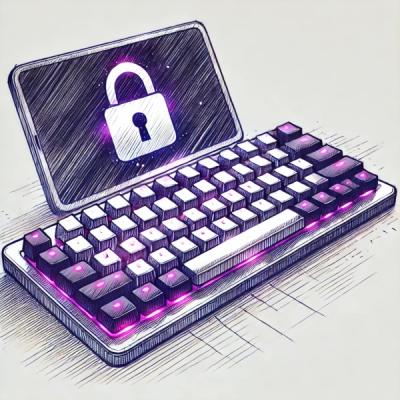
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
papi
Advanced tools
Readme
This is a module for building HTTP API clients.
Initialize a new client.
Your client should inherit the prototype methods from this constructor and call it in your client's constructor.
Options
_log
callsUsage
var papi = require('papi');
var util = require('util');
function GitHub(opts) {
opts = opts || {};
opts.baseUrl = 'https://api.github.com';
opts.header = { accept: 'application/vnd.github.v3+json' };
opts.timeout = 15 * 1000;
papi.Client.call(this, opts);
}
util.inherits(GitHub, papi.Client);
Make an HTTP request.
Your client should use this or the shortcut methods listed below to execute HTTP requests in your client methods.
Arguments
request.err
or request.res
. Call next
without arguments to continue execution, next(err)
to break with an error, or next(false, arguments...)
to trigger the final callback with the given arguments.Request
/user/{id}
)cancel
to abort request_log
callsThere are also _get
, _head
, _post
, _put
, _delete
(_del
), _patch
,
and _options
shortcuts with the same method signature as _request
.
Usage
GitHub.prototype.gists = function(username, callback) {
var opts = {
path: '/users/{username}/gists',
params: { username: username },
};
this._get(opts, function(err, res) {
if (err) return callback(err);
callback(null, res.body);
});
};
Result
statusCode 200
body [ { url: 'https://api.github.com/gists/9458207',
...
Emit log events.
Arguments
Usage
client.on('log', function(tags) {
console.log({
tags: tags,
data: Array.prototype.slice.call(arguments, 1),
});
});;
client._log(['github', 'gist'], 'silas');
Result
{ data: [ 'silas' ], tags: [ 'debug', 'github', 'gist' ] }
Register an extension function.
Arguments
Usage
client._ext('onRequest', function(request, next) {
console.log('request', request.opts.method + ' ' + request.opts.path);
request.start = new Date();
next();
});
client._ext('onResponse', function(request, next) {
var duration = new Date() - request.start;
var statusCode = request.res ? request.res.statusCode : 'none';
console.log('response', request.opts.method, request.opts.path, statusCode, duration + 'ms');
next();
});
Result
request GET /users/{username}/gists
response GET /users/{username}/gists 200 1141ms
Register a plugin.
Arguments
Usage
client._plugin(require('papi-retry'));
Shortcuts for making one-off requests.
See client request for full options list, with the exception
that path
is replaced with url
.
Request
http://example.org/
)There are also get
, head
, post
, put
, delete
(del
), patch
, and
options
shortcuts with the same method signature as request
.
Usage
var papi = require('papi');
papi.get('https://api.github.com/users/silas/gists', function(err, res) {
if (err) throw err;
res.body.forEach(function(gist) {
console.log(gist.url);
});
});
/**
* Module dependencies.
*/
var papi = require('papi');
var util = require('util');
/**
* GitHub API client
*/
function GitHub(opts) {
opts = opts || {};
if (!opts.baseUrl) {
opts.baseUrl = 'https://api.github.com';
}
if (!opts.headers) {
opts.headers = {};
}
if (!opts.headers.accept) {
opts.headers.accept = 'application/vnd.github.v3+json';
}
if (!opts.headers['user-agent']) {
opts.headers['user-agent'] = 'PapiGitHub/0.1.0';
}
if (opts.tags) {
opts.tags = ['github'].concat(opts.tags);
} else {
opts.tags = ['github'];
}
if (!opts.timeout) {
opts.timeout = 60 * 1000;
}
papi.Client.call(this, opts);
if (opts.debug) {
this.on('log', console.log);
}
}
util.inherits(GitHub, papi.Client);
/**
* Get user gists
*/
GitHub.prototype.gists = function(username, callback) {
var opts = {
path: '/users/{username}/gists',
params: { username: username },
};
return this._get(opts, callback);
};
/**
* Print gists for user `silas`
*/
function main() {
var github = new GitHub({ debug: true });
github.gists('silas', function(err, res) {
if (err) throw err;
console.log('----');
res.body.forEach(function(gist) {
if (gist.description) console.log(gist.description);
});
});
}
/**
* Initialize
*/
if (require.main === module) {
main();
} else {
module.exports = GitHub;
}
This work is licensed under the MIT License (see the LICENSE file).
FAQs
Unknown package
We found that papi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.