nuxt-security
Advanced tools
Comparing version 0.8.0 to 0.9.0
import * as _nuxt_schema from '@nuxt/schema'; | ||
import { CorsOptions } from '@nozomuikuta/h3-cors'; | ||
declare type RequestSizeLimiter = { | ||
type RequestSizeLimiter = { | ||
maxRequestSizeInBytes: number; | ||
maxUploadFileRequestInBytes: number; | ||
}; | ||
declare type RateLimiter = { | ||
type RateLimiter = { | ||
tokensPerInterval: number; | ||
@@ -13,3 +13,3 @@ interval: string | number; | ||
}; | ||
declare type XssValidator = { | ||
type XssValidator = { | ||
whiteList: Record<string, any>; | ||
@@ -20,3 +20,3 @@ stripIgnoreTag: boolean; | ||
} | {}; | ||
declare type BasicAuth = { | ||
type BasicAuth = { | ||
name: string; | ||
@@ -27,30 +27,73 @@ pass: string; | ||
}; | ||
declare type HTTPMethod = 'GET' | 'POST' | 'DELETE' | 'PATCH' | 'POST' | string; | ||
declare type AllowedHTTPMethods = HTTPMethod[] | '*'; | ||
declare type MiddlewareConfiguration<MIDDLEWARE> = { | ||
type HTTPMethod = 'GET' | 'POST' | 'DELETE' | 'PATCH' | 'POST' | string; | ||
type AllowedHTTPMethods = HTTPMethod[] | '*'; | ||
type MiddlewareConfiguration<MIDDLEWARE> = { | ||
value: MIDDLEWARE; | ||
route: string; | ||
}; | ||
declare type SecurityHeaders = { | ||
crossOriginResourcePolicy: MiddlewareConfiguration<string> | boolean; | ||
crossOriginOpenerPolicy: MiddlewareConfiguration<string> | boolean; | ||
crossOriginEmbedderPolicy: MiddlewareConfiguration<string> | boolean; | ||
contentSecurityPolicy: MiddlewareConfiguration<string> | boolean; | ||
originAgentCluster: MiddlewareConfiguration<string> | boolean; | ||
referrerPolicy: MiddlewareConfiguration<string> | boolean; | ||
strictTransportSecurity: MiddlewareConfiguration<string> | boolean; | ||
xContentTypeOptions: MiddlewareConfiguration<string> | boolean; | ||
xDNSPrefetchControl: MiddlewareConfiguration<string> | boolean; | ||
xDownloadOptions: MiddlewareConfiguration<string> | boolean; | ||
xFrameOptions: MiddlewareConfiguration<string> | boolean; | ||
xPermittedCrossDomainPolicies: MiddlewareConfiguration<string> | boolean; | ||
xXSSProtection: MiddlewareConfiguration<number> | boolean; | ||
type CrossOriginResourcePolicyValue = 'same-site' | 'same-origin' | 'cross-origin'; | ||
type CrossOriginOpenerPolicyValue = 'unsafe-none' | 'same-origin-allow-popups' | 'same-origin'; | ||
type CrossOriginEmbedderPolicyValue = 'unsafe-none' | 'require-corp'; | ||
type ReferrerPolicyValue = 'no-referrer' | 'no-referrer-when-downgrade' | 'origin' | 'origin-when-cross-origin' | 'same-origin' | 'strict-origin' | 'strict-origin-when-cross-origin' | 'unsafe-url'; | ||
type XContentTypeOptionsValue = 'nosniff'; | ||
type XDnsPrefetchControlValue = 'on' | 'off'; | ||
type XDownloadOptionsValue = 'noopen'; | ||
type XFrameOptionsValue = 'DENY' | 'SAMEORIGIN'; | ||
type XPermittedCrossDomainPoliciesValue = 'none' | 'master-only' | 'by-content-type' | 'by-ftp-filename' | 'all'; | ||
