react-toastify
Advanced tools
Comparing version 1.7.0 to 2.0.0-rc.1
@@ -1,2 +0,2 @@ | ||
!function(e,t){"object"==typeof exports&&"object"==typeof module?module.exports=t(require("react"),require("prop-types"),require("react-transition-group/TransitionGroup")):"function"==typeof define&&define.amd?define(["react","prop-types","react-transition-group/TransitionGroup"],t):"object"==typeof exports?exports.ReactOnScreen=t(require("react"),require("prop-types"),require("react-transition-group/TransitionGroup")):e.ReactOnScreen=t(e.react,e["prop-types"],e["react-transition-group/TransitionGroup"])}(this,function(e,t,n){return function(e){function t(o){if(n[o])return n[o].exports;var r=n[o]={i:o,l:!1,exports:{}};return e[o].call(r.exports,r,r.exports,t),r.l=!0,r.exports}var n={};return t.m=e,t.c=n,t.i=function(e){return e},t.d=function(e,n,o){t.o(e,n)||Object.defineProperty(e,n,{configurable:!1,enumerable:!0,get:o})},t.n=function(e){var n=e&&e.__esModule?function(){return e.default}:function(){return e};return t.d(n,"a",n),n},t.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},t.p="",t(t.s=11)}([function(t,n){t.exports=e},function(e,t,n){"use strict";Object.defineProperty(t,"__esModule",{value:!0}),t.default={POSITION:{TOP_LEFT:"top-left",TOP_RIGHT:"top-right",TOP_CENTER:"top-center",BOTTOM_LEFT:"bottom-left",BOTTOM_RIGHT:"bottom-right",BOTTOM_CENTER:"bottom-center"},TYPE:{INFO:"info",SUCCESS:"success",WARNING:"warning",ERROR:"error",DEFAULT:"default"},ACTION:{SHOW:"SHOW_TOAST",CLEAR:"CLEAR_TOAST",MOUNTED:"CONTAINER_MOUNTED"}}},function(e,n){e.exports=t},function(e,t,n){"use strict";function o(e){if(Array.isArray(e)){for(var t=0,n=Array(e.length);t<e.length;t++)n[t]=e[t];return n}return Array.from(e)}Object.defineProperty(t,"__esModule",{value:!0});var r={eventList:new Map,on:function(e,t){return this.eventList.has(e)||this.eventList.set(e,[]),this.eventList.get(e).push(t),this},off:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:null;return this.eventList.delete(e)},emit:function(e){for(var t=this,n=arguments.length,r=Array(n>1?n-1:0),i=1;i<n;i++)r[i-1]=arguments[i];return this.eventList.has(e)?(this.eventList.get(e).forEach(function(e){return setTimeout(function(){return e.call.apply(e,[t].concat(o(r)))},0)}),!0):(console.warn("<"+e+"> Event is not registered. Did you forgot to bind the event ?"),!1)}};t.default=r},function(e,t,n){"use strict";Object.defineProperty(t,"__esModule",{value:!0}),t.default=function(e){var t=[];return Object.keys(e).forEach(function(n){return t.push(e[n])}),t}},function(e,t,n){"use strict";function o(e){return Object.prototype.toString.call(e).slice(8,-1)}function r(e){return"Number"===o(e)&&!isNaN(e)&&e>0}function i(e){return e.isRequired=function(t,n,o){if(void 0===t[n])return new Error("The prop "+n+" is marked as required in \n "+o+", but its value is undefined.");e(t,n,o)},e}Object.defineProperty(t,"__esModule",{value:!0}),t.falseOrElement=t.falseOrNumber=void 0,t.typeOf=o,t.isValidDelay=r;var s=n(0);t.falseOrNumber=i(function(e,t,n){var o=e[t];return!1===o||r(o)?null:new Error(n+" expect "+t+" \n to be a valid Number > 0 or equal to false. "+o+" given.")}),t.falseOrElement=i(function(e,t,n){var o=e[t];return!1===o||(0,s.isValidElement)(o)?null:new Error(n+" expect "+t+" \n to be a valid react element or equal to false. "+o+" given.")})},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){if(Array.isArray(e)){for(var t=0,n=Array(e.length);t<e.length;t++)n[t]=e[t];return n}return Array.from(e)}function i(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function s(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function u(e,t){if(!e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return!t||"object"!=typeof t&&"function"!=typeof t?e:t}function a(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function, not "+typeof t);e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,enumerable:!1,writable:!0,configurable:!0}}),t&&(Object.setPrototypeOf?Object.setPrototypeOf(e,t):e.__proto__=t)}Object.defineProperty(t,"__esModule",{value:!0});var l=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var o in n)Object.prototype.hasOwnProperty.call(n,o)&&(e[o]=n[o])}return e},c=function(){function e(e,t){for(var n=0;n<t.length;n++){var o=t[n];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(e,o.key,o)}}return function(t,n,o){return n&&e(t.prototype,n),o&&e(t,o),t}}(),f=n(0),p=o(f),d=n(2),y=o(d),h=n(12),O=o(h),v=n(10),m=o(v),b=n(8),T=o(b),g=n(1),_=o(g),E=n(3),P=o(E),C=n(4),N=o(C),j=n(5),R=function(e){function t(e){s(this,t);var n=u(this,(t.__proto__||Object.getPrototypeOf(t)).call(this,e));return n.state={toast:[]},n.collection={},n}return a(t,e),c(t,[{key:"componentDidMount",value:function(){var e=this;P.default.on(_.default.ACTION.SHOW,function(t,n){return e.show(t,n)}).on(_.default.ACTION.CLEAR,function(t){return null!==t?e.removeToast(t):e.clear()}).emit(_.default.ACTION.MOUNTED)}},{key:"componentWillUnmount",value:function(){P.default.off(_.default.ACTION.SHOW),P.default.off(_.default.ACTION.CLEAR)}},{key:"removeToast",value:function(e){this.setState({toast:this.state.toast.filter(function(t){return t!==parseInt(e,10)})})}},{key:"with",value:function(e,t){return(0,f.cloneElement)(e,l({},t,e.props))}},{key:"makeCloseButton",value:function(e,t){var n=this,o=this.props.closeButton;return((0,f.isValidElement)(e)||!1===e)&&(o=e),!1!==o&&this.with(o,{closeToast:function(){return n.removeToast(t)}})}},{key:"getAutoCloseDelay",value:function(e){return!1===e||(0,j.isValidDelay)(e)?e:this.props.autoClose}},{key:"isFunction",value:function(e){return!!(e&&e.constructor&&e.call&&e.apply)}},{key:"canBeRendered",value:function(e){return(0,f.isValidElement)(e)||"String"===(0,j.typeOf)(e)||"Number"===(0,j.typeOf)(e)}},{key:"show",value:function(e,t){var n=this;if(this.canBeRendered(e)){var o=t.toastId,s=function(){return n.removeToast(o)},u={id:o,type:t.type,closeButton:this.makeCloseButton(t.closeButton,o),position:t.position||this.props.position};this.isFunction(t.onOpen)&&(u.onOpen=t.onOpen),this.isFunction(t.onClose)&&(u.onClose=t.onClose),u.autoClose=this.getAutoCloseDelay(!1!==t.autoClose?parseInt(t.autoClose,10):t.autoClose),u.hideProgressBar="boolean"==typeof t.hideProgressBar?t.hideProgressBar:this.props.hideProgressBar,u.closeToast=s,(0,f.isValidElement)(e)&&"String"!==(0,j.typeOf)(e.type)&&(e=this.with(e,{closeToast:s})),this.collection=Object.assign({},this.collection,i({},o,{content:this.makeToast(e,u),position:u.position})),this.setState({toast:[].concat(r(this.state.toast),[o])})}}},{key:"makeToast",value:function(e,t){return p.default.createElement(m.default,l({},t,{key:"toast-"+t.id+" "}),e)}},{key:"clear",value:function(){this.setState({toast:[]})}},{key:"hasToast",value:function(){return this.state.toast.length>0}},{key:"getContainerProps",value:function(e,t){var n={className:"toastify toastify--"+e,style:t?{pointerEvents:"none"}:{}};return null!==this.props.className&&(n.className=n.className+" "+this.props.className),null!==this.props.style&&(n.style=Object.assign({},this.props.style,n.style)),n}},{key:"renderToast",value:function(){var e=this,t={},n=Object.keys(this.collection);return n.forEach(function(o){var r=e.collection[o];t[r.position]||(t[r.position]=[]),e.state.toast.includes(parseInt(o,10))?t[r.position].push(r.content):(t[r.position].push(null),setTimeout(function(){return delete e.collection[o]},10*n.length))}),Object.keys(t).map(function(n){var o=1===t[n].length&&null===t[n][0];return p.default.createElement(O.default,l({component:"div"},e.getContainerProps(n,o),{key:"container-"+n}),t[n].map(function(e){return e}))})}},{key:"render",value:function(){return p.default.createElement("div",null,this.renderToast())}}]),t}(f.Component);R.propTypes={position:y.default.oneOf((0,N.default)(_.default.POSITION)),autoClose:j.falseOrNumber,closeButton:j.falseOrElement,hideProgressBar:y.default.bool,className:y.default.string,style:y.default.object},R.defaultProps={position:_.default.POSITION.TOP_RIGHT,autoClose:5e3,hideProgressBar:!1,closeButton:p.default.createElement(T.default,null),className:null,style:null},t.default=R},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){return Object.assign({},p,e,{toastId:++h})}Object.defineProperty(t,"__esModule",{value:!0});var i=n(3),s=o(i),u=n(1),a=o(u),l=a.default.POSITION,c=a.default.TYPE,f=a.default.ACTION,p={type:c.DEFAULT,autoClose:null,closeButton:null,hideProgressBar:null,position:null},d=!1,y=[],h=0;s.default.on(f.MOUNTED,function(){d=!0,y.forEach(function(e){s.default.emit(e.action,e.content,e.options)}),y=[]});var O=function(e,t){return d?s.default.emit(f.SHOW,e,t):y.push({action:f.SHOW,content:e,options:t}),t.toastId};t.default=Object.assign(function(e,t){return O(e,r(t))},{success:function(e,t){return