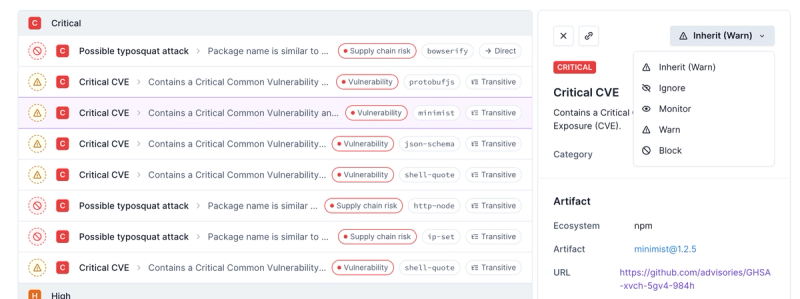
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
pretty-format
Advanced tools
Package description
The pretty-format npm package is a JavaScript library that allows you to serialize any JavaScript value into a string with a human-readable format. It is particularly useful for snapshot testing, where you want to compare the expected and actual output of your test cases.
Pretty-printing of basic JavaScript types
This feature allows you to convert basic JavaScript types like objects, arrays, strings, numbers, etc., into a nicely formatted string.
const prettyFormat = require('pretty-format');
const value = { foo: 'bar', baz: 42 };
console.log(prettyFormat(value));
Customizing output with plugins
pretty-format supports plugins that can be used to customize the output for specific types of values, such as React elements.
const prettyFormat = require('pretty-format');
const ReactElementPlugin = require('pretty-format/plugins/ReactElement');
const reactElement = <div>Hello World</div>;
console.log(prettyFormat(reactElement, { plugins: [ReactElementPlugin] }));
Minimizing diff output
By using pretty-format in combination with a diffing library like jest-diff, you can minimize the output of diffs to make them easier to read and understand.
const prettyFormat = require('pretty-format');
const diff = require('jest-diff');
const oldValue = { a: 'old', b: 'values' };
const newValue = { a: 'new', b: 'values' };
const difference = diff(prettyFormat(oldValue), prettyFormat(newValue));
console.log(difference);
Chalk is a popular npm package for styling terminal strings. While it doesn't serialize objects, it can be used in conjunction with pretty-format to colorize the output, enhancing readability.
Util is a core Node.js module that provides a method called 'inspect' for printing objects in a readable format. It is similar to pretty-format but is built into Node.js and does not support plugins.
js-beautify is an npm package that can format HTML, CSS, and JavaScript code. It is more focused on formatting code rather than serializing arbitrary JavaScript values like pretty-format.
Changelog
jest 22.0.0
[jest-resolve]
Use module.builtinModules
as BUILTIN_MODULES
when it exists[jest-worker]
Remove debug
and inspect
flags from the arguments sent to the child (#5068)[jest-config]
Use all --testPathPattern
and <regexForTestFiles>
args in testPathPattern
(#5066)[jest-cli]
Do not support --watch
inside non-version-controlled environments (#5060)[jest-config]
Escape Windows path separator in testPathPattern CLI arguments (#5054)[jest-jasmine]
Register sourcemaps as node environment to improve performance with jsdom (#5045)[pretty-format]
Do not call toJSON recursively (#5044)[pretty-format]
Fix errors when identity-obj-proxy mocks CSS Modules (#4935)[babel-jest]
Fix support for namespaced babel version 7 (#4918)[expect]
fix .toThrow for promises (#4884)[jest-docblock]
pragmas should preserve urls (#4837)[jest-cli]
Check if npm_lifecycle_script
calls Jest directly (#4629)[jest-cli]
Fix --showConfig to show all configs (#4494)[jest-cli]
Throw if maxWorkers
doesn't have a value (#4591)[jest-cli]
Use fs.realpathSync.native
if available (#5031)[jest-config]
Fix --passWithNoTests
(#4639)[jest-config]
Support rootDir
tag in testEnvironment (#4579)[jest-editor-support]
Fix --showConfig
to support jest 20 and jest 21 (#4575)[jest-editor-support]
Fix editor support test for node 4 (#4640)[jest-mock]
Support mocking constructor in mockImplementationOnce
(#4599)[jest-runtime]
Fix manual user mocks not working with custom resolver (#4489)[jest-util]
Fix runOnlyPendingTimers
for setTimeout
inside setImmediate
(#4608)[jest-message-util]
Always remove node internals from stacktraces (#4695)[jest-resolve]
changes method of determining builtin modules to include missing builtins (#4740)[pretty-format]
Prevent error in pretty-format for window in jsdom test env (#4750)[jest-resolve]
Preserve module identity for symlinks (#4761)[jest-config]
Include error message for preset
json (#4766)[pretty-format]
Throw PrettyFormatPluginError
if a plugin halts with an exception (#4787)[expect]
Keep the stack trace unchanged when PrettyFormatPluginError
is thrown by pretty-format (#4787)[jest-environment-jsdom]
Fix asynchronous test will fail due to timeout issue. (#4669)[jest-cli]
Fix --onlyChanged
path case sensitivity on Windows platform (#4730)[jest-runtime]
Use realpath to match transformers (#5000)[expect]
