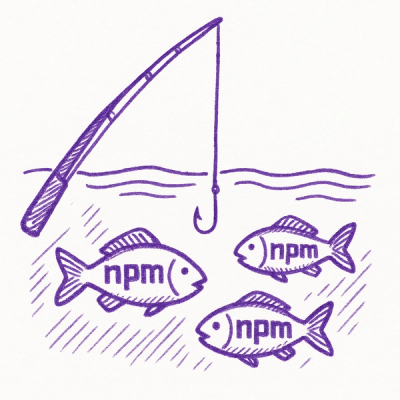
Security News
/Research
npm Phishing Email Targets Developers with Typosquatted Domain
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
react-socks
Advanced tools
Wrap your components with React Socks to prevent unnecessary render in different viewports.
<Breakpoint small down>
<MyAwesomeMobileMenu>
This component will render only in mobile devices
</MyAwesomeMobileMenu>
</Breakpoint>
Conventionally we have been writing css media queries for different viewports to hide and show elements that are always present in the DOM. With React taking over the world, everything is about rendering components into the DOM. React Socks helps you conditionally render elements based on viewports.
Render viewport specific components without hassle
You can define your own breakpoints (Eg. xs, ipad, bigmonitors) and use them
You can improve your app performance if you lazy load your viewport specific components
Simpler and sweeter syntax for ease of use
$ npm install --save react-socks
Just wrap your top level component with BreakpointProvider
and use the Breakpoint
component anywhere you need.
Note: BreakpointProvider
was introduced only in v1.0.0
. It's not available in previous alpha versions.
import { Breakpoint, BreakpointProvider } from 'react-socks';
// entry file (usually App.js or index.js)
const App = () => (
<BreakpointProvider>
<Example />
</BreakpointProvider>
);
// Example.js
const Example = () => {
return (
<div>
<Breakpoint small down>
<div>I will render only in mobile devices</div>
</Breakpoint>
<Breakpoint medium only>
<div>I will render only in tablets (iPad, etc...)</div>
</Breakpoint>
<Breakpoint medium down>
<div>I will render in tablets (iPad, etc...) and everything below (mobile devices)</div>
</Breakpoint>
<Breakpoint medium up>
<div>I will render in tablets (iPad, etc...) and everything above (laptops, desktops)</div>
</Breakpoint>
<Breakpoint large up>
<div>I will render in laptops, desktops and everything above</div>
</Breakpoint>
{/* Add breakpoints on the fly using custom queries */}
<Breakpoint customQuery="(min-width: 500px)">
<div style={{backgroundColor: 'red' }}>
Custom breakpoint: (min-width : 500px)
</div>
</Breakpoint>
<Breakpoint customQuery="(max-width: 1000px)">
<div style={{backgroundColor: 'yellow' }}>
Custom breakpoint: (max-width : 1000px)
</div>
</Breakpoint>
<Breakpoint customQuery="(min-width: 500px) and (max-width: 700px)">
<div style={{backgroundColor: 'lightblue' }}>
Custom breakpoint: (min-width : 500px) && (max-width : 700px)
</div>
</Breakpoint>
</div>
);
};
You can define your own breakpoints.
setDefaultBreakpoints
once in your App.js
or your React entry file.Note: You only need to set default breakpoints once in your app
import { setDefaultBreakpoints } from 'react-socks';
setDefaultBreakpoints([
{ xs: 0 },
{ s: 376 },
{ m: 426 },
{ l: 769 },
{ xl: 1025 }
]);
<Breakpoint m only>
I will render only in m devices
</Breakpoint>
setDefaultBreakpoints([
{ cats: 0 },
{ dinosaurs: 900 }
]);
<Breakpoint cats only>
Only cats can render me
</Breakpoint>
setDefaultBreakpoints([
{ xsmall: 0 }, // all mobile devices
{ small: 576 }, // mobile devices (not sure which one's this big)
{ medium: 768 }, // ipad, ipad pro, ipad mini, etc
{ large: 992 }, // smaller laptops
{ xlarge: 1200 } // laptops and desktops
]);
Import the Breakpoint
component anywhere in the your code and start using it with your breakpoint and modifier props.
// small is breakpoint
// down is modifier
<Breakpoint small down>
<MyAwesomeMobileMenu>
This component will render only in mobile devices
</MyAwesomeMobileMenu>
</Breakpoint>
You have three modifiers
only - will render the component only in the specified breakpoint.
up - will render the component in the specified breakpoint and all the breakpoints above it (greater than the width).
down - will render the component in the specified breakpoint and all the breakpoints below it (less than the width).
Now, you can add a breakpoint of any width by using this prop: customQuery
.
Simply write your media query as a string and pass it to customQuery
<Breakpoint customQuery="(min-width: 500px)">
<div style={{backgroundColor: 'red' }}>
Custom breakpoint: (min-width : 500px)
</div>
</Breakpoint>
<Breakpoint customQuery="(max-width: 1000px)">
<div style={{backgroundColor: 'yellow' }}>
Custom breakpoint: (max-width : 1000px)
</div>
</Breakpoint>
<Breakpoint customQuery="(min-width: 500px) and (max-width: 700px)">
<div style={{backgroundColor: 'lightblue' }}>
Custom breakpoint: (min-width : 500px) && (max-width : 700px)
</div>
</Breakpoint>
Note: customQuery
will be ignored if you have mentioned any modifiers like up
, down
& only
Use customQuery
only if you want to make use of arbitary breakpoints.
If you call useCurrentWidth
in the render function, you can access the current width directly:
import { useCurrentWidth } from 'react-socks'
const CustomComponent = () => {
const width = useCurrentWidth()
if (width < 500) {
return <h1>Hello!</h1>
} else {
return <h2>Hello!</h2>
}
}
You can also use the current breakpoint name with useCurrentBreakpointName
:
import { useCurrentBreakpointName } from 'react-socks'
const CustomComponent = () => {
const breakpoint = useCurrentBreakpointName()
if (breakpoint == 'small') {
return <h1>Hello!</h1>
} else {
return <h2>Hello!</h2>
}
}
Thanks goes to these amazing people 🎉
Dinesh Pandiyan | Capelo | Adarsh | Patryk | WRNGFRNK | Farhad Yasir |
---|---|---|---|---|---|
Entkenntnis | Douglas Moore | Abdul rehman | Nawaz Khan |
MIT © Dinesh Pandiyan
2.2.0
FAQs
React library to render components only on specific viewports
The npm package react-socks receives a total of 4,482 weekly downloads. As such, react-socks popularity was classified as popular.
We found that react-socks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.