O(e,Object.assign(r(t),{type:c.SUCCESS}))},info:function(e,t){return O(e,Object.assign(r(t),{type:c.INFO}))},warn:function(e,t){return O(e,Object.assign(r(t),{type:c.WARNING}))},error:function(e,t){return O(e,Object.assign(r(t),{type:c.ERROR}))},dismiss:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:null;return s.default.emit(f.CLEAR,e)}},{POSITION:l,TYPE:c})},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){var t=e.closeToast;return s.default.createElement("button",{className:"toastify__close",type:"button",onClick:t},"✖")}Object.defineProperty(t,"__esModule",{value:!0});var i=n(0),s=o(i),u=n(2),a=o(u);r.propTypes={closeToast:a.default.func},t.default=r},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){var t=e.delay,n=e.isRunning,o=e.closeToast,r=e.type,i=e.hide,u={animationDuration:t+"ms",animationPlayState:n?"running":"paused"};return u.WebkitAnimationPlayState=u.animationPlayState,i&&(u.opacity=0),s.default.createElement("div",{className:"toastify__progress toastify__progress--"+r,style:u,onAnimationEnd:o})}Object.defineProperty(t,"__esModule",{value:!0});var i=n(0),s=o(i),u=n(2),a=o(u),l=n(1),c=o(l);r.propTypes={delay:a.default.number.isRequired,isRunning:a.default.bool.isRequired,closeToast:a.default.func.isRequired,type:a.default.string,hide:a.default.bool},r.defaultProps={type:c.default.TYPE.DEFAULT,hide:!1},t.default=r},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function i(e,t){if(!e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return!t||"object"!=typeof t&&"function"!=typeof t?e:t}function s(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function, not "+typeof t);e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,enumerable:!1,writable:!0,configurable:!0}}),t&&(Object.setPrototypeOf?Object.setPrototypeOf(e,t):e.__proto__=t)}Object.defineProperty(t,"__esModule",{value:!0});var u=function(){function e(e,t){for(var n=0;n<t.length;n++){var o=t[n];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(e,o.key,o)}}return function(t,n,o){return n&&e(t.prototype,n),o&&e(t,o),t}}(),a=n(0),l=o(a),c=n(2),f=o(c),p=n(9),d=o(p),y=n(1),h=o(y),O=n(4),v=o(O),m=n(5),b=function(e){function t(e){r(this,t);var n=i(this,(t.__proto__||Object.getPrototypeOf(t)).call(this,e));return n.setRef=function(e){n.ref=e},n.pauseToast=function(){n.setState({isRunning:!1})},n.playToast=function(){n.setState({isRunning:!0})},n.ref=null,n.state={isRunning:!0},n}return s(t,e),u(t,[{key:"componentDidMount",value:function(){null!==this.props.onOpen&&this.props.onOpen(this.getChildrenProps())}},{key:"componentWillUnmount",value:function(){null!==this.props.onClose&&this.props.onClose(this.getChildrenProps())}},{key:"getChildrenProps",value:function(){return this.props.children.props}},{key:"getToastProps",value:function(){var e={className:"toastify-content toastify-content--"+this.props.type,ref:this.setRef};return!1!==this.props.autoClose&&(e.onMouseEnter=this.pauseToast,e.onMouseLeave=this.playToast),e}},{key:"componentWillAppear",value:function(e){this.ref.classList.add("toast-enter--"+this.props.position,"toastify-animated"),e()}},{key:"componentWillEnter",value:function(e){this.ref.classList.add("toast-enter--"+this.props.position,"toastify-animated"),e()}},{key:"componentWillLeave",value:function(e){this.ref.classList.remove("toast-enter--"+this.props.position,"toastify-animated"),this.ref.classList.add("toast-exit--"+this.props.position,"toastify-animated"),setTimeout(function(){return e()},750)}},{key:"render",value:function(){var e=this.props,t=e.closeButton,n=e.children,o=e.autoClose,r=e.type,i=e.hideProgressBar,s=e.closeToast;return l.default.createElement("div",this.getToastProps(),!1!==t&&t,l.default.createElement("div",{className:"toastify__body"},n),!1!==o&&l.default.createElement(d.default,{delay:o,isRunning:this.state.isRunning,closeToast:s,hide:i,type:r}))}}]),t}(a.Component);b.propTypes={closeButton:m.falseOrElement.isRequired,autoClose:m.falseOrNumber.isRequired,children:f.default.node.isRequired,closeToast:f.default.func.isRequired,position:f.default.oneOf((0,v.default)(h.default.POSITION)).isRequired,hideProgressBar:f.default.bool,onOpen:f.default.func,onClose:f.default.func,type:f.default.oneOf((0,v.default)(h.default.TYPE))},b.defaultProps={type:h.default.TYPE.DEFAULT,hideProgressBar:!1,onOpen:null,onClose:null},t.default=b},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}Object.defineProperty(t,"__esModule",{value:!0}),t.toast=t.ToastContainer=void 0;var r=n(6),i=o(r),s=n(7),u=o(s);t.ToastContainer=i.default,t.toast=u.default},function(e,t){e.exports=n}])}); | ||
!function(e,t){"object"==typeof exports&&"object"==typeof module?module.exports=t(require("react"),require("prop-types"),require("react-transition-group/TransitionGroup")):"function"==typeof define&&define.amd?define(["react","prop-types","react-transition-group/TransitionGroup"],t):"object"==typeof exports?exports.ReactOnScreen=t(require("react"),require("prop-types"),require("react-transition-group/TransitionGroup")):e.ReactOnScreen=t(e.react,e["prop-types"],e["react-transition-group/TransitionGroup"])}(this,function(e,t,n){return function(e){function t(o){if(n[o])return n[o].exports;var r=n[o]={i:o,l:!1,exports:{}};return e[o].call(r.exports,r,r.exports,t),r.l=!0,r.exports}var n={};return t.m=e,t.c=n,t.i=function(e){return e},t.d=function(e,n,o){t.o(e,n)||Object.defineProperty(e,n,{configurable:!1,enumerable:!0,get:o})},t.n=function(e){var n=e&&e.__esModule?function(){return e.default}:function(){return e};return t.d(n,"a",n),n},t.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},t.p="",t(t.s=11)}([function(t,n){t.exports=e},function(e,t,n){"use strict";Object.defineProperty(t,"__esModule",{value:!0}),t.default={POSITION:{TOP_LEFT:"top-left",TOP_RIGHT:"top-right",TOP_CENTER:"top-center",BOTTOM_LEFT:"bottom-left",BOTTOM_RIGHT:"bottom-right",BOTTOM_CENTER:"bottom-center"},TYPE:{INFO:"info",SUCCESS:"success",WARNING:"warning",ERROR:"error",DEFAULT:"default"},ACTION:{SHOW:"SHOW_TOAST",CLEAR:"CLEAR_TOAST",MOUNTED:"CONTAINER_MOUNTED"}}},function(e,n){e.exports=t},function(e,t,n){"use strict";function o(e){if(Array.isArray(e)){for(var t=0,n=Array(e.length);t<e.length;t++)n[t]=e[t];return n}return Array.from(e)}Object.defineProperty(t,"__esModule",{value:!0});var r={eventList:new Map,on:function(e,t){return this.eventList.has(e)||this.eventList.set(e,[]),this.eventList.get(e).push(t),this},off:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:null;return this.eventList.delete(e)},emit:function(e){for(var t=this,n=arguments.length,r=Array(n>1?n-1:0),s=1;s<n;s++)r[s-1]=arguments[s];return this.eventList.has(e)?(this.eventList.get(e).forEach(function(e){return setTimeout(function(){return e.call.apply(e,[t].concat(o(r)))},0)}),!0):(console.warn("<"+e+"> Event is not registered. Did you forgot to bind the event ?"),!1)}};t.default=r},function(e,t,n){"use strict";Object.defineProperty(t,"__esModule",{value:!0}),t.default=function(e){var t=[];return Object.keys(e).forEach(function(n){return t.push(e[n])}),t}},function(e,t,n){"use strict";function o(e){return Object.prototype.toString.call(e).slice(8,-1)}function r(e){return"Number"===o(e)&&!isNaN(e)&&e>0}function s(e){return e.isRequired=function(t,n,o){if(void 0===t[n])return new Error("The prop "+n+" is marked as required in \n "+o+", but its value is undefined.");e(t,n,o)},e}Object.defineProperty(t,"__esModule",{value:!0}),t.falseOrElement=t.falseOrNumber=void 0,t.typeOf=o,t.isValidDelay=r;var i=n(0);t.falseOrNumber=s(function(e,t,n){var o=e[t];return!1===o||r(o)?null:new Error(n+" expect "+t+" \n to be a valid Number > 0 or equal to false. "+o+" given.")}),t.falseOrElement=s(function(e,t,n){var o=e[t];return!1===o||(0,i.isValidElement)(o)?null:new Error(n+" expect "+t+" \n to be a valid react element or equal to false. "+o+" given.")})},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){if(Array.isArray(e)){for(var t=0,n=Array(e.length);t<e.length;t++)n[t]=e[t];return n}return Array.from(e)}function s(e,t,n){return t in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function i(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function a(e,t){if(!e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return!t||"object"!=typeof t&&"function"!=typeof t?e:t}function u(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function, not "+typeof t);e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,enumerable:!1,writable:!0,configurable:!0}}),t&&(Object.setPrototypeOf?Object.setPrototypeOf(e,t):e.