenerator( | ||
/*#__PURE__*/ | ||
_regeneratorRuntime.mark(function _callee(props) { | ||
var _props$ctx, isServer, store, pageProps; | ||
return _regeneratorRuntime.wrap(function _callee$(_context) { | ||
while (1) { | ||
switch (_context.prev = _context.next) { | ||
case 0: | ||
_props$ctx = props.ctx, isServer = _props$ctx.isServer, store = _props$ctx.store; | ||
pageProps = {}; | ||
return _regeneratorRuntime.wrap(function _callee$(_context) { | ||
while (1) { | ||
switch (_context.prev = _context.next) { | ||
case 0: | ||
_props$ctx = props.ctx, isServer = _props$ctx.isServer, store = _props$ctx.store; | ||
pageProps = {}; | ||
if (!BaseComponent.getInitialProps) { | ||
_context.next = 6; | ||
break; | ||
} | ||
if (!BaseComponent.getInitialProps) { | ||
_context.next = 6; | ||
break; | ||
} | ||
_context.next = 5; | ||
return BaseComponent.getInitialProps(props); | ||
_context.next = 5; | ||
return BaseComponent.getInitialProps(props); | ||
case 5: | ||
pageProps = _context.sent; | ||
case 5: | ||
pageProps = _context.sent; | ||
case 6: | ||
if (!isServer) { | ||
_context.next = 10; | ||
break; | ||
} | ||
case 6: | ||
if (!(config.async && !isServer)) { | ||
_context.next = 8; | ||
break; | ||
} | ||
store.dispatch(END); | ||
_context.next = 10; | ||
return store.sagaTask.toPromise(); | ||
return _context.abrupt("return", pageProps); | ||
case 10: | ||
return _context.abrupt("return", pageProps); | ||
case 8: | ||
// Force saga to end in all other cases | ||
store.dispatch(END); | ||
_context.next = 11; | ||
return store.sagaTask.done; | ||
case 11: | ||
// Restart saga on the client (sync mode) | ||
if (!isServer) { | ||
store.runSagaTask(); | ||
} | ||
return _context.abrupt("return", pageProps); | ||
case 13: | ||
case "end": | ||
return _context.stop(); | ||
} | ||
case 11: | ||
case "end": | ||
return _context.stop(); | ||
} | ||
}, _callee, this); | ||
})); | ||
} | ||
}, _callee, this); | ||
})); | ||
return function getInitialProps(_x) { | ||
return _getInitialProps.apply(this, arguments); | ||
}; | ||
}() | ||
}]); | ||
return function getInitialProps(_x) { | ||
return _getInitialProps.apply(this, arguments); | ||
}; | ||
}() | ||
}]); | ||
return WrappedComponent; | ||
}(Component); | ||
Object.defineProperty(WrappedComponent, "displayName", { | ||
configurable: true, | ||
enumerable: true, | ||
writable: true, | ||
value: "withReduxSaga(".concat(BaseComponent.displayName || BaseComponent.name || 'BaseComponent', ")") | ||
}); | ||
return WrappedComponent; | ||
}; | ||
} | ||
}(Component); | ||
function withReduxSaga(arg) { | ||
var defaultConfig = { | ||
async: false | ||
}; | ||
_defineProperty(WrappedComponent, "displayName", "withReduxSaga(".concat(BaseComponent.displayName || BaseComponent.name || 'BaseComponent', ")")); | ||
if (typeof arg === 'function') { | ||
return hoc(defaultConfig)(arg); | ||
} | ||
return hoc(_objectSpread({}, defaultConfig, arg)); | ||
return WrappedComponent; | ||
} | ||
@@ -244,0 +205,0 @@ |
(function (global, factory) { | ||
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory(require('@babel/runtime/regenerator'), require('react'), require('redux-saga')) : | ||
typeof define === 'function' && define.amd ? define(['@babel/runtime/regenerator', 'react', 'redux-saga'], factory) : | ||
(global['next-redux-saga'] = factory(global._regeneratorRuntime,global.React,global.ReduxSaga)); | ||
