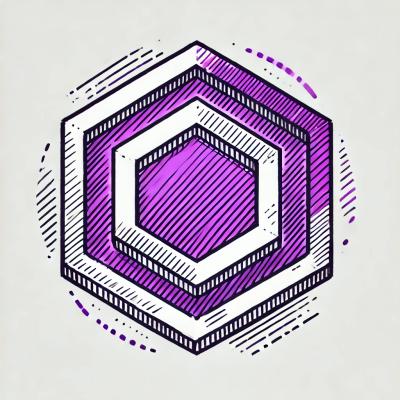
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
playwright-chromium
Advanced tools
The playwright-chromium npm package is a Node library that provides a high-level API to automate Chromium-based browsers. It is part of the Playwright project, which is designed for end-to-end testing and web scraping. The package allows you to control a browser, navigate web pages, interact with elements, and capture screenshots, among other functionalities.
Browser Automation
This feature allows you to automate browser actions such as navigating to a URL, taking screenshots, and closing the browser.
const { chromium } = require('playwright-chromium');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
Web Scraping
This feature allows you to scrape data from web pages by navigating to a URL and extracting information using DOM selectors.
const { chromium } = require('playwright-chromium');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const data = await page.evaluate(() => document.querySelector('h1').innerText);
console.log(data);
await browser.close();
})();
Form Submission
This feature allows you to automate form submissions by filling out input fields and clicking buttons.
const { chromium } = require('playwright-chromium');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com/login');
await page.fill('#username', 'myUsername');
await page.fill('#password', 'myPassword');
await page.click('button[type="submit"]');
await browser.close();
})();
Testing
This feature allows you to perform end-to-end testing by navigating to a URL, performing actions, and making assertions.
const { chromium } = require('playwright-chromium');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const title = await page.title();
console.assert(title === 'Example Domain', 'Title is incorrect');
await browser.close();
})();
Puppeteer is a Node library that provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It is similar to playwright-chromium in terms of browser automation and web scraping capabilities. However, Playwright offers broader browser support and more advanced features for handling multiple contexts and parallelism.
Selenium WebDriver is a popular tool for automating web applications for testing purposes. It supports multiple browsers and programming languages. Compared to playwright-chromium, Selenium is more mature but may lack some of the modern features and performance optimizations found in Playwright.
Cypress is a JavaScript end-to-end testing framework that focuses on making the testing experience more developer-friendly. It provides a rich set of features for testing modern web applications. While Cypress is easier to set up and use, playwright-chromium offers more flexibility and broader browser support.
This package contains the Chromium flavor of the Playwright library. If you want to write end-to-end tests, we recommend @playwright/test.
FAQs
A high-level API to automate Chromium
The npm package playwright-chromium receives a total of 96,930 weekly downloads. As such, playwright-chromium popularity was classified as popular.
We found that playwright-chromium demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.