react-dropzone
Advanced tools
Comparing version 10.1.9 to 10.1.10
@@ -13,8 +13,10 @@ function _toConsumableArray(arr) { return _arrayWithoutHoles(arr) || _iterableToArray(arr) || _nonIterableSpread(); } | ||
function _iterableToArrayLimit(arr, i) { var _arr = []; var _n = true; var _d = false; var _e = undefined; try { for (var _i = arr[Symbol.iterator](), _s; !(_n = (_s = _i.next()).done); _n = true) { _arr.push(_s.value); if (i && _arr.length === i) break; } } catch (err) { _d = true; _e = err; } finally { try { if (!_n && _i["return"] != null) _i["return"](); } finally { if (_d) throw _e; } } return _arr; } | ||
function _iterableToArrayLimit(arr, i) { if (!(Symbol.iterator in Object(arr) || Object.prototype.toString.call(arr) === "[object Arguments]")) { return; } var _arr = []; var _n = true; var _d = false; var _e = undefined; try { for (var _i = arr[Symbol.iterator](), _s; !(_n = (_s = _i.next()).done); _n = true) { _arr.push(_s.value); if (i && _arr.length === i) break; } } catch (err) { _d = true; _e = err; } finally { try { if (!_n && _i["return"] != null) _i["return"](); } finally { if (_d) throw _e; } } return _arr; } | ||
function _arrayWithHoles(arr) { if (Array.isArray(arr)) return arr; } | ||
function _objectSpread(target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i] != null ? arguments[i] : {}; var ownKeys = Object.keys(source); if (typeof Object.getOwnPropertySymbols === 'function') { ownKeys = ownKeys.concat(Object.getOwnPropertySymbols(source).filter(function (sym) { return Object.getOwnPropertyDescriptor(source, sym).enumerable; })); } ownKeys.forEach(function (key) { _defineProperty(target, key, source[key]); }); } return target; } | ||
function ownKeys(object, enumerableOnly) { var keys = Object.keys(object); if (Object.getOwnPropertySymbols) { var symbols = Object.getOwnPropertySymbols(object); if (enumerableOnly) symbols = symbols.filter(function (sym) { return Object.getOwnPropertyDescriptor(object, sym).enumerable; }); keys.push.apply(keys, symbols); } return keys; } | ||
function _objectSpread(target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i] != null ? arguments[i] : {}; if (i % 2) { ownKeys(source, true).forEach(function (key) { _defineProperty(target, key, source[key]); }); } else if (Object.getOwnPropertyDescriptors) { Object.defineProperties(target, Object.getOwnPropertyDescriptors(source)); } else { ownKeys(source).forEach(function (key) { Object.defineProperty(target, key, Object.getOwnPropertyDescriptor(source, key)); }); } } return target; } | ||
function _defineProperty(obj, key, value) { if (key in obj) { Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true }); } else { obj[key] = value; } return obj; } | ||
@@ -313,76 +315,76 @@ | ||
rejectedFiles: [] | ||
/** | ||
* A React hook that creates a drag 'n' drop area. | ||
* | ||
* ```jsx | ||
* function MyDropzone(props) { | ||
* const {getRootProps, getInputProps} = useDropzone({ | ||
* onDrop: acceptedFiles => { | ||
* // do something with the File objects, e.g. upload to some server | ||
* } | ||
* }); | ||
* return ( | ||
* <div {...getRootProps()}> | ||
* <input {...getInputProps()} /> | ||
* <p>Drag and drop some files here, or click to select files</p> | ||
* </div> | ||
* ) | ||
* } | ||
* ``` | ||
* | ||
* @function useDropzone | ||
* | ||
* @param {object} props | ||
* @param {string|string[]} [props.accept] Set accepted file types. | ||
* See https://github.com/okonet/attr-accept for more information. | ||
* Keep in mind that mime type determination is not reliable across platforms. CSV files, | ||
* for example, are reported as text/plain under macOS but as application/vnd.ms-excel under | ||