type CSPSourceValue = "'self'" | "'unsafe-eval'" | "'wasm-unsafe-eval'" | "'unsafe-hashes'" | "'unsafe-inline'" | "'none'" | "'strict-dynamic'" | "'report-sample'" | string; | ||
type CSPSandboxValue = 'allow-downloads' | 'allow-downloads-without-user-activation' | 'allow-forms' | 'allow-modals' | 'allow-orientation-lock' | 'allow-pointer-lock' | 'allow-popups' | 'allow-popups-to-escape-sandbox' | 'allow-presentation' | 'allow-same-origin' | 'allow-scripts' | 'allow-storage-access-by-user-activation' | 'allow-top-navigation' | 'allow-top-navigation-by-user-activation' | 'allow-top-navigation-to-custom-protocols'; | ||
type ContentSecurityPolicyValue = { | ||
'child-src'?: CSPSourceValue[]; | ||
'connect-src'?: CSPSourceValue[]; | ||
'default-src'?: CSPSourceValue[]; | ||
'font-src'?: CSPSourceValue[]; | ||
'frame-src'?: CSPSourceValue[]; | ||
'img-src'?: CSPSourceValue[]; | ||
'manifest-src'?: CSPSourceValue[]; | ||
'media-src'?: CSPSourceValue[]; | ||
'object-src'?: CSPSourceValue[]; | ||
'prefetch-src'?: CSPSourceValue[]; | ||
'script-src'?: CSPSourceValue[]; | ||
'script-src-elem'?: CSPSourceValue[]; | ||
'script-src-attr'?: CSPSourceValue[]; | ||
'style-src'?: CSPSourceValue[]; | ||
'style-src-elem'?: CSPSourceValue[]; | ||
'style-src-attr'?: CSPSourceValue[]; | ||
'worker-src'?: CSPSourceValue[]; | ||
'base-uri'?: CSPSourceValue[]; | ||
'sandbox'?: CSPSandboxValue[]; | ||
'form-action'?: CSPSourceValue[]; | ||
'frame-ancestors'?: ("'self'" | "'none'" | string)[]; | ||
'navigate-to'?: ("'self'" | "'none'" | "'unsafe-allow-redirects'" | string)[]; | ||
'report-uri'?: string[]; | ||
'report-to'?: string[]; | ||
'upgrade-insecure-requests'?: boolean; | ||
}; | ||
type StrictTransportSecurityValue = { | ||
maxAge: number; | ||
includeSubdomains?: boolean; | ||
preload?: boolean; | ||
}; | ||
type SecurityHeaders = { | ||
crossOriginResourcePolicy?: MiddlewareConfiguration<CrossOriginResourcePolicyValue> | false; | ||
crossOriginOpenerPolicy?: MiddlewareConfiguration<CrossOriginOpenerPolicyValue> | false; | ||
crossOriginEmbedderPolicy?: MiddlewareConfiguration<CrossOriginEmbedderPolicyValue> | false; | ||
contentSecurityPolicy?: MiddlewareConfiguration<ContentSecurityPolicyValue | string> | false; | ||
originAgentCluster?: MiddlewareConfiguration<'?1'> | false; | ||
referrerPolicy?: MiddlewareConfiguration<ReferrerPolicyValue> | false; | ||
strictTransportSecurity?: MiddlewareConfiguration<StrictTransportSecurityValue | string> | false; | ||
xContentTypeOptions?: MiddlewareConfiguration<XContentTypeOptionsValue> | false; | ||
xDNSPrefetchControl?: MiddlewareConfiguration<XDnsPrefetchControlValue> | false; | ||
xDownloadOptions?: MiddlewareConfiguration<XDownloadOptionsValue> | false; | ||
xFrameOptions?: MiddlewareConfiguration<XFrameOptionsValue> | false; | ||
