nuka-carousel
Advanced tools
Comparing version 7.0.0 to 8.0.0
@@ -1,366 +0,60 @@ | ||
import React$1, { ReactNode, CSSProperties } from 'react'; | ||
import * as react from 'react'; | ||
import react__default, { ReactNode } from 'react'; | ||
declare type CellAlign = 'center' | 'right' | 'left'; | ||
/** @deprecated use string literals for the values instead */ | ||
declare enum Alignment { | ||
Center = "center", | ||
Right = "right", | ||
Left = "left" | ||
} | ||
declare enum Directions { | ||
Next = "next", | ||
Prev = "prev", | ||
Up = "up", | ||
Down = "down" | ||
} | ||
declare enum Positions { | ||
TopLeft = "TopLeft", | ||
TopCenter = "TopCenter", | ||
TopRight = "TopRight", | ||
CenterLeft = "CenterLeft", | ||
CenterCenter = "CenterCenter", | ||
CenterRight = "CenterRight", | ||
BottomLeft = "BottomLeft", | ||
BottomCenter = "BottomCenter", | ||
BottomRight = "BottomRight" | ||
} | ||
declare type SlideChildren = { | ||
offsetHeight: number; | ||
type ShowArrowsOption = boolean | 'always' | 'hover'; | ||
type ScrollDistanceType = number | 'slide' | 'screen'; | ||
type CarouselCallbacks = { | ||
beforeSlide?: () => void; | ||
afterSlide?: () => void; | ||
}; | ||
declare type SlideHeight = { | ||
height: number; | ||
slideIndex: number; | ||
type CarouselProps = CarouselCallbacks & { | ||
children: ReactNode; | ||
arrows?: ReactNode; | ||
autoplay?: boolean; | ||
autoplayInterval?: number; | ||
className?: string; | ||
dots?: ReactNode; | ||
id?: string; | ||
keyboard?: boolean; | ||
scrollDistance?: ScrollDistanceType; | ||
showArrows?: ShowArrowsOption; | ||
showDots?: boolean; | ||
swiping?: boolean; | ||
title?: string; | ||
wrapMode?: 'nowrap' | 'wrap'; | ||
}; | ||
interface Slide { | ||
children?: [SlideChildren]; | ||
offsetHeight: number; | ||
} | ||
declare enum ScrollMode { | ||
page = "page", | ||
remainder = "remainder" | ||
} | ||
interface DefaultControlsConfig { | ||
containerClassName?: string; | ||
nextButtonClassName?: string; | ||
nextButtonOnClick?: React.MouseEventHandler; | ||
nextButtonStyle?: CSSProperties; | ||
nextButtonText?: ReactNode; | ||
pagingDotsClassName?: string; | ||
pagingDotsContainerClassName?: string; | ||
pagingDotsOnClick?: React.MouseEventHandler; | ||
pagingDotsStyle?: CSSProperties; | ||
prevButtonClassName?: string; | ||
prevButtonOnClick?: React.MouseEventHandler; | ||
prevButtonStyle?: CSSProperties; | ||
prevButtonText?: ReactNode; | ||
} | ||
interface KeyCodeConfig { | ||
firstSlide?: number[]; | ||
lastSlide?: number[]; | ||
nextSlide?: number[]; | ||
pause?: number[]; | ||
previousSlide?: number[]; | ||
} | ||
declare type KeyCodeFunction = 'nextSlide' | 'previousSlide' | 'firstSlide' | 'lastSlide' | 'pause' | null; | ||
interface KeyCodeMap { | ||
[key: number]: keyof KeyCodeConfig; | ||
} | ||
/** @deprecated This is not actually used for anything */ | ||
interface CarouselState { | ||
} | ||
declare type RenderAnnounceSlideMessage = (props: { | ||
currentSlide: number; | ||
count: number; | ||
}) => string; | ||
interface ControlProps extends Pick<InternalCarouselProps, 'carouselId' | 'cellAlign' | 'cellSpacing' | 'defaultControlsConfig' | 'carouselId' | 'onUserNavigation' | 'scrollMode' | 'slidesToScroll' | 'slidesToShow' | 'tabbed' | 'vertical' | 'wrapAround'> { | ||
/** | ||
* Current slide index | ||
*/ | ||
currentSlide: number; | ||
/** | ||
* The indices for the paging dots | ||
*/ | ||
pagingDotsIndices: number[]; | ||
/** | ||
* Go to a specific slide | ||
* @param targetIndex Index to go to | ||
*/ | ||
goToSlide: (targetIndex: number) => void; | ||
/** | ||
* Whether the "next" button should be disabled or not | ||
*/ | ||
nextDisabled: boolean; | ||
/** | ||
* Go to the next slide | ||
*/ | ||
nextSlide: () => void; | ||
/** | ||
* Whether the "previous" button should be disabled or not | ||
*/ | ||
previousDisabled: boolean; | ||
/** | ||
* Go to the previous slide | ||
*/ | ||
previousSlide: () => void; | ||
/** | ||
* Total number of slides | ||
*/ | ||
slideCount: number; | ||
} | ||
declare type RenderControlFunctionNames = 'renderTopLeftControls' | 'renderTopCenterControls' | 'renderTopRightControls' | 'renderCenterLeftControls' | 'renderCenterCenterControls' | 'renderCenterRightControls' | 'renderBottomLeftControls' | 'renderBottomCenterControls' | 'renderBottomRightControls'; | ||
/** | ||
* A function to override what to render on an edge/corner of the modal. | ||
* | ||
* Pass in null to not render the default controls on an edge. | ||
*/ | ||
declare type RenderControls = ((props: ControlProps) => ReactNode) | null; | ||
/** | ||
* Animation easing function accepting a normalized time between 0 and 1, | ||
* inclusive, and returning an eased time, which equals 0 at normalizedTime==0 | ||
* and equals 1 at normalizedTime==1 | ||
*/ | ||
declare type EasingFunction = (normalizedTime: number) => number; | ||
interface InternalCarouselProps { | ||
/** | ||
* If it's set to true, the carousel will adapt its height to the visible slides. | ||
*/ | ||
adaptiveHeight: boolean; | ||
/** | ||
* Whether to smoothly transition the height of the frame when using | ||
* `adaptiveHeight`. | ||
* @default true | ||
*/ | ||
adaptiveHeightAnimation: boolean; | ||
/** | ||
* Hook to be called after a slide is changed | ||
* @param index Index of the current slide | ||
*/ | ||
afterSlide: (index: number) => void; | ||
/** | ||
* Adds a zoom or fade effect on the currently visible slide. | ||
*/ | ||
animation?: 'zoom' | 'fade'; | ||
/** | ||
* Autoplay mode active | ||
* @default false | ||
*/ | ||
autoplay: boolean; | ||
/** | ||
* Interval for autoplay iteration (ms) | ||
* @default 3000 | ||
*/ | ||
autoplayInterval: number; | ||
/** | ||
* Autoplay cycles through slide indexes in reverse | ||
* @default false | ||
*/ | ||
autoplayReverse: boolean; | ||
/** | ||
* Hook to be called before a slide is changed | ||
* @param currentSlide Index of the current slide | ||
* @param endSlide Index of the last slide | ||
*/ | ||
beforeSlide: (currentSlideIndex: number, endSlideIndex: number) => void; | ||
/** | ||
* Unique id attribute for the carousel which may be referenced by aria attributes. | ||
*/ | ||
carouselId?: string; | ||
/** | ||
* When displaying more than one slide, | ||
* sets which position to anchor the current slide to | ||
*/ | ||
cellAlign: CellAlign; | ||
/** | ||
* Space between slides, as an integer, but reflected as px | ||
*/ | ||
cellSpacing: number; | ||
/** | ||
* Explicit children prop to resolve issue with @types/react v18 | ||
*/ | ||
children: ReactNode | ReactNode[]; | ||
/** | ||
* Additional className | ||
*/ | ||
className?: string; | ||
/** | ||
* This prop lets you apply custom classes and styles to the default Next, Previous, and Paging Dots controls | ||
*/ | ||
defaultControlsConfig: DefaultControlsConfig; | ||
/** | ||
* Disable slides animation | ||
* @default false | ||
*/ | ||
disableAnimation: boolean; | ||
/** | ||
* Disable swipe before first slide and after last slide | ||
* @default false | ||
*/ | ||
disableEdgeSwiping: boolean; | ||
/** | ||
* Enable mouse swipe/dragging | ||
*/ | ||
dragging: boolean; | ||
/** | ||
* The percentage (from 0 to 1) of a slide that the user needs to drag before | ||
* @default `0.5` | ||
*/ | ||
dragThreshold: number; | ||
/** | ||
* Animation easing function | ||
*/ | ||
easing: EasingFunction; | ||
/** | ||
* Animation easing function when swipe exceeds edge | ||
*/ | ||
edgeEasing: EasingFunction; | ||
/** | ||
* When set to true, disable keyboard controls | ||
* @default false | ||
*/ | ||
enableKeyboardControls: boolean; | ||
/** | ||
* Customize the aria-label of the frame container of the carousel. | ||
*/ | ||
frameAriaLabel?: string; | ||
/** | ||
* When enableKeyboardControls is enabled, Configure keyCodes for corresponding slide actions as array of keyCodes | ||
*/ | ||
keyCodeConfig: KeyCodeConfig; | ||
/** | ||
* Whether the carousel should be designated as a landmark region. | ||
*/ | ||
landmark: boolean; | ||
/** | ||
* optional callback function | ||
*/ | ||
onDragStart: (e: React.TouchEvent<HTMLDivElement> | React.MouseEvent<HTMLDivElement>) => void; | ||
/** | ||
* optional callback function | ||
*/ | ||
onDrag: (e: React.TouchEvent<HTMLDivElement> | React.MouseEvent<HTMLDivElement>) => void; | ||
/** | ||
* optional callback function | ||
*/ | ||
onDragEnd: (e: React.TouchEvent<HTMLDivElement> | React.MouseEvent<HTMLDivElement>) => void; | ||
/** | ||
* Callback called when user-triggered navigation occurs: dragging/swiping, | ||
* clicking one of the controls (custom controls not included), or using a | ||
* keyboard shortcut | ||
*/ | ||
onUserNavigation: (e: React.TouchEvent | React.MouseEvent | React.KeyboardEvent) => void; | ||
/** | ||
* Pause autoPlay when mouse is over carousel | ||
* @default true | ||
*/ | ||
pauseOnHover: boolean; | ||
/** | ||
* Function for rendering aria-live announcement messages | ||
*/ | ||
renderAnnounceSlideMessage: RenderAnnounceSlideMessage; | ||
/** | ||
* Function for rendering bottom center control | ||
*/ | ||
renderBottomCenterControls: RenderControls; | ||
/** | ||
* Function for rendering bottom left control | ||
*/ | ||
renderBottomLeftControls?: RenderControls; | ||
/** | ||
* Function for rendering bottom right control | ||
*/ | ||
renderBottomRightControls?: RenderControls; | ||
/** | ||
* Function for rendering center center control | ||
*/ | ||
renderCenterCenterControls?: RenderControls; | ||
/** | ||
* Function for rendering center left control | ||
*/ | ||
renderCenterLeftControls: RenderControls; | ||
/** | ||
* Function for rendering center right control | ||
*/ | ||
renderCenterRightControls: RenderControls; | ||
/** | ||
* Function for rendering top center control | ||
*/ | ||
renderTopCenterControls?: RenderControls; | ||
/** | ||
* Function for rendering top left control | ||
*/ | ||
renderTopLeftControls?: RenderControls; | ||
/** | ||
* Function for rendering top right control | ||
*/ | ||
renderTopRightControls?: RenderControls; | ||
/** | ||
* Supports 'page' and 'remainder' scroll modes. | ||
*/ | ||
scrollMode: ScrollMode; | ||
/** | ||
* Manually set the index of the initial slide to be shown | ||
*/ | ||
slideIndex?: number; | ||
/** | ||
* Slides to scroll at once. | ||
*/ | ||
slidesToScroll: number | 'auto'; | ||
/** | ||
* Slides to show at once | ||
*/ | ||
slidesToShow: number; | ||
