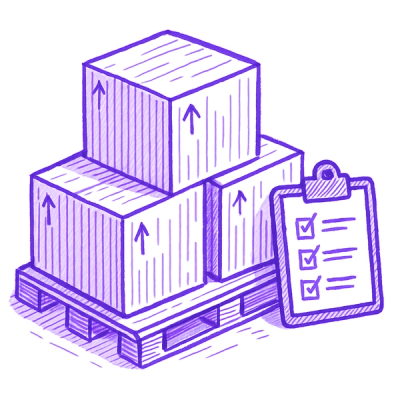
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
react-fusioncharts
Advanced tools
Simple and Lightweight React component for FusionCharts JavaScript Charting Library
A simple and lightweight official React component for FusionCharts JavaScript charting library. react-fusioncharts
enables you to add JavaScript charts in your React application or project without any hassle.
The FusionCharts React integration component has been verified and validated with different versions of Fusioncharts (3.19 / 3.18 / 3.17) with React versions 16/17/18
There are multiple ways to install react-fusioncharts
component.
Direct Download All binaries are located on our github repository.
Install from NPM
npm install --save react-fusioncharts
See npm documentation to know more about npm usage.
Install from Yarn
yarn add react-fusioncharts
See yarn documentation to know more about yarn usage.
For general instructions, refer to this developer docs page.
create-react-app
Import React, react-fusioncharts
and FusionCharts in your app:
import React from 'react';
import ReactDOM from 'react-dom';
import FusionCharts from 'fusioncharts';
import Charts from 'fusioncharts/fusioncharts.charts';
import ReactFC from 'react-fusioncharts';
ReactFC.fcRoot(FusionCharts, Charts);
webpack
or parcel
Import React, react-fusioncharts
and FusionCharts in your app:
import React from 'react';
import ReactDOM from 'react-dom';
import FusionCharts from 'fusioncharts/core';
import Column2d from 'fusioncharts/viz/column2d';
import ReactFC from 'react-fusioncharts';
ReactFC.fcRoot(FusionCharts, Column2d);
Note: This way of import will not work in IE11 and below.
Here is a basic sample that shows how to create a chart using react-fusioncharts
:
import React from 'react';
import ReactDOM from 'react-dom';
import FusionCharts from 'fusioncharts';
import Charts from 'fusioncharts/fusioncharts.charts';
import FusionTheme from 'fusioncharts/themes/fusioncharts.theme.fusion';
import ReactFC from 'react-fusioncharts';
ReactFC.fcRoot(FusionCharts, Charts, FusionTheme);
const dataSource = {
chart: {
caption: 'Countries With Most Oil Reserves [2017-18]',
subCaption: 'In MMbbl = One Million barrels',
xAxisName: 'Country',
yAxisName: 'Reserves (MMbbl)',
numberSuffix: 'K',
theme: 'fusion'
},
data: [
{ label: 'Venezuela', value: '290' },
{ label: 'Saudi', value: '260' },
{ label: 'Canada', value: '180' },
{ label: 'Iran', value: '140' },
{ label: 'Russia', value: '115' },
{ label: 'UAE', value: '100' },
{ label: 'US', value: '30' },
{ label: 'China', value: '30' }
]
};
const chartConfigs = {
type: 'column2d',
width: 600,
height: 400,
dataFormat: 'json',
dataSource: dataSource
};
ReactDOM.render(<ReactFC {...chartConfigs} />, document.getElementById('root'));
To render a map, import the FusionMaps module along with the map definition.
import React from 'react';
import ReactDOM from 'react-dom';
import FusionCharts from 'fusioncharts';
import Maps from 'fusioncharts/fusioncharts.maps';
import World from 'fusioncharts/maps/fusioncharts.world';
import FusionTheme from 'fusioncharts/themes/fusioncharts.theme.fusion';
import ReactFC from 'react-fusioncharts';
ReactFC.fcRoot(FusionCharts, Maps, World, FusionTheme);
// Data Source B
const dataSource = {
chart: {
caption: 'Average Annual Population Growth',
subcaption: ' 1955-2015',
numbersuffix: '%',
includevalueinlabels: '1',
labelsepchar: ': ',
entityFillHoverColor: '#FFF9C4',
theme: 'fusion'
},
colorrange: {
minvalue: '0',
code: '#FFE0B2',
gradient: '1',
color: [
{ minvalue: '0.5', maxvalue: '1.0', color: '#FFD74D' },
{ minvalue: '1.0', maxvalue: '2.0', color: '#FB8C00' },
{ minvalue: '2.0', maxvalue: '3.0', color: '#E65100' }
]
},
data: [
{ id: 'NA', value: '.82', showLabel: '1' },
{ id: 'SA', value: '2.04', showLabel: '1' },
{ id: 'AS', value: '1.78', showLabel: '1' },
{ id: 'EU', value: '.40', showLabel: '1' },
{ id: 'AF', value: '2.58', showLabel: '1' },
{ id: 'AU', value: '1.30', showLabel: '1' }
]
};
const chartConfigs = {
type: 'world',
width: 600,
height: 400,
dataFormat: 'json',
dataSource: dataSource
};
ReactDOM.render(<ReactFC {...chartConfigs} />, document.getElementById('root'));
To attach event callbacks to a FusionCharts component, follow the pattern below.