}(this, (function (_regeneratorRuntime,React,reduxSaga) { 'use strict'; | ||
(global = global || self, global['next-redux-saga'] = factory(global._regeneratorRuntime, global.React, global.ReduxSaga)); | ||
}(this, function (_regeneratorRuntime, React, reduxSaga) { 'use strict'; | ||
@@ -83,21 +83,2 @@ _regeneratorRuntime = _regeneratorRuntime && _regeneratorRuntime.hasOwnProperty('default') ? _regeneratorRuntime['default'] : _regeneratorRuntime; | ||
function _objectSpread(target) { | ||
for (var i = 1; i < arguments.length; i++) { | ||
var source = arguments[i] != null ? arguments[i] : {}; | ||
var ownKeys = Object.keys(source); | ||
if (typeof Object.getOwnPropertySymbols === 'function') { | ||
ownKeys = ownKeys.concat(Object.getOwnPropertySymbols(source).filter(function (sym) { | ||
return Object.getOwnPropertyDescriptor(source, sym).enumerable; | ||
})); | ||
} | ||
ownKeys.forEach(function (key) { | ||
_defineProperty(target, key, source[key]); | ||
}); | ||
} | ||
return target; | ||
} | ||
function _inherits(subClass, superClass) { | ||
@@ -118,2 +99,9 @@ if (typeof superClass !== "function" && superClass !== null) { | ||
function _getPrototypeOf(o) { | ||
_getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { | ||
return o.__proto__ || Object.getPrototypeOf(o); | ||
}; | ||
return _getPrototypeOf(o); | ||
} | ||
function _setPrototypeOf(o, p) { | ||
@@ -144,105 +132,78 @@ _setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { | ||
function hoc(config) { | ||
return function (BaseComponent) { | ||
var WrappedComponent = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(WrappedComponent, _Component); | ||
function withReduxSaga(BaseComponent) { | ||
var WrappedComponent = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(WrappedComponent, _Component); | ||
function WrappedComponent() { | ||
_classCallCheck(this, WrappedComponent); | ||
function WrappedComponent() { | ||
_classCallCheck(this, WrappedComponent); | ||
return _possibleConstructorReturn(this, (WrappedComponent.__proto__ || Object.getPrototypeOf(WrappedComponent)).apply(this, arguments)); | ||
return _possibleConstructorReturn(this, _getPrototypeOf(WrappedComponent).apply(this, arguments)); | ||
} | ||
_createClass(WrappedComponent, [{ | ||
key: "render", | ||
value: function render() { | ||
return React__default.createElement(BaseComponent, this.props); | ||
} | ||
}], [{ | ||
key: "getInitialProps", | ||
value: function () { | ||
var _getInitialProps = _asyncToGenerator( | ||
/*#__PURE__*/ | ||
_regeneratorRuntime.mark(function _callee(props) { | ||
var _props$ctx, isServer, store, pageProps; | ||
_createClass(WrappedComponent, [{ | ||
key: "render", | ||
value: function render() { | ||
return React__default.createElement(BaseComponent, this.props); | ||
} | ||
}], [{ | ||
key: "getInitialProps", | ||
value: function () { | ||
var _getInitialProps = _asyncToGenerator( | ||
/*#__PURE__*/ | ||
_regeneratorRuntime.mark(function _callee(props) { | ||
var _props$ctx, isServer, store, pageProps; | ||
return _regeneratorRuntime.wrap(function _callee$(_context) { | ||
while (1) { | ||
switch (_context.prev = _context.next) { | ||
case 0: | ||
_props$ctx = props.ctx, isServer = _props$ctx.isServer, store = _props$ctx.store; | ||
pageProps = {}; | ||
return _regeneratorRuntime.wrap(function _callee$(_context) { | ||
while (1) { | ||
switch (_context.prev = _context.next) { | ||