/** | ||
* Sets a fixed slide width | ||
*/ | ||
slideWidth?: CSSProperties['width']; | ||
/** | ||
* Animation duration | ||
*/ | ||
speed: number; | ||
/** | ||
* style object | ||
*/ | ||
style: CSSProperties; | ||
/** | ||
* Enable touch swipe/dragging | ||
*/ | ||
swiping: boolean; | ||
/** | ||
* Whether tab pagination is used to set appropriate roles for slides. | ||
*/ | ||
tabbed: boolean; | ||
/** | ||
* Not migrated yet | ||
* | ||
* Enable the slides to transition vertically | ||
*/ | ||
vertical: boolean; | ||
/** | ||
* Used to remove all controls at once. Overwrites the render[Top, Right, Bottom, Left]CenterControls() | ||
* @default false | ||
*/ | ||
withoutControls: boolean; | ||
/** | ||
* Sets infinite wrapAround mode | ||
* @default false | ||
*/ | ||
wrapAround: boolean; | ||
/** | ||
* Adds a number value to set the scale of zoom when animation === "zoom". | ||
* The number value should be set in a range of (0,1). | ||
* @default 0.85 | ||
*/ | ||
zoomScale?: number; | ||
} | ||
/** | ||
* This component has no required props. | ||
*/ | ||
declare type CarouselProps = Partial<InternalCarouselProps>; | ||
type SlideHandle = { | ||
goForward: () => void; | ||
goBack: () => void; | ||
goToPage: (proposedIndex: number) => void; | ||
}; | ||
declare const Carousel: React$1.ForwardRefExoticComponent<Partial<InternalCarouselProps> & React$1.RefAttributes<HTMLDivElement>>; | ||
type CarouselContextType = CarouselProps & { | ||
currentPage: number; | ||
scrollOffset: number[]; | ||
totalPages: number; | ||
goToPage: (idx: number) => void; | ||
goForward: () => void; | ||
goBack: () => void; | ||
}; | ||
declare const CarouselProvider: react__default.Provider<CarouselContextType>; | ||
declare const useCarousel: () => CarouselContextType; | ||
declare const PreviousButton: ({ previousSlide, defaultControlsConfig: { prevButtonClassName, prevButtonStyle, prevButtonText, prevButtonOnClick, }, onUserNavigation, carouselId, previousDisabled: disabled, }: ControlProps) => JSX.Element; | ||
declare const NextButton: ({ nextSlide, defaultControlsConfig: { nextButtonClassName, nextButtonStyle, nextButtonText, nextButtonOnClick, }, carouselId, nextDisabled: disabled, onUserNavigation, }: ControlProps) => JSX.Element; | ||
declare const PagingDots: ({ pagingDotsIndices, defaultControlsConfig: { pagingDotsContainerClassName, pagingDotsClassName, pagingDotsStyle, pagingDotsOnClick, }, carouselId, currentSlide, onUserNavigation, slideCount, goToSlide, tabbed, }: ControlProps) => JSX.Element | null; | ||
declare const Carousel: react.ForwardRefExoticComponent<CarouselCallbacks & { | ||
children: react.ReactNode; | ||
arrows?: react.ReactNode; | ||
autoplay?: boolean | undefined; | ||
autoplayInterval?: number | undefined; | ||
className?: string | undefined; | ||
dots?: react.ReactNode; | ||
id?: string | undefined; | ||
keyboard?: boolean | undefined; | ||
scrollDistance?: ScrollDistanceType | undefined; | ||
showArrows?: ShowArrowsOption | undefined; | ||
showDots?: boolean | undefined; | ||
swiping?: boolean | undefined; | ||
title?: string | undefined; | ||
wrapMode?: "nowrap" | "wrap" | undefined; | ||
} & react.RefAttributes<SlideHandle>>; | ||
export { Alignment, CarouselProps, CarouselState, CellAlign, ControlProps, Directions, EasingFunction, InternalCarouselProps, KeyCodeConfig, KeyCodeFunction, KeyCodeMap, NextButton, PagingDots, Positions, PreviousButton, RenderControlFunctionNames, ScrollMode, Slide, SlideHeight, Carousel as default }; | ||
export { Carousel, type CarouselCallbacks, type CarouselProps, CarouselProvider, type ScrollDistanceType, type ShowArrowsOption, type SlideHandle, useCarousel }; |
1882
dist/index.js
@@ -12,3 +12,2 @@ "use strict"; | ||
var __propIsEnum = Object.prototype.propertyIsEnumerable; | ||
var __pow = Math.pow; | ||
var __defNormalProp = (obj, key, value) => key in obj ? __defProp(obj, key, { enumerable: true, configurable: true, writable: true, value }) : obj[key] = value; | ||
@@ -52,1532 +51,479 @@ var __spreadValues = (a, b) => { | ||
__export(src_exports, { | ||
Alignment: () => Alignment, | ||
Directions: () => Directions, | ||
NextButton: () => NextButton, | ||
PagingDots: () => PagingDots, | ||
Positions: () => Positions, | ||
PreviousButton: () => PreviousButton, | ||
ScrollMode: () => ScrollMode, | ||
default: () => Carousel | ||
Carousel: () => Carousel, | ||
CarouselProvider: () => CarouselProvider, | ||
useCarousel: () => useCarousel | ||
}); | ||
module.exports = __toCommonJS(src_exports); | ||
// src/carousel.tsx | ||
var import_react10 = __toESM(require("react")); | ||
// src/hooks/use-carousel.tsx | ||
var import_react = __toESM(require("react")); | ||
var CarouselContext = import_react.default.createContext( | ||
{} | ||
); | ||
var CarouselProvider = CarouselContext.Provider; | ||
var useCarousel = () => { | ||
const context = (0, import_react.useContext)(CarouselContext); | ||
return context; | ||
}; | ||
// src/slide.tsx | ||
// src/Carousel/Carousel.tsx | ||
var import_react10 = require("react"); | ||
// src/hooks/use-interval.tsx | ||
var import_react2 = require("react"); | ||
function useInterval(callback, delay, enabled = true) { | ||
const _callback = (0, import_react2.useRef)(); | ||
(0, import_react2.useEffect)(() => { | ||
_callback.current = callback; | ||
}, [callback]); | ||
(0, import_react2.useEffect)(() => { | ||
if (enabled && delay !== null) { | ||
const id = setInterval(() => { | ||
if (_callback.current) | ||
_callback.current(); | ||
}, delay); | ||
return () => clearInterval(id); | ||
} | ||
}, [delay, enabled]); | ||
} | ||
// src/hooks/use-slide-intersection-observer.ts | ||
var import_react = require("react"); | ||
var useSlideIntersectionObserver = (elementRef, rootRef, callback) => { | ||
const [entry, setEntry] = (0, import_react.useState)(); | ||
const callbackRef = (0, import_react.useRef)(callback); | ||
(0, import_react.useEffect)(() => { | ||
callbackRef.current = callback; | ||
}, [callback]); | ||
(0, import_react.useEffect)(() => { | ||
const node = elementRef == null ? void 0 : elementRef.current; | ||
const root = rootRef == null ? void 0 : rootRef.current; | ||
if (!window.IntersectionObserver || !node || !root) | ||
// src/hooks/use-paging.tsx | ||
var import_react3 = require("react"); | ||
function usePaging({ | ||
totalPages, | ||
wrapMode | ||
}) { | ||
const [currentPage, setCurrentPage] = (0, import_react3.useState)(0); | ||
const goToPage = (idx) => { | ||
if (idx < 0 || idx >= totalPages) | ||
return; | ||
const observer = new IntersectionObserver( | ||
(entries) => { | ||
entries.forEach((entry2) => { | ||
setEntry(entry2); | ||
callbackRef.current(entry2); | ||
}); | ||
}, | ||
{ | ||
threshold: [0.05, 0.95], | ||
root | ||
} | ||
); | ||
observer.observe(node); | ||
return () => observer.disconnect(); | ||
}, [elementRef, rootRef]); | ||
return entry; | ||
}; | ||
// src/slide.tsx | ||
var import_jsx_runtime = require("react/jsx-runtime"); | ||
var getSlideWidth = (count, wrapAround) => `${wrapAround ? 100 / (3 * count) : 100 / count}%`; | ||
var getSlideStyles = (count, isCurrentSlide, isVisibleSlide, wrapAround, cellSpacing, animation, speed, zoomScale, adaptiveHeight, initializedAdaptiveHeight, slideWidth) => { | ||
const width = slideWidth != null ? slideWidth : getSlideWidth(count, wrapAround); | ||
const visibleSlideOpacity = isVisibleSlide ? 1 : 0; | ||
const animationSpeed = animation === "fade" ? 200 : 500; | ||
let height = "auto"; | ||
if (adaptiveHeight) { | ||
if (initializedAdaptiveHeight) { | ||
height = "100%"; | ||
} else if (isVisibleSlide) { | ||
height = "auto"; | ||
setCurrentPage(idx); | ||
}; | ||
const goForward = () => { | ||
if (wrapMode === "wrap") { | ||
setCurrentPage((prev) => (prev + 1) % totalPages); | ||
} else { | ||
height = "0"; | ||
setCurrentPage((prev) => Math.min(prev + 1, totalPages - 1)); | ||
} | ||
} | ||
return { | ||
width, | ||
height, | ||
padding: `0 ${cellSpacing ? cellSpacing / 2 : 0}px`, | ||
transition: animation ? `${speed || animationSpeed}ms ease 0s` : void 0, | ||
transform: animation === "zoom" ? `scale(${isCurrentSlide && isVisibleSlide ? 1 : zoomScale || 0.85})` : void 0, | ||
opacity: animation === "fade" ? visibleSlideOpacity : 1 | ||
}; | ||
}; | ||
var generateIndex = (index, count, typeOfSlide) => { | ||
if (typeOfSlide === "prev-cloned") { | ||
return index - count; | ||
} | ||
if (typeOfSlide === "next-cloned") { | ||
return index + count; | ||
} | ||
return index; | ||
}; | ||
var Slide = ({ | ||
count, | ||
children, | ||
index, | ||
isCurrentSlide, | ||
typeOfSlide, | ||
wrapAround, | ||
cellSpacing, | ||
slideWidth, | ||
animation, | ||
speed, | ||
zoomScale, | ||
onVisibleSlideHeightChange, | ||
adaptiveHeight, | ||
initializedAdaptiveHeight, | ||
updateIOEntry, | ||
id, | ||
carouselRef, | ||
carouselId, | ||
tabbed | ||
}) => { | ||
var _a; | ||
const customIndex = wrapAround ? generateIndex(index, count, typeOfSlide) : index; | ||
const slideRef = (0, import_react2.useRef)(null); | ||
const entry = useSlideIntersectionObserver(slideRef, carouselRef, (entry2) => { | ||
updateIOEntry(id, (entry2 == null ? void 0 : entry2.intersectionRatio) >= 0.95); | ||
}); | ||
const isVisible = !!(entry == null ? void 0 : entry.isIntersecting); | ||
const isFullyVisible = ((_a = entry == null ? void 0 : entry.intersectionRatio) != null ? _a : 1) >= 0.95; | ||
const prevIsVisibleRef = (0, import_react2.useRef)(false); | ||
(0, import_react2.useEffect)(() => { | ||
var _a2; | ||
const node = slideRef.current; | ||
if (node) { | ||
const slideHeight = (_a2 = node.getBoundingClientRect()) == null ? void 0 : _a2.height; | ||
const prevIsVisible = prevIsVisibleRef.current; | ||
if (isVisible && !prevIsVisible) { | ||
onVisibleSlideHeightChange(customIndex, slideHeight); | ||
} else if (!isVisible && prevIsVisible) { | ||
onVisibleSlideHeightChange(customIndex, null); | ||
const goBack = () => { | ||
if (wrapMode === "wrap") { | ||
setCurrentPage((prev) => (prev - 1 + totalPages) % totalPages); | ||
} else { | ||
setCurrentPage((prev) => Math.max(prev - 1, 0)); | ||
} | ||
}; | ||
return { currentPage, goToPage, goForward, goBack }; | ||
} | ||
// src/hooks/use-debounced.tsx | ||
var import_react4 = require("react"); | ||
function useDebounced(callback, delay) { | ||
const timerRef = (0, import_react4.useRef)(); | ||
return (0, import_react4.useCallback)( | ||
