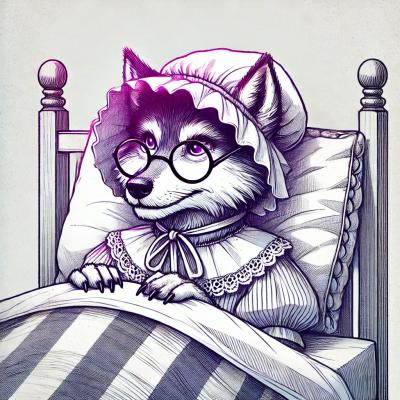
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The 'once' npm package is a utility that allows you to ensure a function can only be called once. It is useful for preventing duplicate initialization, handling setup tasks that should only run a single time, or ensuring a callback is only executed once in response to an event or the resolution of a Promise.
Ensuring a function is only called once
This feature is used to wrap a function so that it can only be executed once. Subsequent calls to the function will have no effect, and the original return value will be returned.
const once = require('once');
const myFunction = once(() => {
console.log('This will only be logged once.');
});
myFunction(); // logs 'This will only be logged once.'
myFunction(); // does nothing
This is a function from the Lodash library that ensures a given function can only be called once. It is similar to 'once' but comes as part of the larger Lodash utility library, which includes a wide range of functions for different purposes.
Memoizee is a library for memoizing functions, which can also be used to ensure a function is only called once by caching the result of the first call. It is more complex and feature-rich than 'once', offering fine-grained control over cache management and function memoization.
Only call a function once.
var once = require('once')
function load (file, cb) {
cb = once(cb)
loader.load('file')
loader.once('load', cb)
loader.once('error', cb)
}
Or add to the Function.prototype in a responsible way:
// only has to be done once
require('once').proto()
function load (file, cb) {
cb = cb.once()
loader.load('file')
loader.once('load', cb)
loader.once('error', cb)
}
Ironically, the prototype feature makes this module twice as complicated as necessary.
FAQs
Run a function exactly one time
The npm package once receives a total of 35,944,616 weekly downloads. As such, once popularity was classified as popular.
We found that once demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.