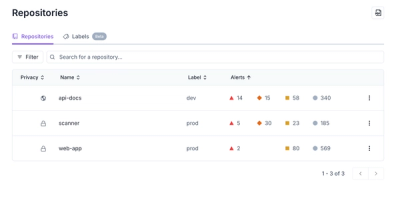
Product
Redesigned Repositories Page: A Faster Way to Prioritize Security Risk
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
prometheus-query
Advanced tools
A Javascript client for Prometheus query API.
⚠️ This library does not export metrics. Please use prom-client instead.
For building shiny Charts, you may like this chartjs plugin: samber/chartjs-plugin-datasource-prometheus.
npm install prometheus-query
prometheus-query-js
has been recoded into Typescript.PrometheusQuery
is not the default export anymore.PrometheusQuery
has been renamed as PrometheusDriver
.<script src="https://cdn.jsdelivr.net/npm/prometheus-query/dist/prometheus-query.umd.min.js"></script>
<script type="application/javacript">
const prom = new Prometheus.PrometheusDriver(...);
</script>
import { PrometheusDriver } from 'prometheus-query';
const prom = new PrometheusDriver({
endpoint: "https://prometheus.demo.do.prometheus.io",
baseURL: "/api/v1" // default value
});
// last `up` value
const q = 'up{instance="demo.do.prometheus.io:9090",job="node"}';
prom.instantQuery(q)
.then((res) => {
const series = res.result;
series.forEach((serie) => {
console.log("Serie:", serie.metric.toString());
console.log("Time:", serie.value.time);
console.log("Value:", serie.value.value);
});
})
.catch(console.error);
Output:
Serie: up{instance="prometheus.demo.do.prometheus.io:9100", job="node"}
Time: Sun Feb 16 2020 18:33:59 GMT+0100 (Central European Standard Time)
Value: 1
// up during past 24h
const q = 'up';
const start = new Date().getTime() - 24 * 60 * 60 * 1000;
const end = new Date();
const step = 6 * 60 * 60; // 1 point every 6 hours
prom.rangeQuery(q, start, end, step)
.then((res) => {
const series = res.result;
series.forEach((serie) => {
console.log("Serie:", serie.metric.toString());
console.log("Values:\n" + serie.values.join('\n'));
});
})
.catch(console.error);
Output:
Serie: up{instance="prometheus.demo.do.prometheus.io:9090", job="prometheus"}
Values:
Sat Feb 15 2020 18:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 00:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 06:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 12:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 18:21:47 GMT+0100 (Central European Standard Time): 1
Serie: up{instance="prometheus.demo.do.prometheus.io:9093", job="alertmanager"}
Values:
Sat Feb 15 2020 18:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 00:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 06:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 12:21:47 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 18:21:47 GMT+0100 (Central European Standard Time): 1
Serie: up{instance="prometheus.demo.do.prometheus.io:9100", job="node"}
Values:
Sat Feb 15 2020 18:20:51 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 00:20:51 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 06:20:51 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 12:20:51 GMT+0100 (Central European Standard Time): 1
Sun Feb 16 2020 18:20:51 GMT+0100 (Central European Standard Time): 1
const match = 'up';
const start = new Date().getTime() - 24 * 60 * 60 * 1000;
const end = new Date();
prom.series(match, start, end)
.then((res) => {
console.log('Series:');
console.log(res.join('\n'));
})
.catch(console.error);
Output:
up{instance="demo.do.prometheus.io:9090", job="prometheus"}
up{instance="demo.do.prometheus.io:9093", job="alertmanager"}
up{instance="demo.do.prometheus.io:9100", job="node"}
prom.alerts()
.then(console.log)
.catch(console.error);
Output:
[
Alert {
activeAt: 2019-11-14T20:04:36.629Z,
annotations: {},
labels: { alertname: 'ExampleAlertAlwaysFiring', job: 'alertmanager' },
state: 'firing',
value: 1
},
Alert {
activeAt: 2019-11-14T20:04:36.629Z,
annotations: {},
labels: { alertname: 'ExampleAlertAlwaysFiring', job: 'node' },
state: 'firing',
value: 1
},
Alert {
activeAt: 2019-11-14T20:04:36.629Z,
annotations: {},
labels: { alertname: 'ExampleAlertAlwaysFiring', job: 'prometheus' },
state: 'firing',
value: 1
},
Alert {
activeAt: 2019-11-14T20:04:36.629Z,
annotations: {},
labels: { alertname: 'ExampleAlertAlwaysFiring', job: 'pushgateway' },
state: 'firing',
value: 1
}
]
Using basic auth:
new PrometheusDriver({
endpoint: "https://prometheus.demo.do.prometheus.io",
auth: {
username: 'foo',
password: 'bar'
}
});
Using cookies:
new PrometheusDriver({
endpoint: "https://prometheus.demo.do.prometheus.io",
withCredentials: true
});
new PrometheusDriver({
endpoint: "https://prometheus.demo.do.prometheus.io",
proxy: {
host: 'proxy.acme.com',
port: 8080
}
});
new PrometheusDriver({
endpoint: "https://prometheus.demo.do.prometheus.io",
proxy: {
host: 'proxy.acme.com',
port: 8080
},
requestInterceptor: {
onFulfilled: (config: AxiosRequestConfig) => {
return config;
},
onRejected: (error: any) => {
return Promise.reject(error.message);
}
},
responseInterceptor: {
onFulfilled: (res: AxiosResponse) => {
return res;
},
onRejected: (error: any) => {
return Promise.reject(error.message);
}
}
});
If you open a Prometheus instance on Internet, it would be a good idea to block some routes.
Start by blocking /api/v1/admin
. I'm pretty sure allowing only /api/v1/query
and /api/v1/query_range
will match your needs.
Also don't use Prometheus as a multitenant timeseries database!
At your own risk... 😘
The Prometheus Query client is open source and contributions from the community (you!) are welcome.
There are many ways to contribute: writing code, documentation, reporting issues...
👤 Samuel Berthe
Give a ⭐️ if this project helped you!
Copyright © 2020 Samuel Berthe.
This project is MIT licensed.
FAQs
A Javascript client for Prometheus query API
The npm package prometheus-query receives a total of 10,443 weekly downloads. As such, prometheus-query popularity was classified as popular.
We found that prometheus-query demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
Security News
Multiple deserialization flaws in PyTorch Lightning could allow remote code execution when loading untrusted model files, affecting versions up to 2.4.0.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.