Write the callback:
As a separate function:
var chartEventCallback = function (eventObj, dataObj) {
[Code goes here]
}
Or, as a component class method:
chartEventCallback (eventObj, dataObj) {
[Code goes here]
}
Attach the callback to an event through the React-FC component:
<ReactFC {...chartConfigs} fcEvent-EVENTNAME={this.chartEventCallback} />
Where, EVENTNAME is to be replaced by the event you want to track.
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import FusionCharts from 'fusioncharts';
import Charts from 'fusioncharts/fusioncharts.charts';
import ReactFC from 'react-fusioncharts';
import FusionTheme from 'fusioncharts/Charts/fusioncharts.theme.fusion';
ReactFC.fcRoot(FusionCharts, Charts, FusionTheme);
const dataSource = /* Data Source A , given above */;
const chartConfigs = {
type: 'column2d',
width: 600,
height: 400,
dataFormat: 'json',
dataSource: dataSource
};
class Chart extends Component {
constructor(props) {
super(props);
this.state = {
actualValue: 'Hover on the plot to see the value along with the label'
};
this.showPlotValue = this.showPlotValue.bind(this);
}
// Event callback handler for 'dataplotRollOver'.
// Shows the value of the hovered plot on the page.
showPlotValue(eventObj, dataObj) {
this.setState({
actualValue: `You’re are currently hovering over ${
dataObj.categoryLabel
} whose value is ${dataObj.displayValue}`
});
}
render() {
return (
<div>
<ReactFC
{...chartConfigs}
fcEvent-dataplotRollOver={this.showPlotValue}
/>
<p style={{ padding: '10px', background: '#f5f2f0' }}>
{this.state.actualValue}
</p>
</div>
);
}
}
ReactDOM.render(<Chart />, document.getElementById('root'));
From fusioncharts@3.13.3-sr.1
and react-fusioncharts@3.0.0
, You can visualize timeseries data easily on react.
Learn more about FusionTime here.
import React from 'react';
import FusionCharts from 'fusioncharts';
import TimeSeries from 'fusioncharts/fusioncharts.timeseries';
import ReactFC from 'react-fusioncharts';
ReactFC.fcRoot(FusionCharts, TimeSeries);
const jsonify = res => res.json();
const dataFetch = fetch(
'https://raw.githubusercontent.com/fusioncharts/dev_centre_docs/fusiontime-beta-release/charts-resources/fusiontime/online-sales-single-series/data.json'
).then(jsonify);
const schemaFetch = fetch(
'https://raw.githubusercontent.com/fusioncharts/dev_centre_docs/fusiontime-beta-release/charts-resources/fusiontime/online-sales-single-series/schema.json'
).then(jsonify);
class ChartViewer extends React.Component {
constructor(props) {
super(props);
this.onFetchData = this.onFetchData.bind(this);
this.state = {
timeseriesDs: {
type: 'timeseries',
renderAt: 'container',
width: '600',
height: '400',
dataSource: {
caption: { text: 'Online Sales of a SuperStore in the US' },
data: null,
yAxis: [
{
plot: [
{
value: 'Sales ($)'
}
]
}
]
}
}
};
}
componentDidMount() {
this.onFetchData();
}
onFetchData() {
Promise.all([dataFetch, schemaFetch]).then(res => {
const data = res[0];
const schema = res[1];
const fusionTable = new FusionCharts.DataStore().createDataTable(
data,
schema
);
const timeseriesDs = Object.assign({}, this.state.timeseriesDs);
timeseriesDs.dataSource.data = fusionTable;
this.setState({
timeseriesDs
});
});
}
render() {
return (
<div>
{this.state.timeseriesDs.dataSource.data ? (
<ReactFC {...this.state.timeseriesDs} />
) : (
'loading'
)}
</div>
);
}
}
Useful links for FusionTime
A custom component to easily add drill down to your react application.