* Windows. In some cases there might not be a mime type set at all. | ||
* See: https://github.com/react-dropzone/react-dropzone/issues/276 | ||
* @param {boolean} [props.multiple=true] Allow drag 'n' drop (or selection from the file dialog) of multiple files | ||
* @param {boolean} [props.preventDropOnDocument=true] If false, allow dropped items to take over the current browser window | ||
* @param {boolean} [props.noClick=false] If true, disables click to open the native file selection dialog | ||
* @param {boolean} [props.noKeyboard=false] If true, disables SPACE/ENTER to open the native file selection dialog. | ||
* Note that it also stops tracking the focus state. | ||
* @param {boolean} [props.noDrag=false] If true, disables drag 'n' drop | ||
* @param {boolean} [props.noDragEventsBubbling=false] If true, stops drag event propagation to parents | ||
* @param {number} [props.minSize=0] Minimum file size (in bytes) | ||
* @param {number} [props.maxSize=Infinity] Maximum file size (in bytes) | ||
* @param {boolean} [props.disabled=false] Enable/disable the dropzone | ||
* @param {getFilesFromEvent} [props.getFilesFromEvent] Use this to provide a custom file aggregator | ||
* @param {Function} [props.onFileDialogCancel] Cb for when closing the file dialog with no selection | ||
* @param {dragCb} [props.onDragEnter] Cb for when the `dragenter` event occurs. | ||
* @param {dragCb} [props.onDragLeave] Cb for when the `dragleave` event occurs | ||
* @param {dragCb} [props.onDragOver] Cb for when the `dragover` event occurs | ||
* @param {dropCb} [props.onDrop] Cb for when the `drop` event occurs. | ||
* Note that this callback is invoked after the `getFilesFromEvent` callback is done. | ||
* | ||
* Files are accepted or rejected based on the `accept`, `multiple`, `minSize` and `maxSize` props. | ||
* `accept` must be a valid [MIME type](http://www.iana.org/assignments/media-types/media-types.xhtml) according to [input element specification](https://www.w3.org/wiki/HTML/Elements/input/file) or a valid file extension. | ||
* If `multiple` is set to false and additional files are droppped, | ||
* all files besides the first will be rejected. | ||
* Any file which does not have a size in the [`minSize`, `maxSize`] range, will be rejected as well. | ||
* | ||
* Note that the `onDrop` callback will always be invoked regardless if the dropped files were accepted or rejected. | ||
* If you'd like to react to a specific scenario, use the `onDropAccepted`/`onDropRejected` props. | ||
* | ||
* `onDrop` will provide you with an array of [File](https://developer.mozilla.org/en-US/docs/Web/API/File) objects which you can then process and send to a server. | ||
* For example, with [SuperAgent](https://github.com/visionmedia/superagent) as a http/ajax library: | ||
* | ||
* ```js | ||
* function onDrop(acceptedFiles) { | ||
* const req = request.post('/upload') | ||
* acceptedFiles.forEach(file => { | ||
* req.attach(file.name, file) | ||
* }) | ||
* req.end(callback) | ||
* } | ||
* ``` | ||
* @param {dropAcceptedCb} [props.onDropAccepted] | ||
* @param {dropRejectedCb} [props.onDropRejected] | ||
* | ||
* @returns {DropzoneState} | ||
*/ | ||
}; | ||
/** | ||
* A React hook that creates a drag 'n' drop area. | ||
* | ||
* ```jsx | ||
* function MyDropzone(props) { | ||
* const {getRootProps, getInputProps} = useDropzone({ | ||
* onDrop: acceptedFiles => { | ||
* // do something with the File objects, e.g. upload to some server | ||
* } | ||
* }); | ||
* return ( | ||
* <div {...getRootProps()}> | ||
* <input {...getInputProps()} /> | ||
* <p>Drag and drop some files here, or click to select files</p> | ||
* </div> | ||
