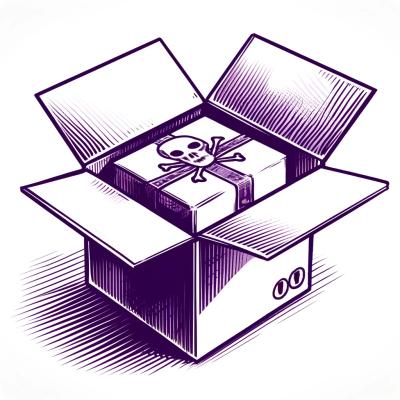
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
ratelimiter
Advanced tools
The 'ratelimiter' npm package is a simple and efficient tool for rate limiting in Node.js applications. It helps control the rate at which requests are processed, preventing abuse and ensuring fair usage of resources. It is particularly useful in scenarios where you need to limit the number of requests a user can make to an API within a certain timeframe.
Basic Rate Limiting
This code demonstrates basic rate limiting using the 'ratelimiter' package. It sets a limit of 5 requests per minute for a user with ID 'user123'. The remaining requests are logged to the console.
const RateLimiter = require('ratelimiter');
const redis = require('redis');
const client = redis.createClient();
const limit = new RateLimiter({
id: 'user123',
db: client,
max: 5, // max requests
duration: 60000 // per minute
});
limit.get((err, limit) => {
if (err) throw err;
console.log(limit.remaining); // remaining requests
});
Custom Rate Limiting
This code demonstrates custom rate limiting where a user with ID 'user456' is allowed 10 requests per 30 seconds. It checks if the user has remaining requests and logs whether the request is allowed or if the rate limit is exceeded.
const RateLimiter = require('ratelimiter');
const redis = require('redis');
const client = redis.createClient();
const limit = new RateLimiter({
id: 'user456',
db: client,
max: 10, // max requests
duration: 30000 // per 30 seconds
});
limit.get((err, limit) => {
if (err) throw err;
if (limit.remaining) {
console.log('Request allowed');
} else {
console.log('Rate limit exceeded');
}
});
The 'express-rate-limit' package is a middleware for Express.js applications that helps to limit repeated requests to public APIs and endpoints. It is easy to set up and configure, making it a popular choice for Express-based applications. Compared to 'ratelimiter', it is more tightly integrated with Express and provides a more straightforward setup for web applications.
The 'rate-limiter-flexible' package is a highly flexible and powerful rate limiting library for Node.js. It supports various backends like Redis, MongoDB, and in-memory storage. It offers more advanced features and customization options compared to 'ratelimiter', making it suitable for complex rate limiting scenarios.
The 'bottleneck' package is a powerful rate limiter and job scheduler for Node.js. It allows for fine-grained control over the rate of function execution and supports clustering and distributed rate limiting. Compared to 'ratelimiter', 'bottleneck' provides more advanced scheduling capabilities and is suitable for more complex use cases.
Rate limiter for Node.js backed by Redis.
NOTE: Promise version available at async-ratelimiter.
v3.4.1 - #55 by @barwin - Remove splice operation.
v3.3.1 - #51 - Remove tidy option as it's always true.
v3.3.0 - #47 by @penghap - Add tidy option to clean old records upon saving new records. Drop support in node 4.
v3.2.0 - #44 by @xdmnl - Return accurate reset time for each limited call.
v3.1.0 - #40 by @ronjouch - Add reset milliseconds to the result object.
v3.0.2 - #33 by @promag - Use sorted set to limit with moving window.
v2.2.0 - #30 by @kp96 - Race condition when using async.times
.
v2.1.3 - #22 by @coderhaoxin - Dev dependencies versions bump.
v2.1.2 - #17 by @waleedsamy - Add Travis CI support.
v2.1.1 - #13 by @kwizzn - Fixes out-of-sync TTLs after running decr().
v2.1.0 - #12 by @luin - Adding support for ioredis.
v2.0.1 - #9 by @ruimarinho - Update redis commands to use array notation.
v2.0.0 - API CHANGE - Change remaining
to include current call instead of decreasing it. Decreasing caused an off-by-one problem and caller could not distinguish between last legit call and a rejected call.
$ npm install ratelimiter
Example Connect middleware implementation limiting against a user._id
:
var id = req.user._id;
var limit = new Limiter({ id: id, db: db });
limit.get(function(err, limit){
if (err) return next(err);
res.set('X-RateLimit-Limit', limit.total);
res.set('X-RateLimit-Remaining', limit.remaining - 1);
res.set('X-RateLimit-Reset', limit.reset);
// all good
debug('remaining %s/%s %s', limit.remaining - 1, limit.total, id);
if (limit.remaining) return next();
// not good
var delta = (limit.reset * 1000) - Date.now() | 0;
var after = limit.reset - (Date.now() / 1000) | 0;
res.set('Retry-After', after);
res.send(429, 'Rate limit exceeded, retry in ' + ms(delta, { long: true }));
});
total
- max
valueremaining
- number of calls left in current duration
without decreasing current get
reset
- time since epoch in seconds at which the rate limiting period will end (or already ended)resetMs
- time since epoch in milliseconds at which the rate limiting period will end (or already ended)id
- the identifier to limit against (typically a user id)db
- redis connection instancemax
- max requests within duration
[2500]duration
- of limit in milliseconds [3600000]MIT
FAQs
abstract rate limiter backed by redis
The npm package ratelimiter receives a total of 422,706 weekly downloads. As such, ratelimiter popularity was classified as popular.
We found that ratelimiter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.