react-dropzone
Advanced tools
Comparing version 10.1.0 to 10.1.1
@@ -73,3 +73,3 @@ function _toConsumableArray(arr) { return _arrayWithoutHoles(arr) || _iterableToArray(arr) || _nonIterableSpread(); } | ||
}; | ||
}); // TODO: Figure out why react-styleguidist cannot create docs if we don't return a jsx element | ||
}, [ref]); // TODO: Figure out why react-styleguidist cannot create docs if we don't return a jsx element | ||
@@ -76,0 +76,0 @@ return React.createElement(Fragment, null, children(_objectSpread({}, props, { |
@@ -34,7 +34,7 @@ By providing `accept` prop you can make the dropzone accept specific file types and reject the others. | ||
return ( | ||
<section> | ||
<div {...getRootProps()}> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
<p>Only *.jpeg and *.png images will be accepted</p> | ||
<em>(Only *.jpeg and *.png images will be accepted)</em> | ||
</div> | ||
@@ -80,7 +80,9 @@ <aside> | ||
return ( | ||
<div {...getRootProps()}> | ||
<input {...getInputProps()} /> | ||
{isDragAccept && "All files will be accepted"} | ||
{isDragReject && "Some files will be rejected"} | ||
{!isDragActive && "Drop some files here..."} | ||
<div className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
{isDragAccept && (<p>All files will be accepted</p>)} | ||
{isDragReject && (<p>Some files will be rejected</p>)} | ||
{!isDragActive && (<p>Drop some files here ...</p>)} | ||
</div> | ||
</div> | ||
@@ -111,7 +113,9 @@ ); | ||
return ( | ||
<div {...getRootProps()}> | ||
<input {...getInputProps()} /> | ||
{isDragAccept && "All files will be accepted"} | ||
{isDragReject && "Some files will be rejected"} | ||
{!isDragActive && "Drop some files here..."} | ||
<div className="container"> | ||
<div {...getRootProps({className: "dropzone"})}> | ||
<input {...getInputProps()} /> | ||
{isDragAccept && (<p>All files will be accepted</p>)} | ||
{isDragReject && (<p>Some files will be rejected</p>)} | ||
{!isDragActive && (<p>Drop some files here ...</p>)} | ||
</div> | ||
</div> | ||
@@ -118,0 +122,0 @@ ); |
@@ -22,4 +22,4 @@ The `useDropzone` hook just binds the necessary handlers to create a drag 'n' drop zone. | ||
return ( | ||
<section> | ||
<div {...getRootProps()}> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
@@ -42,8 +42,9 @@ <p>Drag 'n' drop some files here, or click to select files</p> | ||
```jsx harmony | ||
import React, {useState} from 'react'; | ||
import React from 'react'; | ||
import {useDropzone} from 'react-dropzone'; | ||
function Basic(props) { | ||
const [disabled, setDisabled] = useState(true); | ||
const {acceptedFiles, getRootProps, getInputProps} = useDropzone({disabled}); | ||
const {acceptedFiles, getRootProps, getInputProps} = useDropzone({ | ||
disabled: true | ||
}); | ||
@@ -57,12 +58,4 @@ const files = acceptedFiles.map(file => ( | ||
return ( | ||
<section> | ||
<aside> | ||
<button | ||
type="button" | ||
onClick={() => setDisabled(!disabled)} | ||
> | ||
Toggle disabled | ||
</button> | ||
</aside> | ||
<div {...getRootProps()}> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone disabled'})}> | ||
<input {...getInputProps()} /> | ||
@@ -69,0 +62,0 @@ <p>Drag 'n' drop some files here, or click to select files</p> |
@@ -28,4 +28,4 @@ If you're still using class components, you can use the [`<Dropzone>`](https://react-dropzone.js.org/#components) component provided by the lib: | ||
{({getRootProps, getInputProps}) => ( | ||