case 0: | ||
_props$ctx = props.ctx, isServer = _props$ctx.isServer, store = _props$ctx.store; | ||
pageProps = {}; | ||
if (!BaseComponent.getInitialProps) { | ||
_context.next = 6; | ||
break; | ||
} | ||
if (!BaseComponent.getInitialProps) { | ||
_context.next = 6; | ||
break; | ||
} | ||
_context.next = 5; | ||
return BaseComponent.getInitialProps(props); | ||
_context.next = 5; | ||
return BaseComponent.getInitialProps(props); | ||
case 5: | ||
pageProps = _context.sent; | ||
case 5: | ||
pageProps = _context.sent; | ||
case 6: | ||
if (!isServer) { | ||
_context.next = 10; | ||
break; | ||
} | ||
case 6: | ||
if (!(config.async && !isServer)) { | ||
_context.next = 8; | ||
break; | ||
} | ||
store.dispatch(reduxSaga.END); | ||
_context.next = 10; | ||
return store.sagaTask.toPromise(); | ||
return _context.abrupt("return", pageProps); | ||
case 10: | ||
return _context.abrupt("return", pageProps); | ||
case 8: | ||
// Force saga to end in all other cases | ||
store.dispatch(reduxSaga.END); | ||
_context.next = 11; | ||
return store.sagaTask.done; | ||
case 11: | ||
// Restart saga on the client (sync mode) | ||
if (!isServer) { | ||
store.runSagaTask(); | ||
} | ||
return _context.abrupt("return", pageProps); | ||
case 13: | ||
case "end": | ||
return _context.stop(); | ||
} | ||
case 11: | ||
case "end": | ||
return _context.stop(); | ||
} | ||
}, _callee, this); | ||
})); | ||
} | ||
}, _callee, this); | ||
})); | ||
return function getInitialProps(_x) { | ||
return _getInitialProps.apply(this, arguments); | ||
}; | ||
}() | ||
}]); | ||
return function getInitialProps(_x) { | ||
return _getInitialProps.apply(this, arguments); | ||
}; | ||
}() | ||
}]); | ||
return WrappedComponent; | ||
}(React.Component); | ||
Object.defineProperty(WrappedComponent, "displayName", { | ||
configurable: true, | ||
enumerable: true, | ||
writable: true, | ||
value: "withReduxSaga(".concat(BaseComponent.displayName || BaseComponent.name || 'BaseComponent', ")") | ||
}); | ||
return WrappedComponent; | ||
}; | ||
} | ||
}(React.Component); | ||
function withReduxSaga(arg) { | ||
var defaultConfig = { | ||
async: false | ||
}; | ||
_defineProperty(WrappedComponent, "displayName", "withReduxSaga(".concat(BaseComponent.displayName || BaseComponent.name || 'BaseComponent', ")")); | ||
if (typeof arg === 'function') { | ||
return hoc(defaultConfig)(arg); | ||
} | ||
return hoc(_objectSpread({}, defaultConfig, arg)); | ||
return WrappedComponent; | ||
} | ||
@@ -252,3 +213,3 @@ | ||
}))); | ||
})); | ||
//# sourceMappingURL=next-redux-saga.umd.js.map |
{ | ||
"name": "next-redux-saga", | ||
"version": "3.0.0", | ||
"version": "4.0.0", | ||
"description": "redux-saga HOC for Next.js", | ||
@@ -17,4 +17,2 @@ "repository": "https://github.com/bmealhouse/next-redux-saga.git", | ||
"format": "prettier --write", | ||
"precommit": "xo && lint-staged", | ||
"prepush": "xo && yarn test", | ||
"prerelease": "yarn build", | ||
@@ -25,2 +23,8 @@ "release": "yarn version && git push origin master --tags && release", | ||
}, | ||
"husky": { | ||
"hooks": { | ||
"pre-commit": "xo && lint-staged", | ||
"pre-push": "xo && yarn test" | ||
} | ||
}, | ||
"jest": { | ||
@@ -77,33 +81,41 @@ "collectCoverage": true, | ||
"import/order": 0 | ||
}, | ||
"settings": { | ||
"react": { | ||
"version": "detect" | ||
} | ||
} | ||
}, | ||
"peerDependencies": { | ||
"redux-saga": "1.x" | ||
}, | ||
