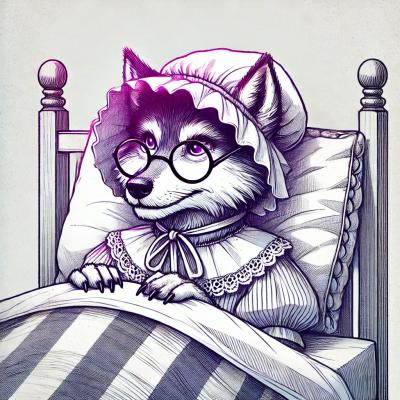
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
react-native-international-phone-number-test
Advanced tools
International mobile phone input component with mask for React Native
$ npm i --save react-native-international-phone-number
OR
$ yarn add react-native-international-phone-number
AND
Update your
metro.config.js
file:
module.exports = { ... resolver: { sourceExts: ['jsx', 'js', 'ts', 'tsx', 'cjs', 'json'], }, };
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
phoneMask,
PhoneInput,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState(undefined);
const [phoneInput, setPhoneInput] = useState('');
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
selectedCountry={selectedCountry}
setSelectedCountry={setSelectedCountry}
value={phoneInput}
onChangeText={(newValue) =>
setPhoneInput(
phoneMask(newValue, selectedCountry.callingCode[0])
)
}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone: {`${selectedCountry?.callingCode[0]} ${phoneInput}`}
</Text>
</View>
</View>
);
}
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
phoneMask,
PhoneInput,
} from 'react-native-international-phone-number';
import { Country } from 'react-native-country-picker-modal';
import { Controller, FieldValues, useForm } from 'react-hook-form';
interface FormProps extends FieldValues {
phoneNumber: string;
}
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | Country
>(undefined);
const { control, handleSubmit, resetField } = useForm();
function onSubmit(form: FormProps) {
Alert.alert(
'Advanced Result',
`${selectedCountry?.callingCode[0]} ${form.phoneNumber}`
);
resetField('phoneNumber');
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<Controller
name="phoneNumber"
control={control}
render={({ field: { onChange, value } }) => (
<PhoneInput
selectedCountry={selectedCountry}
setSelectedCountry={setSelectedCountry}
value={value}
onChangeText={(newValue) =>
onChange(
phoneMask(newValue, selectedCountry.callingCode[0])
)
}
/>
)}
/>
<Button
style={{
width: '100%',
marginTop: 10,
backgroundColor: '#90d7ff',
}}
onPress={handleSubmit(onSubmit)}
/>
<Text>Submit</Text>
</Button>
</View>
);
}
value?
: stringonChangeText?
: (text: string) => voidcontainerStyle?
: StylePropinputStyle?
: StylePropwithDarkTheme?
: booleandisabled?
: booleanplaceholder?
: stringplaceholderTextColor?
: stringphoneMask
: (value: string, countryCode: string) => string $ git clone https://github.com/AstrOOnauta/react-native-international-phone-number.git
Repair, Update and Enjoy 🛠️🚧⚙️
Create a new PR to this repository
FAQs
International mobile phone input component with mask for React Native
We found that react-native-international-phone-number-test demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.