[BREAKING] Replace identity equality with Object.is in toBe matcher (#4917)[jest-message-util]
Add codeframe to test assertion failures (#5087)[jest-config]
Add Global Setup/Teardown options (#4716)[jest-config]
Add testEnvironmentOptions
to apply to jsdom options or node context. (#5003)[jest-jasmine2]
Update Timeout error message to jest.timeout
and display current timeout value (#4990)[jest-runner]
Enable experimental detection of leaked contexts (#4895)[jest-cli]
Add combined coverage threshold for directories. (#4885)[jest-mock]
Add timestamps
to mock state. (#4866)[eslint-plugin-jest]
Add prefer-to-have-length
lint rule. (#4771)[jest-environment-jsdom]
[BREAKING] Upgrade to JSDOM@11 (#4770)[jest-environment-*]
[BREAKING] Add Async Test Environment APIs, dispose is now teardown (#4506)[jest-cli]
Add an option to clear the cache (#4430)[babel-plugin-jest-hoist]
Improve error message, that the second argument of jest.mock
must be an inline function (#4593)[jest-snapshot]
[BREAKING] Concatenate name of test and snapshot (#4460)[jest-cli]
[BREAKING] Fail if no tests are found (#3672)[jest-diff]
Highlight only last of odd length leading spaces (#4558)[jest-docblock]
Add docblock.print()
(#4517)[jest-docblock]
Add strip
(#4571)[jest-docblock]
Preserve leading whitespace in docblock comments (#4576)[jest-docblock]
remove leading newlines from parswWithComments().comments
(#4610)[jest-editor-support]
Add Snapshots metadata (#4570)[jest-editor-support]
Adds an 'any' to the typedef for updateFileWithJestStatus
(#4636)[jest-editor-support]
Better monorepo support (#4572)[jest-environment-jsdom]
Add simple rAF polyfill in jsdom environment to work with React 16 (#4568)[jest-environment-node]
Implement node Timer api (#4622)[jest-jasmine2]
Add testPath to reporter callbacks (#4594)[jest-mock]
Added support for naming mocked functions with .mockName(value)
and .mockGetName()
(#4586)[jest-runtime]
Add module.loaded
, and make module.require
not enumerable (#4623)[jest-runtime]
Add module.parent
(#4614)[jest-runtime]
Support sourcemaps in transformers (#3458)[jest-snapshot]
[BREAKING] Add a serializer for jest.fn
to allow a snapshot of a jest mock (#4668)[jest-worker]
Initial version of parallel worker abstraction, say hello! (#4497)[jest-jasmine2]
Add testLocationInResults
flag to add location information per spec to test results (#4782)[jest-environment-jsdom]
Update JSOM to 11.4, which includes built-in support for requestAnimationFrame
(#4919)[jest-cli]
Hide watch usage output when running on non-interactive environments (#4958)[jest-snapshot]
Promises support for toThrowErrorMatchingSnapshot
(#4946)[jest-cli]
Explain which snapshots are obsolete (#5005)[docs]
Add guide of using with puppeteer (#5093)[jest-util]
jest-util
should not depend on jest-mock
(#4992)[*]
[BREAKING] Drop support for Node.js version 4 (#4769)[docs]
Wrap code comments at 80 characters (#4781)[eslint-plugin-jest]
Removed from the Jest core repo, and moved to https://github.com/jest-community/eslint-plugin-jest (#4867)[babel-jest]
Explicitly bump istanbul to newer versions (#4616)[expect]
Upgrade mocha and rollup for browser testing (#4642)[docs]
Add info about coveragePathIgnorePatterns
(#4602)[docs]
Add Vuejs series of testing with Jest (#4648)[docs]
Mention about optional done
argument in test function (#4556)[jest-cli]
Bump node-notifier version (#4609)[jest-diff]
Simplify highlight for leading and trailing spaces (#4553)[jest-get-type]
Add support for date (#4621)[jest-matcher-utils]
Call chalk.inverse
for trailing spaces (#4578)[jest-runtime]
Add .advanceTimersByTime
; keep .runTimersToTime()
as an alias.[docs]
Include missing dependency in TestEnvironment sample code[docs]
Add clarification for hook execution order[docs]
Update expect.anything()
sample code (#5007)Readme
Stringify any JavaScript value.
$ npm install pretty-format
var prettyFormat = require('pretty-format');
var obj = { foo: 1 };
obj.self = obj;
obj[Symbol('foo')] = 'foo';
obj.bar = new Map();
obj.bar.set('baz', 'bat');
console.log(prettyFormat(obj));
// Object {
// "foo": 1,
// "self": [Circular],
// "bar": Map {
// "baz" => "bat"
// },
// Symbol(foo): "foo"
// }
FAQs
Stringify any JavaScript value.
The npm package pretty-format receives a total of 66,623,110 weekly downloads. As such, pretty-format popularity was classified as popular.
We found that pretty-format demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.