"devDependencies": { | ||
"@babel/core": "7.0.1", | ||
"@babel/runtime": "7.0.0", | ||
"@babel/core": "7.2.2", | ||
"@babel/runtime": "7.3.1", | ||
"babel-core": "7.0.0-bridge.0", | ||
"babel-eslint": "9.0.0", | ||
"babel-jest": "23.6.0", | ||
"enzyme": "3.6.0", | ||
"enzyme-adapter-react-16": "1.5.0", | ||
"enzyme-to-json": "3.3.4", | ||
"eslint": "5.5.0", | ||
"eslint-plugin-prettier": "2.6.2", | ||
"eslint-plugin-react": "7.11.1", | ||
"husky": "0.14.3", | ||
"jest": "23.6.0", | ||
"lint-staged": "7.2.2", | ||
"next": "6.1.2", | ||
"next-redux-wrapper": "2.0.0", | ||
"prettier": "1.14.2", | ||
"prop-types": "15.6.2", | ||
"react": "16.5.1", | ||
"react-dom": "16.5.1", | ||
"react-redux": "5.0.7", | ||
"react-test-renderer": "16.5.1", | ||
"redux": "4.0.0", | ||
"redux-saga": "0.16.0", | ||
"release": "4.0.2", | ||
"rollup": "0.65.2", | ||
"rollup-plugin-babel": "4.0.3", | ||
"xo": "0.23.0" | ||
"babel-eslint": "10.0.1", | ||
"babel-jest": "24.1.0", | ||
"enzyme": "3.8.0", | ||
"enzyme-adapter-react-16": "1.9.1", | ||
"enzyme-to-json": "3.3.5", | ||
"eslint": "5.13.0", | ||
"eslint-plugin-prettier": "3.0.1", | ||
"eslint-plugin-react": "7.12.4", | ||
"husky": "1.3.1", | ||
"jest": "24.1.0", | ||
"lint-staged": "8.1.3", | ||
"next": "7.0.2", | ||
"next-redux-wrapper": "2.1.0", | ||
"prettier": "1.16.4", | ||
"prop-types": "15.7.1", | ||
"react": "16.8.1", | ||
"react-dom": "16.8.1", | ||
"react-redux": "6.0.0", | ||
"react-test-renderer": "16.8.1", | ||
"redux": "4.0.1", | ||
"redux-saga": "1.0.1", | ||
"release": "6.0.0", | ||
"rollup": "1.1.2", | ||
"rollup-plugin-babel": "4.3.2", | ||
"xo": "0.24.0" | ||
}, | ||
@@ -110,0 +122,0 @@ "bugs": { |
@@ -6,5 +6,8 @@ # next-redux-saga | ||
[](https://github.com/prettier/prettier) | ||
[](#contributors) | ||
> redux-saga HOC for [Next.js](https://github.com/zeit/next.js/) | ||
> **Attention:** Synchronous HOC is no longer supported since version 4.0.0! | ||
## Installation | ||
@@ -55,5 +58,4 @@ | ||
/** | ||
* next-redux-saga depends on `runSagaTask` and `sagaTask` being attached to the store. | ||
* next-redux-saga depends on `sagaTask` being attached to the store. | ||
* | ||
* `runSagaTask` is used to rerun the rootSaga on the client when in sync mode (default) | ||
* `sagaTask` is used to await the rootSaga task before sending results to the client | ||
@@ -63,8 +65,4 @@ * | ||
store.runSagaTask = () => { | ||
store.sagaTask = sagaMiddleware.run(rootSaga) | ||
} | ||
store.sagaTask = sagaMiddleware.run(rootSaga) | ||
// run the rootSaga initially | ||
store.runSagaTask() | ||
return store | ||
@@ -132,21 +130,8 @@ } | ||
### Sync vs. Async API | ||
To be consistent with how Next.js works, `next-redux-saga` defaults to **sync mode** in version 2.x. When you trigger a route change on the client, your browser **WILL NOT** navigate to the new page until `getInitialProps()` has completed running all it's asynchronous tasks. | ||
For backwards compatibility with 1.x, **async mode** is still supported, however it is no longer the default behavior. When you trigger a route change on the client in async mode, your browser **WILL** navigate to the new page immediately and continue to carry out the asynchronous tasks from `getInitialProps()`. When the asynchronous tasks have completed, React will rerender the components necessary to display the async data. | ||
```js | ||
// sync mode | ||
