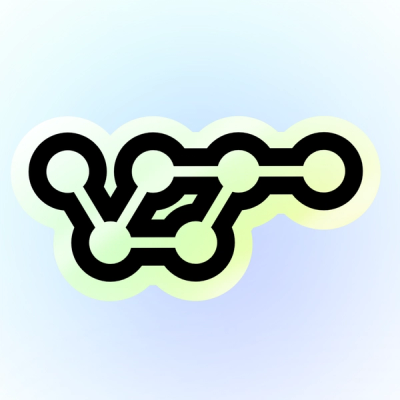
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
react-responsive
Advanced tools
The react-responsive package is a utility for managing media queries in React applications. It allows developers to create responsive components that adapt to different screen sizes and orientations.
Media Query Component
This feature allows you to use media queries directly within your React components. The useMediaQuery hook can be used to conditionally render content based on the screen size, orientation, and resolution.
import { useMediaQuery } from 'react-responsive';
const Example = () => {
const isDesktopOrLaptop = useMediaQuery({ minWidth: 1224 });
const isBigScreen = useMediaQuery({ minWidth: 1824 });
const isTabletOrMobile = useMediaQuery({ maxWidth: 1224 });
const isPortrait = useMediaQuery({ orientation: 'portrait' });
const isRetina = useMediaQuery({ minResolution: '2dppx' });
return (
<div>
{isDesktopOrLaptop && <p>You are a desktop or laptop</p>}
{isBigScreen && <p>You have a huge screen</p>}
{isTabletOrMobile && <p>You are a tablet or mobile phone</p>}
{isPortrait && <p>You are in portrait orientation</p>}
{isRetina && <p>You are retina</p>}
</div>
);
};
Media Query Component with Children
This feature allows you to use the MediaQuery component to wrap children elements that should only be rendered if the media query matches. This is useful for conditionally rendering parts of your component tree based on media queries.
import { MediaQuery } from 'react-responsive';
const Example = () => (
<div>
<MediaQuery minWidth={1224}>
<p>You are a desktop or laptop</p>
</MediaQuery>
<MediaQuery minWidth={1824}>
<p>You have a huge screen</p>
</MediaQuery>
<MediaQuery maxWidth={1224}>
<p>You are a tablet or mobile phone</p>
</MediaQuery>
<MediaQuery orientation="portrait">
<p>You are in portrait orientation</p>
</MediaQuery>
<MediaQuery minResolution="2dppx">
<p>You are retina</p>
</MediaQuery>
</div>
);
react-media is a similar package that provides a declarative way to use media queries in React. It offers a Media component that can be used to conditionally render content based on media queries. Compared to react-responsive, react-media is more focused on providing a simple and declarative API for media queries.
react-responsive-mixin is a mixin for React components that allows them to respond to media query changes. It is less commonly used in modern React applications due to the shift away from mixins in favor of hooks and higher-order components. However, it can still be useful for legacy codebases that rely on mixins.
react-socks is another package for handling media queries in React. It provides a set of components and hooks for responsive design. Compared to react-responsive, react-socks offers a more modern API with hooks and context, making it a good alternative for developers looking for a more contemporary solution.
Package | react-responsive |
Description | Media queries in react for responsive design |
Browser Version | >= IE6* |
The best supported, easiest to use react media query module.
This module is pretty straightforward: You specify a set of requirements, and the children will be rendered if they are met. Also handles changes so if you resize or flip or whatever it all just works.
A MediaQuery element functions like any other React component, which means you can nest them and do all the normal jazz.
var MediaQuery = require('react-responsive');
var A = React.createClass({
render: function(){
return (
<div>
<div>Device Test!</div>
<MediaQuery query='(min-device-width: 1224px)'>
<div>You are a desktop or laptop</div>
<MediaQuery query='(min-device-width: 1824px)'>
<div>You also have a huge screen</div>
</MediaQuery>
<MediaQuery query='(max-width: 1224px)'>
<div>You are sized like a tablet or mobile phone though</div>
</MediaQuery>
</MediaQuery>
<MediaQuery query='(max-device-width: 1224px)'>
<div>You are a tablet or mobile phone</div>
</MediaQuery>
<MediaQuery query='(orientation: portrait)'>
<div>You are portrait</div>
</MediaQuery>
<MediaQuery query='(orientation: landscape)'>
<div>You are landscape</div>
</MediaQuery>
<MediaQuery query='(min-resolution: 2dppx)'>
<div>You are retina</div>
</MediaQuery>
</div>
);
}
});
To make things more idiomatic to react, you can use camelcased shorthands to construct media queries.
