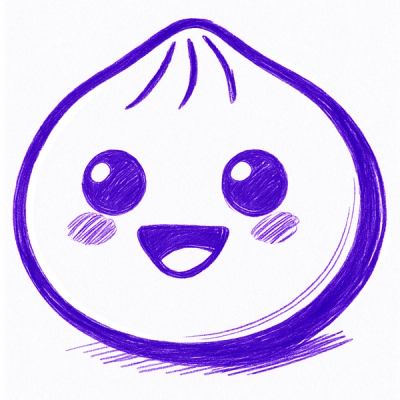
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
react-mkx-storage
Advanced tools
This package provides custom React hooks for managing state in local storage, session storage and cookie storage: useLocalStorage, useSessionStorage and useCookieStorage.
This package provides three custom React hooks for managing state in local storage, session storage and cookie storage: useLocalStorage
, useSessionStorage
and useCookieStorage
.
You can install the package using npm:
npm install react-mkx-storage
Or using yarn:
yarn add react-mkx-storage
useLocalStorage
A custom React hook to manage state in local storage.
key
(string): The key under which to store the value in local storage.initialValue
(string | number | object | array): The initial value to use if no value is found in local storage.import { useLocalStorage } from 'react-mkx-storage';
const MyComponent = () => {
const [value, setValue] = useLocalStorage('key', 'initialValue');
// Use value and setValue as needed
return (
// Your component JSX
);
};
useSessionStorage
A custom React hook to manage state in session storage.
key
(string): The key under which to store the value in session storage.initialValue
(string | number | object | array): The initial value to use if no value is found in session storage.import { useSessionStorage } from 'react-mkx-storage';
const MyComponent = () => {
const [value, setValue] = useSessionStorage('key', 'initialValue');
// Use value and setValue as needed
return (
// Your component JSX
);
};
useCookieStorage
A custom React hook to manage state in Cookie storage.
key
(string): The key under which to store the value in Cookie storage.initialValue
(string | number | object | array): The initial value to use if no value is found in Cookie storage.import { useCookieStorage } from 'react-mkx-storage';
const MyComponent = () => {
const [value, setValue] = useCookieStorage('key', 'initialValue');
// Use value and setValue as needed
return (
// Your component JSX
);
};
![]() | ![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|---|
Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | 11 ✔ |
This project is licensed under the ISC License - see the LICENSE file for details.
FAQs
This package provides custom React hooks for managing state in local storage, session storage and cookie storage: useLocalStorage, useSessionStorage and useCookieStorage.
The npm package react-mkx-storage receives a total of 26 weekly downloads. As such, react-mkx-storage popularity was classified as not popular.
We found that react-mkx-storage demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.