import FusionCharts from 'fusioncharts';
import Charts from 'fusioncharts/fusioncharts.charts';
import ReactFC from 'react-fusioncharts';
import DrillDown from 'react-fusioncharts/components/DrillDown';
DrillDown.fcRoot(FusionCharts, Charts);
class MyComponent extends React.Component{
constructor(props){
super(props);
this.plotChildMap = [ 0, 2, 1 ];
this.dataSource = /*Data Source A : Given above */ ;
this.btnConfig = {text : 'Back'};
this.type= 'column2d';
this.height = 400;
this.width = 400;
}
render(){
return (
<DrillDown
dataSource={dataSource}
plotChildMap={plotChildMap}
btnConfig={btnConfig}
btnStyle={btnStyle}
dataFormat={dataFormat}
type={type}
height={height}
width={width}
...other
>
<ReactFC /> /* ReactFC as a child */
<ReactFC />
...
<DrillDown></DrillDown> /* DrillDown as a child for next level drill down*/
</DrillDown>
)
}
}
plotChildMap[Array(Number)| Array[Object]]
DrillDown
component . Array location are considered plot index of parent, the value corresponding to it are considered which child chart to render.
Eg. [0,2,1]
0(location) -> 0 (value)
means clicking the first (zero indexed) data plot , render the 0th child ,1(location) -> 2(value)
means clicking the second data plot, render the 1st Child (Note: It is 0-indexed )null
for a data plot for which you dont want a drill down.{ "plotPosition": Number, "childPosition": Number }
Eg. [{ plotPosition: 1 , childPosition: 0}, { plotPosition: 0, childPosition: 1}]
[ 0 , { plotPosition:0, childPosition: 1 } ]
btnConfig [Object]- Basic configuration without overriding the default button styles
text
: PropTypes.string - Button Textcolor
: PropTypes.stringbackgroundColor
: PropTypes.stringborderColor
: PropTypes.stringfontSize
: PropTypes.stringfontWeight
: PropTypes.stringpadding
: PropTypes.stringfontFamily
: PropTypes.stringbtnStyle [Object] - CSS styles which override the styles in default btnConfig except text
.
To call APIs we will need the chart object. To get the chart object for an React-FC component, pass a callback through the attribute onRender
.
Write the callback:
As a separate function:
var chartRenderCallback = function (chart) {
[Code goes here]
}
Or, as a component class method:
chartRenderCallback (chart) {
[Code goes here]
}
Pass the callback as a prop, to which the chart object will be returned on rendering
<ReactFC {...chartConfigs} onRender={chartRenderCallback} />
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import FusionCharts from 'fusioncharts';
import Charts from 'fusioncharts/fusioncharts.charts';
import ReactFC from 'react-fusioncharts';
import FusionTheme from 'fusioncharts/themes/fusioncharts.theme.fusion';
ReactFC.fcRoot(FusionCharts, Charts, FusionTheme);
const dataSource = /* Data source A given above */;
const chartConfigs = {
type: 'column2d',
width: 600,
height: 400,
dataFormat: 'json',
dataSource: dataSource
};
class Chart extends Component {
// Convert the chart to a 2D Pie chart after 5 secs.
alterChart(chart) {
setTimeout(() => {
chart.chartType('pie2d');
}, 5000);
}
render() {
return (
<div>
<ReactFC {...chartConfigs} onRender={alterChart} />
</div>
);
}
}
ReactDOM.render(<Chart />, document.getElementById('root'));
links to help you get started:
$ git clone https://github.com/fusioncharts/react-fusioncharts-component.git
$ cd react-fusioncharts-component
$ npm i
$ npm start
npm run build
to start the dev server and point your browser at http://localhost:3000/.The FusionCharts React integration component is open-source and distributed under the terms of the MIT/X11 License. However, you will need to download and include FusionCharts library in your page separately, which has a separate license.
FAQs
Simple and Lightweight React component for FusionCharts JavaScript Charting Library
The npm package react-fusioncharts receives a total of 0 weekly downloads. As such, react-fusioncharts popularity was classified as not popular.
We found that react-fusioncharts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.