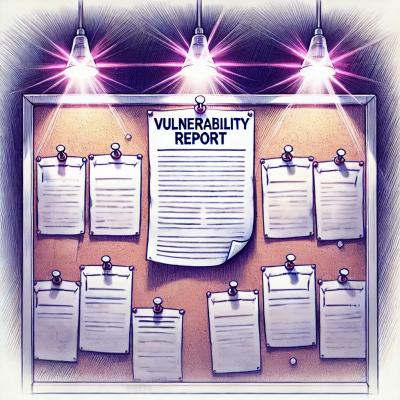
Security News
libxml2 Maintainer Ends Embargoed Vulnerability Reports, Citing Unsustainable Burden
Libxml2’s solo maintainer drops embargoed security fixes, highlighting the burden on unpaid volunteers who keep critical open source software secure.
objects-to-csv-delimited
Advanced tools
Converts an array of objects into a CSV file. Saves CSV to disk or returns as string.
https://github.com/anton-bot/objects-to-csv
In this version you can pass a delimiter when instanciating the module:
const ObjectToCsv = require('objects-to-csv-delimited')
const sample = [
{index : '0', text : 'line 1'},
{index : '1', text : 'line 2'},
{index : '2', text : 'line 3'}
]
new ObjectToCsv(sample,{
delimiter : ';'
}).toDisk('./sample.csv')
A comma will be used as default if nothing is passed in the second parameter.
Converts an array of JavaScript objects into the CSV format. You can save the CSV to file or return it as a string.
The keys in the first object of the array will be used as column names.
Any special characters in the values (such as commas) will be properly escaped.
const ObjectsToCsv = require('objects-to-csv');
// Sample data - two columns, three rows:
const data = [
{code: 'CA', name: 'California'},
{code: 'TX', name: 'Texas'},
{code: 'NY', name: 'New York'},
];
// If you use "await", code must be inside an asynchronous function:
(async () => {
const csv = new ObjectsToCsv(data);
// Save to file:
await csv.toDisk('./test.csv');
// Return the CSV file as string:
console.log(await csv.toString());
})();
There are two methods, toDisk(filename)
and toString()
.
Converts the data and saves the CSV file to disk. The filename
must include the
path as well.
The options
is an optional parameter which is an object that contains the
settings. Supported options:
append
- whether to append to the file. Default is false
(overwrite the file).
Set to true
to append. Column names will be added only once at the beginning
of the file. If the file does not exist, it will be created.bom
- whether to add the Unicode Byte Order Mark at the beginning of the
file. Default is false
; set to true
to be able to view Unicode in Excel
properly. Otherwise Excel will display Unicode incorrectly.allColumns
- whether to check all array items for keys to convert to columns rather
than only the first. This will sort the columns alphabetically. Default is false
;
set to true
to check all items for potential column names.const ObjectsToCsv = require('objects-to-csv');
const sampleData = [{ id: 1, text: 'this is a test' }];
// Run asynchronously, without awaiting:
new ObjectsToCsv(sampleData).toDisk('./test.csv');
// Alternatively, you can append to the existing file:
new ObjectsToCsv(sampleData).toDisk('./test.csv', { append: true });
// `allColumns: true` collects column names from all objects in the array,
// instead of only using the first one. In this case the CSV file will
// contain three columns:
const mixedData = [
{ id: 1, name: 'California' },
{ id: 2, description: 'A long description.' },
];
new ObjectsToCsv(mixedData).toDisk('./test.csv', { allColumns: true });
Returns the CSV file as a string.
Two optional parameters are available:
header
controls whether the column names will be
returned as the first row of the file. Default is true
. Set it to false
to
get only the data rows, without the column names.allColumns
controls whether to check every item for potential keys to process,
rather than only the first item; this will sort the columns alphabetically by key name.
Default is false
. Set it to true
to process keys that may not be present
in the first object of the array.const ObjectsToCsv = require('objects-to-csv');
const sampleData = [{ id: 1, text: 'this is a test' }];
async function printCsv(data) {
console.log(
await new ObjectsToCsv(data).toString()
);
}
printCsv(sampleData);
Use Node.js version 8 or above.
OBS.: This modified version was tested using Node.js 14
FAQs
Converts an array of objects into a CSV file. Saves CSV to disk or returns as string.
The npm package objects-to-csv-delimited receives a total of 792 weekly downloads. As such, objects-to-csv-delimited popularity was classified as not popular.
We found that objects-to-csv-delimited demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Libxml2’s solo maintainer drops embargoed security fixes, highlighting the burden on unpaid volunteers who keep critical open source software secure.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.