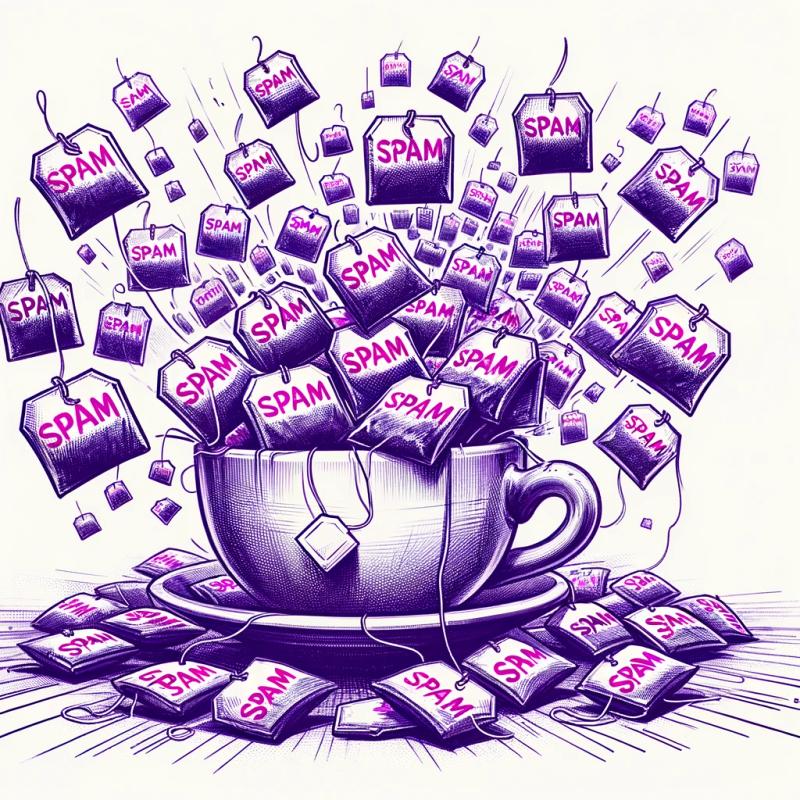
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-native-ssl-public-key-pinning
Advanced tools
Readme
Simple and secure SSL public key pinning for React Native. Uses OkHttp CertificatePinner on Android and TrustKit on iOS.
npm install react-native-ssl-public-key-pinning
OR for Yarn use:
yarn add react-native-ssl-public-key-pinning
Before building for iOS, make sure to run the following commands:
cd ios && pod install && cd ..
npx expo install react-native-ssl-public-key-pinning
and then follow the steps to create a development build or production build
expo-dev-client
Network Inspector on iOS (Optional)If you are building an iOS Expo development build and want to test out your pinning configuration, you will need to disable expo-dev-client
's network inspector as it interferes with the pinning setup. Note that the network inspector is automatically disabled on production builds and so this library would function properly on production builds without needing to go through the following steps.
expo-build-properties
npx expo install expo-build-properties
app.json
{
"expo": {
"plugins": [
[
"expo-build-properties",
{
"ios": {
"networkInspector": false
}
}
]
]
}
}
npx expo prebuild
initializeSslPinning
as early as possible in your App entry point with your SSL pinning configuration.import { initializeSslPinning } from 'react-native-ssl-public-key-pinning';
await initializeSslPinning({
'google.com': {
includeSubdomains: true,
publicKeyHashes: [
'CLOmM1/OXvSPjw5UOYbAf9GKOxImEp9hhku9W90fHMk=',
'hxqRlPTu1bMS/0DITB1SSu0vd4u/8l8TjPgfaAp63Gc=',
'Vfd95BwDeSQo+NUYxVEEIlvkOlWY2SalKK1lPhzOx78=',
'QXnt2YHvdHR3tJYmQIr0Paosp6t/nggsEGD4QJZ3Q0g=',
'mEflZT5enoR1FuXLgYYGqnVEoZvmf9c2bVBpiOjYQ0c=',
],
},
});
// ...
