react-dropzone
Advanced tools
Comparing version 6.2.4 to 7.0.0
@@ -214,3 +214,2 @@ var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; | ||
multiple = _props.multiple, | ||
disablePreview = _props.disablePreview, | ||
accept = _props.accept, | ||
@@ -249,6 +248,2 @@ getDataTransferItems = _props.getDataTransferItems; | ||
fileList.forEach(function (file) { | ||
if (!disablePreview) { | ||
file.preview = window.URL.createObjectURL(file); // eslint-disable-line no-param-reassign | ||
} | ||
if (fileAccepted(file, accept) && fileMatchSize(file, _this4.props.maxSize, _this4.props.minSize)) { | ||
@@ -470,3 +465,2 @@ acceptedFiles.push(file); | ||
preventDropOnDocument = props.preventDropOnDocument, | ||
disablePreview = props.disablePreview, | ||
disableClick = props.disableClick, | ||
@@ -479,3 +473,3 @@ onDropAccepted = props.onDropAccepted, | ||
getDataTransferItems = props.getDataTransferItems, | ||
divProps = _objectWithoutProperties(props, ['acceptedFiles', 'preventDropOnDocument', 'disablePreview', 'disableClick', 'onDropAccepted', 'onDropRejected', 'onFileDialogCancel', 'maxSize', 'minSize', 'getDataTransferItems']); | ||
divProps = _objectWithoutProperties(props, ['acceptedFiles', 'preventDropOnDocument', 'disableClick', 'onDropAccepted', 'onDropRejected', 'onFileDialogCancel', 'maxSize', 'minSize', 'getDataTransferItems']); | ||
/* eslint-enable no-unused-vars */ | ||
@@ -541,7 +535,2 @@ | ||
/** | ||
* Enable/disable preview generation | ||
*/ | ||
disablePreview: PropTypes.bool, | ||
/** | ||
* If false, allow dropped items to take over the current browser window | ||
@@ -683,3 +672,2 @@ */ | ||
disabled: false, | ||
disablePreview: false, | ||
disableClick: false, | ||
@@ -686,0 +674,0 @@ inputProps: {}, |
@@ -50,3 +50,7 @@ { | ||
"setupTestFrameworkScriptFile": "<rootDir>/testSetup.js", | ||
"clearMocks": true | ||
"clearMocks": true, | ||
"coveragePathIgnorePatterns": [ | ||
"/node_modules/", | ||
"<rootDir>/testSetup.js" | ||
] | ||
}, | ||
@@ -147,3 +151,3 @@ "keywords": [ | ||
}, | ||
"version": "6.2.4", | ||
"version": "7.0.0", | ||
"engines": { | ||
@@ -150,0 +154,0 @@ "node": ">= 6" |
@@ -6,5 +6,5 @@ 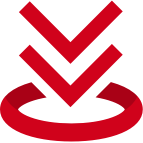 | ||
[](https://www.npmjs.com/package/react-dropzone) | ||
[](https://travis-ci.org/react-dropzone/react-dropzone) | ||
[](https://codecov.io/gh/react-dropzone/react-dropzone) | ||
[](#backers) | ||
[](https://travis-ci.org/react-dropzone/react-dropzone) | ||
[](https://codecov.io/gh/react-dropzone/react-dropzone) | ||
[](#backers) | ||
[](#sponsors) | ||
@@ -41,6 +41,6 @@ | ||
import Dropzone from 'react-dropzone' | ||
``` | ||
``` | ||
and specify the `onDrop` method that accepts two arguments. The first argument represents the accepted files and the second argument the rejected files. | ||
```javascript static | ||
@@ -50,3 +50,3 @@ function onDrop(acceptedFiles, rejectedFiles) { | ||
} | ||
``` | ||
``` | ||
@@ -94,6 +94,2 @@ Files accepted or rejected based on `accept` prop. This must be a valid [MIME type](http://www.iana.org/assignments/media-types/media-types.xhtml) according to [input element specification](https://www.w3.org/wiki/HTML/Elements/input/file). | ||
### Word of caution when working with previews | ||
*Important*: `react-dropzone` doesn't manage dropped files. You need to destroy the object URL yourself whenever you don't need the `preview` by calling `window.URL.revokeObjectURL(file.preview);` to avoid memory leaks. | ||
### Testing | ||
@@ -119,3 +115,3 @@ | ||
dropzone.update() | ||
const child = updatedDropzone.find(DummyChildComponent) | ||
@@ -122,0 +118,0 @@ expect(child).toHaveProp('isDragActive', true) |
@@ -171,3 +171,2 @@ /* eslint prefer-template: 0 */ | ||
multiple, | ||
disablePreview, | ||
accept, | ||
@@ -206,6 +205,2 @@ getDataTransferItems | ||
fileList.forEach(file => { | ||
if (!disablePreview) { | ||
file.preview = window.URL.createObjectURL(file) // eslint-disable-line no-param-reassign | ||
} | ||
if ( | ||
@@ -440,3 +435,2 @@ fileAccepted(file, accept) && | ||
preventDropOnDocument, | ||
disablePreview, | ||
disableClick, | ||