__proto__=t)}Object.defineProperty(t,"__esModule",{value:!0});var l="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e},c=Object.assign||function(e){for(var t=1;t<arguments.length;t++){var n=arguments[t];for(var o in n)Object.prototype.hasOwnProperty.call(n,o)&&(e[o]=n[o])}return e},f=function(){function e(e,t){for(var n=0;n<t.length;n++){var o=t[n];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(e,o.key,o)}}return function(t,n,o){return n&&e(t.prototype,n),o&&e(t,o),t}}(),p=n(0),d=o(p),y=n(2),h=o(y),m=n(12),O=o(m),v=n(10),b=o(v),T=n(8),g=o(T),C=n(1),N=o(C),_=n(3),E=o(_),P=n(4),k=o(P),R=n(5),j=function(e){function t(e){i(this,t);var n=a(this,(t.__proto__||Object.getPrototypeOf(t)).call(this,e));return n.isToastActive=function(e){return-1!==n.state.toast.indexOf(parseInt(e,10))},n.state={toast:[]},n.collection={},n}return u(t,e),f(t,[{key:"componentDidMount",value:function(){var e=this,t=N.default.ACTION,n=t.SHOW,o=t.CLEAR,r=t.MOUNTED;E.default.on(n,function(t,n){return e.show(t,n)}).on(o,function(t){return null!==t?e.removeToast(t):e.clear()}).emit(r,this)}},{key:"componentWillUnmount",value:function(){E.default.off(N.default.ACTION.SHOW),E.default.off(N.default.ACTION.CLEAR)}},{key:"removeToast",value:function(e){this.setState({toast:this.state.toast.filter(function(t){return t!==parseInt(e,10)})})}},{key:"with",value:function(e,t){return(0,p.cloneElement)(e,c({},t,e.props))}},{key:"makeCloseButton",value:function(e,t){var n=this,o=this.props.closeButton;return((0,p.isValidElement)(e)||!1===e)&&(o=e),!1!==o&&this.with(o,{closeToast:function(){return n.removeToast(t)}})}},{key:"getAutoCloseDelay",value:function(e){return!1===e||(0,R.isValidDelay)(e)?e:this.props.autoClose}},{key:"isFunction",value:function(e){return!!(e&&e.constructor&&e.call&&e.apply)}},{key:"canBeRendered",value:function(e){return(0,p.isValidElement)(e)||"String"===(0,R.typeOf)(e)||"Number"===(0,R.typeOf)(e)}},{key:"show",value:function(e,t){var n=this;if(!this.canBeRendered(e))throw new Error("The element you provided cannot be rendered. You provided an element of type "+(void 0===e?"undefined":l(e)));var o=t.toastId,i=function(){return n.removeToast(o)},a={id:o,type:t.type,closeButton:this.makeCloseButton(t.closeButton,o),position:t.position||this.props.position,pauseOnHover:null!==t.pauseOnHover?t.pauseOnHover:this.props.pauseOnHover,closeOnClick:null!==t.closeOnClick?t.closeOnClick:this.props.closeOnClick,className:t.className||this.props.toastClassName,bodyClassName:t.bodyClassName||this.props.bodyClassName,progressClassName:t.progressClassName||this.props.progressClassName};this.isFunction(t.onOpen)&&(a.onOpen=t.onOpen),this.isFunction(t.onClose)&&(a.onClose=t.onClose),a.autoClose=this.getAutoCloseDelay(!1!==t.autoClose?parseInt(t.autoClose,10):t.autoClose),a.hideProgressBar="boolean"==typeof t.hideProgressBar?t.hideProgressBar:this.props.hideProgressBar,a.closeToast=i,(0,p.isValidElement)(e)&&"String"!==(0,R.typeOf)(e.type)&&(e=this.with(e,{closeToast:i})),this.collection=Object.assign({},this.collection,s({},o,{content:this.makeToast(e,a),position:a.position})),this.setState({toast:[].concat(r(this.state.toast),[o])})}},{key:"makeToast",value:function(e,t){return d.default.createElement(b.default,c({},t,{key:"toast-"+t.id+" "}),e)}},{key:"clear",value:function(){this.setState({toast:[]})}},{key:"hasToast",value:function(){return this.state.toast.length>0}},{key:"getContainerProps",value:function(e,t){var n={className:"toastify toastify--"+e,style:t?{pointerEvents:"none"}:{}};return null!==this.props.className&&(n.className=n.className+" "+this.props.className),null!==this.props.style&&(n.style=Object.assign({},this.props.style,n.style)),n}},{key:"renderToast",value:function(){var e=this,t={},n=this.props.newestOnTop?Object.keys(this.collection).reverse():Object.keys(this.collection);return n.forEach(function(o){var r=e.collection[o];t[r.position]||(t[r.position]=[]),-1!==e.state.toast.indexOf(parseInt(o,10))?t[r.position].push(r.content):(t[r.position].push(null),setTimeout(function(){return delete e.collection[o]},10*n.length))}),Object.keys(t).map(function(n){var o=1===t[n].length&&null===t[n][0];return d.default.createElement(O.default,c({component:"div"},e.getContainerProps(n,o),{key:"container-"+n}),t[n].map(function(e){return e}))})}},{key:"render",value:function(){return d.default.createElement("div",null,this.renderToast())}}]),t}(p.Component);j.propTypes={position:h.default.oneOf((0,k.default)(N.default.POSITION)),autoClose:R.falseOrNumber,closeButton:R.falseOrElement,hideProgressBar:h.default.bool,pauseOnHover:h.default.bool,closeOnClick:h.default.bool,newestOnTop:h.default.bool,className:h.default.string,style:h.default.object,toastClassName:h.default.string,bodyClassName:h.default.string,progressClassName:h.default.string},j.defaultProps={position:N.default.POSITION.TOP_RIGHT,autoClose:5e3,hideProgressBar:!1,closeButton:d.default.createElement(g.default,null),pauseOnHover:!0,closeOnClick:!0,newestOnTop:!1,className:null,style:null,toastClassName:"",bodyClassName:"",progressClassName:""},t.default=j},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){return Object.assign({},d,e,{toastId:++m})}function s(e,t){return null!==y?a.default.emit(p.SHOW,e,t):h.push({action:p.SHOW,content:e,options:t}),t.toastId}Object.defineProperty(t,"__esModule",{value:!0});var i=n(3),a=o(i),u=n(1),l=o(u),c=l.default.POSITION,f=l.default.TYPE,p=l.default.ACTION,d={type:f.DEFAULT,autoClose:null,closeButton:null,hideProgressBar:null,position:null,pauseOnHover:null,closeOnClick:null,className:null,bodyClassName:null,progressClassName:null},y=null,h=[],m=0,O=Object.assign(function(e,t){return s(e,r(t))},{success:function(e,t){return s(e,Object.assign(r(t),{type:f.SUCCESS}))},info:function(e,t){return s(e,Object.assign(r(t),{type:f.INFO}))},warn:function(e,t){return s(e,Object.assign(r(t),{type:f.WARNING}))},warning:function(e,t){return s(e,Object.assign(r(t),{type:f.WARNING}))},error:function(e,t){return s(e,Object.assign(r(t),{type:f.ERROR}))},dismiss:function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:null;return a.default.emit(p.CLEAR,e)},isActive:function(){return!1}},{POSITION:c,TYPE:f});a.default.on(p.MOUNTED,function(e){y=e,O.isActive=function(e){return y.isToastActive(e)},h.forEach(function(e){a.default.emit(e.action,e.content,e.options)}),h=[]}),t.default=O},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){var t=e.closeToast;return i.default.createElement("button",{className:"toastify__close",type:"button",onClick:t},"✖")}Object.defineProperty(t,"__esModule",{value:!0});var s=n(0),i=o(s),a=n(2),u=o(a);r.propTypes={closeToast:u.default.func},t.default=r},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e){var t=e.delay,n=e.isRunning,o=e.closeToast,r=e.type,s=e.hide,a=e.className,u={animationDuration:t+"ms",animationPlayState:n?"running":"paused"};return u.WebkitAnimationPlayState=u.animationPlayState,s&&(u.opacity=0),i.default.createElement("div",{className:"toastify__progress toastify__progress--"+r+" "+a,style:u,onAnimationEnd:o})}Object.defineProperty(t,"__esModule",{value:!0});var s=n(0),i=o(s),a=n(2),u=o(a),l=n(1),c=o(l);r.propTypes={delay:u.default.number.isRequired,isRunning:u.default.bool.isRequired,closeToast:u.default.func.isRequired,type:u.default.string,hide:u.default.bool,className:u.default.string},r.defaultProps={type:c.default.TYPE.DEFAULT,hide:!1,className:""},t.default=r},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}function r(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function s(e,t){if(!e)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return!t||"object"!=typeof t&&"function"!=typeof t?e:t}function i(e,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function, not "+typeof t);e.prototype=Object.create(t&&t.prototype,{constructor:{value:e,enumerable:!1,writable:!0,configurable:!0}}),t&&(Object.setPrototypeOf?Object.setPrototypeOf(e,t):e.__proto__=t)}Object.defineProperty(t,"__esModule",{value:!0});var a=function(){function e(e,t){for(var n=0;n<t.length;n++){var o=t[n];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(e,o.key,o)}}return function(t,n,o){return n&&e(t.prototype,n),o&&e(t,o),t}}(),u=n(0),l=o(u),c=n(2),f=o(c),p=n(9),d=o(p),y=n(1),h=o(y),m=n(4),O=o(m),v=n(5),b=function(e){function t(e){r(this,t);var n=s(this,(t.__proto__||Object.getPrototypeOf(t)).call(this,e));return n.setRef=function(e){n.ref=e},n.pauseToast=function(){n.setState({isRunning:!1})},n.playToast=function(){n.setState({isRunning:!0})},n.ref=null,n.state={isRunning:!0},n}return i(t,e),a(t,[{key:"componentDidMount",value:function(){null!==this.props.onOpen&&this.props.onOpen(this.getChildrenProps())}},{key:"componentWillUnmount",value:function(){null!==this.props.onClose&&this.props.onClose(this.getChildrenProps())}},{key:"getChildrenProps",value:function(){return this.props.children.props}},{key:"getToastProps",value:function(){var e={className:"toastify-content toastify-content--"+this.props.type+" "+this.props.className,ref:this.setRef};return!1!