<section> | ||
<div {...getRootProps()}> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
@@ -32,0 +32,0 @@ <p>Drag 'n' drop some files here, or click to select files</p> |
If you'd like to prevent drag events propagation from the child to parent, you can use the `{noDragEventsBubbling}` property on the child: | ||
```jsx harmony | ||
import React, {useCallback, useMemo, useReducer} from 'react'; | ||
import React from 'react'; | ||
import {useDropzone} from 'react-dropzone'; | ||
const initialParentState = { | ||
parent: {}, | ||
child: {} | ||
}; | ||
function OuterDropzone(props) { | ||
const {getRootProps} = useDropzone({ | ||
// Note how this callback is never invoked if drop occurs on the inner dropzone | ||
onDrop: files => console.log(files) | ||
}); | ||
const parentStyle = { | ||
width: 200, | ||
height: 200, | ||
border: '2px dashed #888' | ||
}; | ||
const stateStyle = { | ||
fontFamily: 'monospace' | ||
}; | ||
const childStyle = { | ||
width: 160, | ||
height: 160, | ||
margin: 20, | ||
border: '2px dashed #ccc' | ||
}; | ||
function Parent(props) { | ||
const [state, dispatch] = useReducer(parentReducer, initialParentState); | ||
const dropzoneProps = useMemo(() => computeDropzoneProps({dispatch}, 'parent'), [dispatch]); | ||
const {getRootProps} = useDropzone(dropzoneProps); | ||
const childProps = useMemo(() => ({dispatch}), [dispatch]); | ||
return ( | ||
<section> | ||
<div {...getRootProps({style: parentStyle})}> | ||
<Child {...childProps} /> | ||
<div className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<InnerDropzone /> | ||
<p>Outer dropzone</p> | ||
</div> | ||
<aside style={stateStyle}> | ||
<p>Parent: {JSON.stringify(state.parent)}</p> | ||
<p>Child: {JSON.stringify(state.child)}</p> | ||
</aside> | ||
</section> | ||
</div> | ||
); | ||
} | ||
function Child(props) { | ||
const dropzoneProps = useMemo(() => ({ | ||
...computeDropzoneProps(props, 'child'), | ||
noDragEventsBubbling: true | ||
}), [ | ||
props.dispatch | ||
]); | ||
const {getRootProps} = useDropzone(dropzoneProps); | ||
function InnerDropzone(props) { | ||
const {getRootProps} = useDropzone({noDragEventsBubbling: true}); | ||
return ( | ||
<div | ||
{...getRootProps({ | ||
style: childStyle | ||
})} | ||
/> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<p>Inner dropzone</p> | ||
</div> | ||
); | ||
} | ||
<OuterDropzone /> | ||
``` | ||
function parentReducer(state, action) { | ||
switch (action.type) { | ||
case 'onDragEnter': | ||
case 'onDragOver': | ||
case 'onDragLeave': | ||
case 'onDrop': | ||
return computeDragState(action, state); | ||
default: | ||
return state; | ||
} | ||
} | ||
Note that internally we use `event.stopPropagation()` to achieve the behavior illustrated above, but this comes with its own [drawbacks](https://javascript.info/bubbling-and-capturing#stopping-bubbling). | ||
function computeDragState(action, state) { | ||
const {type, payload} = action; | ||
const {node} = payload; | ||
const events = {...state[node]}; | ||
if (type !== events.current) { | ||
events.previous = events.current; | ||
} | ||
events.current = type; | ||
return { | ||
...state, | ||
[node]: events | ||
}; | ||
} | ||
If you'd like to selectively turn off the default dropzone behavior for `onClick`, use the `{noClick}` property: | ||
```jsx harmony | ||
import React from 'react'; | ||
import {useDropzone} from 'react-dropzone'; | ||