(...args) => { | ||
if (timerRef.current) { | ||
clearTimeout(timerRef.current); | ||
} | ||
prevIsVisibleRef.current = isVisible; | ||
} | ||
}, [customIndex, isVisible, onVisibleSlideHeightChange]); | ||
const currentSlideClass = isCurrentSlide && isFullyVisible ? " slide-current" : ""; | ||
return /* @__PURE__ */ (0, import_jsx_runtime.jsx)( | ||
"div", | ||
__spreadProps(__spreadValues({ | ||
ref: slideRef | ||
}, { inert: isFullyVisible ? void 0 : "true" }), { | ||
className: `slide${currentSlideClass}${typeOfSlide ? ` ${typeOfSlide}` : ""}${isFullyVisible ? " slide-visible" : ""}`, | ||
style: getSlideStyles( | ||
count, | ||
isCurrentSlide, | ||
isFullyVisible, | ||
wrapAround, | ||
cellSpacing, | ||
animation, | ||
speed, | ||
zoomScale, | ||
adaptiveHeight, | ||
initializedAdaptiveHeight, | ||
slideWidth | ||
), | ||
id: typeOfSlide ? void 0 : `${carouselId}-slide-${index + 1}`, | ||
role: tabbed ? "tabpanel" : "group", | ||
"aria-roledescription": tabbed ? void 0 : "slide", | ||
children | ||
}) | ||
timerRef.current = setTimeout(() => { | ||
callback(...args); | ||
}, delay); | ||
}, | ||
[callback, delay] | ||
); | ||
}; | ||
var slide_default = Slide; | ||
} | ||
// src/announce-slide.tsx | ||
var import_jsx_runtime2 = require("react/jsx-runtime"); | ||
var styles = { | ||
position: "absolute", | ||
width: "1px", | ||
height: "1px", | ||
overflow: "hidden", | ||
padding: 0, | ||
margin: "-1px", | ||
clip: "rect(0, 0, 0, 0)", | ||
whiteSpace: "nowrap", | ||
border: 0 | ||
}; | ||
var AnnounceSlide = ({ | ||
message, | ||
ariaLive = "polite" | ||
}) => /* @__PURE__ */ (0, import_jsx_runtime2.jsx)("div", { "aria-live": ariaLive, "aria-atomic": "true", style: styles, tabIndex: -1, children: message }); | ||
var defaultRenderAnnounceSlideMessage = ({ | ||
currentSlide, | ||
count | ||
}) => `Slide ${currentSlide + 1} of ${count}`; | ||
var announce_slide_default = AnnounceSlide; | ||
// src/hooks/use-measurement.tsx | ||
var import_react6 = require("react"); | ||
// src/slider-list.tsx | ||
var import_react5 = __toESM(require("react")); | ||
// src/utils/array.ts | ||
function arraySeq(length, initialValue) { | ||
return new Array(length).fill(0).map((_, i) => i * initialValue); | ||
} | ||
function arraySum(values) { | ||
let sum = 0; | ||
return values.map((value) => sum += value); | ||
} | ||
function nint(array, target) { | ||
return array.reduce( | ||
(prev, curr) => Math.abs(curr - target) < Math.abs(prev - target) ? curr : prev | ||
); | ||
} | ||
// src/default-controls.tsx | ||
var import_react3 = require("react"); | ||
// src/utils/css.ts | ||
function cls(...classes) { | ||
return classes.filter(Boolean).join(" "); | ||
} | ||
// src/types.ts | ||
var Alignment = /* @__PURE__ */ ((Alignment2) => { | ||
Alignment2["Center"] = "center"; | ||
Alignment2["Right"] = "right"; | ||
Alignment2["Left"] = "left"; | ||
return Alignment2; | ||
})(Alignment || {}); | ||
var Directions = /* @__PURE__ */ ((Directions2) => { | ||
Directions2["Next"] = "next"; | ||
Directions2["Prev"] = "prev"; | ||
Directions2["Up"] = "up"; | ||
Directions2["Down"] = "down"; | ||
return Directions2; | ||
})(Directions || {}); | ||
var Positions = /* @__PURE__ */ ((Positions2) => { | ||
Positions2["TopLeft"] = "TopLeft"; | ||
Positions2["TopCenter"] = "TopCenter"; | ||
Positions2["TopRight"] = "TopRight"; | ||
Positions2["CenterLeft"] = "CenterLeft"; | ||
Positions2["CenterCenter"] = "CenterCenter"; | ||
Positions2["CenterRight"] = "CenterRight"; | ||
Positions2["BottomLeft"] = "BottomLeft"; | ||
Positions2["BottomCenter"] = "BottomCenter"; | ||
Positions2["BottomRight"] = "BottomRight"; | ||
return Positions2; | ||
})(Positions || {}); | ||
var ScrollMode = /* @__PURE__ */ ((ScrollMode2) => { | ||
ScrollMode2["page"] = "page"; | ||
ScrollMode2["remainder"] = "remainder"; | ||
return ScrollMode2; | ||
})(ScrollMode || {}); | ||
// src/utils/browser.ts | ||
var isBrowser = () => typeof window !== "undefined"; | ||
// src/utils.ts | ||
var getNextMoveIndex = (scrollMode, wrapAround, currentSlide, slideCount, slidesToScroll, slidesToShow, cellAlign) => { | ||
if (wrapAround) { | ||
return currentSlide + slidesToScroll; | ||
} | ||
if (currentSlide >= slideCount - 1 || cellAlign === "left" && currentSlide >= slideCount - slidesToShow) { | ||
return currentSlide; | ||
} | ||
if (scrollMode === "remainder" /* remainder */ && cellAlign === "left") { | ||
return Math.min(currentSlide + slidesToScroll, slideCount - slidesToShow); | ||
} | ||
return Math.min(currentSlide + slidesToScroll, slideCount - 1); | ||
}; | ||
var getPrevMoveIndex = (scrollMode, wrapAround, currentSlide, slidesToScroll, slidesToShow, cellAlign) => { | ||
if (wrapAround) { | ||
return currentSlide - slidesToScroll; | ||
} | ||
if (currentSlide <= 0 || cellAlign === "right" && currentSlide <= slidesToShow - 1) { | ||
return currentSlide; | ||
} | ||
if (scrollMode === "remainder" /* remainder */ && cellAlign === "right") { | ||
return Math.max(currentSlide - slidesToScroll, slidesToShow - 1); | ||
} | ||
return Math.max(currentSlide - slidesToScroll, 0); | ||
}; | ||
var getDefaultSlideIndex = (slideIndex, slideCount, slidesToShow, slidesToScroll, cellAlign, autoplayReverse, scrollMode) => { | ||
if (slideIndex !== void 0) { | ||
return slideIndex; | ||
} | ||
const dotIndexes = getDotIndexes( | ||
slideCount, | ||
slidesToScroll, | ||
scrollMode, | ||
slidesToShow, | ||
false, | ||
cellAlign | ||
); | ||
return autoplayReverse ? dotIndexes[dotIndexes.length - 1] : dotIndexes[0]; | ||
}; | ||
var getBoundedIndex = (rawIndex, slideCount) => { | ||
return (rawIndex % slideCount + slideCount) % slideCount; | ||
}; | ||
// src/hooks/use-resize-observer.tsx | ||
var import_react5 = require("react"); | ||
function useResizeObserver(element) { | ||
const [dimensions, setDimensions] = (0, import_react5.useState)(); | ||
(0, import_react5.useEffect)(() => { | ||
if (!element.current) | ||
return; | ||
const target = element.current; | ||
const observer = new ResizeObserver( | ||
() => setDimensions(target.getBoundingClientRect()) | ||
); | ||
observer.observe(target); | ||
return () => { | ||
observer.unobserve(target); | ||
}; | ||
}, [element]); | ||
return dimensions; | ||
} | ||
// src/default-controls.tsx | ||
var import_jsx_runtime3 = require("react/jsx-runtime"); | ||
var defaultButtonStyles = (disabled) => ({ | ||
border: 0, | ||
background: "rgba(0,0,0,0.4)", | ||
color: "white", | ||
padding: 10, | ||
textTransform: "uppercase", | ||
opacity: disabled ? 0.3 : 1, | ||
cursor: disabled ? "not-allowed" : "pointer" | ||
}); | ||
var prevButtonDisabled = ({ | ||
cellAlign, | ||
currentSlide, | ||
slidesToShow, | ||
wrapAround | ||
}) => { | ||
if (wrapAround) { | ||
return false; | ||
} | ||
if (currentSlide === 0) { | ||
return true; | ||
} | ||
if (cellAlign === "right" && currentSlide <= slidesToShow - 1) { | ||
return true; | ||
} | ||
return false; | ||
}; | ||
var PreviousButton = ({ | ||
previousSlide, | ||
defaultControlsConfig: { | ||
prevButtonClassName, | ||
prevButtonStyle = {}, | ||
prevButtonText, | ||
prevButtonOnClick | ||
}, | ||
onUserNavigation, | ||
carouselId, | ||
previousDisabled: disabled | ||
}) => { | ||
const handleClick = (event) => { | ||
prevButtonOnClick == null ? void 0 : prevButtonOnClick(event); | ||
if (event.defaultPrevented) | ||
// src/hooks/use-measurement.tsx | ||
function useMeasurement({ element, scrollDistance }) { | ||
const [totalPages, setTotalPages] = (0, import_react6.useState)(0); | ||
const [scrollOffset, setScrollOffset] = (0, import_react6.useState)(arraySeq(totalPages, 0)); | ||
const dimensions = useResizeObserver(element); | ||
(0, import_react6.useEffect)(() => { | ||
var _a; | ||
const container = element.current; | ||
if (!(container && dimensions)) | ||
return; | ||
onUserNavigation(event); | ||
event.preventDefault(); | ||
previousSlide(); | ||
}; | ||
return /* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"button", | ||
{ | ||
className: prevButtonClassName, | ||
style: __spreadValues(__spreadValues({}, defaultButtonStyles(disabled)), prevButtonStyle), | ||
disabled, | ||
onClick: handleClick, | ||
"aria-label": "previous", | ||
"aria-controls": `${carouselId}-slider-frame`, | ||
type: "button", | ||
children: prevButtonText || "Prev" | ||
} | ||
); | ||
}; | ||
var nextButtonDisabled = ({ | ||
cellAlign, | ||
currentSlide, | ||
slideCount, | ||
slidesToShow, | ||
wrapAround | ||
}) => { | ||
if (wrapAround) { | ||
return false; | ||
} | ||
if (currentSlide >= slideCount - 1) { | ||
return true; | ||
} | ||
if (cellAlign === "left" && currentSlide >= slideCount - slidesToShow) { | ||
return true; | ||
} | ||
return false; | ||
}; | ||
var NextButton = ({ | ||
nextSlide, | ||
defaultControlsConfig: { | ||
nextButtonClassName, | ||
nextButtonStyle = {}, | ||
nextButtonText, | ||
nextButtonOnClick | ||
}, | ||
carouselId, | ||
nextDisabled: disabled, | ||
onUserNavigation | ||
}) => { | ||
const handleClick = (event) => { | ||
nextButtonOnClick == null ? void 0 : nextButtonOnClick(event); | ||
if (event.defaultPrevented) | ||
return; | ||
onUserNavigation(event); | ||
event.preventDefault(); | ||
nextSlide(); | ||
}; | ||
return /* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"button", | ||
{ | ||
className: nextButtonClassName, | ||
style: __spreadValues(__spreadValues({}, defaultButtonStyles(disabled)), nextButtonStyle), | ||
disabled, | ||
onClick: handleClick, | ||
"aria-label": "next", | ||
"aria-controls": `${carouselId}-slider-frame`, | ||
type: "button", | ||
children: nextButtonText || "Next" | ||
} | ||
); | ||
}; | ||
var getDotIndexes = (slideCount, slidesToScroll, scrollMode, slidesToShow, wrapAround, cellAlign) => { | ||
const dotIndexes = []; | ||
const scrollSlides = slidesToScroll <= 0 ? 1 : slidesToScroll; | ||
if (wrapAround) { | ||
for (let i = 0; i < slideCount; i += scrollSlides) { | ||
dotIndexes.push(i); | ||
} | ||
return dotIndexes; | ||
} | ||
if (cellAlign === "center") { | ||
for (let i = 0; i < slideCount - 1; i += scrollSlides) { | ||
dotIndexes.push(i); | ||
} | ||
if (slideCount > 0) { | ||
dotIndexes.push(slideCount - 1); | ||
} | ||
return dotIndexes; | ||
} | ||
if (cellAlign === "left") { | ||
if (slidesToShow >= slideCount) { | ||
return [0]; | ||
} | ||
const lastPossibleIndexWithoutWhitespace = slideCount - slidesToShow; | ||