withReduxSaga(ExamplePage) | ||
// async mode | ||
withReduxSaga({async: true})(ExamplePage) | ||
``` | ||
## Contributors | ||
| [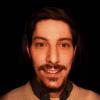](https://github.com/bmealhouse) | [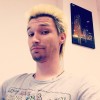](https://github.com/JerryCauser) | [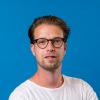](https://github.com/RobbinHabermehl) | | ||
| ----------------------------------------------------------------------------------------------- | ----------------------------------------------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- | | ||
| [Brent Mealhouse](https://github.com/bmealhouse) | [Artem Abzanov](https://github.com/JerryCauser) | [Robbin Habermehl](https://github.com/RobbinHabermehl) | | ||
<!-- ALL-CONTRIBUTORS-LIST:START - Do not remove or modify this section --> | ||
<!-- prettier-ignore --> | ||
<table><tr><td align="center"><a href="https://twitter.com/bmealhouse"><img src="https://avatars3.githubusercontent.com/u/3741255?v=4" width="100px;" alt="Brent Mealhouse"/><br /><sub><b>Brent Mealhouse</b></sub></a><br /><a href="https://github.com/bmealhouse/next-redux-saga/commits?author=bmealhouse" title="Code">💻</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=bmealhouse" title="Tests">⚠️</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=bmealhouse" title="Documentation">📖</a> <a href="#maintenance-bmealhouse" title="Maintenance">🚧</a> <a href="#question-bmealhouse" title="Answering Questions">💬</a></td><td align="center"><a href="https://bbortt.github.io"><img src="https://avatars0.githubusercontent.com/u/12272901?v=4" width="100px;" alt="Timon Borter"/><br /><sub><b>Timon Borter</b></sub></a><br /><a href="https://github.com/bmealhouse/next-redux-saga/commits?author=bbortt" title="Code">💻</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=bbortt" title="Tests">⚠️</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=bbortt" title="Documentation">📖</a> <a href="#maintenance-bbortt" title="Maintenance">🚧</a> <a href="#question-bbortt" title="Answering Questions">💬</a></td><td align="center"><a href="https://abzanov.com"><img src="https://avatars3.githubusercontent.com/u/5141037?v=4" width="100px;" alt="Artem Abzanov"/><br /><sub><b>Artem Abzanov</b></sub></a><br /><a href="https://github.com/bmealhouse/next-redux-saga/commits?author=JerryCauser" title="Code">💻</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=JerryCauser" title="Tests">⚠️</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=JerryCauser" title="Documentation">📖</a></td><td align="center"><a href="https://github.com/RobbinHabermehl"><img src="https://avatars1.githubusercontent.com/u/1640272?v=4" width="100px;" alt="Robbin Habermehl"/><br /><sub><b>Robbin Habermehl</b></sub></a><br /><a href="https://github.com/bmealhouse/next-redux-saga/commits?author=RobbinHabermehl" title="Code">💻</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=RobbinHabermehl" title="Tests">⚠️</a> <a href="https://github.com/bmealhouse/next-redux-saga/commits?author=RobbinHabermehl" title="Documentation">📖</a></td></tr></table> | ||
<!-- ALL-CONTRIBUTORS-LIST:END --> | ||
@@ -163,2 +148,2 @@ ## Contributing | ||
MIT | ||
This project is licensed under the terms of MIT license. See the [license file](https://github.com/bmealhouse/next-redux-saga/blob/master/LICENSE) for more information. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
26399
1
339
145