function computeDropzoneProps(props, node) { | ||
const rootProps = {}; | ||
function DropzoneWithoutClick(props) { | ||
const {getRootProps, getInputProps, acceptedFiles} = useDropzone({noClick: true}); | ||
const files = acceptedFiles.map(file => <li key={file.path}>{file.path}</li>); | ||
['onDragEnter', 'onDragOver', 'onDragLeave', 'onDrop'].forEach(type => { | ||
Object.assign(rootProps, { | ||
[type]: (...args) => { | ||
const event = type === 'onDrop' ? args.pop() : args.shift(); | ||
props.dispatch({ | ||
type, | ||
payload: {node} | ||
}); | ||
} | ||
}); | ||
}) | ||
return rootProps; | ||
return ( | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
<p>Dropzone without click events</p> | ||
</div> | ||
<aside> | ||
<h4>Files</h4> | ||
<ul>{files}</ul> | ||
</aside> | ||
</section> | ||
); | ||
} | ||
<Parent /> | ||
<DropzoneWithoutClick /> | ||
``` | ||
Note that internally we use `event.stopPropagation()` to achieve the behavior illustrated above, but this comes with its own [drawbacks](https://javascript.info/bubbling-and-capturing#stopping-bubbling). | ||
If you'd like to selectively turn off the default dropzone behavior for `onClick`, `onKeyDown` (both open the file dialog), `onFocus` and `onBlur` (sets the `isFocused` state) and drag events; use the `{noClick}`, `{noKeyboard}` and `{noDrag}` properties: | ||
If you'd like to selectively turn off the default dropzone behavior for `onKeyDown`, `onFocus` and `onBlur`, use the `{noKeyboard}` property: | ||
```jsx harmony | ||
import React, {useCallback, useReducer} from 'react'; | ||
import React from 'react'; | ||
import {useDropzone} from 'react-dropzone'; | ||
const initialEvtsState = { | ||
noClick: true, | ||
noKeyboard: true, | ||
noDrag: true, | ||
files: [] | ||
}; | ||
function DropzoneWithoutKeyboard(props) { | ||
const {getRootProps, getInputProps, acceptedFiles} = useDropzone({noKeyboard: true}); | ||
const files = acceptedFiles.map(file => <li key={file.path}>{file.path}</li>); | ||
function Events(props) { | ||
const [state, dispatch] = useReducer(reducer, initialEvtsState); | ||
const createToggleHandler = type => () => dispatch({type}); | ||
return ( | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
<p>Dropzone without keyboard events</p> | ||
<em>(SPACE/ENTER and focus events are disabled)</em> | ||
</div> | ||
<aside> | ||
<h4>Files</h4> | ||
<ul>{files}</ul> | ||
</aside> | ||
</section> | ||
); | ||
} | ||
const onDrop = useCallback(files => dispatch({ | ||
type: 'setFiles', | ||
payload: files | ||
}), []); | ||
<DropzoneWithoutKeyboard /> | ||
``` | ||
const {getRootProps, getInputProps, isFocused} = useDropzone({...state, onDrop}); | ||
const files = state.files.map(file => <li key={file.path}>{file.path}</li>); | ||
Note that you can prevent the default behavior for click and keyboard events if you ommit the input: | ||
```jsx harmony | ||
import React from 'react'; | ||
import {useDropzone} from 'react-dropzone'; | ||
const options = ['noClick', 'noKeyboard', 'noDrag'].map(key => ( | ||
<div key={key}> | ||
<input | ||
id={key} | ||
type="checkbox" | ||
onChange={createToggleHandler(key)} | ||
checked={state[key]} | ||
/> | ||
<label htmlFor={key}> | ||
{key} | ||
</label> | ||
</div> | ||
)); | ||
function DropzoneWithoutClick(props) { | ||
const {getRootProps, acceptedFiles} = useDropzone(); | ||
const files = acceptedFiles.map(file => <li key={file.path}>{file.path}</li>); | ||