for (let i = 0; i < lastPossibleIndexWithoutWhitespace; i += scrollSlides) { | ||
dotIndexes.push(i); | ||
} | ||
if (scrollMode === "remainder" /* remainder */) { | ||
dotIndexes.push(lastPossibleIndexWithoutWhitespace); | ||
} else { | ||
dotIndexes.push(dotIndexes[dotIndexes.length - 1] + scrollSlides); | ||
} | ||
return dotIndexes; | ||
} | ||
if (cellAlign === "right") { | ||
if (slidesToShow >= slideCount) { | ||
return [slideCount - 1]; | ||
} | ||
const firstPossibleIndexWithoutWhitespace = slidesToShow - 1; | ||
if (scrollMode === "remainder" /* remainder */) { | ||
for (let i = firstPossibleIndexWithoutWhitespace; i < slideCount - 1; i += scrollSlides) { | ||
dotIndexes.push(i); | ||
const scrollWidth = container.scrollWidth; | ||
const visibleWidth = container.offsetWidth; | ||
const remainder = scrollWidth - visibleWidth; | ||
switch (scrollDistance) { | ||
case "screen": { | ||
const pageCount = Math.ceil(scrollWidth / visibleWidth); | ||
setTotalPages(pageCount); | ||
setScrollOffset(arraySeq(pageCount, visibleWidth)); | ||
break; | ||
} | ||
dotIndexes.push(slideCount - 1); | ||
} else { | ||
for (let i = slideCount - 1; i > firstPossibleIndexWithoutWhitespace; i -= scrollSlides) { | ||
dotIndexes.push(i); | ||
case "slide": { | ||
const children = ((_a = container.querySelector("#nuka-wrapper")) == null ? void 0 : _a.children) || []; | ||
const offsets = Array.from(children).map( | ||
(child) => child.offsetWidth | ||
); | ||
const scrollOffsets = arraySum([0, ...offsets.slice(0, -1)]); | ||
const pageCount = scrollOffsets.findIndex((offset) => offset >= remainder) + 1; | ||
setTotalPages(pageCount); | ||
setScrollOffset(scrollOffsets); | ||
break; | ||
} | ||
dotIndexes.push(dotIndexes[dotIndexes.length - 1] - scrollSlides); | ||
dotIndexes.reverse(); | ||
default: { | ||
if (typeof scrollDistance === "number") { | ||
const pageCount = Math.ceil(remainder / scrollDistance) + 1; | ||
setTotalPages(pageCount); | ||
setScrollOffset(arraySeq(pageCount, scrollDistance)); | ||
} | ||
} | ||
} | ||
return dotIndexes; | ||
} | ||
return dotIndexes; | ||
}; | ||
var PagingDots = ({ | ||
pagingDotsIndices, | ||
defaultControlsConfig: { | ||
pagingDotsContainerClassName, | ||
pagingDotsClassName, | ||
pagingDotsStyle = {}, | ||
pagingDotsOnClick | ||
}, | ||
carouselId, | ||
currentSlide, | ||
onUserNavigation, | ||
slideCount, | ||
goToSlide, | ||
tabbed | ||
}) => { | ||
const listStyles = { | ||
position: "relative", | ||
top: -10, | ||
display: "flex", | ||
margin: 0, | ||
padding: 0, | ||
listStyleType: "none" | ||
}; | ||
const getButtonStyles = (0, import_react3.useCallback)( | ||
(active) => ({ | ||
cursor: "pointer", | ||
opacity: active ? 1 : 0.5, | ||
background: "transparent", | ||
border: "none", | ||
fill: "black" | ||
}), | ||
[] | ||
); | ||
const currentSlideBounded = getBoundedIndex(currentSlide, slideCount); | ||
if (!tabbed) | ||
return null; | ||
return /* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"div", | ||
{ | ||
className: pagingDotsContainerClassName, | ||
style: listStyles, | ||
role: "tablist", | ||
"aria-label": "Choose slide to display.", | ||
children: pagingDotsIndices.map((slideIndex, i) => { | ||
const isActive = currentSlideBounded === slideIndex || // sets navigation dots active if the current slide falls in the current index range | ||
currentSlideBounded < slideIndex && (i === 0 || currentSlideBounded > pagingDotsIndices[i - 1]); | ||
return /* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"button", | ||
{ | ||
className: [ | ||
"paging-item", | ||
pagingDotsClassName, | ||
isActive ? "active" : null | ||
].join(" "), | ||
type: "button", | ||
style: __spreadValues(__spreadValues({}, getButtonStyles(isActive)), pagingDotsStyle), | ||
onClick: (event) => { | ||
pagingDotsOnClick == null ? void 0 : pagingDotsOnClick(event); | ||
if (event.defaultPrevented) | ||
return; | ||
onUserNavigation(event); | ||
goToSlide(slideIndex); | ||
}, | ||
"aria-label": `slide ${slideIndex + 1}`, | ||
"aria-selected": isActive, | ||
"aria-controls": `${carouselId}-slide-${slideIndex + 1}`, | ||
role: "tab", | ||
children: /* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"svg", | ||
{ | ||
className: "paging-dot", | ||
width: "6", | ||
height: "6", | ||
"aria-hidden": "true", | ||
focusable: "false", | ||
viewBox: "0 0 6 6", | ||
children: /* @__PURE__ */ (0, import_jsx_runtime3.jsx)("circle", { cx: "3", cy: "3", r: "3" }) | ||
} | ||
) | ||
}, | ||
slideIndex | ||
); | ||
}) | ||
} | ||
); | ||
}; | ||
}, [element, scrollDistance, dimensions]); | ||
return { totalPages, scrollOffset }; | ||
} | ||
// src/hooks/use-tween.ts | ||
var import_react4 = require("react"); | ||
var useTween = (durationMs, easingFunction, navigationNum, shouldInterrupt) => { | ||
const [normalizedTimeRaw, setNormalizedTime] = (0, import_react4.useState)(1); | ||
const startTime = (0, import_react4.useRef)(Date.now()); | ||
const rAF = (0, import_react4.useRef)(); | ||
const isFirstRender = (0, import_react4.useRef)(true); | ||
const lastNavigationNum = (0, import_react4.useRef)(null); | ||
const normalizedTime = lastNavigationNum.current === null || lastNavigationNum.current === navigationNum || shouldInterrupt ? normalizedTimeRaw : 0; | ||
(0, import_react4.useEffect)(() => { | ||
lastNavigationNum.current = navigationNum; | ||
if (isFirstRender.current) { | ||
isFirstRender.current = false; | ||
// src/hooks/use-hover.tsx | ||
var import_react7 = require("react"); | ||
function useHover({ | ||
element, | ||
enabled | ||
}) { | ||
const [hovered, setHovered] = (0, import_react7.useState)(false); | ||
const target = element == null ? void 0 : element.current; | ||
(0, import_react7.useEffect)(() => { | ||
if (!(target && target.addEventListener)) | ||
return; | ||
if (enabled) { | ||
const onMouseOver = () => setHovered(true); | ||
const onMouseOut = () => setHovered(false); | ||
target.addEventListener("mouseover", onMouseOver); | ||
target.addEventListener("mouseout", onMouseOut); | ||
return () => { | ||
target.removeEventListener("mouseover", onMouseOver); | ||
target.removeEventListener("mouseout", onMouseOut); | ||
}; | ||
} | ||
if (shouldInterrupt) { | ||
}, [target, enabled]); | ||
return hovered; | ||
} | ||
// src/hooks/use-keyboard.tsx | ||
var import_react8 = require("react"); | ||
function useKeyboard({ | ||
element, | ||
enabled, | ||
goForward, | ||
goBack | ||
}) { | ||
const target = element == null ? void 0 : element.current; | ||
(0, import_react8.useEffect)(() => { | ||
if (target && enabled) { | ||
const onKeyDown = (e) => { | ||
if (e.key === "ArrowLeft") { | ||
goBack(); | ||
} else if (e.key === "ArrowRight") { | ||
goForward(); | ||
} | ||
}; | ||
target.addEventListener("keydown", onKeyDown); | ||
return () => target.removeEventListener("keydown", onKeyDown); | ||
} | ||
}, [enabled, goBack, goForward, target]); | ||
} | ||
// src/hooks/use-reduced-motion.tsx | ||
var import_react9 = require("react"); | ||
var QUERY = "(prefers-reduced-motion: no-preference)"; | ||
var getInitialState = () => isBrowser() ? !window.matchMedia(QUERY).matches : true; | ||
function useReducedMotion({ enabled }) { | ||
const [reduceMotion, setReducedMotion] = (0, import_react9.useState)(getInitialState); | ||
(0, import_react9.useEffect)(() => { | ||
if (!(isBrowser() && enabled)) | ||
return; | ||
} | ||
startTime.current = Date.now(); | ||
setNormalizedTime(0); | ||
const tick = () => { | ||
rAF.current = requestAnimationFrame(() => { | ||
const currentTime = Date.now(); | ||
const normalizedTime2 = Math.min( | ||
1, | ||
(currentTime - startTime.current) / durationMs | ||
); | ||
setNormalizedTime(normalizedTime2); | ||
if (normalizedTime2 < 1) { | ||
tick(); | ||
} else { | ||
rAF.current = void 0; | ||
} | ||
}); | ||
const mediaQueryList = window.matchMedia(QUERY); | ||
const listener = (event) => { | ||
setReducedMotion(!event.matches); | ||
}; | ||
tick(); | ||
mediaQueryList.addEventListener("change", listener); | ||
return () => { | ||
if (rAF.current !== void 0) { | ||
cancelAnimationFrame(rAF.current); | ||
setNormalizedTime(1); | ||
} | ||
mediaQueryList.removeEventListener("change", listener); | ||
}; | ||
}, [navigationNum, durationMs, shouldInterrupt]); | ||
return { | ||
isAnimating: normalizedTime !== 1, | ||
value: easingFunction(normalizedTime) | ||
}; | ||
}; | ||
}, [enabled]); | ||
return reduceMotion; | ||
} | ||
// src/slider-list.tsx | ||
var import_jsx_runtime4 = require("react/jsx-runtime"); | ||
var getPercentOffsetForSlide = (currentSlide, slideCount, slidesToShow, cellAlign, wrapAround) => { | ||
const renderedSlideCount = wrapAround ? 3 * slideCount : slideCount; | ||
const singleSlidePercentOfWhole = 100 / renderedSlideCount; | ||
let slide0Offset = wrapAround ? -100 / 3 : 0; | ||
if (cellAlign === "right" && slidesToShow > 1) { | ||
const excessSlides = slidesToShow - 1; | ||
slide0Offset += singleSlidePercentOfWhole * excessSlides; | ||
} | ||
if (cellAlign === "center" && slidesToShow > 1) { | ||
const excessSlides = slidesToShow - 1; | ||
const excessLeftSlides = excessSlides / 2; | ||
slide0Offset += singleSlidePercentOfWhole * excessLeftSlides; | ||
} | ||
const currentSlideOffsetFrom0 = 100 / renderedSlideCount * currentSlide; | ||
return slide0Offset - currentSlideOffsetFrom0; | ||
}; | ||
var SliderList = import_react5.default.forwardRef( | ||
({ | ||
animation, | ||
animationDistance, | ||
cellAlign, | ||
children, | ||
currentSlide, | ||
disableAnimation, | ||
disableEdgeSwiping, | ||
draggedOffset, | ||
easing, | ||
edgeEasing, | ||
isDragging, | ||
scrollMode, | ||
slideCount, | ||
slidesToScroll, | ||
slidesToShow, | ||
speed, | ||
wrapAround, | ||
slideWidth, | ||
setIsAnimating | ||
}, forwardedRef) => { | ||
const renderedSlideCount = wrapAround ? 3 * slideCount : slideCount; | ||
const listVisibleWidth = slideWidth ? `calc(${slideWidth} * ${renderedSlideCount})` : `${renderedSlideCount * 100 / slidesToShow}%`; | ||
const percentOffsetForSlideProps = [ | ||
slideCount, | ||
slidesToShow, | ||
cellAlign, | ||
wrapAround | ||
]; | ||
const dotIndexes = getDotIndexes( | ||
slideCount, | ||
slidesToScroll, | ||
scrollMode, | ||
slidesToShow, | ||
wrapAround, | ||
cellAlign | ||
); | ||
let clampedDraggedOffset = `${draggedOffset}px`; | ||
if (isDragging && disableEdgeSwiping && !wrapAround) { | ||
const clampOffsets = [ | ||
dotIndexes[0], | ||
dotIndexes[dotIndexes.length - 1] | ||
