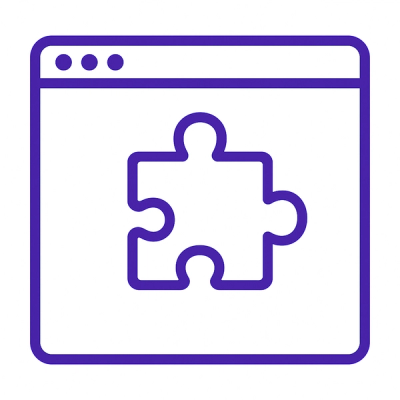
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
pouchdb-upsert
Advanced tools
A tiny plugin for PouchDB that provides two convenience methods:
upsert()
- update a document, or insert a new one if it doesn't exist ("upsert"). Will keep retrying (forever) if it gets 409 conflicts.putIfNotExists()
- create a new document if it doesn't exist. Does nothing if it already exists.So basically, if you're tired of manually dealing with 409s or 404s in your PouchDB code, then this is the plugin for you.
bower install pouchdb-upsert
Or download from the dist/
folder and include it after pouchdb.js
:
<script src="pouchdb.js"></script>
<script src="pouchdb.upsert.js"></script>
npm install pouchdb-upsert
Then attach it to the PouchDB
object:
var PouchDB = require('pouchdb');
PouchDB.plugin(require('pouchdb-upsert'));
Perform an upsert (update or insert) operation. If you don't specify a callback
, then this function returns a Promise.
docId
- the _id
of the document.diffFunc
- function that takes the existing doc as input and returns an updated doc.
diffFunc
returns falsey, then the update won't be performed (as an optimization).{}
will be the input to diffFunc
.Note: By design, the goal of this repo is to just provide a handler for synchronized logic. diffFunc
must not make asynchronous calls.
A doc with a basic counter:
db.upsert('myDocId', function (doc) {
if (!doc.count) {
doc.count = 0;
}
doc.count++;
return doc;
}).then(function (res) {
// success, res is {rev: '1-xxx', updated: true, id: 'myDocId'}
}).catch(function (err) {
// error
});
Resulting doc (after 1 upsert
):
{
_id: 'myDocId',
_rev: '1-cefef1ec19869d9441a47021f3fd4710',
count: 1
}
Resulting doc (after 3 upsert
s):
{
_id: 'myDocId',
_rev: '3-536ef59f3ed17a181dc683a255caf1d9',
count: 3
}
A diffFunc
that only updates the doc if it's missing a certain field:
db.upsert('myDocId', function (doc) {
if (!doc.touched) {
doc.touched = true;
return doc;
}
return false; // don't update the doc; it's already been "touched"
}).then(function (res) {
// success, res is {rev: '1-xxx', updated: true, id: 'myDocId'}
}).catch(function (err) {
// error
});
Resulting doc:
{
_id: 'myDocId',
_rev: '1-cefef1ec19869d9441a47021f3fd4710',
touched: true
}
The next time you try to upsert
, the res
will be {rev: '1-xxx', updated: false, id: 'myDocId'}
. The updated: false
indicates that the upsert
function did not actually update the document, and the rev
returned will be the previous winning revision.
You can also return a new object. The _id
and _rev
are added automatically:
db.upsert('myDocId', function (doc) {
return {thisIs: 'awesome!'};
}).then(function (res) {
// success, res is {rev: '1-xxx', updated: true, id: 'myDocId'}
}).catch(function (err) {
// error
});
Resulting doc:
{
_id: 'myDocId',
_rev: '1-cefef1ec19869d9441a47021f3fd4710',
thisIs: 'awesome!'
}
Put a new document with the given docId
, if it doesn't already exist. If you don't specify a callback
, then this function returns a Promise.
docId
- the _id
of the document. Optional if you already include it in the doc
doc
- the document to insert. Should contain an _id
if docId
is not specifiedIf the document already exists, then the Promise will just resolve immediately.
Put a doc if it doesn't exist
db.putIfNotExists('myDocId', {yo: 'dude'}).then(function (res) {
// success, res is {rev: '1-xxx', updated: true, id: 'myDocId'}
}).catch(function (err) {
// error
});
Resulting doc:
{
_id: 'myDocId',
_rev: '1-cefef1ec19869d9441a47021f3fd4710',
yo: 'dude'
}
If you call putIfNotExists
multiple times, then the document will not be updated the 2nd, 3rd, or 4th time (etc.).
If it's not updated, then the res
will be {rev: '1-xxx', updated: false, id: 'myDocId'}
, where rev
is the first revision and updated: false
indicates that it wasn't updated.
You can also just include the _id
inside the document itself:
db.putIfNotExists({_id: 'myDocId', yo: 'dude'}).then(function (res) {
// success, res is {rev: '1-xxx', updated: true, id: 'myDocId'}
}).catch(function (err) {
// error
});
Resulting doc (same as example 1):
{
_id: 'myDocId',
_rev: '1-cefef1ec19869d9441a47021f3fd4710',
yo: 'dude'
}
npm install
npm run build
This will run the tests in Node using LevelDB:
npm test
You can also check for 100% code coverage using:
npm run coverage
If you have mocha installed globally you can run single test with:
TEST_DB=local mocha --reporter spec --grep search_phrase
The TEST_DB
environment variable specifies the database that PouchDB should use (see package.json
).
npm run test-browser
npm run test-local
FAQs
PouchDB upsert and putIfNotExists functions
The npm package pouchdb-upsert receives a total of 2,142 weekly downloads. As such, pouchdb-upsert popularity was classified as popular.
We found that pouchdb-upsert demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.