return ( | ||
<section> | ||
<aside> | ||
{options} | ||
</aside> | ||
<div {...getRootProps()}> | ||
<input {...getInputProps()} /> | ||
<p>{getDesc(state)} (<em>{isFocused ? 'focused' : 'not focused'}</em>)</p> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<p>Dropzone without click events</p> | ||
</div> | ||
@@ -171,50 +111,30 @@ <aside> | ||
<DropzoneWithoutClick /> | ||
``` | ||
function getDesc(state) { | ||
if (state.noClick && state.noKeyboard && state.noDrag) { | ||
return `Dropzone will not respond to any events`; | ||
} else if (state.noClick && state.noKeyboard) { | ||
return `Drag 'n' drop files here`; | ||
} else if (state.noClick && state.noDrag) { | ||
return `Press SPACE/ENTER to open the file dialog`; | ||
} else if (state.noKeyboard && state.noDrag) { | ||
return `Click to open the file dialog`; | ||
} else if (state.noClick) { | ||
return `Drag 'n' drop files here or press SPACE/ENTER to open the file dialog`; | ||
} else if (state.noKeyboard) { | ||
return `Drag 'n' drop files here or click to open the file dialog`; | ||
} else if (state.noDrag) { | ||
return `Click/press SPACE/ENTER to open the file dialog`; | ||
} | ||
return `Drag 'n' drop files here or click/press SPACE/ENTER to open the file dialog`; | ||
} | ||
If you'd like to selectively turn off the default dropzone behavior for drag events, use the `{noDrag}` property: | ||
```jsx harmony | ||
import React from 'react'; | ||
import {useDropzone} from 'react-dropzone'; | ||
function reducer(state, action) { | ||
switch (action.type) { | ||
case 'noClick': | ||
return { | ||
...state, | ||
noClick: !state.noClick | ||
}; | ||
case 'noKeyboard': | ||
return { | ||
...state, | ||
noKeyboard: !state.noKeyboard | ||
}; | ||
case 'noDrag': | ||
return { | ||
...state, | ||
noDrag: !state.noDrag | ||
}; | ||
case 'setFiles': | ||
return { | ||
...state, | ||
files: action.payload | ||
}; | ||
default: | ||
return state; | ||
} | ||
function DropzoneWithoutDrag(props) { | ||
const {getRootProps, getInputProps, acceptedFiles} = useDropzone({noDrag: true}); | ||
const files = acceptedFiles.map(file => <li key={file.path}>{file.path}</li>); | ||
return ( | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
<p>Dropzone with no drag events</p> | ||
<em>(Drag 'n' drop is disabled)</em> | ||
</div> | ||
<aside> | ||
<h4>Files</h4> | ||
<ul>{files}</ul> | ||
</aside> | ||
</section> | ||
); | ||
} | ||
<Events /> | ||
<DropzoneWithoutDrag /> | ||
``` | ||
@@ -230,9 +150,12 @@ | ||
{({getRootProps, getInputProps}) => ( | ||
<div | ||
{...getRootProps({ | ||
onDrop: event => event.stopPropagation() | ||
})} | ||
> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
<div className="container"> | ||
<div | ||
{...getRootProps({ | ||
className: 'dropzone', | ||
onDrop: event => event.stopPropagation() | ||
})} | ||
> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
</div> | ||
</div> | ||
@@ -239,0 +162,0 @@ )} |
@@ -14,3 +14,3 @@ You can programmatically invoke the default OS file prompt; just use the `open` method returned by the hook. | ||
function Dropzone(props) { | ||
const {getRootProps, getInputProps, open} = useDropzone({ | ||
const {getRootProps, getInputProps, open, acceptedFiles} = useDropzone({ | ||
// Disable click and keydown behavior | ||
@@ -21,9 +21,21 @@ noClick: true, | ||
const files = acceptedFiles.map(file => ( | ||
<li key={file.path}> | ||
{file.path} - {file.size} bytes | ||