].map( | ||
(index) => getPercentOffsetForSlide(index, ...percentOffsetForSlideProps) | ||
); | ||
clampedDraggedOffset = `clamp(${clampOffsets[1]}%, ${draggedOffset}px, ${clampOffsets[0]}%)`; | ||
} | ||
const slideBasedOffset = getPercentOffsetForSlide( | ||
currentSlide, | ||
...percentOffsetForSlideProps | ||
); | ||
const isEdgeEasing = !disableEdgeSwiping && !wrapAround && (currentSlide === dotIndexes[0] && animationDistance < 0 || currentSlide === dotIndexes[dotIndexes.length - 1] && animationDistance > 0); | ||
const { value: transition, isAnimating } = useTween( | ||
speed, | ||
!isEdgeEasing ? easing : edgeEasing, | ||
// animationDistance is assumed to be unique enough that it can be used to | ||
// detect when a new animation should start. This is used in addition to | ||
// currentSlide because some animations, such as those with edgeEasing, do | ||
// not occur due to a change in value of currentSlide | ||
currentSlide + animationDistance, | ||
isDragging || disableAnimation || animation === "fade" | ||
); | ||
let positioning; | ||
if (isDragging || slideBasedOffset !== 0 || isAnimating) { | ||
if (isDragging) { | ||
positioning = `translateX(${clampedDraggedOffset})`; | ||
} else { | ||
const transitionOffset = isAnimating ? (1 - transition) * animationDistance : 0; | ||
positioning = `translateX(calc(${slideBasedOffset}% - ${transitionOffset}px))`; | ||
// src/Carousel/NavButtons.tsx | ||
var import_jsx_runtime = require("react/jsx-runtime"); | ||
function NavButtons() { | ||
const { currentPage, totalPages, wrapMode, goBack, goForward } = useCarousel(); | ||
const allowWrap = wrapMode !== "nowrap"; | ||
const enablePrevNavButton = allowWrap || currentPage > 0; | ||
const enableNextNavButton = allowWrap || currentPage < totalPages - 1; | ||
const prevNavClassName = cls( | ||
"nuka-nav-button", | ||
"nuka-nav-button-prev", | ||
enablePrevNavButton && "nuka-nav-button-enabled" | ||
); | ||
const nextNavClassName = cls( | ||
"nuka-nav-button", | ||
"nuka-nav-button-next", | ||
enableNextNavButton && "nuka-nav-button-enabled" | ||
); | ||
return /* @__PURE__ */ (0, import_jsx_runtime.jsxs)(import_jsx_runtime.Fragment, { children: [ | ||
/* @__PURE__ */ (0, import_jsx_runtime.jsx)("div", { className: prevNavClassName, onClick: goBack, children: /* @__PURE__ */ (0, import_jsx_runtime.jsx)( | ||
"svg", | ||
{ | ||
xmlns: "http://www.w3.org/2000/svg", | ||
viewBox: "0 0 20 20", | ||
fill: "currentcolor", | ||
children: /* @__PURE__ */ (0, import_jsx_runtime.jsx)( | ||
"path", | ||
{ | ||
fillRule: "evenodd", | ||
d: "M11.78 5.22a.75.75 0 0 1 0 1.06L8.06 10l3.72 3.72a.75.75 0 1 1-1.06 1.06l-4.25-4.25a.75.75 0 0 1 0-1.06l4.25-4.25a.75.75 0 0 1 1.06 0Z", | ||
clipRule: "evenodd" | ||
} | ||
) | ||
} | ||
} | ||
(0, import_react5.useEffect)(() => { | ||
setIsAnimating(isAnimating); | ||
}, [isAnimating, setIsAnimating]); | ||
return /* @__PURE__ */ (0, import_jsx_runtime4.jsx)( | ||
"div", | ||
) }), | ||
/* @__PURE__ */ (0, import_jsx_runtime.jsx)("div", { className: nextNavClassName, onClick: goForward, children: /* @__PURE__ */ (0, import_jsx_runtime.jsx)( | ||
"svg", | ||
{ | ||
ref: forwardedRef, | ||
className: "slider-list", | ||
style: { | ||
width: listVisibleWidth, | ||
textAlign: "left", | ||
userSelect: "auto", | ||
transform: positioning, | ||
display: "flex" | ||
}, | ||
children | ||
xmlns: "http://www.w3.org/2000/svg", | ||
viewBox: "0 0 20 20", | ||
fill: "currentcolor", | ||
children: /* @__PURE__ */ (0, import_jsx_runtime.jsx)( | ||
"path", | ||
{ | ||
fillRule: "evenodd", | ||
d: "M8.22 5.22a.75.75 0 0 1 1.06 0l4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.75.75 0 0 1-1.06-1.06L11.94 10 8.22 6.28a.75.75 0 0 1 0-1.06Z", | ||
clipRule: "evenodd" | ||
} | ||
) | ||
} | ||
); | ||
} | ||
); | ||
SliderList.displayName = "SliderList"; | ||
) }) | ||
] }); | ||
} | ||
// src/controls.tsx | ||
var import_react6 = require("react"); | ||
// src/Carousel/PageIndicators.tsx | ||
var import_jsx_runtime2 = require("react/jsx-runtime"); | ||
var PageIndicators = () => { | ||
const { totalPages, currentPage, goToPage } = useCarousel(); | ||
const className = (index) => cls( | ||
"nuka-page-indicator", | ||
currentPage === index ? "nuka-page-indicator-active" : "" | ||
); | ||
return /* @__PURE__ */ (0, import_jsx_runtime2.jsx)("div", { className: "nuka-page-container", "data-testid": "pageIndicatorContainer", children: [...Array(totalPages)].map((_, index) => /* @__PURE__ */ (0, import_jsx_runtime2.jsx)( | ||
"button", | ||
{ | ||
onClick: () => goToPage(index), | ||
className: className(index), | ||
children: /* @__PURE__ */ (0, import_jsx_runtime2.jsx)("span", { className: "nuka-hidden", children: index + 1 }) | ||
}, | ||
index | ||
)) }); | ||
}; | ||
// src/control-styles.ts | ||
var commonStyles = { | ||
position: "absolute", | ||
display: "flex", | ||
zIndex: 1, | ||
top: 0, | ||
left: 0, | ||
bottom: 0, | ||
right: 0 | ||
}; | ||
var getControlContainerFlexStyles = (pos) => { | ||
let alignItems; | ||
switch (pos) { | ||
case "TopLeft" /* TopLeft */: | ||
case "TopCenter" /* TopCenter */: | ||
case "TopRight" /* TopRight */: | ||
alignItems = "flex-start"; | ||
break; | ||
case "CenterLeft" /* CenterLeft */: | ||
case "CenterCenter" /* CenterCenter */: | ||
case "CenterRight" /* CenterRight */: | ||
alignItems = "center"; | ||
break; | ||
case "BottomLeft" /* BottomLeft */: | ||
case "BottomCenter" /* BottomCenter */: | ||
case "BottomRight" /* BottomRight */: | ||
alignItems = "flex-end"; | ||
break; | ||
// #style-inject:#style-inject | ||
function styleInject(css, { insertAt } = {}) { | ||
if (!css || typeof document === "undefined") | ||
return; | ||
const head = document.head || document.getElementsByTagName("head")[0]; | ||
const style = document.createElement("style"); | ||
style.type = "text/css"; | ||
if (insertAt === "top") { | ||
if (head.firstChild) { | ||
head.insertBefore(style, head.firstChild); | ||
} else { | ||
head.appendChild(style); | ||
} | ||
} else { | ||
head.appendChild(style); | ||
} | ||
let justifyContent; | ||
switch (pos) { | ||
case "TopLeft" /* TopLeft */: | ||
case "CenterLeft" /* CenterLeft */: | ||
case "BottomLeft" /* BottomLeft */: | ||
justifyContent = "flex-start"; | ||
break; | ||
case "TopCenter" /* TopCenter */: | ||
case "CenterCenter" /* CenterCenter */: | ||
case "BottomCenter" /* BottomCenter */: | ||
justifyContent = "center"; | ||
break; | ||
case "TopRight" /* TopRight */: | ||
case "CenterRight" /* CenterRight */: | ||
case "BottomRight" /* BottomRight */: | ||
justifyContent = "flex-end"; | ||
break; | ||
if (style.styleSheet) { | ||
style.styleSheet.cssText = css; | ||
} else { | ||
style.appendChild(document.createTextNode(css)); | ||
} | ||
return { alignItems, justifyContent }; | ||
}; | ||
var getControlContainerStyles = (pos) => { | ||
return __spreadValues(__spreadValues({}, getControlContainerFlexStyles(pos)), commonStyles); | ||
}; | ||
} | ||
// src/controls.tsx | ||
var import_jsx_runtime5 = require("react/jsx-runtime"); | ||
var controlsMap = [ | ||
{ funcName: "renderTopLeftControls", key: "TopLeft" /* TopLeft */ }, | ||
{ funcName: "renderTopCenterControls", key: "TopCenter" /* TopCenter */ }, | ||
{ funcName: "renderTopRightControls", key: "TopRight" /* TopRight */ }, | ||
{ funcName: "renderCenterLeftControls", key: "CenterLeft" /* CenterLeft */ }, | ||
{ funcName: "renderCenterCenterControls", key: "CenterCenter" /* CenterCenter */ }, | ||
{ funcName: "renderCenterRightControls", key: "CenterRight" /* CenterRight */ }, | ||
{ funcName: "renderBottomLeftControls", key: "BottomLeft" /* BottomLeft */ }, | ||
{ funcName: "renderBottomCenterControls", key: "BottomCenter" /* BottomCenter */ }, | ||
{ funcName: "renderBottomRightControls", key: "BottomRight" /* BottomRight */ } | ||
]; | ||
var renderControls = (props, slideCount, currentSlide, goToSlide, nextSlide, prevSlide, slidesToScroll) => { | ||
if (props.withoutControls) { | ||
return null; | ||
} | ||
const disableCheckProps = __spreadProps(__spreadValues({}, props), { | ||
currentSlide, | ||
slideCount | ||
}); | ||
const nextDisabled = nextButtonDisabled(disableCheckProps); | ||
const previousDisabled = prevButtonDisabled(disableCheckProps); | ||
const pagingDotsIndices = getDotIndexes( | ||
slideCount, | ||
slidesToScroll, | ||
props.scrollMode, | ||
props.slidesToShow, | ||
props.wrapAround, | ||
props.cellAlign | ||
); | ||
return controlsMap.map((control) => { | ||
var _a; | ||
if (!props[control.funcName] || typeof props[control.funcName] !== "function") { | ||
return /* @__PURE__ */ (0, import_jsx_runtime5.jsx)(import_react6.Fragment, {}, control.funcName); | ||
} | ||
return /* @__PURE__ */ (0, import_jsx_runtime5.jsx)( | ||
"div", | ||
{ | ||
style: __spreadProps(__spreadValues({}, getControlContainerStyles(control.key)), { | ||
pointerEvents: "none" | ||
}), | ||
children: /* @__PURE__ */ (0, import_jsx_runtime5.jsx)( | ||
"div", | ||
{ | ||
className: [ | ||
`slider-control-${control.key.toLowerCase()}`, | ||
props.defaultControlsConfig.containerClassName || "" | ||
].join(" ").trim(), | ||
style: { pointerEvents: "auto" }, | ||
children: (_a = props[control.funcName]) == null ? void 0 : _a.call(props, { | ||
cellAlign: props.cellAlign, | ||
cellSpacing: props.cellSpacing, | ||
currentSlide, | ||
defaultControlsConfig: props.defaultControlsConfig || {}, | ||
carouselId: props.carouselId, | ||
pagingDotsIndices, | ||
goToSlide, | ||
nextDisabled, | ||
nextSlide, | ||
onUserNavigation: props.onUserNavigation, | ||
previousDisabled, | ||
previousSlide: prevSlide, | ||
scrollMode: props.scrollMode, | ||
slideCount, | ||
slidesToScroll, | ||
slidesToShow: props.slidesToShow || 1, | ||
tabbed: props.tabbed, | ||
vertical: props.vertical, | ||
wrapAround: props.wrapAround | ||
}) | ||
} | ||
) | ||
}, | ||
control.funcName | ||
); | ||
}); | ||
}; | ||
var controls_default = renderControls; | ||
// src/Carousel/Carousel.css | ||
