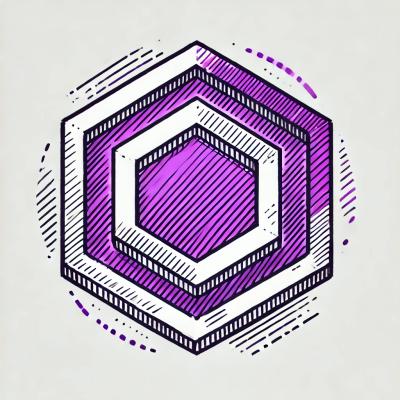
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
permit-fe-sdk
Advanced tools
This package lets you easily integrate Permit.io advanced permissions into your frontend application. It is integrated with CASL and so can be used with any frontend framework.
npm install permit-fe-sdk
yarn add permit-fe-sdk
demo_server
folder for a sample server configuration.If you're working with a different frontend framework, consult the CASL documentation. It provides guidance on importing data into CASL ability. This SDK can then help generate the necessary data for CASL.
You can create a react component called an AbilityLoader
to handle this:
import { Ability } from '@casl/ability';
import { Permit, permitState } from 'permit-fe-sdk';
const getAbility = async (loggedInUser) => {
const permit = Permit({
loggedInUser: loggedInUser, // This is the unique userId from your authentication provider in the current session.
backendUrl: '/api/your-endpoint',
});
await permit.loadLocalState([
{ action: 'view', resource: 'statement' },
{ action: 'view', resource: 'products' },
{ action: 'delete', resource: 'file' },
{ action: 'create', resource: 'document' },
]);
const caslConfig = permitState.getCaslJson();
return caslConfig && caslConfig.length ? new Ability(caslConfig) : undefined;
};
If needed, the SDK allows you to pass custom headers to the backend. This can be useful for passing authentication tokens or other necessary information.
const permit = Permit({
loggedInUser: loggedInUser,
backendUrl: '/api/your-endpoint',
customRequestHeaders: {
Authorization: 'Bearer your-token',
});
loadLocalStateBulk
When working with access control in your application, it's crucial to understand the differences between various policy models: Role-Based Access Control (RBAC), Attribute-Based Access Control (ABAC), and Relationship-Based Access Control (ReBAC). Each of these models has its own way of determining permissions, which affects how you should configure and pass data to the loadLocalStateBulk function in your application.
The loadLocalStateBulk
function allows you to load multiple permission checks at once, optimizing the performance of your application by reducing the number of backend calls. Below is a general example demonstrating how different access control models can be integrated into a single bulk request.
await permit.loadLocalStateBulk([
// RBAC example
{ action: 'view', resource: 'statement' },
{ action: 'view', resource: 'products' },
{ action: 'delete', resource: 'file' },
// ABAC example
{
action: 'view',
resource: 'files_for_poland_employees',
userAttributes: {
department: 'Engineering',
salary: '100K',
},
resourceAttributes: { country: 'PL' },
},
// ReBAC example
{ action: 'create', resource: 'document:my_file.doc' },
]);
Let's break this down into smaller policy-based chunks:
RBAC assigns permissions to users based on their roles within an organization. This is a straightforward model where access rights are predetermined by the user's job function.
loadLocalStateBulk
For RBAC, you typically pass the action and resource without any additional attributes:
await permit.loadLocalStateBulk([
{ action: 'view', resource: 'statement' },
{ action: 'view', resource: 'products' },
{ action: 'delete', resource: 'file' },
]);
In this example:
action
represents the type of operation (e.g., 'view', 'delete').resource
represents the object being acted upon (e.g., 'file', 'products').ABAC is more dynamic than RBAC. It grants access based on user attributes, resource attributes, and environment conditions. This model is particularly useful when access control needs to be fine-grained.
For ABAC, you include additional userAttributes and resourceAttributes to specify the conditions under which access is granted:
await permit.loadLocalStateBulk([
{
action: 'view',
resource: 'files_for_poland_employees',
userAttributes: {
department: 'Engineering',
salary: '100K',
},
resourceAttributes: { country: 'PL' },
},
]);
In this example:
userAttributes
defines attributes associated with the user, such as department or salary.resourceAttributes
specifies attributes tied to the resource, like the country of operation.ReBAC (Relationship-Based Access Control) determines access permissions based on the relationships between users and resources. This model is particularly useful when permissions need to reflect complex relationships, such as group memberships or ownership of specific resources.
loadLocalStateBulk
In ReBAC, you not only specify the action and the resource but also include a resource instance key to identify the specific instance of the resource that the relationship pertains to. This key is essential to precisely define the access control based on the user's relationship to that particular instance.
For example, consider a document management system where permissions are defined based on whether a user is the owner of a document or a member of a group that has access to it.
await permit.loadLocalStateBulk([
{
action: 'create',
resource: 'document:my_file.doc', // `document` is the resource type, `my_file.doc` is the resource instance key
},
]);
In this example:
action
specifies the operation to be performed (e.g., 'create').resource
consists of two parts:
Resource type
(e.g., 'document')Resource instance key
(e.g., 'my_file.doc')The resource instance key (my_file.doc
in this case) identifies the specific document that the user is allowed to create or manage based on their relationship with it.
To check permissions based on relationships in ReBAC, you can use the permit.check function. This function checks whether a user has the necessary relationship to perform an action on a resource instance.
For example, to check if a user is a member of a specific group that has access to a resource:
permit.check(userId, action, {
type: 'member_group', // Specifies the relationship type
key: group, // Specifies the particular group key
});
Or, using shorthand object notation:
permit.check(userId, action, `member_group:${group}`);
By including the resource instance key and defining relationships precisely, ReBAC enables fine-grained control over who can perform what actions on specific resource instances based on their relationship with those resources.
The flexibility of the loadLocalStateBulk function allows you to mix different access control models in a single request. This can be particularly powerful in applications that require a combination of role-based, attribute-based, and relationship-based access controls.
Here is how you can combine all three models in one bulk call:
await permit.loadLocalStateBulk([
// RBAC examples
{ action: 'view', resource: 'statement' },
{ action: 'view', resource: 'products' },
{ action: 'delete', resource: 'file' },
// ABAC example
{
action: 'view',
resource: 'files_for_poland_employees',
userAttributes: {
department: 'Engineering',
salary: '100K',
},
resourceAttributes: { country: 'PL' },
},
// ReBAC example
{ action: 'create', resource: 'document:my_file.doc' },
]);
By passing a structured array to loadLocalStateBulk
, you can efficiently manage permissions across different models without needing separate function calls for each model type.
After performing the necessary policy checks, the next step is to ensure that the current user is authenticated. Once confirmed, you should assign the abilities returned from the checks to the user. This allows your application to enforce the correct permissions based on the user's access rights.
if (isSignedIn) {
getAbility(user.id).then((caslAbility) => {
setAbility(caslAbility);
});
}
If you would like to see how the normal or bulk local states should be handled in your API - refer to the demo_server
folder for a sample server configuration.
For any questions, please reach out to us in the Permit community.
FAQs
## Overview
The npm package permit-fe-sdk receives a total of 592 weekly downloads. As such, permit-fe-sdk popularity was classified as not popular.
We found that permit-fe-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.