react-tabs-scrollable
a simple react scrollable tabs with a lot of additional features and with fully supporting of RTL mode

Install
npm install --save react-tabs-scrollable@latest
yarn add react-tabs-scrollable@latest
npm install --save react-tabs-scrollable@1.0.10
yarn add react-tabs-scrollable@1.0.10
Demos
V1 docs
V1 docs
Features
- Typescript out of box
- Many modes to control the behaviour of the selected tab.
- Easy to start with it takes you less than minute to start it up!
- Many features and so easy to customize
- Fully support for RTL (actually the reason why I built this component is because RTL)
- Fully accessible
- You can control and have access to everything inside it.
- Small sized 9.6k (gzipped: 3.9k)
- Great to use in navigation, menus and lists or any proper use for tabs
- Easy to style, you have the css file so you can edit it as you would like, and there are style and className props to all the elements used inside the package.
- You have access to all refs.
- And much more ..
What's new in V2?
-
I rebuild the package from scratch with typescript to avoid the unnecessary bugs and to make it more elegant and easy to use with the typescript auto-complete.
Note: this's my first time using typescript so expect many bugs with types since i was interfering a lot of types to any, and because I dont have the proper internet, I couldnt search well for them -_-.
But overall I think everything works fine!.
-
I deleted the unnecessary code and made it more readable and clean.
-
I deleted selectedTabCoordinates
and replaced it with getTabsBoundingClientRects
function that returns DOMRect object for the tabsContainer
and tab
, and it's way performant comparing to the old selectedTabCoordinates
, it just runs when the scroll stops and when you switch to RTL.
-
I renamed the two action onRightBtnClick
and onLeftBtnClick
to onRightNavBtnClick
and onLeftNavBtnClick
-
I made the API and the enternals of the component more exposed to the developers who wants to use it (Please see the API table below to see all the new props), since I added about 15 new props including refs to all the elements inside the pacakge, and I added custom styles to style it as you want.
-
I added new features to make the component more compatible with my new package react-kfc-menu
such as mode
prop that controls the behavior of the selected tab scroll, now you can change the whole behavior of it with the new 4 modes I've added to it.
-
I added <TabScreen />
component if you want to use tab panels with the tabs.
I'm planning to add Swipeable component to make the TabScreens more interactive with drag and touch events on both, Desktop and mobile.
Please If you faced any bugs feel free to open an issue.
Usage
#Simple Tabs
import React from "react";
import { Tabs, Tab } from "react-tabs-scrollable";
import "react-tabs-scrollable/dist/rts.css";
const SimpleTabs = () => {
const [activeTab, setActiveTab] = React.useState(1);
const onTabClick = (e, index) => {
console.log(e);
setActiveTab(index);
};
return (
<>
<Tabs activeTab={activeTab} onTabClick={onTabClick}>
{/* generating an array to loop through it */}
{[...Array(20).keys()].map((item) => (
<Tab key={item}>Tab {item}</Tab>
))}
</Tabs>
</>
);
};
export default SimpleTabs;
#Tabs with TabScreen
import React from "react";
import { Tabs, Tab } from "react-tabs-scrollable";
import "react-tabs-scrollable/dist/rts.css";
const SimpleTabs = () => {
const [activeTab, setActiveTab] = React.useState(1);
const onTabClick = (e, index) => {
console.log(e);
setActiveTab(index);
};
const tabsArray = [...Array(20).keys()];
return (
<>
<Tabs activeTab={activeTab} onTabClick={onTabClick}>
{/* generating an array to loop through it */}
{tabsArray.map((item) => (
<Tab key={item}>Tab {item}</Tab>
))}
</Tabs>
{tabsArray.map((item) => (
<TabScreen
key={item}
activeTab={activeTab}
index={item}
// You can add animation with adding a custom class
className="some-animation-class"
>
TabScreen {item}
</TabScreen>
))}
</>
);
};
export default SimpleTabs;
#Full example with all features
<Tabs
activeTab={activeTab}
onTabClick={(val) => console.log(val)}
action={tabRef}
isRTL={false}
didReachEnd={(val) => console.log(val)}
didReachStart={(val) => console.log(val)}
tabsScrollAmount={3}
animationDuration={300}
navBtnCLickAnimationDuration={300}
selectedAnimationDuration={300}
rightBtnIcon={">"}
leftBtnIcon={"<"}
hideNavBtns={false}
hideNavBtnsOnMobile={true}
showTabsScroll={false}
getTabsBoundingClientRects={(val) => console.log(val)}
action={ref}
mode="scrollSelectedToCenterFromView"
navBtnAs="div"
tabsContainerClassName=""
tabsUpperContainerClassName=""
tabsContainerClassName=""
leftNavBtnClassName=""
rightNavBtnClassName=""
navBtnClassName=""
navBtnContainerClassName=""
navBtnStyle={{}}
tabsContainerStyle={{}}
tabsUpperContainerStyle={{}}
tabsContainerRef={ref}
tabRef={ref}
leftNavBtnRef={ref}
rightNavBtnRef={ref}
>
<Tab>item </Tab>
{[...Array(20).keys()].map((tab) => (
<Tab key={tab}>Tab {tab}</Tab>
))}
</Tabs>
API
Name | Default | Type | Description |
activeTab* | - | integer | the selected tab value which must be passed to the commponent |
onTabClick* | - | function | function(event, value) => void callback function fires on tab click. It has two props, the first on is the event object the second on is the selected tab value |
mode | scrollSelectedToStart | string | it controls the behavoiur of the selected tab whether to move it to start | center | end | center if the tab is in the view.
