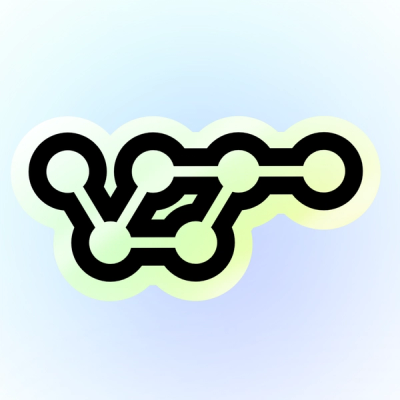
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
rc-dialog
Advanced tools
The rc-dialog package is a React component for creating and managing modals, dialogs, or popups in a React application. It provides a flexible and accessible way to generate dialog windows that can contain a wide range of content, from simple text to complex forms and interactive elements. The package offers various customization options, including animations, styles, and positioning, making it a versatile tool for UI development.
Basic Dialog Creation
This code sample demonstrates how to create a basic dialog with a title and some content. The dialog's visibility is controlled by a state variable.
import React from 'react';
import Dialog from 'rc-dialog';
const MyDialog = () => {
const [visible, setVisible] = React.useState(false);
return (
<>
<button onClick={() => setVisible(true)}>Open Dialog</button>
<Dialog
visible={visible}
onClose={() => setVisible(false)}
title='Dialog Title'
>
<p>This is a basic dialog example.</p>
</Dialog>
</>
);
};
export default MyDialog;
Customizing Styles
This example shows how to apply custom styles to the dialog, including its width and the padding of its body content.
import React from 'react';
import Dialog from 'rc-dialog';
const MyStyledDialog = () => {
const [visible, setVisible] = React.useState(false);
return (
<>
<button onClick={() => setVisible(true)}>Open Styled Dialog</button>
<Dialog
visible={visible}
onClose={() => setVisible(false)}
title='Styled Dialog'
style={{ width: 600 }}
bodyStyle={{ padding: 20 }}
>
<p>This dialog has customized styles.</p>
</Dialog>
</>
);
};
export default MyStyledDialog;
Modal with Footer
This code snippet illustrates how to add a footer to the dialog, which in this case contains a button to close the modal.
import React from 'react';
import Dialog from 'rc-dialog';
const ModalWithFooter = () => {
const [visible, setVisible] = React.useState(false);
return (
<>
<button onClick={() => setVisible(true)}>Open Modal with Footer</button>
<Dialog
visible={visible}
onClose={() => setVisible(false)}
title='Modal Title'
footer={<button onClick={() => setVisible(false)}>Close</button>}
>
<p>This modal includes a footer with a close button.</p>
</Dialog>
</>
);
};
export default ModalWithFooter;
react-modal is a popular package for creating accessible modals in React applications. It offers similar functionalities to rc-dialog, such as customizable styles and easy management of modal states. However, react-modal places a stronger emphasis on accessibility features.
material-ui (specifically the Dialog component within it) provides a comprehensive solution for creating dialogs and modals in React applications that adhere to Material Design principles. Compared to rc-dialog, material-ui's Dialog component comes with a wider range of pre-designed styles and animations, making it a good choice for applications following Material Design guidelines.
react dialog component
http://localhost:8007/examples/
online example: https://dialog.react-component.vercel.app/
var Dialog = require('rc-dialog');
ReactDOM.render(
<Dialog title={title} onClose={callback1} visible>
<p>first dialog</p>
</Dialog>
), document.getElementById('t1'));
// use dialog
Name | Type | Default | Description | Version |
---|---|---|---|---|
prefixCls | String | rc-dialog | The dialog dom node's prefixCls | |
className | String | additional className for dialog | ||
classNames | { header?: string; body?: string; footer?: string; mask?: string; content?: string; wrapper?: string; } | pass className to target area | ||
styles | { header?: CSSProperties; body?: CSSProperties; footer?: CSSProperties; mask?: CSSProperties; content?: CSSProperties; wrapper?: CSSProperties; } | pass styles to target area | ||
style | Object | {} | Root style for dialog element.Such as width, height | |
zIndex | Number | |||
visible | Boolean | false | current dialog's visible status | |
animation | String | part of dialog animation css class name | ||
maskAnimation | String | part of dialog's mask animation css class name | ||
transitionName | String | dialog animation css class name | ||
maskTransitionName | String | mask animation css class name | ||
title | String|React.Element | Title of the dialog | ||
footer | React.Element | footer of the dialog | ||
closable | Boolean | ({ closeIcon?: React.ReactNode; disabled?: boolean } & React.AriaAttributes | true | whether show close button | |
mask | Boolean | true | whether show mask | |
maskClosable | Boolean | true | whether click mask to close | |
keyboard | Boolean | true | whether support press esc to close | |
mousePosition | {x:number,y:number} | set pageX and pageY of current mouse(it will cause transform origin to be set). | ||
onClose | function() | called when click close button or mask | ||
afterClose | function() | called when close animation end | ||
getContainer | function(): HTMLElement | to determine where Dialog will be mounted | ||
destroyOnClose | Boolean | false | to unmount child compenents on onClose | |
closeIcon | ReactNode | specific the close icon. | ||
forceRender | Boolean | false | Create dialog dom node before dialog first show | |
focusTriggerAfterClose | Boolean | true | focus trigger element when dialog closed | |
modalRender | (node: ReactNode) => ReactNode | Custom modal content render | 8.3.0 |
npm install
npm start
npm test
npm run chrome-test
npm run coverage
open coverage/ dir
rc-dialog is released under the MIT license.
FAQs
dialog ui component for react
The npm package rc-dialog receives a total of 1,193,718 weekly downloads. As such, rc-dialog popularity was classified as popular.
We found that rc-dialog demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.