For a list of all possible shorthands and value types see https://github.com/wearefractal/react-responsive/blob/master/src/mediaQuery.js#L9
Any numbers given as a shorthand will be expanded to px (1234
will become '1234px'
)
var MediaQuery = require('react-responsive');
var A = React.createClass({
render: function(){
return (
<div>
<div>Device Test!</div>
<MediaQuery minDeviceWidth={1224}>
<div>You are a desktop or laptop</div>
<MediaQuery minDeviceWidth={1824}>
<div>You also have a huge screen</div>
</MediaQuery>
<MediaQuery maxWidth={1224}>
<div>You are sized like a tablet or mobile phone though</div>
</MediaQuery>
</MediaQuery>
<MediaQuery maxDeviceWidth={1224}>
<div>You are a tablet or mobile phone</div>
</MediaQuery>
<MediaQuery orientation='portrait'>
<div>You are portrait</div>
</MediaQuery>
<MediaQuery orientation='landscape'>
<div>You are landscape</div>
</MediaQuery>
<MediaQuery minResolution='2dppx'>
<div>You are retina</div>
</MediaQuery>
</div>
);
}
});
You may also specify a function for the child of the MediaQuery component. When the component renders, it is passed whether or not the given media query matches. This function must return a single element or null
.
<MediaQuery minDeviceWidth={700}>
{(matches) => {
if (matches) {
return <div>Media query matches!</div>;
} else {
return <div>Media query does not match!</div>;
}
}}
</MediaQuery>
You may specify an optional component
property on the MediaQuery
that indicates what component to wrap children with. Any additional props defined on the MediaQuery
will be passed through to this "wrapper" component. If the component
property is not defined and the MediaQuery
has more than 1 child, a div
will be used as the "wrapper" component by default. However, if the component
prop is not defined and there is only 1 child, that child will be rendered alone without a component wrapping it.
Specifying Wrapper Component
<MediaQuery minDeviceWidth={700} component="ul">
<li>Item 1</li>
<li>Item 2</li>
</MediaQuery>
// renders the following when the media query condition is met
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
Unwrapped Component
<MediaQuery minDeviceWidth={700}>
<div>Unwrapped component</div>
</MediaQuery>
// renders the following when the media query condition is met
<div>Unwrapped component</div>
Default div Wrapper Component
<MediaQuery minDeviceWidth={1200} className="some-class">
<div>Wrapped</div>
<div>Content</div>
</MediaQuery>
// renders the following when the media query condition is met
<div className="some-class">
<div>Wrapped</div>
<div>Content</div>
</div>
Server rendering can be done by passing static values through the values
property.
The values property can contain orientation
, scan
, aspectRatio
, deviceAspectRatio
,
height
, deviceHeight
, width
, deviceWidth
, color
, colorIndex
, monochrome
,
resolution
and type
to be matched against the media query.
type
can be one of: all
, grid
, aural
, braille
, handheld
, print
, projection
,
screen
, tty
, tv
or embossed
.
var MediaQuery = require('react-responsive');
var A = React.createClass({
render: function(){
return (
<div>
<div>Device Test!</div>
<MediaQuery minDeviceWidth={1224} values={{deviceWidth: 1600}}>
<div>You are a desktop or laptop</div>
<MediaQuery minDeviceWidth={1824}>
<div>You also have a huge screen</div>
</MediaQuery>
<MediaQuery maxWidth={1224}>
<div>You are sized like a tablet or mobile phone though</div>
</MediaQuery>
</MediaQuery>
<MediaQuery maxDeviceWidth={1224}>
<div>You are a tablet or mobile phone</div>
</MediaQuery>
<MediaQuery orientation='portrait'>
<div>You are portrait</div>
</MediaQuery>
<MediaQuery orientation='landscape'>
<div>You are landscape</div>
</MediaQuery>
<MediaQuery minResolution='2dppx'>
<div>You are retina</div>
</MediaQuery>
</div>
);
}
});
Chrome | 9 |
Firefox (Gecko) | 6 |
MS Edge | All |
Internet Explorer | 10 |
Opera | 12.1 |
Safari | 5.1 |
Pretty much everything. Check out these polyfills:
FAQs
Media queries in react for responsive design
We found that react-responsive demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.