==this.props.autoClose&&!0===this.props.pauseOnHover&&(e.onMouseEnter=this.pauseToast,e.onMouseLeave=this.playToast),this.props.closeOnClick&&(e.onClick=this.props.closeToast),e}},{key:"componentWillAppear",value:function(e){this.ref.classList.add("toast-enter--"+this.props.position,"toastify-animated"),e()}},{key:"componentWillEnter",value:function(e){this.ref.classList.add("toast-enter--"+this.props.position,"toastify-animated"),e()}},{key:"componentWillLeave",value:function(e){this.ref.classList.remove("toast-enter--"+this.props.position,"toastify-animated"),this.ref.classList.add("toast-exit--"+this.props.position,"toastify-animated"),setTimeout(function(){return e()},750)}},{key:"render",value:function(){var e=this.props,t=e.closeButton,n=e.children,o=e.autoClose,r=e.type,s=e.hideProgressBar,i=e.closeToast;return l.default.createElement("div",this.getToastProps(),l.default.createElement("div",{className:"toastify__body "+this.props.bodyClassName},n),!1!==t&&t,!1!==o&&l.default.createElement(d.default,{delay:o,isRunning:this.state.isRunning,closeToast:i,hide:s,type:r,className:this.props.progressClassName}))}}]),t}(u.Component);b.propTypes={closeButton:v.falseOrElement.isRequired,autoClose:v.falseOrNumber.isRequired,children:f.default.node.isRequired,closeToast:f.default.func.isRequired,position:f.default.oneOf((0,O.default)(h.default.POSITION)).isRequired,pauseOnHover:f.default.bool.isRequired,closeOnClick:f.default.bool.isRequired,hideProgressBar:f.default.bool,onOpen:f.default.func,onClose:f.default.func,type:f.default.oneOf((0,O.default)(h.default.TYPE)),className:f.default.string,bodyClassName:f.default.string,progressClassName:f.default.string},b.defaultProps={type:h.default.TYPE.DEFAULT,hideProgressBar:!1,onOpen:null,onClose:null,className:"",bodyClassName:"",progressClassName:""},t.default=b},function(e,t,n){"use strict";function o(e){return e&&e.__esModule?e:{default:e}}Object.defineProperty(t,"__esModule",{value:!0}),t.toast=t.ToastContainer=void 0;var r=n(6),s=o(r),i=n(7),a=o(i);t.ToastContainer=s.default,t.toast=a.default},function(e,t){e.exports=n}])}); | ||
//# sourceMappingURL=ReactToastify.min.js.map |
@@ -12,5 +12,5 @@ 'use strict'; | ||
var _toastify = require('./toastify'); | ||
var _toaster = require('./toaster'); | ||
var _toastify2 = _interopRequireDefault(_toastify); | ||
var _toaster2 = _interopRequireDefault(_toaster); | ||
@@ -20,2 +20,2 @@ function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
exports.ToastContainer = _ToastContainer2.default; | ||
exports.toast = _toastify2.default; | ||
exports.toast = _toaster2.default; |
@@ -26,3 +26,4 @@ 'use strict'; | ||
type = _ref.type, | ||
hide = _ref.hide; | ||
hide = _ref.hide, | ||
className = _ref.className; | ||
@@ -40,3 +41,3 @@ var style = { | ||
return _react2.default.createElement('div', { | ||
className: 'toastify__progress toastify__progress--' + type, | ||
className: 'toastify__progress toastify__progress--' + type + ' ' + className, | ||
style: style, | ||
@@ -71,3 +72,8 @@ onAnimationEnd: closeToast | ||
*/ | ||
hide: _propTypes2.default.bool | ||
hide: _propTypes2.default.bool, | ||
/** | ||
* Optionnal className | ||
*/ | ||
className: _propTypes2.default.string | ||
}; | ||
@@ -77,5 +83,6 @@ | ||
type: _config2.default.TYPE.DEFAULT, | ||
hide: false | ||
hide: false, | ||
className: '' | ||
}; | ||
exports.default = ProgressBar; |
@@ -85,7 +85,7 @@ 'use strict'; | ||
var toastProps = { | ||
className: 'toastify-content toastify-content--' + this.props.type, | ||
className: 'toastify-content toastify-content--' + this.props.type + ' ' + this.props.className, | ||
ref: this.setRef | ||
}; | ||
if (this.props.autoClose !== false) { | ||
if (this.props.autoClose !== false && this.props.pauseOnHover === true) { | ||
toastProps.onMouseEnter = this.pauseToast; | ||
@@ -95,2 +95,4 @@ toastProps.onMouseLeave = this.playToast; | ||
this.props.closeOnClick && (toastProps.onClick = this.props.closeToast); | ||
return toastProps; | ||
@@ -134,8 +136,8 @@ } | ||
this.getToastProps(), | ||
closeButton !== false && closeButton, | ||
_react2.default.createElement( | ||
'div', | ||
{ className: 'toastify__body' }, | ||
{ className: 'toastify__body ' + this.props.bodyClassName }, | ||
children | ||
), | ||
closeButton !== false && closeButton, | ||
autoClose !== false && _react2.default.createElement(_ProgressBar2.default, { | ||
@@ -146,3 +148,4 @@ delay: autoClose, | ||
hide: hideProgressBar, | ||
type: type | ||
type: type, | ||
className: this.props.progressClassName | ||
}) | ||
@@ -162,6 +165,11 @@ ); | ||
position: _propTypes2.default.oneOf((0, _objectValues2.default)(_config2.default.POSITION)).isRequired, | ||
pauseOnHover: _propTypes2.default.bool.isRequired, | ||
closeOnClick: _propTypes2.default.bool.isRequired, | ||
hideProgressBar: _propTypes2.default.bool, | ||
onOpen: _propTypes2.default.func, | ||
onClose: _propTypes2.default.func, | ||
type: _propTypes2.default.oneOf((0, _objectValues2.default)(_config2.default.TYPE)) | ||
type: _propTypes2.default.oneOf((0, _objectValues2.default)(_config2.default.TYPE)), | ||
className: _propTypes2.default.string, | ||
bodyClassName: _propTypes2.default.string, | ||
progressClassName: _propTypes2.default.string | ||
}; | ||
@@ -172,4 +180,7 @@ Toast.defaultProps = { | ||
onOpen: null, | ||
onClose: null | ||
onClose: null, | ||
className: '', | ||
bodyClassName: '', | ||
progressClassName: '' | ||
}; | ||
exports.default = Toast; |
@@ -1,2 +0,2 @@ | ||
'use strict'; | ||
"use strict"; | ||
@@ -7,2 +7,4 @@ Object.defineProperty(exports, "__esModule", { | ||
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; | ||
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; }; | ||
@@ -12,35 +14,35 @@ | ||
var _react = require('react'); | ||
var _react = require("react"); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _propTypes = require('prop-types'); | ||
var _propTypes = require("prop-types"); | ||
var _propTypes2 = _interopRequireDefault(_propTypes); | ||
var _TransitionGroup = require('react-transition-group/TransitionGroup'); | ||
var _TransitionGroup = require("react-transition-group/TransitionGroup"); | ||
var _TransitionGroup2 = _interopRequireDefault(_TransitionGroup); | ||
var _Toast = require('./Toast'); | ||
var _Toast = require("./Toast"); | ||
var _Toast2 = _interopRequireDefault(_Toast); | ||
var _DefaultCloseButton = require('./DefaultCloseButton'); | ||
var _DefaultCloseButton = require("./DefaultCloseButton"); | ||
var _DefaultCloseButton2 = _interopRequireDefault(_DefaultCloseButton); | ||
var _config = require('./config'); | ||
var _config = require("./config"); | ||
var _config2 = _interopRequireDefault(_config); | ||
var _EventManager = require('./util/EventManager'); | ||
var _EventManager = require("./util/EventManager"); | ||
var _EventManager2 = _interopRequireDefault(_EventManager); | ||
var _objectValues = require('./util/objectValues'); | ||
var _objectValues = require("./util/objectValues"); | ||
var _objectValues2 = _interopRequireDefault(_objectValues); | ||
var _propValidator = require('./util/propValidator'); | ||
var _propValidator = require("./util/propValidator"); | ||
@@ -67,2 +69,6 @@ function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
_this.isToastActive = function (id) { | ||
return _this.state.toast.indexOf(parseInt(id, 10)) !== -1; | ||
}; | ||
_this.state = { | ||
@@ -76,14 +82,19 @@ toast: [] | ||
_createClass(ToastContainer, [{ | ||
key: 'componentDidMount', | ||
key: "componentDidMount", | ||
value: function componentDidMount() { | ||
var _this2 = this; | ||
_EventManager2.default.on(_config2.default.ACTION.SHOW, function (content, options) { | ||
var _config$ACTION = _config2.default.ACTION, | ||
SHOW = _config$ACTION.SHOW, | ||
CLEAR = _config$ACTION.CLEAR, | ||
MOUNTED = _config$ACTION.MOUNTED; | ||
_EventManager2.default.on(SHOW, function (content, options) { | ||
return _this2.show(content, options); | ||
}).on(_config2.default.ACTION.CLEAR, function (id) { | ||
}).on(CLEAR, function (id) { | ||
return id !== null ? _this2.removeToast(id) : _this2.clear(); | ||
}).emit(_config2.default.ACTION.MOUNTED); | ||
}).emit(MOUNTED, this); | ||
} | ||
}, { | ||
key: 'componentWillUnmount', | ||
key: "componentWillUnmount", | ||
value: function componentWillUnmount() { | ||
@@ -94,3 +105,3 @@ _EventManager2.default.off(_config2.default.ACTION.SHOW); | ||
}, { | ||
key: 'removeToast', | ||
key: "removeToast", | ||
value: function removeToast(id) { | ||
@@ -104,3 +115,3 @@ this.setState({ | ||
}, { | ||
key: 'with', | ||
key: "with", | ||
value: function _with(component, props) { | ||
@@ -110,3 +121,3 @@ return (0, _react.cloneElement)(component, _extends({}, props, component.props)); | ||
}, { | ||
key: 'makeCloseButton', | ||
key: "makeCloseButton", | ||
value: function makeCloseButton(toastClose, toastId) { | ||
@@ -128,3 +139,3 @@ var _this3 = this; | ||