// This request will have public key pinning enabled
const response = await fetch('https://www.google.com');
import { addSslPinningErrorListener } from 'react-native-ssl-public-key-pinning';
useEffect(() => {
const subscription = addSslPinningErrorListener((error) => {
// Triggered when an SSL pinning error occurs due to pin mismatch
console.log(error.serverHostname);
});
return () => {
subscription.remove();
};
}, []);
API | Description |
---|---|
isSslPinningAvailable(): boolean | Returns whether the SslPublicKeyPinning NativeModule is available on the current app installation. Useful if you're using Expo Go and want to avoid initializing pinning if it's not available. |
initializeSslPinning(options: PinningOptions): Promise<void> | Initializes and enables SSL public key pinning for the domains and options you specify. |
disableSslPinning(): Promise<void> | Disables SSL public key pinning. |
addSslPinningErrorListener(callback: ErrorListenerCallback): EmitterSubscription | Subscribes to SSL pinning errors due to pin mismatch. Useful if you would like to report errors or inform the user of security issues. |
Option | Type | Mandatory | Description |
---|---|---|---|
includeSubdomains | boolean | No | Whether all subdomains of the specified domain should also be pinned. Defaults to false . |
publicKeyHashes | string[] | Yes | An array of SSL pins, where each pin is the base64-encoded SHA-256 hash of a certificate's Subject Public Key Info. Note that at least two pins are needed per domain on iOS. |
expirationDate | string | No | A string containing the date, in yyyy-MM-dd format, on which the domain’s configured SSL pins expire, thus disabling pinning validation. If this is not set, then the pins do not expire. Expiration helps prevent connectivity issues in Apps which do not get updates to their pin set, such as when the user disables App updates. |
includeSubdomains: true
is not supported and you have to match your domains exactly. This is because wildcard domain support (which this library uses for includeSubdomains
) was only introduced in OkHttp v4.3, and RN v65 is the first version that upgraded OkHttp to v4.fetch
or XMLHttpRequest
would also be affected by the pinning (e.g. axios
). However, native libraries that implement their own methods of performing network requests would not be affected by the pinning configuration.initializeSslPinning
to throw an exception if only one pin is provided.react-native-code-push
or expo-updates
. Doing this will help ensure your key hashes are up to date without needing users to download a new version from the Play Store/App Store since all pinning configurations are done through the JS API.Run the following command, replacing <hostname>
with your server's hostname.
echo | openssl s_client -servername <hostname> -connect <hostname>:443 2>/dev/null | openssl x509 -pubkey -noout | openssl pkey -pubin -outform DER | openssl dgst -sha256 -binary | openssl enc -base64
openssl x509 -in certificate.crt -pubkey -noout | openssl pkey -pubin -outform der | openssl dgst -sha256 -binary | openssl enc -base64
openssl rsa -in private.key -pubout -out public_key.pem
openssl rsa -in public_key.pem -pubin -outform DER | openssl dgst -sha256 -binary | openssl enc -base64
If your site is accessible publicly, you can use https://www.ssllabs.com/ssltest/index.html to retrieve the public key hash of your certificates.
An easy way to test you've set everything up correctly is by temporarily providing the wrong public key hashes to initializeSslPinning
. For example:
await initializeSslPinning({
'google.com': {
includeSubdomains: true,
publicKeyHashes: [
'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA=',
'BBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBB=',
],
},
});
// ...
// This request should fail with an error
const response = await fetch('https://www.google.com');
Any requests you make to the pinned domain should fail since the server is not providing certificates that match your hashes. You can then switch back to the correct public key hashes while leaving everything else the same, and once you ensure the requests succeed again you'll know you've set it all up correctly!
See the contributing guide to learn how to contribute to the repository and the development workflow.
FAQs
Simple and secure SSL public key pinning for React Native. No native configuration needed, set up in <5 minutes.
The npm package react-native-ssl-public-key-pinning receives a total of 6,253 weekly downloads. As such, react-native-ssl-public-key-pinning popularity was classified as popular.
We found that react-native-ssl-public-key-pinning demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.