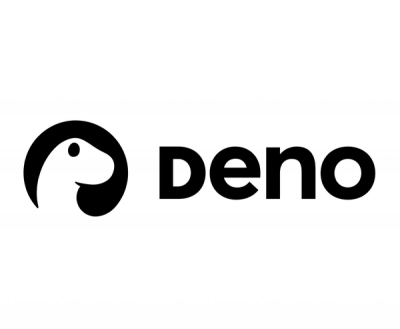
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
poloniex-api-node
Advanced tools
poloniex-api-node is a simple node.js wrapper for Poloniex REST and WebSocket API.
See detailed GitHub Releases
Legacy Poloniex API is no longer supported. Poloniex has announced that the legacy API is slated to be decommissioned on Jan 31st, 2023. Module supporting the legacy API has been moved to branch legacy_API, and it is not longer maintained.
npm install --save poloniex-api-node
new Poloniex({ apiKey, apiSecret })
To access the private Poloniex API methods you must supply your API key id and key secret. If you are only accessing the public API endpoints, you can leave the parameter out.
Examples:
const poloniex = new Poloniex({ apiKey: 'myKey', apiSecret: 'mySecret' })
apiCallRateLimits
- API call rate limits for endpoints sets (resource-intensive and non-resource-intensive private and public endpoints)
Example output:
{
riPub: 10,
nriPub: 200,
nriPriv: 50,
riPriv: 10
}
apiCallRates
- Current call rate for resource-intensive and non-resource-intensive private and public API calls
Example output:
{
riPub: 8,
nriPub: 100,
nriPriv: 5,
riPriv: 7
}
See https://docs.poloniex.com/#rate-limits.
All methods accept one object parameter, which is used to pass all request parameters for the API call.
Official Poloniex API documentation lists all valid Request Parameters for API calls. When a Request Parameter needs to be included, a property with the exact same name needs to be added to the object parameter.
All methods return a promise.
Example:
import Poloniex from 'poloniex-api-node'
let poloniex = new Poloniex({ apiKey: 'myKey', apiSecret: 'mySecret' })
const tradesHistory = poloniex.getTradesHistory({ limit: 1000, symbols: 'BTC_USDT' })
.then((result) => console.log(result))
.catch((err) => console.log(err))
An optional property getApiCallRateInfo
can be specified. When set to true
the corresponding API call is not sent to the exchange, instead the current API call rate is returned. See rate limits.
Example:
const callRateInfo = poloniex.getTradesHistory({ limit: 1000, symbols: 'BTC_USDT', getApiCallRateInfo: true })
Example output:
[
'riPriv', // id of the API endpoind set (can be 'riPub', 'nriPub', 'riPriv' or 'nriPriv')
2, // current API call rate
10 // API call rate limit
]
getSymbols
- get symbols and their trade infogetCurrencies
- get supported currenciesgetTimestamp
- get current server timegetPrices
- get the latest trade price for symbolsgetMarkPrice
- get the latest mark price for cross margin symbolsgetMarkPriceComponents
- get components of the mark price for a given symbolgetOrderBook
- get the order book for a given symbolgetCandles
- returns OHLC for a symbol at given timeframe (interval)getTrades
- returns a list of recent tradesgetTicker
- returns ticker in last 24 hours for all symbolsgetCollateralInfo
- get collateral information for currenciesgetBorrowRatesInfo
- get borrow rates information for all tiers and currenciesgetAccountsInfo
- get a list of all accounts of a usegetAccountsBalances
- get a list of accounts of a user with each account’s id, type and balancesgetAccountsActivity
- get a list of activities such as airdrop, rebates, staking, credit/debit adjustments, and other (historical adjustments)accountsTransfer
- transfer amount of currency from an account to another account for a usergetAccountsTransferRecords
- get a list of transfer records of a usergetFeeInfo
- get fee rategetSubaccountsInfo
- get a list of all the accounts within an Account Group for a usergetSubaccountsBalances
- get balances information by currency and account typesubaccountsTransfer
- transfer amount of currency from an account and account type to another account and account type among the accounts in the account groupgetSubaccountsTransferRecords
- get a list of transfer records of a usergetDepositAddresses
- get deposit addresses for a usergetWalletsActivityRecords
- get deposit and withdrawal activity historycreateNewCurrencyAddress
- Create a new address for a currencywithdrawCurrency
- immediately places a withdrawal for a given currencygetMarginAccountInfo
- get account margin informationgetMarginBorrowStatus
- get borrow status of currenciesgetMarginMaxSize