styleInject(".nuka-hidden {\n position: absolute;\n width: 1px;\n height: 1px;\n padding: 0;\n margin: -1px;\n overflow: hidden;\n clip: rect(0, 0, 0, 0);\n white-space: nowrap;\n border-width: 0;\n}\n.nuka-container {\n position: relative;\n}\n.nuka-slide-container {\n position: relative;\n}\n.nuka-overflow {\n overflow: scroll;\n scroll-behavior: smooth;\n -ms-overflow-style: none;\n scrollbar-width: none;\n}\n.nuka-overflow::-webkit-scrollbar {\n display: none;\n}\n.nuka-wrapper {\n display: flex;\n}\n.nuka-nav-button {\n position: absolute;\n top: calc(50% - 2rem);\n margin: 1rem;\n display: none;\n height: 2rem;\n width: 2rem;\n cursor: pointer;\n background-color: rgba(146, 148, 151, 0.5);\n color: white;\n border-radius: 9999px;\n font-size: 1rem;\n user-select: none;\n}\n.nuka-nav-button.nuka-nav-button-prev {\n left: 0;\n margin-right: 1rem;\n}\n.nuka-nav-button.nuka-nav-button-next {\n right: 0;\n margin-left: 1rem;\n}\n.nuka-nav-button:hover {\n background-color: rgba(146, 148, 151, 0.65);\n}\n.nuka-nav-button-enabled {\n display: block;\n}\n.nuka-container-auto-hide .nuka-nav-button {\n display: none;\n}\n.nuka-container-auto-hide:hover .nuka-nav-button-enabled {\n display: block;\n}\n.nuka-page-container {\n padding-top: 1rem;\n padding-bottom: 1rem;\n display: flex;\n gap: 0.5rem;\n justify-content: center;\n align-items: center;\n}\n.nuka-page-indicator {\n width: 0.75rem;\n height: 0.75rem;\n cursor: pointer;\n border-radius: 9999px;\n border-style: none;\n background-color: rgba(146, 148, 151, 0.65);\n}\n.nuka-page-indicator.nuka-page-indicator-active,\n.nuka-page-indicator.nuka-page-indicator-active:hover {\n background-color: rgb(229 231 235 / 1);\n}\n.nuka-page-indicator:hover {\n background-color: rgb(229 231 235 / 1);\n}\n"); | ||
// src/default-carousel-props.tsx | ||
var import_jsx_runtime6 = require("react/jsx-runtime"); | ||
var easeOut = (t) => __pow(t - 1, 3) + 1; | ||
var defaultProps = { | ||
adaptiveHeight: false, | ||
adaptiveHeightAnimation: true, | ||
afterSlide: () => { | ||
}, | ||
// src/Carousel/Carousel.tsx | ||
var import_jsx_runtime3 = require("react/jsx-runtime"); | ||
var defaults = { | ||
arrows: /* @__PURE__ */ (0, import_jsx_runtime3.jsx)(NavButtons, {}), | ||
autoplay: false, | ||
autoplayInterval: 3e3, | ||
autoplayReverse: false, | ||
beforeSlide: () => { | ||
}, | ||
cellAlign: "left", | ||
cellSpacing: 0, | ||
defaultControlsConfig: {}, | ||
disableAnimation: false, | ||
disableEdgeSwiping: false, | ||
dragging: true, | ||
dragThreshold: 0.5, | ||
easing: easeOut, | ||
edgeEasing: easeOut, | ||
enableKeyboardControls: false, | ||
frameAriaLabel: "Slider", | ||
keyCodeConfig: { | ||
nextSlide: [39, 68, 38, 87], | ||
previousSlide: [37, 65, 40, 83], | ||
firstSlide: [81], | ||
lastSlide: [69], | ||
pause: [32] | ||
}, | ||
landmark: false, | ||
onDragStart: () => { | ||
}, | ||
onDrag: () => { | ||
}, | ||
onDragEnd: () => { | ||
}, | ||
onUserNavigation: () => { | ||
}, | ||
pauseOnHover: true, | ||
renderAnnounceSlideMessage: defaultRenderAnnounceSlideMessage, | ||
renderBottomCenterControls: (props) => /* @__PURE__ */ (0, import_jsx_runtime6.jsx)(PagingDots, __spreadValues({}, props)), | ||
renderCenterLeftControls: (props) => /* @__PURE__ */ (0, import_jsx_runtime6.jsx)(PreviousButton, __spreadValues({}, props)), | ||
renderCenterRightControls: (props) => /* @__PURE__ */ (0, import_jsx_runtime6.jsx)(NextButton, __spreadValues({}, props)), | ||
scrollMode: "page" /* page */, | ||
slidesToScroll: 1, | ||
slidesToShow: 1, | ||
speed: 500, | ||
style: {}, | ||
dots: /* @__PURE__ */ (0, import_jsx_runtime3.jsx)(PageIndicators, {}), | ||
id: "nuka-carousel", | ||
keyboard: true, | ||
scrollDistance: "screen", | ||
showArrows: false, | ||
showDots: false, | ||
swiping: true, | ||
tabbed: true, | ||
vertical: false, | ||
withoutControls: false, | ||
wrapAround: false, | ||
children: /* @__PURE__ */ (0, import_jsx_runtime6.jsx)(import_jsx_runtime6.Fragment, {}) | ||
wrapMode: "nowrap" | ||
}; | ||
var default_carousel_props_default = defaultProps; | ||
// src/hooks/use-frame-height.ts | ||
var import_react8 = require("react"); | ||
// src/hooks/use-state-with-ref.ts | ||
var import_react7 = require("react"); | ||
var useStateWithRef = (initialState) => { | ||
const [value, setValue] = (0, import_react7.useState)(initialState); | ||
const valueRef = (0, import_react7.useRef)(initialState); | ||
const setValueAndRef = (0, import_react7.useCallback)((newValue) => { | ||
valueRef.current = newValue; | ||
setValue(newValue); | ||
}, []); | ||
return [value, setValueAndRef, valueRef]; | ||
}; | ||
// src/hooks/use-frame-height.ts | ||
var useFrameHeight = (adaptiveHeight, slidesToShow, slideCount) => { | ||
const [visibleHeights, setVisibleHeights, visibleHeightsRef] = useStateWithRef([]); | ||
const [initializedAdaptiveHeight, setInitializedAdaptiveHeight] = (0, import_react8.useState)(false); | ||
const handleVisibleSlideHeightChange = (0, import_react8.useCallback)( | ||
(slideIndex, height) => { | ||
const latestVisibleHeights = visibleHeightsRef.current; | ||
let newVisibleHeights; | ||
if (height === null) { | ||
newVisibleHeights = latestVisibleHeights.filter( | ||
(slideHeight) => slideHeight.slideIndex !== slideIndex | ||
); | ||
} else { | ||
newVisibleHeights = [...latestVisibleHeights, { slideIndex, height }]; | ||
} | ||
setVisibleHeights(newVisibleHeights); | ||
if (newVisibleHeights.length >= Math.min(slideCount, Math.ceil(slidesToShow))) { | ||
setInitializedAdaptiveHeight(true); | ||
} | ||
}, | ||
[slideCount, setVisibleHeights, slidesToShow, visibleHeightsRef] | ||
); | ||
const frameHeight = (0, import_react8.useMemo)(() => { | ||
if (adaptiveHeight) { | ||
if (!initializedAdaptiveHeight) { | ||
return "auto"; | ||
} | ||
const maxHeight = Math.max( | ||
0, | ||
...visibleHeights.map((height) => height.height) | ||
); | ||
return `${maxHeight}px`; | ||
} else { | ||
return "auto"; | ||
} | ||
}, [adaptiveHeight, initializedAdaptiveHeight, visibleHeights]); | ||
return { | ||
handleVisibleSlideHeightChange, | ||
frameHeight, | ||
initializedAdaptiveHeight | ||
}; | ||
}; | ||
// src/hooks/use-forward-ref.ts | ||
var import_react9 = require("react"); | ||
var useForwardRef = (ref) => { | ||
const targetRef = (0, import_react9.useRef)(null); | ||
(0, import_react9.useEffect)(() => { | ||
if (!ref) | ||
return; | ||
if (typeof ref === "function") { | ||
ref(targetRef.current); | ||
} else { | ||
ref.current = targetRef.current; | ||
} | ||
}, [ref]); | ||
return targetRef; | ||
}; | ||
// src/carousel.tsx | ||
var import_jsx_runtime7 = require("react/jsx-runtime"); | ||
var Carousel = import_react10.default.forwardRef( | ||
(rawProps, ref) => { | ||
const props = rawProps; | ||
const internalCarouselId = (0, import_react10.useId)(); | ||
var Carousel = (0, import_react10.forwardRef)( | ||
(props, ref) => { | ||
const options = __spreadValues(__spreadValues({}, defaults), props); | ||
const { | ||
adaptiveHeight, | ||
adaptiveHeightAnimation, | ||
afterSlide, | ||
animation, | ||
arrows, | ||
autoplay, | ||
autoplayInterval, | ||
autoplayReverse, | ||
beforeSlide, | ||
carouselId = internalCarouselId, | ||
cellAlign: propsCellAlign, | ||
cellSpacing, | ||
children, | ||
className, | ||
disableAnimation, | ||
dragging: desktopDraggingEnabled, | ||
dragThreshold: propsDragThreshold, | ||
enableKeyboardControls, | ||
frameAriaLabel, | ||
keyCodeConfig, | ||
landmark, | ||
onDrag, | ||
onDragEnd, | ||
onDragStart, | ||
onUserNavigation, | ||
pauseOnHover, | ||
renderAnnounceSlideMessage, | ||
scrollMode: propsScrollMode, | ||
slideIndex, | ||
slidesToScroll: propsSlidesToScroll, | ||
slidesToShow: propsSlidesToShow, | ||
slideWidth, | ||
speed, | ||
style, | ||
swiping: mobileDraggingEnabled, | ||
tabbed, | ||
wrapAround, | ||
zoomScale | ||
} = props; | ||
const filteredSlides = import_react10.default.Children.toArray(children).filter(Boolean); | ||
const slideCount = filteredSlides.length; | ||
const cellAlign = slideWidth || propsSlidesToScroll === "auto" ? "left" : propsCellAlign; | ||
const scrollMode = propsSlidesToScroll === "auto" ? "remainder" /* remainder */ : propsScrollMode; | ||
const [slideIOEntries, setSlideIOEntries] = (0, import_react10.useState)( | ||
/* @__PURE__ */ new Map() | ||
); | ||
const visibleCount = Array.from(slideIOEntries).filter( | ||
([, visible]) => visible | ||
).length; | ||
const [constantVisibleCount, setConstantVisibleCount] = (0, import_react10.useState)(visibleCount); | ||
const slidesToShow = slideWidth ? constantVisibleCount : propsSlidesToShow; | ||
const slidesToScroll = animation === "fade" ? slidesToShow : propsSlidesToScroll === "auto" ? Math.max(constantVisibleCount, 1) : propsSlidesToScroll; | ||
const [currentSlide, setCurrentSlide] = (0, import_react10.useState)( | ||
() => getDefaultSlideIndex( | ||
slideIndex, | ||
slideCount, | ||
slidesToShow, | ||
slidesToScroll, | ||
cellAlign, | ||
autoplayReverse, | ||
scrollMode | ||
) | ||
); | ||
const [pause, setPause] = (0, import_react10.useState)(false); | ||
const [isDragging, setIsDragging] = (0, import_react10.useState)(false); | ||
const [dragDistance, setDragDistance] = (0, import_react10.useState)(0); | ||
const [animationDistance, setAnimationDistance] = (0, import_react10.useState)(0); | ||
const [isAnimating, setIsAnimating] = (0, import_react10.useState)(false); | ||
const updateSlideIOEntry = (0, import_react10.useCallback)( | ||
(id, isFullyVisible) => { | ||
if (!!slideIOEntries.get(id) === isFullyVisible) | ||
return; | ||
setSlideIOEntries((prev) => { | ||
const newMap = new Map(prev); | ||
newMap.set(id, isFullyVisible); | ||
return newMap; | ||
}); | ||
}, | ||
[slideIOEntries] | ||
); | ||
const prevDragged = (0, import_react10.useRef)(false); | ||
(0, import_react10.useEffect)(() => { | ||
if (isDragging) | ||
prevDragged.current = true; | ||
if (!(isDragging || isAnimating)) { | ||
if (!prevDragged.current) | ||
setConstantVisibleCount(visibleCount); | ||
prevDragged.current = false; | ||
} | ||
}, [isAnimating, isDragging, visibleCount]); | ||
const prevXPosition = (0, import_react10.useRef)(null); | ||
const preDragOffset = (0, import_react10.useRef)(0); | ||
const sliderListRef = (0, import_react10.useRef)(null); | ||
const defaultCarouselRef = (0, import_react10.useRef)(null); | ||
const autoplayTimeout = (0, import_react10.useRef)(); | ||
const autoplayLastTriggeredRef = (0, import_react10.useRef)(null); | ||
const isMounted = (0, import_react10.useRef)(true); | ||
const setSliderListRef = (0, import_react10.useCallback)((node) => { | ||
if (node) { | ||
node.querySelectorAll(".slider-list img").forEach((el) => el.setAttribute("draggable", "false")); | ||
} | ||
sliderListRef.current = node; | ||
}, []); | ||
(0, import_react10.useEffect)(() => { | ||