}, { | ||
key: 'getAutoCloseDelay', | ||
key: "getAutoCloseDelay", | ||
value: function getAutoCloseDelay(toastAutoClose) { | ||
@@ -134,67 +145,66 @@ return toastAutoClose === false || (0, _propValidator.isValidDelay)(toastAutoClose) ? toastAutoClose : this.props.autoClose; | ||
}, { | ||
key: 'isFunction', | ||
key: "isFunction", | ||
value: function isFunction(object) { | ||
return !!(object && object.constructor && object.call && object.apply); | ||
} | ||
/** | ||
* TODO: check if throwing an error can be helpful | ||
*/ | ||
}, { | ||
key: 'canBeRendered', | ||
key: "canBeRendered", | ||
value: function canBeRendered(content) { | ||
return (0, _react.isValidElement)(content) || (0, _propValidator.typeOf)(content) === 'String' || (0, _propValidator.typeOf)(content) === 'Number'; | ||
return (0, _react.isValidElement)(content) || (0, _propValidator.typeOf)(content) === "String" || (0, _propValidator.typeOf)(content) === "Number"; | ||
} | ||
}, { | ||
key: 'show', | ||
key: "show", | ||
value: function show(content, options) { | ||
var _this4 = this; | ||
if (this.canBeRendered(content)) { | ||
var toastId = options.toastId; | ||
var closeToast = function closeToast() { | ||
return _this4.removeToast(toastId); | ||
}; | ||
var toastOptions = { | ||
id: toastId, | ||
type: options.type, | ||
closeButton: this.makeCloseButton(options.closeButton, toastId), | ||
position: options.position || this.props.position | ||
}; | ||
if (!this.canBeRendered(content)) { | ||
throw new Error("The element you provided cannot be rendered. You provided an element of type " + (typeof content === "undefined" ? "undefined" : _typeof(content))); | ||
} | ||
var toastId = options.toastId; | ||
var closeToast = function closeToast() { | ||
return _this4.removeToast(toastId); | ||
}; | ||
var toastOptions = { | ||
id: toastId, | ||
type: options.type, | ||
closeButton: this.makeCloseButton(options.closeButton, toastId), | ||
position: options.position || this.props.position, | ||
pauseOnHover: options.pauseOnHover !== null ? options.pauseOnHover : this.props.pauseOnHover, | ||
closeOnClick: options.closeOnClick !== null ? options.closeOnClick : this.props.closeOnClick, | ||
className: options.className || this.props.toastClassName, | ||
bodyClassName: options.bodyClassName || this.props.bodyClassName, | ||
progressClassName: options.progressClassName || this.props.progressClassName | ||
}; | ||
this.isFunction(options.onOpen) && (toastOptions.onOpen = options.onOpen); | ||
this.isFunction(options.onOpen) && (toastOptions.onOpen = options.onOpen); | ||
this.isFunction(options.onClose) && (toastOptions.onClose = options.onClose); | ||
this.isFunction(options.onClose) && (toastOptions.onClose = options.onClose); | ||
toastOptions.autoClose = this.getAutoCloseDelay(options.autoClose !== false ? parseInt(options.autoClose, 10) : options.autoClose); | ||
toastOptions.autoClose = this.getAutoCloseDelay(options.autoClose !== false ? parseInt(options.autoClose, 10) : options.autoClose); | ||
toastOptions.hideProgressBar = typeof options.hideProgressBar === 'boolean' ? options.hideProgressBar : this.props.hideProgressBar; | ||
toastOptions.hideProgressBar = typeof options.hideProgressBar === "boolean" ? options.hideProgressBar : this.props.hideProgressBar; | ||
toastOptions.closeToast = closeToast; | ||
toastOptions.closeToast = closeToast; | ||
if ((0, _react.isValidElement)(content) && (0, _propValidator.typeOf)(content.type) !== 'String') { | ||
content = this.with(content, { | ||
closeToast: closeToast | ||
}); | ||
} | ||
if ((0, _react.isValidElement)(content) && (0, _propValidator.typeOf)(content.type) !== "String") { | ||
content = this.with(content, { | ||
closeToast: closeToast | ||
}); | ||
} | ||
this.collection = Object.assign({}, this.collection, _defineProperty({}, toastId, { | ||
content: this.makeToast(content, toastOptions), | ||
position: toastOptions.position | ||
})); | ||
this.collection = Object.assign({}, this.collection, _defineProperty({}, toastId, { | ||
content: this.makeToast(content, toastOptions), | ||
position: toastOptions.position | ||
})); | ||
this.setState({ | ||
toast: [].concat(_toConsumableArray(this.state.toast), [toastId]) | ||
}); | ||
} | ||
this.setState({ | ||
toast: [].concat(_toConsumableArray(this.state.toast), [toastId]) | ||
}); | ||
} | ||
}, { | ||
key: 'makeToast', | ||
key: "makeToast", | ||
value: function makeToast(content, options) { | ||
return _react2.default.createElement( | ||
_Toast2.default, | ||
_extends({}, options, { | ||
key: 'toast-' + options.id + ' ' | ||
}), | ||
_extends({}, options, { key: "toast-" + options.id + " " }), | ||
content | ||
@@ -204,3 +214,3 @@ ); | ||
}, { | ||
key: 'clear', | ||
key: "clear", | ||
value: function clear() { | ||
@@ -210,3 +220,3 @@ this.setState({ toast: [] }); | ||
}, { | ||
key: 'hasToast', | ||
key: "hasToast", | ||
value: function hasToast() { | ||
@@ -216,11 +226,11 @@ return this.state.toast.length > 0; | ||
}, { | ||
key: 'getContainerProps', | ||
key: "getContainerProps", | ||
value: function getContainerProps(pos, disablePointer) { | ||
var props = { | ||
className: 'toastify toastify--' + pos, | ||
style: disablePointer ? { pointerEvents: 'none' } : {} | ||
className: "toastify toastify--" + pos, | ||
style: disablePointer ? { pointerEvents: "none" } : {} | ||
}; | ||
if (this.props.className !== null) { | ||
props.className = props.className + ' ' + this.props.className; | ||
props.className = props.className + " " + this.props.className; | ||
} | ||
@@ -235,3 +245,3 @@ | ||
}, { | ||
key: 'renderToast', | ||
key: "renderToast", | ||
value: function renderToast() { | ||
@@ -241,3 +251,3 @@ var _this5 = this; | ||
var toastToRender = {}; | ||
var collection = Object.keys(this.collection); | ||
var collection = this.props.newestOnTop ? Object.keys(this.collection).reverse() : Object.keys(this.collection); | ||
@@ -248,3 +258,3 @@ collection.forEach(function (toastId) { | ||
if (_this5.state.toast.includes(parseInt(toastId, 10))) { | ||
if (_this5.state.toast.indexOf(parseInt(toastId, 10)) !== -1) { | ||
toastToRender[item.position].push(item.content); | ||
@@ -267,5 +277,5 @@ } else { | ||
_extends({ | ||
component: 'div' | ||
component: "div" | ||
}, _this5.getContainerProps(position, disablePointer), { | ||
key: 'container-' + position | ||
key: "container-" + position | ||
}), | ||
@@ -279,6 +289,6 @@ toastToRender[position].map(function (item) { | ||
}, { | ||
key: 'render', | ||
key: "render", | ||
value: function render() { | ||
return _react2.default.createElement( | ||
'div', | ||
"div", | ||
null, | ||
@@ -315,2 +325,17 @@ this.renderToast() | ||
/** | ||
* Pause toast duration on hover | ||
*/ | ||
pauseOnHover: _propTypes2.default.bool, | ||
/** | ||
* Dismiss toast on click | ||
*/ | ||
closeOnClick: _propTypes2.default.bool, | ||
/** | ||
* Newest on top | ||
*/ | ||
newestOnTop: _propTypes2.default.bool, | ||
/** | ||
* An optional className | ||
@@ -323,3 +348,18 @@ */ | ||
*/ | ||
style: _propTypes2.default.object | ||
style: _propTypes2.default.object, | ||
/** | ||
* An optional className for the toast | ||
*/ | ||
toastClassName: _propTypes2.default.string, | ||
/** | ||
* An optional className for the toast body | ||
*/ | ||
bodyClassName: _propTypes2.default.string, | ||
/** | ||
* An optional className for the toast progress bar | ||
*/ | ||
progressClassName: _propTypes2.default.string | ||
}; | ||
@@ -331,5 +371,11 @@ ToastContainer.defaultProps = { | ||
closeButton: _react2.default.createElement(_DefaultCloseButton2.default, null), | ||
pauseOnHover: true, | ||
closeOnClick: true, | ||
newestOnTop: false, | ||
className: null, | ||
style: null | ||
style: null, | ||
toastClassName: '', | ||
bodyClassName: '', | ||
progressClassName: '' | ||
}; | ||
exports.default = ToastContainer; |
{ | ||
"name": "react-toastify", | ||
"version": "1.7.0", | ||
"version": "2.0.0-rc.1", | ||
"description": "React notification made easy", | ||
@@ -5,0 +5,0 @@ "keywords": [ |
423
README.md
@@ -1,10 +0,22 @@ | ||
# React Toastify [](https://travis-ci.org/fkhadra/react-toastify) []() []() []() | ||
# React Toastify [](https://travis-ci.org/fkhadra/react-toastify) []() []() []() | ||
React-Toastify allow you to add toast notification to your app with ease. | ||
 | ||
React-Toastify allow you to add notification to your app with ease. | ||
* [Demo](#demo) | ||
* [Installation](#installation) | ||
* [Features](#features) | ||
* [How it works ?](#how-it-works-) | ||
* [Usage](#usage) | ||
* [Simple](#simple) | ||
* [Positioning toast](#positioning-toast) | ||
* [Remove a toast programmatically](#remove-a-toast-programmatically) | ||
* [Prevent duplicate](#prevent-duplicate) | ||
* [Define hook](#define-hook) | ||
