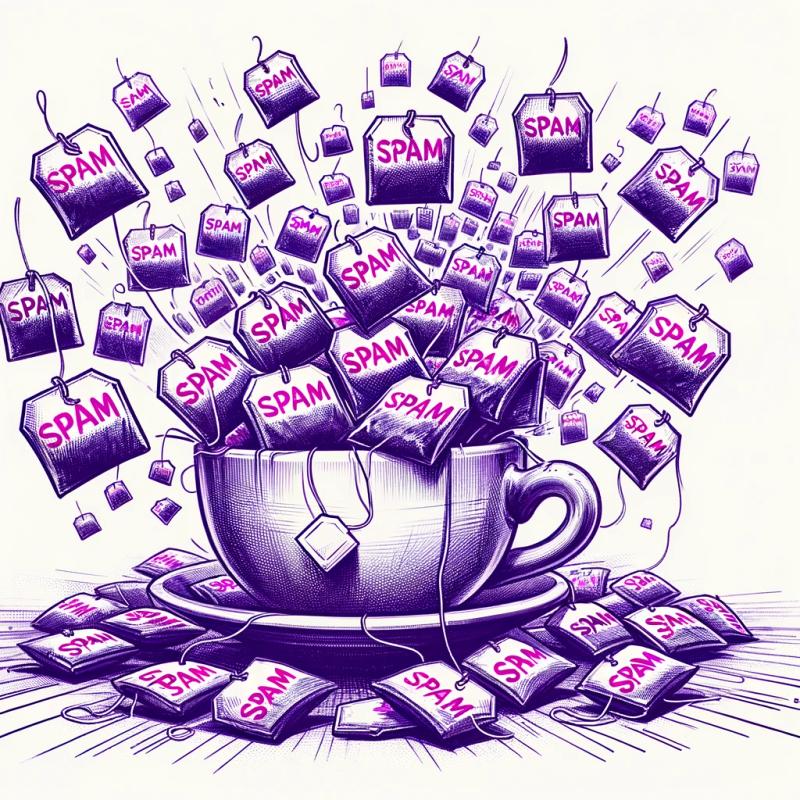
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-native-animated-dots-carousel
Advanced tools
Readme
Package to configure you dots pagination carousel just like Instagram does. With this package you could make whatever configuration you might need on your projects.
Installation can be done through npm
or yarn
:
npm i react-native-animated-dots-carousel --save
yarn add react-native-animated-dots-carousel
import * as React from 'react';
import { StyleSheet, View, Text, TouchableOpacity } from 'react-native';
import AnimatedDotsCarousel from 'react-native-animated-dots-carousel';
const LENGTH = 10;
export default function App() {
const [index, setIndex] = React.useState<number>(0);
const increaseIndex = () => {
setIndex(Math.min(index + 1, LENGTH - 1));
};
const decreaseIndex = () => {
setIndex(Math.max(index - 1, 0));
};
return (
<View style={styles.container}>
<TouchableOpacity
style={{ borderWidth: 1, marginTop: 20, backgroundColor: 'white' }}
onPress={increaseIndex}
>
<Text>Increase</Text>
</TouchableOpacity>
<TouchableOpacity
style={{ borderWidth: 1, marginTop: 20, backgroundColor: 'white' }}
onPress={decreaseIndex}
>
<Text>Decrease</Text>
</TouchableOpacity>
<AnimatedDotsCarousel
length={LENGTH}
currentIndex={index}
maxIndicators={4}
interpolateOpacityAndColor={true}
activeIndicatorConfig={{
color: 'red',
margin: 3,
opacity: 1,
size: 8,
}}
inactiveIndicatorConfig={{
color: 'white',
margin: 3,
opacity: 0.5,
size: 8,
}}
decreasingDots={[
{
config: { color: 'white', margin: 3, opacity: 0.5, size: 6 },
quantity: 1,
},
{
config: { color: 'white', margin: 3, opacity: 0.5, size: 4 },
quantity: 1,
},
]}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: 'black',
},
});
export interface DotConfig {
size: number;
opacity: number;
color: string;
margin: number;
borderWidth?: number;
borderColor?: string;
}
export interface DecreasingDot {
quantity: number;
config: DotConfig;
}
Prop | Type | Required(Default Value) | Description |
---|---|---|---|
length | number | required | Length of the list you want to associate with the carousel dots |
currentIndex | number | required | Current index of the list. |
maxIndicators | number | required | This number represents how many indicators you want to show, without decreasing size. Counting inactive indicators and the active indicator |
activeIndicatorConfig | DotConfig | required | This is an object with the configuration of the active indicator |
inactiveIndicatorConfig | DotConfig | required | This is an object with the configuration of the inactive indicator |
decreasingDots | DecreasingDot[] | required | This is a list where you have to define the quantiy per element and the dot config. The quantity represents how many dots with this config you want per side (simetrically). The size of this elements should be decreasing size if you want this to look nice. |
verticalOrientation | boolean | false | If you want this oriented vertically or horizontally. Default is horizontally |
interpolateOpacityAndColor | boolean | true | Default is true. With this setted to true you will be able to see an animation everytime the activeDot change. |
See the open issues for a list of proposed features (and known issues).
Contributions are what make the open source community such an amazing place to be learn, inspire, and create. Any contributions you make are greatly appreciated.
git checkout -b feature/AmazingFeature
)git commit -m 'Add some AmazingFeature'
)git push origin feature/AmazingFeature
)Distributed under the MIT License. See LICENSE
for more information.
Project Link: https://github.com/felire/react-native-animated-dots-carousel
FAQs
Package to configure you dots pagination carousel just like Instagram does
The npm package react-native-animated-dots-carousel receives a total of 6,107 weekly downloads. As such, react-native-animated-dots-carousel popularity was classified as popular.
We found that react-native-animated-dots-carousel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.