isMounted.current = true; | ||
return () => { | ||
isMounted.current = false; | ||
}; | ||
}, []); | ||
const forwardedRef = useForwardRef(ref); | ||
const carouselRef = forwardedRef || defaultCarouselRef; | ||
const goToSlide = (0, import_react10.useCallback)( | ||
(targetSlideUnbounded) => { | ||
if (!sliderListRef.current || !carouselRef.current) | ||
return; | ||
const targetSlideBounded = getBoundedIndex( | ||
targetSlideUnbounded, | ||
slideCount | ||
); | ||
const slideChanged = targetSlideUnbounded !== currentSlide; | ||
slideChanged && beforeSlide(currentSlide, targetSlideBounded); | ||
const currentOffset = sliderListRef.current.getBoundingClientRect().left - carouselRef.current.getBoundingClientRect().left; | ||
const sliderWidth = sliderListRef.current.offsetWidth; | ||
let targetOffset = getPercentOffsetForSlide( | ||
targetSlideBounded, | ||
slideCount, | ||
slidesToShow, | ||
cellAlign, | ||
wrapAround | ||
) / 100 * sliderWidth; | ||
if (wrapAround) { | ||
const slideSetWidth = sliderWidth / 3; | ||
if (targetSlideUnbounded < 0) { | ||
targetOffset += slideSetWidth; | ||
} | ||
if (targetSlideUnbounded >= slideCount) { | ||
targetOffset -= slideSetWidth; | ||
} | ||
} | ||
setAnimationDistance(targetOffset - currentOffset); | ||
if (slideChanged) { | ||
setCurrentSlide(targetSlideBounded); | ||
const msToEndOfAnimation = !disableAnimation ? speed || 500 : 40; | ||
setTimeout(() => { | ||
if (!isMounted.current) | ||
return; | ||
afterSlide(targetSlideBounded); | ||
}, msToEndOfAnimation); | ||
} | ||
}, | ||
[ | ||
afterSlide, | ||
beforeSlide, | ||
carouselRef, | ||
cellAlign, | ||
currentSlide, | ||
disableAnimation, | ||
speed, | ||
slideCount, | ||
slidesToShow, | ||
wrapAround | ||
] | ||
); | ||
const nextSlide = (0, import_react10.useCallback)(() => { | ||
const nextSlideIndex = getNextMoveIndex( | ||
scrollMode, | ||
wrapAround, | ||
currentSlide, | ||
slideCount, | ||
slidesToScroll, | ||
slidesToShow, | ||
cellAlign | ||
dots, | ||
id, | ||
keyboard, | ||
scrollDistance, | ||
showArrows, | ||
showDots, | ||
swiping, | ||
title, | ||
wrapMode | ||
} = options; | ||
const carouselRef = (0, import_react10.useRef)(null); | ||
const containerRef = (0, import_react10.useRef)(null); | ||
const { totalPages, scrollOffset } = useMeasurement({ | ||
element: containerRef, | ||
scrollDistance | ||
}); | ||
const { currentPage, goBack, goForward, goToPage } = usePaging({ | ||
totalPages, | ||
wrapMode | ||
}); | ||
const onContainerScroll = useDebounced(() => { | ||
if (!containerRef.current) | ||
return; | ||
const scrollLeft = containerRef.current.scrollLeft; | ||
const closestPageIndex = scrollOffset.indexOf( | ||
nint(scrollOffset, scrollLeft) | ||
); | ||
if (currentSlide !== nextSlideIndex) { | ||
goToSlide(nextSlideIndex); | ||
} | ||
}, [ | ||
cellAlign, | ||
currentSlide, | ||
goToSlide, | ||
slidesToScroll, | ||
scrollMode, | ||
slideCount, | ||
slidesToShow, | ||
wrapAround | ||
goToPage(closestPageIndex); | ||
}, 100); | ||
useKeyboard({ | ||
element: carouselRef, | ||
enabled: keyboard, | ||
goForward, | ||
goBack | ||
}); | ||
(0, import_react10.useImperativeHandle)(ref, () => ({ goForward, goBack, goToPage }), [ | ||
goForward, | ||
goBack, | ||
goToPage | ||
]); | ||
const prevSlide = (0, import_react10.useCallback)(() => { | ||
const prevSlideIndex = getPrevMoveIndex( | ||
scrollMode, | ||
wrapAround, | ||
currentSlide, | ||
slidesToScroll, | ||
slidesToShow, | ||
cellAlign | ||
); | ||
if (currentSlide !== prevSlideIndex) { | ||
goToSlide(prevSlideIndex); | ||
} | ||
}, [ | ||
cellAlign, | ||
currentSlide, | ||
goToSlide, | ||
slidesToScroll, | ||
scrollMode, | ||
slidesToShow, | ||
wrapAround | ||
]); | ||
const prevMovedToSlideIndex = (0, import_react10.useRef)(slideIndex); | ||
const isHovered = useHover({ element: containerRef, enabled: autoplay }); | ||
const prefersReducedMotion = useReducedMotion({ enabled: autoplay }); | ||
const autoplayEnabled = autoplay && !(isHovered || prefersReducedMotion); | ||
useInterval(goForward, autoplayInterval, autoplayEnabled); | ||
(0, import_react10.useEffect)(() => { | ||
if (slideIndex !== void 0 && slideIndex !== prevMovedToSlideIndex.current && !autoplayReverse) { | ||
goToSlide(slideIndex); | ||
prevMovedToSlideIndex.current = slideIndex; | ||
if (containerRef.current) { | ||
beforeSlide && beforeSlide(); | ||
containerRef.current.scrollLeft = scrollOffset[currentPage]; | ||
afterSlide && setTimeout(() => afterSlide(), 0); | ||
} | ||
}, [slideIndex, autoplayReverse, goToSlide]); | ||
(0, import_react10.useEffect)(() => { | ||
let pauseStarted = null; | ||
if (pause) { | ||
pauseStarted = Date.now(); | ||
} | ||
return () => { | ||
if (pauseStarted !== null && autoplayLastTriggeredRef.current !== null) { | ||
autoplayLastTriggeredRef.current += Date.now() - pauseStarted; | ||
} | ||
}; | ||
}, [pause]); | ||
(0, import_react10.useEffect)(() => { | ||
if (autoplay && !pause) { | ||
const adjustedTimeoutMs = autoplayLastTriggeredRef.current !== null ? autoplayInterval - (Date.now() - autoplayLastTriggeredRef.current) : autoplayInterval; | ||
autoplayTimeout.current = setTimeout(() => { | ||
autoplayLastTriggeredRef.current = Date.now(); | ||
if (autoplayReverse) { | ||
prevSlide(); | ||
} else { | ||
nextSlide(); | ||
} | ||
}, adjustedTimeoutMs); | ||
} | ||
if (autoplay && pause) { | ||
clearTimeout(autoplayTimeout.current); | ||
} | ||
return () => { | ||
clearTimeout(autoplayTimeout.current); | ||
}; | ||
}, [ | ||
pause, | ||
autoplay, | ||
autoplayInterval, | ||
autoplayReverse, | ||
prevSlide, | ||
nextSlide | ||
]); | ||
const onKeyDown = (event) => { | ||
let keyCommand = null; | ||
Object.keys(keyCodeConfig).forEach( | ||
(command) => { | ||
var _a; | ||
if ((_a = keyCodeConfig[command]) == null ? void 0 : _a.includes(event.keyCode)) { | ||
keyCommand = command; | ||
} | ||
} | ||
); | ||
if (keyCommand === null) | ||
return; | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
switch (keyCommand) { | ||
case "nextSlide": | ||
onUserNavigation(event); | ||
nextSlide(); | ||
break; | ||
case "previousSlide": | ||
onUserNavigation(event); | ||
prevSlide(); | ||
break; | ||
case "firstSlide": | ||
case "lastSlide": { | ||
onUserNavigation(event); | ||
const dotIndices = getDotIndexes( | ||
slideCount, | ||
slidesToScroll, | ||
scrollMode, | ||
slidesToShow, | ||
wrapAround, | ||
cellAlign | ||
); | ||
if (keyCommand === "firstSlide") { | ||
goToSlide(dotIndices[0]); | ||
} else { | ||
goToSlide(dotIndices[dotIndices.length - 1]); | ||
} | ||
break; | ||
} | ||
case "pause": | ||
setPause((p) => !p); | ||
break; | ||
} | ||
}; | ||
const dragPositions = (0, import_react10.useRef)([]); | ||
const handleDragEnd = (e) => { | ||
if (!isDragging || !carouselRef.current) | ||
return; | ||
setIsDragging(false); | ||
let distanceFromInertia = 0; | ||
if (dragPositions.current.length > 1) { | ||
const startMove = dragPositions.current[0]; | ||
const endMove = dragPositions.current[dragPositions.current.length - 1]; | ||
const timeOffset = endMove.time - startMove.time; | ||
const goodInertiaFeelConstant = 9; | ||
const goodFrictionFeelConstant = 0.92; | ||
const initialVelocity = goodInertiaFeelConstant * Math.abs((endMove.pos - startMove.pos) / timeOffset); | ||
let velocity = initialVelocity; | ||
while (Math.abs(velocity) > 1) { | ||
distanceFromInertia += velocity; | ||
velocity *= goodFrictionFeelConstant; | ||
} | ||
} | ||
dragPositions.current = []; | ||
const adjustedDragDistance = Math.abs(dragDistance) + Math.abs(distanceFromInertia); | ||
onDragEnd(e); | ||
prevXPosition.current = null; | ||
setDragDistance(0); | ||
const oneScrollWidth = carouselRef.current.offsetWidth * Math.min(1, slidesToScroll / slidesToShow); | ||
const dragThreshold = oneScrollWidth * propsDragThreshold; | ||
if (adjustedDragDistance < dragThreshold) { | ||
goToSlide(currentSlide); | ||
return; | ||
} | ||
const canMaintainVisualContinuity = slidesToShow >= 2 * slidesToScroll; | ||
const timesToMove = canMaintainVisualContinuity ? 1 + Math.floor((adjustedDragDistance - dragThreshold) / oneScrollWidth) : 1; | ||
let nextSlideIndex = currentSlide; | ||
for (let index = 0; index < timesToMove; index += 1) { | ||
if (dragDistance > 0) { | ||
nextSlideIndex = getNextMoveIndex( | ||
scrollMode, | ||
wrapAround, | ||
nextSlideIndex, | ||
slideCount, | ||
slidesToScroll, | ||
slidesToShow, | ||
cellAlign | ||
); | ||
} else { | ||
nextSlideIndex = getPrevMoveIndex( | ||
scrollMode, | ||
wrapAround, | ||
nextSlideIndex, | ||
slidesToScroll, | ||
slidesToShow, | ||
cellAlign | ||
); | ||
} | ||
} | ||
if (nextSlideIndex !== currentSlide) { | ||
onUserNavigation(e); | ||
} | ||
goToSlide(nextSlideIndex); | ||
}; | ||
const onTouchStart = (0, import_react10.useCallback)( | ||
(e) => { | ||
if (!mobileDraggingEnabled || !sliderListRef.current || !carouselRef.current) { | ||
return; | ||
} | ||
setIsDragging(true); | ||
preDragOffset.current = sliderListRef.current.getBoundingClientRect().left - carouselRef.current.getBoundingClientRect().left; | ||
onDragStart(e); | ||
}, | ||
[carouselRef, onDragStart, mobileDraggingEnabled] | ||
}, [currentPage, scrollOffset, beforeSlide, afterSlide]); | ||
const containerClassName = cls( | ||
"nuka-container", | ||
showArrows === "hover" && "nuka-container-auto-hide", | ||
className | ||
); | ||
const handlePointerMove = (0, import_react10.useCallback)( | ||
(xPosition) => { | ||
if (!isDragging) | ||
return; | ||
const isFirstMove = prevXPosition.current === null; | ||
const delta = prevXPosition.current !== null ? xPosition - prevXPosition.current : 0; | ||
const nextDragDistance = dragDistance + delta; | ||
const now = Date.now(); | ||
while (dragPositions.current.length > 0) { | ||
if (now - dragPositions.current[0].time <= 100) { | ||
break; | ||
} | ||
dragPositions.current.shift(); | ||
} | ||
dragPositions.current.push({ pos: nextDragDistance, time: now }); | ||
if (!isFirstMove) { | ||
setDragDistance(nextDragDistance); | ||
} | ||
prevXPosition.current = xPosition; | ||
}, | ||
[isDragging, dragDistance] | ||
); | ||
const onTouchMove = (0, import_react10.useCallback)( | ||
(e) => { | ||
if (!isDragging || !carouselRef.current) | ||
return; | ||
onDragStart(e); | ||
const moveValue = carouselRef.current.offsetWidth - e.touches[0].pageX; | ||
handlePointerMove(moveValue); | ||
}, | ||
[isDragging, carouselRef, handlePointerMove, onDragStart] | ||