* [Set a custom close button or simply remove it](#set-a-custom-close-button-or-simply-remove-it) | ||
* [Define your style](#define-your-style) | ||
* [Mobile](#mobile) | ||
* [Api](#api) | ||
* [Browser Support](#browser-support) | ||
* [Release Notes](#release-notes) | ||
@@ -16,3 +28,3 @@ * [Contribute](#contribute) | ||
Live demo [here](https://fkhadra.github.io/react-toastify/) | ||
[A demo is worth thousand word](https://fkhadra.github.io/react-toastify/) | ||
@@ -35,3 +47,3 @@ ## Installation | ||
- Can display a react component inside the toast ! | ||
- Don't rely on `findDOMNode` | ||
- Don't rely on `findDOMNode` or any DOM hack | ||
- Has ```onOpen``` and ```onClose``` hooks. Both can access the props passed to the react component rendered inside the toast | ||
@@ -44,118 +56,322 @@ - Can be positioned per toast | ||
## How it works ? | ||
The component use a dead simple pubsub(observer pattern) to listen and trigger event. The pubsub allow us to display a toast from everywhere in our app. | ||
## Usage | ||
- Add a ToastContainer to your app | ||
### Simple | ||
By default all toasts will inherits ToastContainer's props. **Props defined on toast supersede ToastContainer's props.** | ||
```javascript | ||
//index.js | ||
import React from 'react'; | ||
import { render } from 'react-dom'; | ||
import { ToastContainer } from 'react-toastify'; | ||
import 'react-toastify/dist/ReactToastify.min.css'; | ||
import React, { Component } from 'react'; | ||
import { ToastContainer, toast } from 'react-toastify'; | ||
import 'react-toastify/dist/ReactToastify.min.css'; | ||
const App = () => { | ||
return ( | ||
<div> | ||
{/*Your others component*/} | ||
<ToastContainer /> | ||
</div> | ||
); | ||
}; | ||
class App extends Component { | ||
notify = () => toast("Wow so easy !"); | ||
render( | ||
<App/>, | ||
document.getElementById('root') | ||
); | ||
render(){ | ||
return ( | ||
<div> | ||
<button onClick={this.notify}>Notify !</button> | ||
{/* One container to rule them all! */} | ||
<ToastContainer | ||
position="top-right" | ||
type="default" | ||
autoClose={5000} | ||
hideProgressBar={false} | ||
newestOnTop={false} | ||
closeOnClick | ||
pauseOnHover | ||
/> | ||
{/*Can be written <ToastContainer />. Props defined are the same as the default one. */} | ||
</div> | ||
); | ||
} | ||
} | ||
``` | ||
### Positioning toast | ||
By default all the toasts will be positionned on the top right of your browser. If a position is set on a toast, the one defined on ToastContainer will be overwritten | ||
The following values are allowed: **top-right, top-center, top-left, bottom-right, bottom-center, bottom-left** | ||
For convenience, toast expose a POSITION property to avoid any typo. | ||
```javascript | ||
// toast.POSITION.TOP_LEFT, toast.POSITION.TOP_RIGHT, toast.POSITION.TOP_CENTER | ||
// toast.POSITION.BOTTOM_LEFT,toast.POSITION.BOTTOM_RIGHT, toast.POSITION.BOTTOM_CENTER | ||
import React, { Component } from 'react'; | ||
import { toast } from 'react-toastify'; | ||
class Position extends Component { | ||
notify = () => { | ||
toast("Default Notification !"); | ||
toast.success("Success Notification !", { | ||
position: toast.POSITION.TOP_CENTER | ||
}); | ||
toast.error("Error Notification !", { | ||
position: toast.POSITION.TOP_LEFT | ||
}); | ||
toast.warn("Warning Notification !", { | ||
position: toast.POSITION.BOTTOM_LEFT | ||
}); | ||
toast.info("Info Notification !", { | ||
position: toast.POSITION.BOTTOM_CENTER | ||
}); | ||
toast("Custom Style Notification !", { | ||
position: toast.POSITION.BOTTOM_RIGHT, | ||
className: 'dark-toast', | ||
progressClassName: 'transparent-progress' | ||
}); | ||
}; | ||
render(){ | ||
return <button onClick={this.notify}>Notify</button>; | ||
} | ||
} | ||
``` | ||
- Display a Toast from everywhere ! | ||
### Remove a toast programmatically | ||
An id is returned each time you display a toast, use it to remove a given toast programmatically by calling ```toast.dismiss(id)``` | ||
Without args, all the displayed toasts will be dismissed. | ||
```javascript | ||
//foo.js | ||
import React from 'react'; | ||
import { toast } from 'react-toastify'; | ||
import React, { Component } from 'react'; | ||
import { toast } from 'react-toastify'; | ||
const Greet = ({ name }) => <div>Hello {name}</div> | ||
function handleClick() { | ||
toast(<Greet name="John" />); | ||
} | ||
const ToastBtn = () => { | ||
return( | ||
<button onClick={handleClick}>My Awesome Button</button> | ||
) | ||
} | ||
class Example extends Component { | ||
toastId = null; | ||
notify = () => this.toastId = toast("Lorem ipsum dolor"); | ||
Define hook | ||
dismiss = () => toast.dismiss(this.toastId); | ||
dismissAll = () => toast.dismiss(); | ||
render(){ | ||
return ( | ||
<div> | ||
<button onClick={this.notify}>Notify</button> | ||
<button onClick={this.dismiss}>Dismiss</button> | ||
<button onClick={this.dismissAll}>Dismiss All</button> | ||
</div> | ||
); | ||
} | ||
} | ||
``` | ||
## Api | ||
### ToastContainer (Type : React Component) | ||
|Props|Type|Default|Description| | ||
|-----|----|-------|-----------| | ||
|position|string|top-right|Define where the toast appear| | ||
|autoClose|false\|int|5000|Delay in ms to close the toast. If set to false, the notification need to be closed manualy| | ||
|className|string|-|Add optional classes to the container| | ||
|style|object|-|Add optional inline style to the container| | ||
|closeButton|React Element\|false|-|A React Component to replace the default close button or `false` to hide the button| | ||
|hideProgressBar|bool|false|Display or not the progress bar below the toast(remaining time)| | ||
### Prevent duplicate | ||
- Position accept the following value : | ||
To prevent duplicates, you can check if a given toast is active by calling toast.isActive(id) like the snippet below. With this approach, you can decide with more precision whether or not you want to display a toast. | ||
```javascript | ||
top-right, top-center, top-left, bottom-right, bottom-center, bottom-left | ||
import React, { Component } from 'react'; | ||
import { toast } from 'react-toastify'; | ||
class Example extends Component { | ||
toastId = null; | ||
notify = () => { | ||
if (! toast.isActive(this.toastId)) { | ||
this.toastId = toast("I cannot be duplicated !"); | ||
} | ||
} | ||
render(){ | ||
return ( | ||
<div> | ||
<button onClick={this.notify}>Notify</button> | ||
</div> | ||
); | ||
} | ||
} | ||
``` | ||
You can use the toast object to avoid any typo : | ||
### Define hook | ||
You can define two hooks on toast: | ||
- onOpen is called inside componentDidMount | ||
- onClose is called inside componentWillUnmount | ||
```javascript | ||
import { toast } from 'react-toastify'; | ||
toast.POSITION.TOP_LEFT, toast.POSITION.TOP_RIGHT, toast.POSITION.TOP_CENTER | ||
toast.POSITION.BOTTOM_LEFT,toast.POSITION.BOTTOM_RIGHT, toast.POSITION.BOTTOM_CENTER | ||
``` | ||
- When using a custom close button, the component will receive a prop ```closeToast```. You need to call it to close the toast. | ||
import React, { Component } from 'react'; | ||
import { toast } from 'react-toastify'; | ||
class Hook extends Component { | ||
notify = () => toast("Lorem ipsum dolor", { | ||
onOpen: (childrenProps) => window.alert('I counted to infinity once then..'), | ||
onClose: (childrenProps) => window.alert('I counted to infinity twice') | ||
}); | ||
render(){ | ||
return <button onClick={this.notify}>Notify</button>; | ||
} | ||
} | ||
``` | ||
### Set a custom close button or simply remove it | ||
When you use a custom close button, your button will receive a ```closeToast``` props. You need to call it in order to close the toast. The customCloseButton can be passed to the ToastContainer so all the toast display the custom close or you can set it by toast. | ||
If ```customCloseButton``` is set to false, the close button will be not displayed. | ||
```javascript | ||
//The classname toastify__close is used to position the icon on the top right side, you don't need it. | ||
const FontAwesomeCloseButton = ({ closeToast }) => ( | ||
<i | ||
className="toastify__close fa fa-times" | ||
onClick={closeToast} | ||
/> | ||
); | ||
import React, { Component } from 'react'; | ||
import { toast } from 'react-toastify'; | ||
... | ||
<ToastContainer autoClose={false} position={toast.POSITION.TOP_CENTER} closeButton={<FontAwesomeCloseButton />}/> | ||
... | ||
const CloseButton = ({ YouCanPassProps, closeToast }) => ( | ||
<i | ||
className="material-icons" | ||
onClick={closeToast} | ||
> | ||
delete | ||
</i> | ||
); | ||
class CustomClose extends Component { | ||
notify = () => { | ||
toast("The close button change when Chuck Norris display a toast",{ | ||
closeButton: <CloseButton YouCanPassProps="foo" /> | ||
}); | ||
}; | ||
render(){ | ||