* ) | ||
* } | ||
* ``` | ||
* | ||
* @function useDropzone | ||
* | ||
* @param {object} props | ||
* @param {string|string[]} [props.accept] Set accepted file types. | ||
* See https://github.com/okonet/attr-accept for more information. | ||
* Keep in mind that mime type determination is not reliable across platforms. CSV files, | ||
* for example, are reported as text/plain under macOS but as application/vnd.ms-excel under | ||
* Windows. In some cases there might not be a mime type set at all. | ||
* See: https://github.com/react-dropzone/react-dropzone/issues/276 | ||
* @param {boolean} [props.multiple=true] Allow drag 'n' drop (or selection from the file dialog) of multiple files | ||
* @param {boolean} [props.preventDropOnDocument=true] If false, allow dropped items to take over the current browser window | ||
* @param {boolean} [props.noClick=false] If true, disables click to open the native file selection dialog | ||
* @param {boolean} [props.noKeyboard=false] If true, disables SPACE/ENTER to open the native file selection dialog. | ||
* Note that it also stops tracking the focus state. | ||
* @param {boolean} [props.noDrag=false] If true, disables drag 'n' drop | ||
* @param {boolean} [props.noDragEventsBubbling=false] If true, stops drag event propagation to parents | ||
* @param {number} [props.minSize=0] Minimum file size (in bytes) | ||
* @param {number} [props.maxSize=Infinity] Maximum file size (in bytes) | ||
* @param {boolean} [props.disabled=false] Enable/disable the dropzone | ||
* @param {getFilesFromEvent} [props.getFilesFromEvent] Use this to provide a custom file aggregator | ||
* @param {Function} [props.onFileDialogCancel] Cb for when closing the file dialog with no selection | ||
* @param {dragCb} [props.onDragEnter] Cb for when the `dragenter` event occurs. | ||
* @param {dragCb} [props.onDragLeave] Cb for when the `dragleave` event occurs | ||
* @param {dragCb} [props.onDragOver] Cb for when the `dragover` event occurs | ||
* @param {dropCb} [props.onDrop] Cb for when the `drop` event occurs. | ||
* Note that this callback is invoked after the `getFilesFromEvent` callback is done. | ||
* | ||
* Files are accepted or rejected based on the `accept`, `multiple`, `minSize` and `maxSize` props. | ||
* `accept` must be a valid [MIME type](http://www.iana.org/assignments/media-types/media-types.xhtml) according to [input element specification](https://www.w3.org/wiki/HTML/Elements/input/file) or a valid file extension. | ||
* If `multiple` is set to false and additional files are droppped, | ||
* all files besides the first will be rejected. | ||
* Any file which does not have a size in the [`minSize`, `maxSize`] range, will be rejected as well. | ||
* | ||
* Note that the `onDrop` callback will always be invoked regardless if the dropped files were accepted or rejected. | ||
* If you'd like to react to a specific scenario, use the `onDropAccepted`/`onDropRejected` props. | ||
* | ||
* `onDrop` will provide you with an array of [File](https://developer.mozilla.org/en-US/docs/Web/API/File) objects which you can then process and send to a server. | ||
* For example, with [SuperAgent](https://github.com/visionmedia/superagent) as a http/ajax library: | ||
* | ||
* ```js | ||
* function onDrop(acceptedFiles) { | ||
* const req = request.post('/upload') | ||
* acceptedFiles.forEach(file => { | ||
* req.attach(file.name, file) | ||
* }) | ||
* req.end(callback) | ||
* } | ||
* ``` | ||
* @param {dropAcceptedCb} [props.onDropAccepted] | ||
* @param {dropRejectedCb} [props.onDropRejected] | ||
* | ||
* @returns {DropzoneState} | ||
*/ | ||
}; | ||
export function useDropzone() { | ||