xPermittedCrossDomainPolicies?: MiddlewareConfiguration<XPermittedCrossDomainPoliciesValue> | false; | ||
xXSSProtection?: MiddlewareConfiguration<string> | false; | ||
}; | ||
interface ModuleOptions { | ||
headers: SecurityHeaders | boolean; | ||
requestSizeLimiter: MiddlewareConfiguration<RequestSizeLimiter> | boolean; | ||
rateLimiter: MiddlewareConfiguration<RateLimiter> | boolean; | ||
xssValidator: MiddlewareConfiguration<XssValidator> | boolean; | ||
corsHandler: MiddlewareConfiguration<CorsOptions> | boolean; | ||
allowedMethodsRestricter: MiddlewareConfiguration<AllowedHTTPMethods> | boolean; | ||
headers: SecurityHeaders | false; | ||
requestSizeLimiter: MiddlewareConfiguration<RequestSizeLimiter> | false; | ||
rateLimiter: MiddlewareConfiguration<RateLimiter> | false; | ||
xssValidator: MiddlewareConfiguration<XssValidator> | false; | ||
corsHandler: MiddlewareConfiguration<CorsOptions> | false; | ||
allowedMethodsRestricter: MiddlewareConfiguration<AllowedHTTPMethods> | false; | ||
hidePoweredBy: boolean; | ||
@@ -57,0 +100,0 @@ basicAuth: MiddlewareConfiguration<BasicAuth> | boolean; |
{ | ||
"name": "nuxt-security", | ||
"configKey": "security", | ||
"version": "0.8.0" | ||
"version": "0.9.0" | ||
} |
@@ -1,2 +0,2 @@ | ||
export declare type BasicAuth = { | ||
export type BasicAuth = { | ||
name: string; | ||
@@ -3,0 +3,0 @@ pass: string; |
@@ -1,2 +0,2 @@ | ||
declare const _default: import("h3").EventHandler<unknown>; | ||
declare const _default: import("h3").EventHandler<void>; | ||
export default _default; |
{ | ||
"name": "nuxt-security", | ||
"version": "0.8.0", | ||
"version": "0.9.0", | ||
"license": "MIT", | ||
"type": "module", | ||
"homepage": "https://nuxt-security.vercel.app", | ||
"description": "🛡 Security Module for Nuxt based on OWASP Top 10 and Helmet", | ||
"repository": { | ||
@@ -15,3 +16,7 @@ "url": "https://github.com/Baroshem/nuxt-security" | ||
"owasp", | ||
"helmet" | ||
"helmet", | ||
"basic-auth", | ||
"rate-limit", | ||
"xss", | ||
"cors" | ||
], | ||
@@ -41,4 +46,6 @@ "exports": { | ||
"basic-auth": "^2.0.1", | ||
"defu": "^6.1.1", | ||
"limiter": "^2.1.0", | ||
"memory-cache": "^0.2.0", | ||
"pathe": "^1.0.0", | ||
"xss": "^1.0.14" | ||
@@ -50,2 +57,3 @@ }, | ||
"@nuxtjs/eslint-config-typescript": "latest", | ||
"@types/memory-cache": "^0.2.2", | ||
"eslint": "latest", | ||
@@ -52,0 +60,0 @@ "nuxt": "^3.0.0" |
@@ -84,4 +84,4 @@ [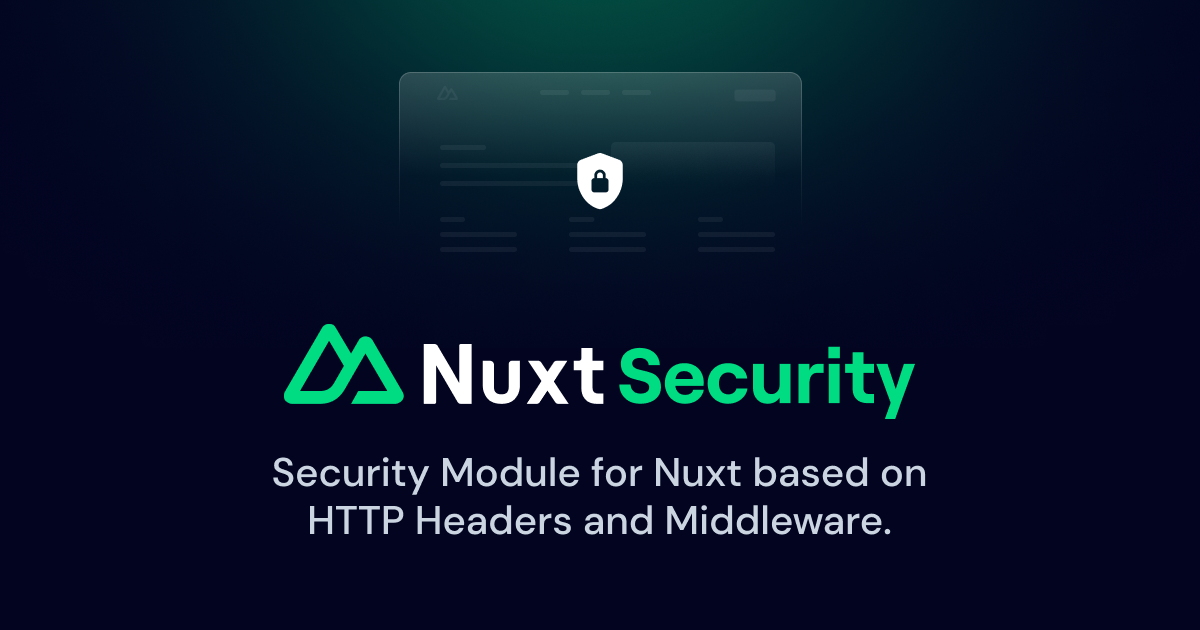](https://nuxt-security.vercel.app) | ||
- Run `npm run dev:prepare` to generate type stubs. | ||
- Use `npm run dev` to start playground in development mode. | ||
- Run `yarn dev:prepare` to generate type stubs. | ||
- Use `yarn dev` to start playground in development mode. | ||
@@ -88,0 +88,0 @@ ## License |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
23792
8
6
461
+ Addeddefu@^6.1.1
+ Addedpathe@^1.0.0