What is plist?
The plist npm package is a library for Node.js that allows users to parse and build XML Property List (plist) data. Property lists are a structured file format used primarily by macOS and iOS for storing serialized objects. The plist package provides functionality to convert between plist files and JavaScript objects, making it useful for tasks such as configuration management, data serialization, and interacting with Apple ecosystem data formats.
What are plist's main functionalities?
Parsing plist files
This feature allows you to read and parse plist files into JavaScript objects. The code sample demonstrates how to read a plist file asynchronously and parse its content.
const plist = require('plist');
const fs = require('fs');
fs.readFile('example.plist', 'utf8', function(err, data) {
if (err) throw err;
const parsedData = plist.parse(data);
console.log(parsedData);
});
Building plist files
This feature allows you to create plist files from JavaScript objects. The code sample shows how to convert an object into plist format and then write it to a file.
const plist = require('plist');
const fs = require('fs');
const obj = {
key1: 'value1',
key2: 'value2'
};
const plistContent = plist.build(obj);
fs.writeFile('example.plist', plistContent, function(err) {
if (err) throw err;
console.log('Plist file has been saved!');
});
Other packages similar to plist
simple-plist
simple-plist is another npm package that provides similar functionality to plist. It allows for reading and writing plist files. Compared to plist, simple-plist may offer a simpler API and potentially fewer features, focusing on basic plist operations.
bplist-parser
bplist-parser is a package specifically designed to parse binary plist files. While plist handles both XML and binary formats, bplist-parser is optimized for binary plists, which might make it faster or more efficient for that specific use case.
plist.js
Apple's Property list parser/builder for Node.js and browsers

Provides facilities for reading and writing Plist (property list) files.
These are often used in programming OS X and iOS applications, as well
as the iTunes configuration XML file.
Plist files represent stored programming "object"s. They are very similar
to JSON. A valid Plist file is representable as a native JavaScript Object
and vice-versa.
Usage
Node.js
Install using npm
:
$ npm install --save plist
Then require()
the plist module in your file:
var plist = require('plist');
var val = plist.parse('<plist><string>Hello World!</string></plist>');
console.log(val);
Browser
Include the dist/plist.js
in a <script>
tag in your HTML file:
<script src="plist.js"></script>
<script>
var val = plist.parse('<plist><string>Hello World!</string></plist>');
console.log(val);
</script>
API
Parsing
Parsing a plist from filename:
var fs = require('fs');
var plist = require('plist');
var obj = plist.parse(fs.readFileSync('myPlist.plist', 'utf8'));
console.log(JSON.stringify(obj));
Parsing a plist from string payload:
var plist = require('plist');
var xml =
'<?xml version="1.0" encoding="UTF-8"?>' +
'<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">' +
'<plist version="1.0">' +
'<key>metadata</key>' +
'<dict>' +
'<key>bundle-identifier</key>' +
'<string>com.company.app</string>' +
'<key>bundle-version</key>' +
'<string>0.1.1</string>' +
'<key>kind</key>' +
'<string>software</string>' +
'<key>title</key>' +
'<string>AppName</string>' +
'</dict>' +
'</plist>';
console.log(plist.parse(xml));
Building
Given an existing JavaScript Object, you can turn it into an XML document
that complies with the plist DTD:
var plist = require('plist');
var json = [
"metadata",
{
"bundle-identifier": "com.company.app",
"bundle-version": "0.1.1",
"kind": "software",
"title": "AppName"
}
];
console.log(plist.build(json));
Cross Platform Testing Credits
Much thanks to Sauce Labs for providing free resources that enable cross-browser testing on this project!
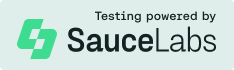
License
(The MIT License)