@@ -698,3 +700,3 @@ var _ref2 = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}, | ||
tabIndex: 0 | ||
} : {}, rest); | ||
} : {}, {}, rest); | ||
}; | ||
@@ -727,3 +729,3 @@ }, [rootRef, onKeyDownCb, onFocusCb, onBlurCb, onClickCb, onDragEnterCb, onDragOverCb, onDragLeaveCb, onDropCb, noKeyboard, noDrag, disabled]); | ||
return _objectSpread({}, inputProps, rest); | ||
return _objectSpread({}, inputProps, {}, rest); | ||
}; | ||
@@ -733,3 +735,3 @@ }, [inputRef, accept, multiple, onDropCb, disabled]); | ||
var isMultipleAllowed = multiple || fileCount <= 1; | ||
var isDragAccept = fileCount > 0 && allFilesAccepted(draggedFiles, accept); | ||
var isDragAccept = fileCount > 0 && allFilesAccepted(draggedFiles, accept, maxSize, minSize); | ||
var isDragReject = fileCount > 0 && (!isDragAccept || !isMultipleAllowed); | ||
@@ -736,0 +738,0 @@ return _objectSpread({}, state, { |
@@ -12,5 +12,5 @@ function _typeof(obj) { if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") { _typeof = function _typeof(obj) { return typeof obj; }; } else { _typeof = function _typeof(obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; } return _typeof(obj); } | ||
} | ||
export function allFilesAccepted(files, accept) { | ||
export function allFilesAccepted(files, accept, maxSize, minSize) { | ||
return files.every(function (file) { | ||
return fileAccepted(file, accept); | ||
return fileAccepted(file, accept) && fileMatchSize(file, maxSize, minSize); | ||
}); | ||
@@ -17,0 +17,0 @@ } // React's synthetic events has event.isPropagationStopped, |
@@ -115,4 +115,8 @@ { | ||
"@commitlint/prompt-cli": "^7.0.0", | ||
"@types/react": "^16.8.3", | ||
"@types/react-dom": "^16.8.1", | ||
"@testing-library/dom": "^6.5.0", | ||
"@testing-library/jest-dom": "^4.1.0", | ||
"@testing-library/react": "^9.3.0", | ||
"@testing-library/react-hooks": "^2.0.3", | ||
"@types/react": "^16.9.0", | ||
"@types/react-dom": "^16.9.0", | ||
"babel-eslint": "*", | ||
@@ -142,8 +146,6 @@ "babel-jest": "^24.1.0", | ||
"prettier": "*", | ||
"react": "^16.8.2", | ||
"react-dom": "^16.8.2", | ||
"react-hooks-testing-library": "^0.3.4", | ||
"react": "^16.9.0", | ||
"react-dom": "^16.9.0", | ||
"react-styleguidist": "^9.0.1", | ||
"react-test-renderer": "^16.8.2", | ||
"react-testing-library": "^6.0.0", | ||
"react-test-renderer": "^16.9.0", | ||
"rimraf": "^2.5.2", | ||
@@ -170,3 +172,3 @@ "rollup": "^1.3.0", | ||
}, | ||
"version": "10.1.9", | ||
"version": "10.1.10", | ||
"engines": { | ||
@@ -173,0 +175,0 @@ "node": ">= 8" |
@@ -95,3 +95,3 @@ 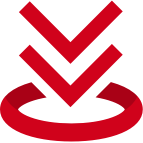 | ||
acceptedFiles.forEach(file => reader.readAsBinaryString(file)) | ||
acceptedFiles.forEach(file => reader.readAsArrayBuffer(file)) | ||
}, []) | ||
@@ -98,0 +98,0 @@ const {getRootProps, getInputProps, isDragActive} = useDropzone({onDrop}) |
@@ -728,3 +728,3 @@ /* eslint prefer-template: 0 */ | ||
const isMultipleAllowed = multiple || fileCount <= 1 | ||
const isDragAccept = fileCount > 0 && allFilesAccepted(draggedFiles, accept) | ||
const isDragAccept = fileCount > 0 && allFilesAccepted(draggedFiles, accept, maxSize, minSize) | ||
const isDragReject = fileCount > 0 && (!isDragAccept || !isMultipleAllowed) | ||
@@ -731,0 +731,0 @@ |
@@ -13,4 +13,6 @@ import accepts from 'attr-accept' | ||
export function allFilesAccepted(files, accept) { | ||
return files.every(file => fileAccepted(file, accept)) | ||
export function allFilesAccepted(files, accept, maxSize, minSize) { | ||
return files.every(file => { | ||
return fileAccepted(file, accept) && fileMatchSize(file, maxSize, minSize) | ||
}) | ||
} | ||
@@ -17,0 +19,0 @@ |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
336810
4976
65