</li> | ||
)); | ||
return ( | ||
<div {...getRootProps()}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here</p> | ||
<button type="button" onClick={open}> | ||
Open File Dialog | ||
</button> | ||
<div className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here</p> | ||
<button type="button" onClick={open}> | ||
Open File Dialog | ||
</button> | ||
</div> | ||
<aside> | ||
<h4>Files</h4> | ||
<ul>{files}</ul> | ||
</aside> | ||
</div> | ||
@@ -53,13 +65,25 @@ ); | ||
<Dropzone ref={dropzoneRef} noClick noKeyboard> | ||
{({getRootProps, getInputProps}) => { | ||
{({getRootProps, getInputProps, acceptedFiles}) => { | ||
return ( | ||
<div {...getRootProps()}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here</p> | ||
<button | ||
type="button" | ||
onClick={openDialog} | ||
> | ||
Open File Dialog | ||
</button> | ||
<div className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here</p> | ||
<button | ||
type="button" | ||
onClick={openDialog} | ||
> | ||
Open File Dialog | ||
</button> | ||
</div> | ||
<aside> | ||
<h4>Files</h4> | ||
<ul> | ||
{acceptedFiles.map(file => ( | ||
<li key={file.path}> | ||
{file.path} - {file.size} bytes | ||
</li> | ||
))} | ||
</ul> | ||
</aside> | ||
</div> | ||
@@ -66,0 +90,0 @@ ); |
@@ -24,4 +24,4 @@ The hook accepts a `getFilesFromEvent` prop that enhances the handling of dropped file system objects and allows more flexible use of them e.g. passing a function that accepts drop event of a folder and resolves it to an array of files adds plug-in functionality of folders drag-and-drop. | ||
return ( | ||
<section> | ||
<div {...getRootProps()}> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
@@ -28,0 +28,0 @@ <p>Drag 'n' drop some files here, or click to select files</p> |
@@ -69,4 +69,4 @@ Starting with version 7.0.0, the `{preview}` property generation on the [File](https://developer.mozilla.org/en-US/docs/Web/API/File) objects and the `{disablePreview}` property on the `<Dropzone>` have been removed. | ||
return ( | ||
<section> | ||
<div {...getRootProps()}> | ||
<section className="container"> | ||
<div {...getRootProps({className: 'dropzone'})}> | ||
<input {...getInputProps()} /> | ||
@@ -73,0 +73,0 @@ <p>Drag 'n' drop some files here, or click to select files</p> |
@@ -10,18 +10,22 @@ The hook fn doesn't set any styles on either of the prop fns (`getRootProps()`/`getInputProps()`). | ||
const baseStyle = { | ||
width: 200, | ||
height: 200, | ||
flex: 1, | ||
display: 'flex', | ||
flexDirection: 'column', | ||
alignItems: 'center', | ||
padding: '20px', | ||
borderWidth: 2, | ||
borderColor: '#666', | ||
borderRadius: 2, | ||
borderColor: '#eeeeee', | ||
borderStyle: 'dashed', | ||
borderRadius: 5 | ||
backgroundColor: '#fafafa', | ||
color: '#bdbdbd', | ||
outline: 'none', | ||
transition: 'border .24s ease-in-out' | ||
}; | ||
const activeStyle = { | ||
borderStyle: 'solid', | ||
borderColor: '#6c6', | ||
backgroundColor: '#eee' | ||
borderColor: '#2196f3' | ||
}; | ||
const acceptStyle = { | ||
borderStyle: 'solid', | ||
borderColor: '#00e676' | ||
@@ -31,3 +35,2 @@ }; | ||
const rejectStyle = { | ||
borderStyle: 'solid', | ||
borderColor: '#ff1744' | ||
@@ -56,5 +59,7 @@ }; | ||
return ( | ||
<div {...getRootProps({style})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
<div className="container"> | ||
<div {...getRootProps({style})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
