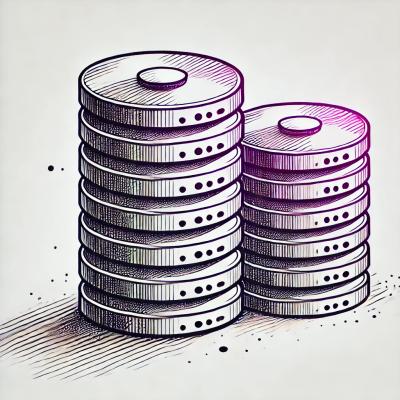
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
react-bootstrap-typeahead
Advanced tools
The react-bootstrap-typeahead package is a flexible, robust, and highly customizable typeahead (autocomplete) component for React. It is built using Bootstrap styles and provides a variety of features to enhance user experience in form inputs.
Basic Typeahead
This feature allows you to create a basic typeahead input where users can type and get suggestions from a predefined list of options.
import React from 'react';
import { Typeahead } from 'react-bootstrap-typeahead';
const options = ['John', 'Paul', 'George', 'Ringo'];
function BasicTypeahead() {
return (
<Typeahead
id="basic-typeahead"
labelKey="name"
options={options}
placeholder="Choose a name..."
/>
);
}
export default BasicTypeahead;
Multiple Selections
This feature allows users to select multiple options from the typeahead suggestions, making it useful for forms that require multiple inputs.
import React from 'react';
import { Typeahead } from 'react-bootstrap-typeahead';
const options = ['John', 'Paul', 'George', 'Ringo'];
function MultiSelectTypeahead() {
return (
<Typeahead
id="multi-select-typeahead"
labelKey="name"
multiple
options={options}
placeholder="Choose several names..."
/>
);
}
export default MultiSelectTypeahead;
Custom Rendering
This feature allows you to customize the rendering of the typeahead options, providing more control over the appearance and behavior of the suggestions.
import React from 'react';
import { Typeahead } from 'react-bootstrap-typeahead';
const options = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Paul' },
{ id: 3, name: 'George' },
{ id: 4, name: 'Ringo' }
];
function CustomRenderTypeahead() {
return (
<Typeahead
id="custom-render-typeahead"
labelKey="name"
options={options}
placeholder="Choose a Beatle..."
renderMenuItemChildren={(option, props) => (
<div>
<span>{option.name}</span>
</div>
)}
/>
);
}
export default CustomRenderTypeahead;
react-autosuggest is a popular package for building autocomplete and autosuggest components in React. It offers a high degree of customization and flexibility, similar to react-bootstrap-typeahead, but does not rely on Bootstrap for styling.
downshift is a highly customizable library for building autocomplete, dropdown, and select components in React. It provides a set of primitives to build complex components, offering more flexibility but requiring more setup compared to react-bootstrap-typeahead.
react-select is a flexible and beautiful Select Input control for React with support for multi-select, async options, and more. It is highly customizable and provides a rich set of features, making it a strong alternative to react-bootstrap-typeahead.
React-based typeahead component that uses standard the Bootstrap theme for as a base and supports both single- and multi-selection.
It is highly recommended that you use npm to install the module in your project and build using a tool like webpack or browserify.
npm install react-bootstrap-typeahead
If you want to use the component in a standalone manner, both an unminified development version and a minified production version are included in the dist
folder.
react-bootstrap-typeahead
works very much like any standard input
element. It requires an array of options to display, similar to a select
.
<Typeahead
onChange={this._handleChange}
options={data}
/>
react-bootstrap-typeahead
allows single-selection by default, but also supports multi-selection. Simply set the multiple
prop and the component turns into a tokenizer:
<Typeahead
multiple
onChange={this._handleChange}
options={data}
/>
Like an input
, the component can be controlled or uncontrolled. Use the selected
prop to control it via the parent, or defaultSelected
to optionally set defaults and then allow the component to control itself.
<Typeahead
onChange={this._handleChange}
options={data}
selected={selected}
/>
react-bootstrap-typeahead
has some expectations about the shape of your data. It expects an array of objects, each of which should have a string property to be used as the label for display. By default, the key is named label
, but you can specify a different key via the labelKey
prop.
As far as the source of the data, the component simply handles rendering and selection. It is agnostic about the data source (eg: an async endpoint), which should be handled separately.
An example file is included with the module. Simply open index.html
in a browser.
Name | Type | Default | Description |
---|---|---|---|
defaultSelected | array | [] | Specify any pre-selected options. Use only if you want the component to be uncontrolled. |
emptyLabel | string | 'No matches found.' | Message to display in the menu if there are no valid results. |
labelKey | string | 'label' | Specify which option key to use for display. By default, the selector will use the label key. |
maxHeight | number | 300 | Maximum height of the dropdown menu, in px. |
multiple | boolean | false | Whether or not multiple selections are allowed. |
options required | array | Full set of options, including any pre-selected options. | |
placeholder | string | Placeholder text for the input. | |
selected | array | [] | The selected option(s) displayed in the input. Use this prop if you want to control the component via it's parent. |
Token
and MenuItem
renderingFAQs
React typeahead with Bootstrap styling
The npm package react-bootstrap-typeahead receives a total of 0 weekly downloads. As such, react-bootstrap-typeahead popularity was classified as not popular.
We found that react-bootstrap-typeahead demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.