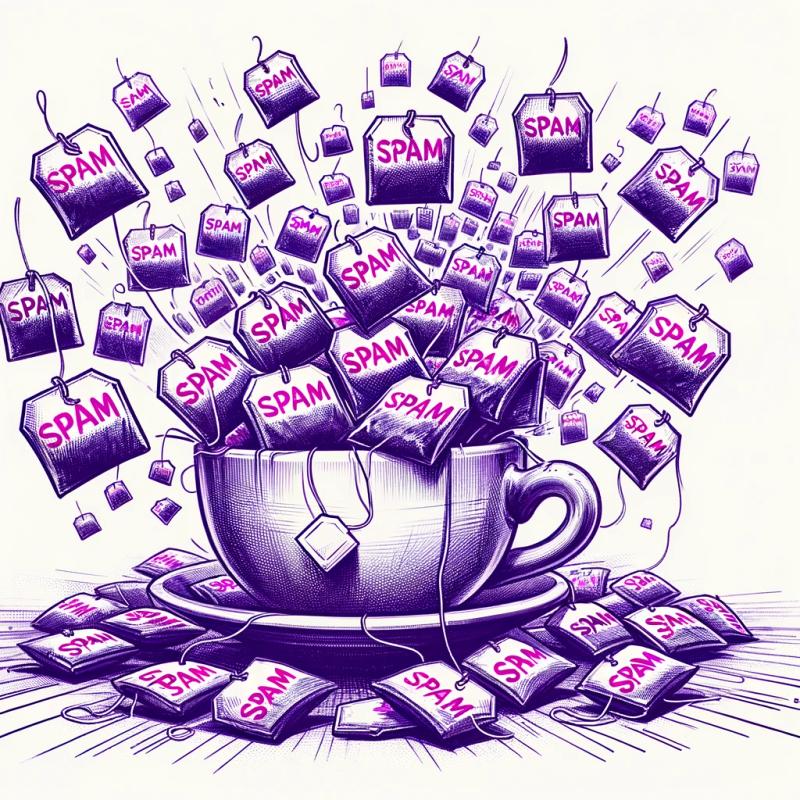
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
node-laravel-encryptor
Advanced tools
Readme
NodeJS version of Laravel's Encrypter Class, tested 5.4.30 to 6.0 Illuminate/Encryption/Encrypter.php
With this module you can create the encrypted payload for a cookie from Node Js and be read by Laravel.
You can use it too as standalone module to encrypt and decrypt data with verified signature.
Laravel only allows AES-128-CBC
AES-256-CBC
.
If no algorithm is defined default is AES-256-CBC
{
"iv": "iv in base64",
"value": "encrypted data",
"mac": "Hash HMAC"
}
$> npm i node-laravel-encryptor
const {Encryptor} = require('node-laravel-encryptor');
let encryptor = new Encryptor({
key: 'Laravel APP_KEY without base64:',
});
encryptor
.encrypt({foo: 'bar'})
.then(enc => console.log(enc));
encryptor
.decrypt(enc)
.then(dec => console.log(dec));
const {EncryptorSync} = require('node-laravel-encryptor');
let encryptor = new EncryptorSync({
key: 'Laravel APP_KEY without base64:',
});
const enc = encryptor.encrypt({foo: 'bar'});
console.log(encryptor.decrypt(enc))
base64:
DEPRECIATED
if no key_length
is given default is 64.
arguments:
if no serialize
option is given default is to serialize.
arguments:
if no serialize
option is given default is to unserialize.