); | ||
const onMouseDown = (0, import_react10.useCallback)( | ||
(e) => { | ||
if (!desktopDraggingEnabled || !sliderListRef.current || !carouselRef.current) | ||
return; | ||
setIsDragging(true); | ||
preDragOffset.current = sliderListRef.current.getBoundingClientRect().left - carouselRef.current.getBoundingClientRect().left; | ||
onDragStart(e); | ||
}, | ||
[carouselRef, desktopDraggingEnabled, onDragStart] | ||
); | ||
const onMouseMove = (0, import_react10.useCallback)( | ||
(e) => { | ||
if (!isDragging || !carouselRef.current) | ||
return; | ||
onDrag(e); | ||
const offsetX = e.clientX - carouselRef.current.getBoundingClientRect().left; | ||
const moveValue = carouselRef.current.offsetWidth - offsetX; | ||
handlePointerMove(moveValue); | ||
}, | ||
[carouselRef, isDragging, handlePointerMove, onDrag] | ||
); | ||
const onMouseUp = (e) => { | ||
e.preventDefault(); | ||
handleDragEnd(e); | ||
}; | ||
const onMouseEnter = (0, import_react10.useCallback)(() => { | ||
if (pauseOnHover) { | ||
setPause(true); | ||
} | ||
}, [pauseOnHover]); | ||
const onMouseLeave = (0, import_react10.useCallback)(() => { | ||
if (pauseOnHover) { | ||
setPause(false); | ||
} | ||
}, [pauseOnHover]); | ||
const { | ||
frameHeight, | ||
handleVisibleSlideHeightChange, | ||
initializedAdaptiveHeight | ||
} = useFrameHeight(adaptiveHeight, slidesToShow, slideCount); | ||
const renderSlides = (typeOfSlide) => { | ||
const slides = filteredSlides.map((child, index) => { | ||
return /* @__PURE__ */ (0, import_jsx_runtime7.jsx)( | ||
slide_default, | ||
{ | ||
id: `${typeOfSlide}-${index}`, | ||
carouselId, | ||
count: slideCount, | ||
index, | ||
isCurrentSlide: currentSlide === index, | ||
typeOfSlide, | ||
wrapAround, | ||
cellSpacing, | ||
animation, | ||
speed, | ||
zoomScale, | ||
onVisibleSlideHeightChange: handleVisibleSlideHeightChange, | ||
slideWidth, | ||
updateIOEntry: updateSlideIOEntry, | ||
adaptiveHeight, | ||
initializedAdaptiveHeight, | ||
carouselRef, | ||
tabbed, | ||
children: child | ||
}, | ||
`${typeOfSlide}-${index}` | ||
); | ||
}); | ||
return slides; | ||
}; | ||
return /* @__PURE__ */ (0, import_jsx_runtime7.jsxs)( | ||
"div", | ||
{ | ||
className: "slider-container", | ||
style: { | ||
position: "relative" | ||
}, | ||
onMouseEnter, | ||
onMouseLeave, | ||
"aria-label": frameAriaLabel, | ||
role: landmark ? "region" : "group", | ||
"aria-roledescription": "carousel", | ||
id: carouselId, | ||
"data-testid": carouselId, | ||
children: [ | ||
/* @__PURE__ */ (0, import_jsx_runtime7.jsx)( | ||
announce_slide_default, | ||
{ | ||
ariaLive: autoplay && !pause ? "off" : "polite", | ||
message: renderAnnounceSlideMessage({ | ||
currentSlide, | ||
count: slideCount | ||
}) | ||
} | ||
), | ||
controls_default( | ||
__spreadProps(__spreadValues({}, props), { carouselId }), | ||
slideCount, | ||
currentSlide, | ||
goToSlide, | ||
nextSlide, | ||
prevSlide, | ||
slidesToScroll | ||
), | ||
/* @__PURE__ */ (0, import_jsx_runtime7.jsx)( | ||
"div", | ||
{ | ||
className: ["slider-frame", className || ""].join(" ").trim(), | ||
style: __spreadValues({ | ||
overflow: "hidden", | ||
width: "100%", | ||
position: "relative", | ||
outline: "none", | ||
touchAction: "pan-y", | ||
height: frameHeight, | ||
transition: adaptiveHeightAnimation ? "height 300ms ease-in-out" : void 0, | ||
willChange: "height", | ||
userSelect: "none" | ||
}, style), | ||
tabIndex: enableKeyboardControls ? 0 : -1, | ||
onKeyDown: enableKeyboardControls ? onKeyDown : void 0, | ||
ref: carouselRef, | ||
onMouseUp, | ||
onMouseDown, | ||
onMouseMove, | ||
onMouseLeave: onMouseUp, | ||
onTouchStart, | ||
onTouchEnd: handleDragEnd, | ||
onTouchMove, | ||
id: `${carouselId}-slider-frame`, | ||
"data-testid": `${carouselId}-slider-frame`, | ||
children: /* @__PURE__ */ (0, import_jsx_runtime7.jsxs)( | ||
SliderList, | ||
const providerValues = __spreadProps(__spreadValues({}, options), { | ||
totalPages, | ||
currentPage, | ||
scrollOffset, | ||
goBack, | ||
goForward, | ||
goToPage | ||
}); | ||
return /* @__PURE__ */ (0, import_jsx_runtime3.jsxs)(CarouselProvider, { value: providerValues, children: [ | ||
/* @__PURE__ */ (0, import_jsx_runtime3.jsxs)( | ||
"div", | ||
{ | ||
className: containerClassName, | ||
"aria-labelledby": "nuka-carousel-heading", | ||
tabIndex: keyboard ? 0 : void 0, | ||
ref: carouselRef, | ||
id, | ||
children: [ | ||
title && /* @__PURE__ */ (0, import_jsx_runtime3.jsx)("h3", { id: "nuka-carousel-heading", className: "nuka-hidden", children: title }), | ||
/* @__PURE__ */ (0, import_jsx_runtime3.jsxs)("div", { className: "nuka-slide-container", children: [ | ||
/* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"div", | ||
{ | ||
animationDistance, | ||
cellAlign, | ||
currentSlide, | ||
disableEdgeSwiping: props.disableEdgeSwiping, | ||
draggedOffset: preDragOffset.current - dragDistance, | ||
disableAnimation, | ||
easing: props.easing, | ||
edgeEasing: props.edgeEasing, | ||
isDragging, | ||
ref: setSliderListRef, | ||
scrollMode, | ||
animation, | ||
slideCount, | ||
slidesToScroll, | ||
slidesToShow, | ||
speed, | ||
slideWidth, | ||
wrapAround, | ||
setIsAnimating, | ||
children: [ | ||
wrapAround ? renderSlides("prev-cloned") : null, | ||
renderSlides(), | ||
wrapAround ? renderSlides("next-cloned") : null | ||
] | ||
className: "nuka-overflow", | ||
ref: containerRef, | ||
onTouchMove: onContainerScroll, | ||
id: "nuka-overflow", | ||
"data-testid": "nuka-overflow", | ||
style: { touchAction: swiping ? "pan-x" : "none" }, | ||
children: /* @__PURE__ */ (0, import_jsx_runtime3.jsx)( | ||
"div", | ||
{ | ||
className: "nuka-wrapper", | ||
id: "nuka-wrapper", | ||
"data-testid": "nuka-wrapper", | ||
children | ||
} | ||
) | ||
} | ||
) | ||
} | ||
) | ||
] | ||
} | ||
); | ||
), | ||
showArrows && arrows | ||
] }) | ||
] | ||
} | ||
), | ||
showDots && dots | ||
] }); | ||
} | ||
); | ||
Carousel.defaultProps = default_carousel_props_default; | ||
Carousel.displayName = "Carousel"; | ||
// Annotate the CommonJS export names for ESM import in node: | ||
0 && (module.exports = { | ||
Alignment, | ||
Directions, | ||
NextButton, | ||
PagingDots, | ||
Positions, | ||
PreviousButton, | ||
ScrollMode | ||
Carousel, | ||
CarouselProvider, | ||
useCarousel | ||
}); | ||
//# sourceMappingURL=index.js.map |
{ | ||
"name": "nuka-carousel", | ||
"version": "7.0.0", | ||
"version": "8.0.0", | ||
"description": "Pure React Carousel", | ||
@@ -8,16 +8,14 @@ "main": "dist/index.js", | ||
"types": "dist/index.d.ts", | ||
"engines": { | ||
"node": ">=12.0.0" | ||
}, | ||
"devDependencies": { | ||
"@babel/core": "^7.17.2", | ||
"@babel/core": "^7.24.3", | ||
"@storybook/addon-actions": "^7.4.6", | ||
"@storybook/addon-essentials": "^7.4.6", | ||
"@storybook/addon-interactions": "^7.6.17", | ||
"@storybook/addon-links": "^7.4.6", | ||
"@storybook/jest": "^0.2.3", | ||
"@storybook/react": "^7.4.6", | ||
"@storybook/react-webpack5": "^7.4.6", | ||
"@storybook/testing-library": "^0.2.2", | ||
"@testing-library/jest-dom": "^5.16.5", | ||
"@testing-library/react": "^13.3.0", | ||
"@types/d3-ease": "^3.0.0", | ||
"@types/exenv": "^1.2.0", | ||
"@types/jest-axe": "^3.5.6", | ||
@@ -30,18 +28,14 @@ "@types/node": "^18.7.5", | ||
"chromatic": "^6.2.0", | ||
"d3-ease": "^3.0.1", | ||
"exenv": "^1.2.0", | ||
"jest": "^29.7.0", | ||
"jest-axe": "^8.0.0", | ||
"jest-environment-jsdom": "^28.1.3", | ||
"prop-types": "^15.6.0", | ||
"react": "^18.0.0", | ||
"react-dom": "^18.0.0", | ||
"react-move": "^6.5.0", | ||
"require-from-string": "^2.0.2", | ||
"storybook": "^7.4.6", | ||
"tsup": "^6.7.0", | ||
"typescript": "^4.5.2", | ||
"url-loader": "^4.1.1", | ||
"webpack": "5.61.0", | ||
"webpack-cli": "^4.9.1", | ||
"webpack-dev-server": "^4.6.0" | ||
"tsup": "^8.0.2", | ||
"typescript": "^5.4.2", | ||
"webpack": "^5.91.0", | ||
"webpack-cli": "^5.1.4", | ||
"webpack-dev-server": "^5.0.4" | ||
}, | ||
@@ -70,3 +64,3 @@ "peerDependencies": { | ||
"homepage": "https://github.com/FormidableLabs/nuka-carousel", | ||
"sideEffects": false, | ||
"sideEffects": true, | ||
"publishConfig": { | ||
@@ -85,9 +79,8 @@ "provenance": true | ||
"preversion": "pnpm run check", | ||
"test": "pnpm run test:unit && pnpm run test:e2e", | ||
"test:ci": "pnpm run test:unit:ci && pnpm run test:e2e:ci", | ||
"test:unit": "jest --testPathIgnorePatterns=\\(/cypress /es /lib\\)", | ||
"test": "pnpm run test:unit", | ||
"test:ci": "pnpm run test:unit:ci", | ||
"test:unit": "jest --testPathIgnorePatterns=\\(/es /lib\\)", | ||
"test:unit:ci": "pnpm run test:unit", | ||
"test:unit:watch": "pnpm run test:unit --watchAll", | ||
"test:e2e": "cypress open", | ||
"test:e2e:ci": "cypress run", | ||
"test:storybook": "test-storybook", | ||
"package": "pnpm pack", | ||
@@ -94,0 +87,0 @@ "storybook": "storybook dev -p 6006", |
@@ -1,2 +0,2 @@ | ||
[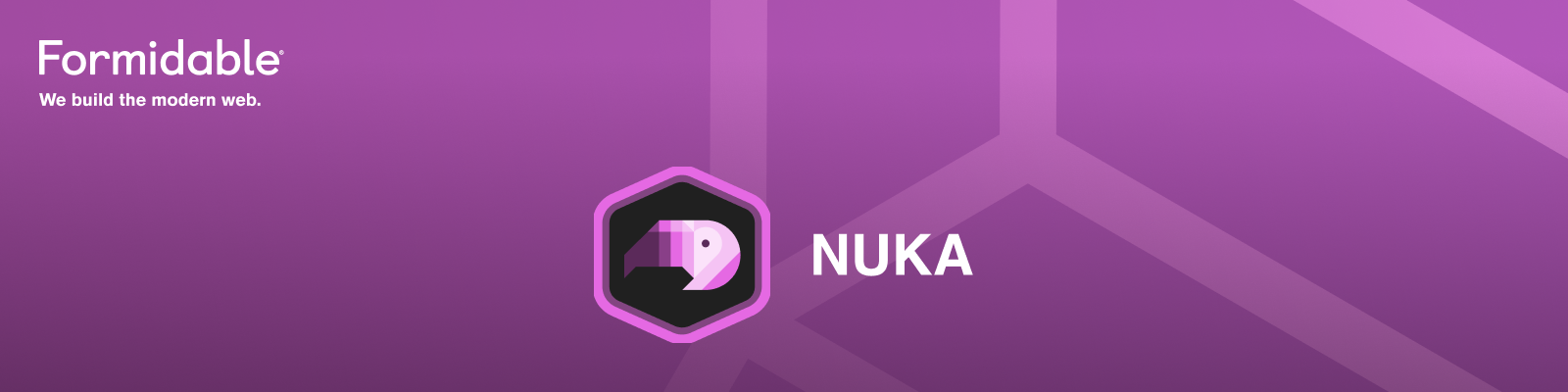](https://formidable.com/open-source/nuka-carousel) | ||
[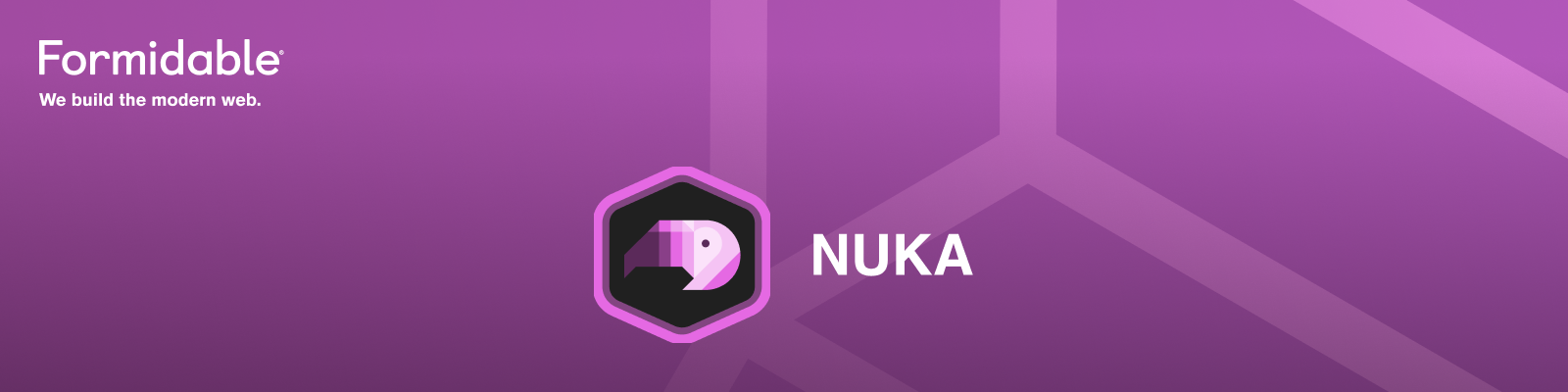](https://commerce.nearform.com/open-source/nuka-carousel) | ||
@@ -18,7 +18,7 @@ [![Maintenance Status][maintenance-image]](#maintenance-status) | ||
Come learn more and see a live demo at our [docs site](https://formidable.com/open-source/nuka-carousel)! | ||
Come learn more and see a live demo at our [docs site](https://commerce.nearform.com/open-source/nuka-carousel)! | ||
## Support | ||
Have a question about react-live? Submit an issue in this repository using the | ||
Have a question about nuka-carousel? Submit an issue in this repository using the | ||
["Question" template](https://github.com/FormidableLabs/nuka-carousel/issues/new?template=question.md). | ||
@@ -37,4 +37,4 @@ | ||
**Active:** Formidable is actively working on this project, and we expect to continue for work for the foreseeable future. Bug reports, feature requests and pull requests are welcome. | ||
**Active:** Nearform is actively working on this project, and we expect to continue for work for the foreseeable future. Bug reports, feature requests and pull requests are welcome. | ||
[maintenance-image]: https://img.shields.io/badge/maintenance-active-green.svg?color=brightgreen&style=flat |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Long strings
Supply chain riskContains long string literals, which may be a sign of obfuscated or packed code.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
30
9
103449
1045
2
1