- get maximum and available buy/sell amount for a given symbolcreateOrder
- create an order for an accountcreateBatchOrders
- create multiple orders via a single requestreplaceOrder
- cancel an existing active order, new or partially filled, and place a new ordergetOpenOrders
- get a list of active orders for an accountgetOrderDetails
- get an order’s statuscancelOrder
- cancel an active ordercancelBatchOrders
- batch cancel one or many active orders in an account by IDscancelAllOrders
- cancel all orders in an accountsetKillSwitch
- set a timer that cancels all regular and smartorders after the timeout has expiredgetKillSwitchStatus
- get status of kill switchcreateSmartOrder
- create a smart order for an accountreplaceSmartOrder
- cancel an existing untriggered smart order and place a new smart ordergetSmartOpenOrders
- get a list of (pending) smart orders for an accountgetSmartOrderDetails
- get a smart order’s statuscancelSmartOrder
- cancel a smart ordercancelBatchSmartOrders
- batch cancel one or many smart orders in an account by IDscancelAllSmartOrders
- batch cancel all smart orders in an accountgetOrdersHistory
- get a list of historical orders in an accountgetSmartOrdersHistory
- get a list of historical smart orders in an accountgetTradesHistory
- get a list of all trades for an accountgetOrderTrades
- get a list of all trades for an order specified by its orderIdThe module uses forever-websocket to connect to Poloniex websocket server.
ForeverWebSocket
extends WebSocket
, and in additions supports:
newPublicWebSocket(options)
- establishes a WebSocket connection for public channelsnewAuthenticatedWebSocket(options)
- establishes a WebSocket connection for authenticated channelsoptions
parameter properties (check ForeverWebSocket
for additional explanations):
Property name | Type | Attributes | Default | Description |
---|---|---|---|---|
reconnect | object or null | optional | { factor: 1.5, initialDelay: 50, maxDelay: 10000, randomizeDelay: false } | Reconnecting parameters. Defaults to exponential backoff strategy |
timeout | number | optional | no timeout | Timeout in milliseconds after which the websockets reconnects when no messages are received |
newPublicWebSocket()
and newAuthenticatedWebSocket()
return a ForeverWebSocket
instance.
Ping function is activated by default, ping requests are issued every 30 seconds to keep the connection alive.
{
"event": "ping"
}
See https://docs.poloniex.com/#public-channels
Example:
import Poloniex from 'poloniex-api-node'
const poloniex = new Poloniex()
// Create a new WebSocket connected to public endpoint. The WebSocket should not reconnect if disconnected.
const ws = poloniex.newPublicWebSocket({ reconnect: null })
// Specify event handlers
ws.on('open', () => console.log('Websocket connection open'))
ws.on('message', (data) => console.log('Websocket data received'))
ws.on('error', () => console.log('Websocket error'))
ws.on('close', () => console.log('Websocket connection closed'))
See https://docs.poloniex.com/#authenticated-channels
When newAuthenticatedWebSocket()
is used to open a WebSocket connection to access private channels, the authentication auth
message is automatically sent to Poloniex immediately after the WebSocket connection is open.
Subscriptions for authentication channels can be sent after poloniex server responds with successful authentication confirmation:
{
"data": {
"success": true,
"ts": 1645597033915
},
"channel": "auth"
}
Example:
import Poloniex from 'poloniex-api-node'
const poloniex = new Poloniex({ apiKey: 'myKey', apiSecret: 'mySecret' })
// Create a new WebSocket connected to private endpoint.
const ws = poloniex.newAuthenticatedWebSocket()
// Specify event handlers
ws.on('open', () => console.log('Websocket connection open'))
ws.on('message', (data) => {
// if 'auth` message is received and authentication is sucessfull, send subscribe message
if (data.channel === 'auth' && data.data.success) {
ws.send({
event: 'subscribe',
channel: ['orders'],
symbols: ['all']
})
}
})
This project exists thanks to all the people who contribute.
FAQs
Simple node.js wrapper for Poloniex REST and WebSocket API.
We found that poloniex-api-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.