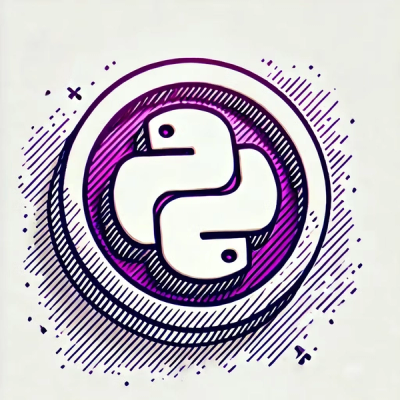
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
react-solana-nftmint
Advanced tools
Mint NFTs on Solana With this package, all you need to mint NFTs is to provide your JSON file as an URL to the mint function.
The props should be self-explanatory, but they are:
yarn add react-solana-nftmint
or npm i -S react-solana-nftmint
import * as anchor from "@project-serum/anchor";
import { useAnchorWallet } from "@solana/wallet-adapter-react";
import { LAMPORTS_PER_SOL, PublicKey } from "@solana/web3.js";
import React, { useCallback } from "react";
import useMint, { MintProps } from "react-solana-nftmint";
export default function MyComponent() {
const anchorWallet = useAnchorWallet()
const props: MintProps = {
rpc: "https://api.devnet.solana.com",
title: "Sample NFT",
creators: [
{
address: new PublicKey("6xnRdTedrerREnaveYndZPioRuK1JcQPfnyA5mQME6vT"),
verified: false,
share: 100 // sums must total 100 if you have more than one creator
},
],
mintPrice: new anchor.BN(0.05 * LAMPORTS_PER_SOL),
symbol: "",
seller: new PublicKey("6xnRdTedrerREnaveYndZPioRuK1JcQPfnyA5mQME6vT"),
royalty: 500,
}
const { mintNft, ready, uploading, error, mintSuccess } = useMint(props, anchorWallet);
const handleMint = useCallback(async () => {
if (!anchorWallet) {
return
}
let nftURL = "https://files.sdrive.app/15zg0r4.json";
await mintNft(nftURL);
}, [props, anchorWallet])
return (
<div>
{error && <div>{error}</div>}
{uploading && <div>NFT is cooking...</div>}
{mintSuccess && <div>Minted!</div>}
<button disabled={!ready} onClick={handleMint}>Mint {props.title}</button>
</div>
)
};
FAQs
UseEffect hook to mint NFT 1/1s
The npm package react-solana-nftmint receives a total of 0 weekly downloads. As such, react-solana-nftmint popularity was classified as not popular.
We found that react-solana-nftmint demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.