</div> | ||
</div> | ||
@@ -82,15 +87,21 @@ ); | ||
if (props.isDragActive) { | ||
return '#6c6'; | ||
return '#2196f3'; | ||
} | ||
return '#666'; | ||
return '#eeeeee'; | ||
} | ||
const Container = styled.div` | ||
width: 200px; | ||
height: 200px; | ||
flex: 1; | ||
display: flex; | ||
flex-direction: column; | ||
align-items: center; | ||
padding: 20px; | ||
border-width: 2px; | ||
border-radius: 5px; | ||
border-radius: 2px; | ||
border-color: ${props => getColor(props)}; | ||
border-style: ${props => props.isDragActive ? 'solid' : 'dashed'}; | ||
background-color: ${props => props.isDragActive ? '#eee' : ''}; | ||
border-style: dashed; | ||
background-color: #fafafa; | ||
color: #bdbdbd; | ||
outline: none; | ||
transition: border .24s ease-in-out; | ||
`; | ||
@@ -108,6 +119,8 @@ | ||
return ( | ||
<Container {...getRootProps({isDragActive, isDragAccept, isDragReject})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
</Container> | ||
<div className="container"> | ||
<Container {...getRootProps({isDragActive, isDragAccept, isDragReject})}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
</Container> | ||
</div> | ||
); | ||
@@ -114,0 +127,0 @@ } |
@@ -168,3 +168,3 @@ { | ||
}, | ||
"version": "10.1.0", | ||
"version": "10.1.1", | ||
"engines": { | ||
@@ -171,0 +171,0 @@ "node": ">= 8" |
@@ -153,2 +153,4 @@ 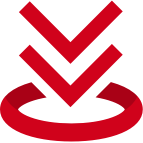 | ||
import {useDropzone} from 'react-dropzone' | ||
// NOTE: After v4.0.0, styled components exposes a ref using forwardRef, | ||
// therefore, no need for using innerRef as refKey | ||
import styled from 'styled-components' | ||
@@ -168,2 +170,22 @@ | ||
If you're working with [Material UI](https://material-ui.com) and would like to apply the root props on some component that does not expose a ref, use [RootRef](https://material-ui.com/api/root-ref): | ||
```jsx static | ||
import React from 'react' | ||
import {useDropzone} from 'react-dropzone' | ||
import RootRef from '@material-ui/core/RootRef' | ||
function PaperDropzone() { | ||
const {getRootProps, getInputProps} = useDropzone() | ||
const {ref, ...rootProps} = getRootProps() | ||
<RootRef rootRef={ref}> | ||
<Paper {...rootProps}> | ||
<input {...getInputProps()} /> | ||
<p>Drag 'n' drop some files here, or click to select files</p> | ||
</Paper> | ||
</RootRef> | ||
} | ||
``` | ||
*Important*: do not set the `ref` prop on the elements where `getRootProps()`/`getInputProps()` props are set, instead, get the refs from the hook itself: | ||
@@ -170,0 +192,0 @@ |
@@ -57,3 +57,3 @@ /* eslint prefer-template: 0 */ | ||
} | ||
}) | ||
}, [ref]) | ||
@@ -60,0 +60,0 @@ // TODO: Figure out why react-styleguidist cannot create docs if we don't return a jsx element |
/* eslint import/no-extraneous-dependencies: 0 */ | ||
const path = require('path') | ||
const { createConfig, babel } = require('webpack-blocks') | ||
const { createConfig, babel, css } = require('webpack-blocks') | ||
@@ -9,3 +9,3 @@ // https://react-styleguidist.js.org/docs/configuration.html | ||
styleguideDir: path.join(__dirname, 'styleguide'), | ||
webpackConfig: createConfig([babel()]), | ||
webpackConfig: createConfig([babel(), css()]), | ||
exampleMode: 'expand', | ||
@@ -18,2 +18,3 @@ usageMode: 'expand', | ||
}, | ||
require: [path.join(__dirname, 'examples/theme.css')], | ||
sections: [ | ||
@@ -20,0 +21,0 @@ { |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
333636
4937
378