it contains 3 modes:
|
action | - | ref | react ref fires when the component mounts. It's useful if you want to control some functionalities programmatically. It supports 4 function :
onLeftNavBtnClick ,onRightNavBtnClick, goToStart, goToEnd
onLeftNavBtnClick : to control the left btn click and use your own navigation button. you can call it by so ref.current.onLeftNavBtnClick()
onRightNavBtnClick : to control the right btn click and use your own navigation button. you can call it by so ref.current.onRightNavBtnClick()
goToStart : to control the tabs to go to the start of the tabs container. you can call it by so ref.current.goToStart()
goToEnd : to control the tabs to go to the end of the tabs container. you can call it by so ref.current.goToEnd()
|
isRTL | false | boolean | sets if the direction of the tabs is RTL or not |
didReachEnd | - | function | sets if the tabs reached the end point of the container didReachEnd={(val) => console.log(val)} |
didReachStart | - | function | sets if the tabs reached the start point of the container didReachStart={(val) => console.log(val)} |
tabsScrollAmount | 3 | string | integer | sets how many tabs you want to scroll on every move tabsScrollAmount={3} |
animationDuration | 300s | integer | sets the animation duration of the scroll when you click on the navigation buttons
note : this will overwirte the animationDuration value animationDuration={300} |
navBtnCLickAnimationDuration | 300s | integer | sets the animation of the scroll when you click on the navigation buttons
note : this will overwirte the animationDuration value navBtnCLickAnimationDuration={300} |
selectedAnimationDuration | 300s | integer | sets the animation of the scroll when you click on a tab that is out of the view
note : this will overwirte the animationDuration value selectedAnimationDuration={300} |
rightBtnIcon | feather arrow-right svg icon | string | jsx | sets the right navitgation button icon rightBtnIcon={'>'} |
leftBtnIcon | feather arrow-left svg icon | string | jsx | sets the left navitgation button icon leftBtnIcon={'>'} |
hideNavBtns | false | boolean | hides the navigantion button hideNavBtns={false} |
hideNavBtnsOnMobile | true | boolean | hides the navigation buttons on mobile devices |
showTabsScroll | false | boolean | shows the scroll of the tabsn |
getTabsBoundingClientRects | - | function | returns a callback with the DOMRects object of the selected tab and the tabsContainer. |
tabsContainerRef | - | ref | the tabs container ref object |
tabRef | - | ref | the tabs ref object and it returns an array of all the tab ref |
leftNavBtnRef | - | ref | sets the left navigation btn's ref |
rightNavBtnRef | - | ref | sets the right navigation btn's ref |
navBtnStyle | - | object | sets the navigation btns' style object |
tabsContainerStyle | - | object | Sets tabs container's style object |
tabsUpperContainerStyle | - | object | Sets the tabs upper container's style object |
leftNavBtnClassName | - | string | Sets the left navigation button's className |
rightNavBtnClassName | - | string | Sets the right navigation button's className |
navBtnClassName | - | string | Sets the navigation buttons' className |
navBtnContainerClassName | - | string | Sets the navigation buttons' container className |
tabsUpperContainerClassName | - | string | Sets the upper tabs container's className |
tabsContainerClassName | - | string | Sets the tabs container's className |
navBtnAs | button | string | Sets the HTML element of the navigation buttons |
Tab
Name | Default | Type | Description |
tabAs | button | string | You can change the HTML element of the
Tab
Note: Changing the underline element will affect on the accessiblity of the tab
|
style | - | object |
sets Tab's style object
|
className | - | string | you can pass a custom className to the Tab component |
TabScreen
Name | Default | Type | Description |
activeTab* | - | integer | the selected tab value which must be passed to the commponent |
index* | - | integer | string | the index of the tabscreen which must match the activeTab integer |
as | dov | string |
You can change the HTML element of the
TabScreen
|
style | - | object |
sets Tab's style object
|
className | - | string | you can pass a custom className to the TabScreen component |
If you liked this project don't forget to see my other projects on NPM and github
Contrubite
Show your support by giving a ⭐. Any feature requests are welcome!
Anyone is welcomed to contribute in this project.
Financial Contributions
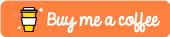
Buying me coffee will help me sustain the updates and work on new project for the open-source
Organizations
Support this project with your organization / company or individual.