$> npm run test
To be able to run PHP test you must have installed:
$> npm run test
node Laravel Encrypter
✓ should cipher and decipher
✓ should fail cipher and decipher object without serialize
✓ should cipher and decipher with no key_length defined
✓ should cipher and decipher with no serialize nor unserialize
✓ should fail cipher not valid Laravel Key
✓ should fail cipher not valid algorithm
✓ should fail decipher not valid data
✓ should cipher and decipher multiple times
✓ should decipher data at Laravel correctly (52ms)
✓ should decipher from Laravel correctly (50ms)
✓ should cipher and decipher Sync Mode
✓ should decipher data, Sync Mode, at Laravel correctly (45ms)
12 passing (173ms)
Express Crypto Cookie Compatible with Laravel
✓ should create one request to Express aSync Mode, receive cookie and decipher (38ms)
✓ should create one request to Express Sync Mode, receive cookie and decipher
2 passing (61ms)
In order to run Artillery integration test and stress test with aSync|Sync mode we have
to install artillery and artillery expect plugin.
$> npm install -g artillery artillery-plugin-expect
$> npm run artillery_server_async
$> npm run artillery_server_sync
$> npm run artillery
Summary report @ 23:42:25(+0200) 2019-09-18
Scenarios launched: 2000
Scenarios completed: 2000
Requests completed: 11009
RPS sent: 109.59
Request latency:
min: 0.6
max: 33.4
median: 1
p95: 1.8
p99: 3.1
Scenario counts:
Integration Test, parallel request no loop: 999 (49.95%)
Integration Test, parallel request: 1001 (50.05%)
Codes:
200: 11009
Summary report @ 23:55:08(+0200) 2019-09-18
Scenarios launched: 2000
Scenarios completed: 2000
Requests completed: 11144
RPS sent: 110.94
Request latency:
min: 0.6
max: 28.3
median: 1
p95: 1.8
p99: 3.1
Scenario counts:
Integration Test, parallel request no loop: 984 (49.2%)
Integration Test, parallel request: 1016 (50.8%)
Codes:
200: 11144
Blocking the Event Loop: Node core modules
Several Node core modules have synchronous expensive APIs, including:
Encryption Compression File system Child process
These APIs are expensive, because they involve significant computation (encryption, compression), require I/O (file I/O), or potentially both (child process). These APIs are intended for scripting convenience, but are not intended for use in the server context. If you execute them on the Event Loop, they will take far longer to complete than a typical JavaScript instruction, blocking the Event Loop.
In a server, you should not use the following synchronous APIs from these modules:
Encryption: crypto.randomBytes (synchronous version)
When we cipher some data we use crypto.randomBytes
function to generate cryptographically secure random bytes.
There are two modes with crypto.randomBytes
synchronous or asynchronous, official docs says you should
not use crypto.randomBytes
synchronous mode on a production server.
This module gives you the two options and other modules (with thousands downloads) I saw are using crypto.randomBytes
in synchronous mode.
If you want to write an encrypted cookie from a middleware you will prefer aSync mode,
but inside a route function you will want to write a cookie as simple as this res.ciperCookie(data)
and not execute a Promise and wait.
get('/very-complicated', (req, res) => {
res.ciperCookieSync(data);
doSomething()
.then(doOtherThing)
.then(doMuchMoreThings)
.then(data => res.json(data))
.catch(e => next(e))
})
get('/very-complicated', (req, res) => {
res.ciperCookiesaAsync(data)
.then(doSomething)
.then(doMuchMoreThings)
.then(data => res.json(data))
.catch(e => next(e))
})
We can get rid of this Promises in route functions (expressJs) making a last middleware that should do the job of writing custom data into a cookie or anywhere in aSync Mode, here a quick and dirt idea:
get('/very-complicated', (req, res, next) => {
res.cipherCookie = data; //we store cookie data inside res object
doSomething()
.then(doOtherThing)
.then(doMuchMoreThings)
.then(data => {
res.container = { data } //we pass data to next function
next(); //call next function, our middleware
})
.catch(e => next(e))
})
(req, res, next) => {
if(res.cipherCookie){
return res.ciperCookiesaAsync(res.cipherCookie)
.then(() => res.json(res.container))
.catch(e => next(e))
}
next();
};
FAQs
node version Laravel Illuminate/Encryption/Encrypter.php
The npm package node-laravel-encryptor receives a total of 347 weekly downloads. As such, node-laravel-encryptor popularity was classified as not popular.
We found that node-laravel-encryptor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.