@@ -511,7 +505,2 @@ onDropAccepted, | ||
/** | ||
* Enable/disable preview generation | ||
*/ | ||
disablePreview: PropTypes.bool, | ||
/** | ||
* If false, allow dropped items to take over the current browser window | ||
@@ -653,3 +642,2 @@ */ | ||
disabled: false, | ||
disablePreview: false, | ||
disableClick: false, | ||
@@ -656,0 +644,0 @@ inputProps: {}, |
@@ -1044,47 +1044,2 @@ /* eslint jsx-a11y/click-events-have-key-events: 0 */ | ||
describe('preview', () => { | ||
const expectedEvent = expect.anything() | ||
it('should generate previews for non-images', async () => { | ||
const onDrop = jest.fn() | ||
const dropzone = mount(<Dropzone onDrop={onDrop} />) | ||
await dropzone.simulate('drop', createDtWithFiles(files)) | ||
expect(onDrop).toHaveBeenCalledWith( | ||
expect.arrayContaining([expect.objectContaining({ preview: 'data://file1.pdf' })]), | ||
[], | ||
expectedEvent | ||
) | ||
}) | ||
it('should generate previews for images', async () => { | ||
const onDrop = jest.fn() | ||
const dropzone = mount(<Dropzone onDrop={onDrop} />) | ||
await dropzone.simulate('drop', createDtWithFiles(images)) | ||
expect(onDrop).toHaveBeenCalledWith( | ||
expect.arrayContaining([expect.objectContaining({ preview: 'data://cats.gif' })]), | ||
[], | ||
expectedEvent | ||
) | ||
}) | ||
it('should not generate previews if disablePreview is true', async () => { | ||
const onDrop = jest.fn() | ||
const dropzone = mount(<Dropzone disablePreview onDrop={onDrop} />) | ||
await dropzone.simulate('drop', createDtWithFiles(images)) | ||
expect(onDrop).not.toHaveBeenCalledWith( | ||
expect.arrayContaining([expect.objectContaining({ preview: expect.anything() })]), | ||
[], | ||
expectedEvent | ||
) | ||
onDrop.mockClear() | ||
await dropzone.simulate('drop', createDtWithFiles(files)) | ||
expect(onDrop).not.toHaveBeenCalledWith( | ||
expect.arrayContaining([expect.objectContaining({ preview: expect.anything() })]), | ||
[], | ||
expectedEvent | ||
) | ||
}) | ||
}) | ||
describe('onCancel', () => { | ||
@@ -1091,0 +1046,0 @@ beforeEach(() => { |
@@ -41,2 +41,6 @@ /* eslint import/no-extraneous-dependencies: 0 */ | ||
{ | ||
name: 'Previews', | ||
content: 'examples/Previews/Readme.md' | ||
}, | ||
{ | ||
name: 'Nested Dropzone', | ||
@@ -43,0 +47,0 @@ content: 'examples/Nesting/Readme.md' |
@@ -9,7 +9,1 @@ /* eslint prefer-template: 0 */ | ||
Enzyme.configure({ adapter: new Adapter() }) | ||
global.window.URL = { | ||
createObjectURL: function createObjectURL(arg) { | ||
return `data://${arg.name}` | ||
} | ||
} |
import * as React from "react"; | ||
import {func} from "prop-types"; | ||
export interface FileWithPreview extends File { | ||
preview?: string; | ||
} | ||
export type DropFileEventHandler = ( | ||
acceptedOrRejected: FileWithPreview[], | ||
acceptedOrRejected: File[], | ||
event: React.DragEvent<HTMLDivElement> | ||
) => void; | ||
export type DropFilesEventHandler = ( | ||
accepted: FileWithPreview[], | ||
rejected: FileWithPreview[], | ||
accepted: File[], | ||
rejected: File[], | ||
event: React.DragEvent<HTMLDivElement> | ||
@@ -18,5 +15,5 @@ ) => void; | ||
type DropzoneRenderArgs = { | ||
draggedFiles: FileWithPreview[]; | ||
acceptedFiles: FileWithPreview[]; | ||
rejectedFiles: FileWithPreview[]; | ||
draggedFiles: File[]; | ||
acceptedFiles: File[]; | ||
rejectedFiles: File[]; | ||
isDragActive: boolean; | ||
@@ -35,3 +32,2 @@ isDragAccept: boolean; | ||
disabled?: boolean; | ||
disablePreview?: boolean; | ||
preventDropOnDocument?: boolean; | ||
@@ -53,3 +49,3 @@ inputProps?: React.InputHTMLAttributes<HTMLInputElement>; | ||
onFileDialogCancel?(): void; | ||
getDataTransferItems?(event: React.DragEvent<HTMLDivElement> | DragEvent | React.ChangeEvent<HTMLInputElement> | Event): Promise<Array<File | DataTransferItem>>; | ||
getDataTransferItems?(event: React.DragEvent<HTMLDivElement> | React.ChangeEvent<HTMLInputElement> | DragEvent | Event): Promise<Array<File | DataTransferItem>>; | ||
children?: React.ReactNode | DropzoneRenderFunction; | ||
@@ -56,0 +52,0 @@ ref?: React.Ref<Dropzone>; |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
46
231053
3650
195