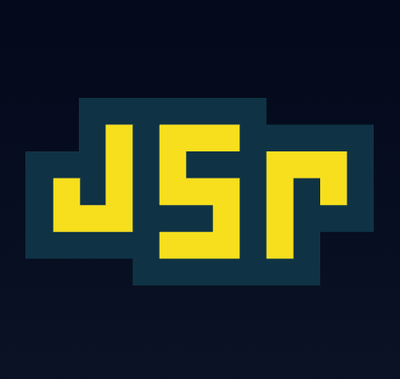
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
react-loadable-ssr-addon
Advanced tools
Server Side Render add-on for React Loadable. Load splitted chunks was never that easy.
Server Side Render add-on for React Loadable. Load splitted chunks was never that easy.
React Loadable SSR Add-on
is a server side render
add-on for React Loadable
that helps you to load dynamically all files dependencies, e.g. splitted chunks
, css
, etc.
Oh yeah, and we also provide support for SRI (Subresource Integrity).
Download our NPM Package
npm install react-loadable-ssr-addon
# or
yarn add react-loadable-ssr-addon
Note: react-loadable-ssr-addon
should not be listed in the devDependencies
.
First we need to import the package into our component;
const ReactLoadableSSRAddon = require('react-loadable-ssr-addon');
module.exports = {
entry: {
// ...
},
output: {
// ...
},
module: {
// ...
},
plugins: [
new ReactLoadableSSRAddon({
filename: 'assets-manifest.json',
}),
],
};
// import `getBundles` to map required modules and its dependencies
import { getBundles } from 'react-loadable-ssr-addon';
// then import the assets manifest file generated by the Webpack Plugin
import manifest from './your-output-folder/assets-manifest.json';
...
// react-loadable ssr implementation
const modules = new Set();
const html = ReactDOMServer.renderToString(
<Loadable.Capture report={moduleName => modules.add(moduleName)}>
<App />
</Loadable.Capture>
);
...
// now we concatenate the loaded `modules` from react-loadable `Loadable.Capture` method
// with our application entry point
const modulesToBeLoaded = [...manifest.entrypoints, ...Array.from(modules)];
// also if you find your project still fetching the files after the placement
// maybe a good idea to switch the order from the implementation above to
// const modulesToBeLoaded = [...Array.from(modules), ...manifest.entrypoints];
// see the issue #6 regarding this thread
// https://github.com/themgoncalves/react-loadable-ssr-addon/issues/6
// after that, we pass the required modules to `getBundles` map it.
// `getBundles` will return all the required assets, group by `file type`.
const bundles = getBundles(manifest, modulesToBeLoaded);
// so it's easy to implement it
const styles = bundles.css || [];
const scripts = bundles.js || [];
res.send(`
<!doctype html>
<html lang="en">
<head>...</head>
${styles.map(style => {
return `<link href="/dist/${style.file}" rel="stylesheet" />`;
}).join('\n')}
<body>
<div id="app">${html}</div>
${scripts.map(script => {
return `<script src="/dist/${script.file}"></script>`
}).join('\n')}
</html>
`);
See how easy to implement it is?
filename
Type: string
Default: react-loadable.json
Assets manifest file name. May contain relative or absolute path.
integrity
Type: boolean
Default: false
Enable or disable generation of Subresource Integrity (SRI). hash.
integrityAlgorithms
Type: array
Default: [ 'sha256', 'sha384', 'sha512' ]
Algorithms to generate hash.
integrityPropertyName
Type: string
Default: integrity
Custom property name to be output in the assets manifest file.
Full configuration example
new ReactLoadableSSRAddon({
filename: 'assets-manifest.json',
integrity: false,
integrityAlgorithms: [ 'sha256', 'sha384', 'sha512' ],
integrityPropertyName: 'integrity',
})
getBundles
import { getBundles } from 'react-loadable-ssr-addon';
/**
* getBundles
* @param {object} manifest - The assets manifest content generate by ReactLoadableSSRAddon
* @param {array} chunks - Chunks list to be loaded
* @returns {array} - Assets list group by file type
*/
const bundles = getBundles(manifest, modules);
const styles = bundles.css || [];
const scripts = bundles.js || [];
const xml = bundles.xml || [];
const json = bundles.json || [];
...
