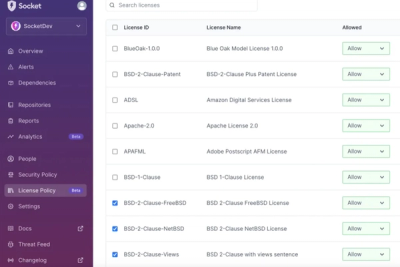
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
***WARNING: This code is still in early WIP, but most components are already working. It has NOT been thoroughly tested yet.***
WARNING: This code is still in early WIP, but most components are already working. It has NOT been thoroughly tested yet.
A simple Node.js module that interfaces with local and remote BOINC Client instances.
Currently tested and working with BOINC Client versions:
Windows:
Linux:
Just install module nodeboinc
with your favorite package manager.
NPM:
npm i nodeboinc
Yarn:
yarn add nodeboinc
const { RPC } = require('nodeboinc');
const connection = new RPC({
hostname: '127.0.0.1',
port: 31416,
password: 'password',
options: {
...
}
});
connection.on('ready', () => {
// Your code!
})
options
object.getInitialState
(boolean, default = true): Whether or not the entire client state should be fetched when the connection is first established.resolveErrorNumbers
TO BE IMPLEMENTED (boolean, default = true): Whether or not to resolve the respective error description of error numbers returned by the BOINC Client (when applicable).enablePolling
TO BE IMPLEMENTED (boolean, default = true): Whether or not to enable polling for asychronous requests. This is used in the asynchronous requests defined below.pollInterval
(number, default = 500): The time interval in milliseconds between polling requests. This is used in the following asynchronous requests:
setProject('attach')
setAccountManager('attach')
setAccountManager('update')
saveResponses
(boolean, default = true): If enabled, all methods that make a request to the BOINC client will save the response received from it in the state
object of the connection. Refer to the implemented methods for more information.
connection.responses.workunits = {
timestamp: // Unix timestamp of last response
request: // Type / options passed to the request
message: // As received from the BOINC Client
}
debug
(boolean, default = false): Enables debug output in the console and logs all requests/responses to a file.debugPath
(string, default = 'debug.log'): Defines the path where the debug log should be created if debug is enabled.All methods currently return a promise. Response format for all methods is the following:
{
success: true/false,
timestamp: // Unix timestamp of response
request: // Type / options passed to the request
message: // As received from the BOINC Client
}
rawRequest(payload)
: Is used internally by all other methods. Used for sending raw XML to the BOINC Client. The payload should NOT contain the outer <boinc_gui_rpc_request>
tag.
getState()
: Returns the current client state. Note: By default, this is being run automatically when the connection is established. Refer to the getInitialState
option. Response saved in connection.responses.state
getWU(activeOnly)
: Returns current workunits. Response saved in connection.responses.wu
getMode()
: Returns current run status (execution modes, suspension reasons, etc). Response saved in connection.responses.mode
getStatistics()
: Returns available statistics per project. Response saved in connection.responses.statistics
getWUHistory()
: Returns old tasks. Response saved in connection.responses.wuHistory
Note: (it is not specified by the BOINC documentation how far back the task history spans!)
getDiskUsage()
: Returns total and per project disk usage. Response saved in connection.responses.diskUsage
getServerVersion()
: Returns the BOINC client version. Response saved in connection.responses.serverVersion
getMessageCount()
: Returns the number of messages in the log. Response saved in connection.responses.messageCount
getMessages(seqno, translatable)
: Returns messages from the log. Response saved in connection.responses.messages
getProject(action)
: Returns project information.
connection.responses.project.list
connection.responses.project.attached
getDailyTransfers()
: Returns daily network transfer history. Response saved in connection.responses.dailyTransfers
getNotices(onlyPublic)
: Returns private and non-private notices. Response saved in connection.responses.notices
retryNetworkOps()
: Immediately retry all pending network operations. Returns true if the request was successful
retryTransfer(projectUrl, filename)
: Immediately retry a single pending network operation. Returns true if the request was successful
abortTransfer(projectUrl, filename)
: Immediately abort a single pending network operation. Returns true if the request was successful
runBenchmarks()
: Commands the BOINC client to start the CPU benchmark. Returns true if the request was successful
setMode(type, target, duration)
: Sets the run mode for the specified type. If mode is set to "all", all types will be set to the specified target for the specified duration. Returns an object in the form of {type: type, result: true/false}
setProject(action, options)
: Various project operations.
getProxySettings()
: Returns the current proxy settings. Response saved in connection.responses.proxy
getAccountManager()
: Returns information regarding the currently configured account manager. Response saved in connection.responses.accountManager
setAccountManager()
: Various account manager operations.
FAQs
***WARNING: This code is still in early WIP, but most components are already working. It has NOT been thoroughly tested yet.***
The npm package nodeboinc receives a total of 15 weekly downloads. As such, nodeboinc popularity was classified as not popular.
We found that nodeboinc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.