return <button onClick={this.notify}>Notify</button>; | ||
} | ||
} | ||
``` | ||
### Define your style | ||
Taste and colours are not always the same ! You have several options. | ||
- Overwrite ```toastify-content``` and ```toastify__progress``` | ||
- Create two css classes and pass them to a toast | ||
```css | ||
.dark-toast{ | ||
background: #323232; | ||
color: #fff; | ||
} | ||
.dark-toast > .toastify__close{ | ||
color: #fff; | ||
} | ||
.transparent-progress{ | ||
background: rgba(255,255,255,.7); | ||
} | ||
.fancy-progress{ | ||
background: repeating-radial-gradient(red, yellow 10%, green 15%); | ||
} | ||
``` | ||
```javascript | ||
import React, { Component } from 'react'; | ||
import { toast } from 'react-toastify'; | ||
class Style extends Component { | ||
notify = () => { | ||
toast("Dark style notification with default type progress bar",{ | ||
className: 'dark-toast', | ||
autoClose: 5000 | ||
}); | ||
toast("Dark style with transparent progress bar, the one used with others types.",{ | ||
className: 'dark-toast', | ||
progressClassName: 'transparent-progress', | ||
autoClose: 5000 | ||
}); | ||
toast("Dark style with ugly progress bar",{ | ||
className: 'dark-toast', | ||
progressClassName: 'fancy-progress', | ||
autoClose: 5000 | ||
}); | ||
}; | ||
render(){ | ||
return <button onClick={this.notify}>Notify</button>; | ||
} | ||
} | ||
``` | ||
- Or pass your classes to the ToastContainer to set the classes for all your toast in one shot. | ||
```javascript | ||
render(){ | ||
return( | ||
{/*Component*/} | ||
<ToastContainer | ||
toastClassName="dark-toast" | ||
progressClassName="transparent-progress" | ||
/> | ||
{/*Component*/} | ||
); | ||
} | ||
``` | ||
### Mobile | ||
On mobile the toast will take all the width available. | ||
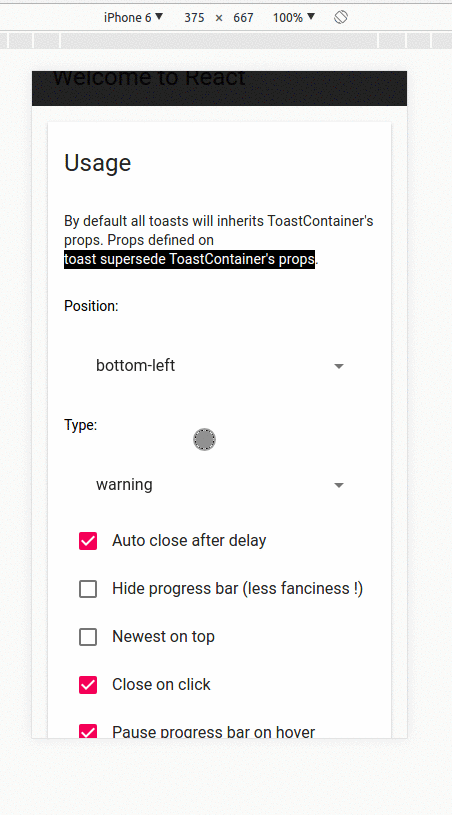 | ||
## Api | ||
### ToastContainer (Type : React Component) | ||
| Props | Type | Default | Description | | ||
| ----------------- | -------------- | --------- | --------------------------------------------------------------- | | ||
| position | string | top-right | One of top-right, top-center, top-left, bottom-right, bottom-center, bottom-left | | ||
| autoClose | false or int | 5000 | Delay in ms to close the toast. If set to false, the notification need to be closed manualy | | ||
| closeButton | React Element or false | - | A React Component to replace the default close button or `false` to hide the button | | ||
| hideProgressBar | bool | false | Display or not the progress bar below the toast(remaining time) | | ||
| pauseOnHover | bool | true | Keep the timer running or not on hover | | ||
| closeOnClick | bool | true | Dismiss toast on click | | ||
| newestOnTop | bool | false | Display newest toast on top | | ||
| className | string | - | Add optional classes to the container | | ||
| style | object | - | Add optional inline style to the container | | ||
| toastClassName | string | - | Add optional classes to the toast | | ||
| bodyClassName | string | - | Add optional classes to the toast body | | ||
| progressClassName | string | - | Add optional classes to the progress bar | | ||
### toast (Type: Object) | ||
All the method of toast return a **toastId** except `dismiss`. The **toastId** can be used to remove a toast programmatically. | ||
All the method but `dismiss` can take 2 parameters : | ||
All the method of toast return a **toastId** except `dismiss` and `isActive`. | ||
The **toastId** can be used to remove a toast programmatically or to check if the toast is displayed. | ||
|Parameter|Type|Required|Description| | ||
|---------|----|--------|-----| | ||
|content|string\|React Element|✓|Element that will be displayed| | ||
|options|object|✘|Possible keys : autoClose, type, closeButton, hideProgressBar|| | ||
| Parameter | Type | Required | Description | | ||
| --------- | ------- | ------------- | ------------------------------------------------------------- | | ||
| content | string\ | React Element | ✓ | Element that will be displayed | | ||
| options | object | ✘ | Possible keys : autoClose, type, closeButton, hideProgressBar | | | ||
- Available options : | ||
- `type`: Kind of notification. One of "default", "success", "info", "warning", "error". You can use `toast.TYPE.INFO` and so on to avoid any typo. | ||
- `onOpen`: callback before showing the notification. If you display a component its props will be passed to the callback as first parameter. | ||
- `onClose`: callback after closing the notification. If you display a component its props will be passed to the callback as first parameter. | ||
- `onOpen`: Called inside componentDidMount | ||
- `onClose`: Called inside componentWillUnmount | ||
- `autoClose`: same as ToastContainer. | ||
- `closeButton`: same as ToastContainer. | ||
- `closeOnClick`: same as ToastContainer. | ||
- `hideProgressBar`: same as ToastContainer. | ||
- `position`: same as ToastContainer | ||
- `pauseOnHover`: same as ToastContainer | ||
- `className`: same as ToastContainer toastClassName | ||
- `bodyClassName`: same as ToastContainer | ||
- `progressClassName`: same as ToastContainer | ||
:warning:️ *Toast options supersede ToastContainer props* :warning: | ||
:warning:️ *autoClose, closeButton, hideProgressBar, position supersede ToastContainer props* :warning: | ||
```javascript | ||
@@ -170,6 +386,6 @@ const Img = ({ src }) => <div><img width={48} src={src} /></div>; | ||
hideProgressBar: false, | ||
position: toast.POSITION.TOP_LEFT | ||
position: toast.POSITION.TOP_LEFT, | ||
pauseOnHover: true | ||
}; | ||
// each method return a toast id except dismiss. | ||
const toastId = toast(<Img foo={bar}/>, options) // default, type: 'default' | ||
@@ -182,7 +398,34 @@ toast.success("Hello", options) // add type: 'success' to options | ||
toast.dismiss(toastId) // Remove given toast | ||
toast.isActive(toastId) //Check if a toast is displayed or not | ||
``` | ||
## Browser Support | ||
Chrome | Firefox | IE 11 | Edge | Safari | ||
--- | --- | --- | --- | --- | | ||
✔ | ✔ | Partial, need to fix animation asap | ✔ | ✔ | | ||
## Release Notes | ||
### V2.0.0 | ||
This version may introduce breaking changes due to redesign. My apologies. | ||
But, it brings a lots of new and exciting features ! | ||
#### New Features | ||
- The default design has been reviewed. The component is now usable out of the box without the need to touch the css. Relate to [issue #28](https://github.com/fkhadra/react-toastify/issues/28) | ||
- The toast timer can keep running on hover. [issue #33](https://github.com/fkhadra/react-toastify/issues/33) | ||
- Added a possibility to check if a given toast is displayed or not. By using that method we can prevent duplicate. [issue #3](https://github.com/fkhadra/react-toastify/issues/3) | ||
- Can decide to close the toast on click | ||
- Can show newest toast on top | ||
- Can define additionnal className for toast[issue #21](https://github.com/fkhadra/react-toastify/issues/21) | ||
- Much more mobile ready than the past | ||
#### Bug Fixes | ||
- The space in of left boxes from window & right boxes from window is different.[issue #25](https://github.com/fkhadra/react-toastify/issues/25) | ||
- Partial support of ie11. I still need to fix the animation but I need a computer with ie11 for that xD [issue #26](https://github.com/fkhadra/react-toastify/issues/26) | ||
### v1.7.0 | ||
@@ -189,0 +432,0 @@ |
import ToastContainer from './ToastContainer'; | ||
import toastify from './toastify'; | ||
import toaster from './toaster'; | ||
export { ToastContainer, toastify as toast }; | ||
export { ToastContainer, toaster as toast }; |
@@ -6,3 +6,3 @@ import React from 'react'; | ||
function ProgressBar({ delay, isRunning, closeToast, type, hide }) { | ||
function ProgressBar({ delay, isRunning, closeToast, type, hide, className }) { | ||
const style = { | ||
@@ -20,3 +20,3 @@ animationDuration: `${delay}ms`, | ||
<div | ||
className={`toastify__progress toastify__progress--${type}`} | ||
className={`toastify__progress toastify__progress--${type} ${className}`} | ||
style={style} | ||
@@ -52,3 +52,8 @@ onAnimationEnd={closeToast} | ||
*/ | ||
hide: PropTypes.bool | ||
hide: PropTypes.bool, | ||
/** | ||
* Optionnal className | ||
*/ | ||
className: PropTypes.string | ||
}; | ||
@@ -58,5 +63,6 @@ | ||
type: config.TYPE.DEFAULT, | ||
hide: false | ||
hide: false, | ||
className: '' | ||
}; | ||
export default ProgressBar; |
@@ -16,6 +16,11 @@ import React, { Component } from 'react'; | ||
position: PropTypes.oneOf(objectValues(config.POSITION)).isRequired, | ||
pauseOnHover: PropTypes.bool.isRequired, | ||
closeOnClick: PropTypes.bool.isRequired, | ||
hideProgressBar: PropTypes.bool, | ||
onOpen: PropTypes.func, | ||
onClose: PropTypes.func, | ||
type: PropTypes.oneOf(objectValues(config.TYPE)) | ||