Basic Structure
{
"entrypoints": [ ],
"origins": {
"app": [ ]
},
"assets": {
"app": {
"js": [
{
"file": "",
"hash": "",
"publicPath": "",
"integrity": ""
}
]
}
}
}
entrypoints
Type: array
List of all application entry points defined in Webpack entry
.
origins
Type: array
Origin name requested. List all assets required for the requested origin.
assets
Type: array
of objects
Lists all application assets generate by Webpack, group by file type,
containing an array of objects
with the following format:
[file-type]: [
{
"file": "", // assets file
"hash": "", // file hash generated by Webpack
"publicPath": "", // assets file + webpack public path
"integrity": "" // integrity base64 hash, if enabled
}
]
{
"entrypoints": [
"app"
],
"origins": {
"./home": [
"home"
],
"./about": [
"about"
],
"app": [
"vendors",
"app"
],
"vendors": [
"app",
"vendors"
]
},
"assets": {
"home": {
"js": [
{
"file": "home.chunk.js",
"hash": "fdb00ffa16dfaf9cef0a",
"publicPath": "/dist/home.chunk.js",
"integrity": "sha256-Xxf7WVjPbdkJjgiZt7mvZvYv05+uErTC9RC2yCHF1RM= sha384-9OgouqlzN9KrqXVAcBzVMnlYOPxOYv/zLBOCuYtUAMoFxvmfxffbNIgendV4KXSJ sha512-oUxk3Swi0xIqvIxdWzXQIDRYlXo/V/aBqSYc+iWfsLcBftuIx12arohv852DruxKmlqtJhMv7NZp+5daSaIlnw=="
}
]
},
"about": {
"js": [
{
"file": "about.chunk.js",
"hash": "7e88ef606abbb82d7e82",
"publicPath": "/dist/about.chunk.js",
"integrity": "sha256-ZPrPWVJRjdS4af9F1FzkqTqqSGo1jYyXNyctwTOLk9o= sha384-J1wiEV8N1foqRF7W9SEvg2s/FhQbhpKFHBTNBJR8g1yEMNRMi38y+8XmjDV/Iu7w sha512-b16+PXStO68CP52R+0ZktccMiaI1v0jOy34l/DqyGN7kEae3DpV3xPNoC8vt1WfE1kCAH7dlnHDdp1XRVhZX+g=="
}
]
},
"app": {
"css": [
{
"file": "app.css",
"hash": "5888714915d8e89a8580",
"publicPath": "/dist/app.css",
"integrity": "sha256-3y4DyCC2cLII5sc2kaElHWhBIVMHdan/tA0akReI9qg= sha384-vCMVPKjSrrNpfnhmCD9E8SyHdfPdnM3DO/EkrbNI2vd0m2wH6BnfPja6gt43nDIF"
}
],
"js": [
{
"file": "app.bundle.js",
"hash": "0cbd05b10204597c781d",
"publicPath": "/dist/app.bundle.js",
"integrity": "sha256-sGdw+WVvXK1ZVQnYHI4FpecOcZtWZ99576OHCdrGil8= sha384-DZZzkPtPCTCR5UOWuGCyXQvsjyvZPoreCzqQGyrNV8+HyV9MdoYZawHX7NdGGLyi sha512-y29BlwBuwKB+BeXrrQYEBrK+mfWuOb4ok6F57kGbtrwa/Xq553Zb7lgss8RNvFjBSaMUdvXiJuhmP3HZA0jNeg=="
}
]
},
"vendors": {
"css": [
{
"file": "vendors.css",
"hash": "5a9586c29103a034feb5",
"publicPath": "/dist/vendors.css"
}
],
"js": [
{
"file": "vendors.chunk.js",
"hash": "5a9586c29103a034feb5",
"publicPath": "/dist/vendors.chunk.js"
}
]
}
}
}
Marcos Gonçalves – LinkedIn – Website
Distributed under the MIT license. Click here for more information.
https://github.com/themgoncalves/react-loadable-ssr-addon
git checkout -b feature/fooBar
)git commit -m ':zap: Add some fooBar'
)git push origin feature/fooBar
)⚡️ New feature (:zap:
)
🐛 Bug fix (:bug:
)
🔥 P0 fix (:fire:
)
✅ Tests (:white_check_mark:
)
🚀 Performance improvements (:rocket:
)
🖍 CSS / Styling (:crayon:
)
♿ Accessibility (:wheelchair:
)
🌐 Internationalization (:globe_with_meridians:
)
📖 Documentation (:book:
)
🏗 Infrastructure / Tooling / Builds / CI (:building_construction:
)
⏪ Reverting a previous change (:rewind:
)
♻️ Refactoring (like moving around code w/o any changes) (:recycle:
)
🚮 Deleting code (:put_litter_in_its_place:
)
FAQs
Server Side Render add-on for React Loadable. Load splitted chunks was never that easy.
The npm package react-loadable-ssr-addon receives a total of 1,883 weekly downloads. As such, react-loadable-ssr-addon popularity was classified as popular.
We found that react-loadable-ssr-addon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.