type: PropTypes.oneOf(objectValues(config.TYPE)), | ||
className: PropTypes.string, | ||
bodyClassName: PropTypes.string, | ||
progressClassName: PropTypes.string | ||
}; | ||
@@ -27,3 +32,6 @@ | ||
onOpen: null, | ||
onClose: null | ||
onClose: null, | ||
className: '', | ||
bodyClassName: '', | ||
progressClassName: '' | ||
}; | ||
@@ -57,7 +65,7 @@ | ||
const toastProps = { | ||
className: `toastify-content toastify-content--${this.props.type}`, | ||
className: `toastify-content toastify-content--${this.props.type} ${this.props.className}`, | ||
ref: this.setRef | ||
}; | ||
if (this.props.autoClose !== false) { | ||
if (this.props.autoClose !== false && this.props.pauseOnHover === true) { | ||
toastProps.onMouseEnter = this.pauseToast; | ||
@@ -67,2 +75,4 @@ toastProps.onMouseLeave = this.playToast; | ||
this.props.closeOnClick && (toastProps.onClick = this.props.closeToast); | ||
return toastProps; | ||
@@ -110,6 +120,6 @@ } | ||
> | ||
{closeButton !== false && closeButton} | ||
<div className="toastify__body"> | ||
<div className={`toastify__body ${this.props.bodyClassName}`}> | ||
{children} | ||
</div> | ||
{closeButton !== false && closeButton} | ||
{ | ||
@@ -123,2 +133,3 @@ autoClose !== false && | ||
type={type} | ||
className={this.props.progressClassName} | ||
/> | ||
@@ -125,0 +136,0 @@ } |
@@ -1,14 +0,10 @@ | ||
import React, { | ||
Component, | ||
isValidElement, | ||
cloneElement | ||
} from 'react'; | ||
import PropTypes from 'prop-types'; | ||
import Transition from 'react-transition-group/TransitionGroup'; | ||
import React, { Component, isValidElement, cloneElement } from "react"; | ||
import PropTypes from "prop-types"; | ||
import Transition from "react-transition-group/TransitionGroup"; | ||
import Toast from './Toast'; | ||
import DefaultCloseButton from './DefaultCloseButton'; | ||
import config from './config'; | ||
import EventManager from './util/EventManager'; | ||
import objectValues from './util/objectValues'; | ||
import Toast from "./Toast"; | ||
import DefaultCloseButton from "./DefaultCloseButton"; | ||
import config from "./config"; | ||
import EventManager from "./util/EventManager"; | ||
import objectValues from "./util/objectValues"; | ||
import { | ||
@@ -19,6 +15,5 @@ falseOrNumber, | ||
typeOf | ||
} from './util/propValidator'; | ||
} from "./util/propValidator"; | ||
class ToastContainer extends Component { | ||
static propTypes = { | ||
@@ -46,2 +41,17 @@ /** | ||
/** | ||
* Pause toast duration on hover | ||
*/ | ||
pauseOnHover: PropTypes.bool, | ||
/** | ||
* Dismiss toast on click | ||
*/ | ||
closeOnClick: PropTypes.bool, | ||
/** | ||
* Newest on top | ||
*/ | ||
newestOnTop: PropTypes.bool, | ||
/** | ||
* An optional className | ||
@@ -54,3 +64,18 @@ */ | ||
*/ | ||
style: PropTypes.object | ||
style: PropTypes.object, | ||
/** | ||
* An optional className for the toast | ||
*/ | ||
toastClassName: PropTypes.string, | ||
/** | ||
* An optional className for the toast body | ||
*/ | ||
bodyClassName: PropTypes.string, | ||
/** | ||
* An optional className for the toast progress bar | ||
*/ | ||
progressClassName: PropTypes.string, | ||
}; | ||
@@ -63,4 +88,10 @@ | ||
closeButton: <DefaultCloseButton />, | ||
pauseOnHover: true, | ||
closeOnClick: true, | ||
newestOnTop: false, | ||
className: null, | ||
style: null | ||
style: null, | ||
toastClassName: '', | ||
bodyClassName: '', | ||
progressClassName: '', | ||
}; | ||
@@ -77,8 +108,7 @@ | ||
componentDidMount() { | ||
const { SHOW, CLEAR, MOUNTED } = config.ACTION; | ||
EventManager | ||
.on(config.ACTION.SHOW, | ||
(content, options) => this.show(content, options)) | ||
.on(config.ACTION.CLEAR, | ||
id => (id !== null ? this.removeToast(id) : this.clear())) | ||
.emit(config.ACTION.MOUNTED); | ||
.on(SHOW, (content, options) => this.show(content, options)) | ||
.on(CLEAR, id => (id !== null ? this.removeToast(id) : this.clear())) | ||
.emit(MOUNTED, this); | ||
} | ||
@@ -91,2 +121,4 @@ | ||
isToastActive = id => this.state.toast.indexOf(parseInt(id, 10)) !== -1; | ||
removeToast(id) { | ||
@@ -98,2 +130,4 @@ this.setState({ | ||
is | ||
with(component, props) { | ||
@@ -127,29 +161,37 @@ return cloneElement(component, { ...props, ...component.props }); | ||
/** | ||
* TODO: check if throwing an error can be helpful | ||
*/ | ||
canBeRendered(content) { | ||
return isValidElement(content) | ||
|| typeOf(content) === 'String' | ||
|| typeOf(content) === 'Number'; | ||
return ( | ||
isValidElement(content) || | ||
typeOf(content) === "String" || | ||
typeOf(content) === "Number" | ||
); | ||
} | ||
show(content, options) { | ||
if (this.canBeRendered(content)) { | ||
const toastId = options.toastId; | ||
const closeToast = () => this.removeToast(toastId); | ||
const toastOptions = { | ||
id: toastId, | ||
type: options.type, | ||
closeButton: this.makeCloseButton(options.closeButton, toastId), | ||
position: options.position || this.props.position | ||
}; | ||
if (!(this.canBeRendered(content))) { | ||
throw new Error(`The element you provided cannot be rendered. You provided an element of type ${typeof content}`); | ||
} | ||
const toastId = options.toastId; | ||
const closeToast = () => this.removeToast(toastId); | ||
const toastOptions = { | ||
id: toastId, | ||
type: options.type, | ||
closeButton: this.makeCloseButton(options.closeButton, toastId), | ||
position: options.position || this.props.position, | ||
pauseOnHover: | ||
options.pauseOnHover !== null | ||
? options.pauseOnHover | ||
: this.props.pauseOnHover, | ||
closeOnClick: options.closeOnClick !== null ? options.closeOnClick : this.props.closeOnClick, | ||
className: options.className || this.props.toastClassName, | ||
bodyClassName: options.bodyClassName || this.props.bodyClassName, | ||
progressClassName: options.progressClassName || this.props.progressClassName, | ||
}; | ||
this.isFunction(options.onOpen) && | ||
(toastOptions.onOpen = options.onOpen); | ||
this.isFunction(options.onOpen) && (toastOptions.onOpen = options.onOpen); | ||
this.isFunction(options.onClose) && | ||
(toastOptions.onClose = options.onClose); | ||
this.isFunction(options.onClose) && | ||
(toastOptions.onClose = options.onClose); | ||
toastOptions.autoClose = this.getAutoCloseDelay( | ||
toastOptions.autoClose = this.getAutoCloseDelay( | ||
options.autoClose !== false | ||
@@ -160,26 +202,25 @@ ? parseInt(options.autoClose, 10) | ||
toastOptions.hideProgressBar = typeof options.hideProgressBar === | ||
'boolean' | ||
? options.hideProgressBar | ||
: this.props.hideProgressBar; | ||
toastOptions.hideProgressBar = | ||
typeof options.hideProgressBar === "boolean" | ||
? options.hideProgressBar | ||
: this.props.hideProgressBar; | ||
toastOptions.closeToast = closeToast; | ||
toastOptions.closeToast = closeToast; | ||
if (isValidElement(content) && typeOf(content.type) !== 'String') { | ||
content = this.with(content, { | ||
closeToast | ||
}); | ||
if (isValidElement(content) && typeOf(content.type) !== "String") { | ||
content = this.with(content, { | ||
closeToast | ||
}); | ||
} | ||
this.collection = Object.assign({}, this.collection, { | ||
[toastId]: { | ||
content: this.makeToast(content, toastOptions), | ||
position: toastOptions.position | ||
} | ||
}); | ||
this.collection = Object.assign({}, this.collection, { | ||
[toastId]: { | ||
content: this.makeToast(content, toastOptions), | ||
position: toastOptions.position | ||
} | ||
}); | ||
this.setState({ | ||
toast: [...this.state.toast, toastId] | ||
}); | ||
} | ||
this.setState({ | ||
toast: [...this.state.toast, toastId] | ||
}); | ||
} | ||
@@ -189,6 +230,3 @@ | ||
return ( | ||
<Toast | ||
{...options} | ||
key={`toast-${options.id} `} | ||
> | ||
<Toast {...options} key={`toast-${options.id} `}> | ||
{content} | ||
@@ -210,3 +248,3 @@ </Toast> | ||
className: `toastify toastify--${pos}`, | ||
style: disablePointer ? { pointerEvents: 'none' } : {} | ||
style: disablePointer ? { pointerEvents: "none" } : {} | ||
}; | ||
@@ -219,6 +257,3 @@ | ||
if (this.props.style !== null) { | ||
props.style = Object.assign({}, | ||
this.props.style, | ||
props.style | ||
); | ||
props.style = Object.assign({}, this.props.style, props.style); | ||
} | ||
@@ -231,3 +266,5 @@ | ||
const toastToRender = {}; | ||
const collection = Object.keys(this.collection); | ||
const collection = this.props.newestOnTop | ||
? Object.keys(this.collection).reverse() | ||
: Object.keys(this.collection); | ||
@@ -238,3 +275,3 @@ collection.forEach(toastId => { | ||
if (this.state.toast.includes(parseInt(toastId, 10))) { | ||
if (this.state.toast.indexOf(parseInt(toastId, 10)) !== -1) { | ||
toastToRender[item.position].push(item.content); | ||
@@ -246,4 +283,5 @@ } else { | ||
setTimeout( | ||
() => delete this.collection[toastId] | ||
, collection.length * 10); | ||
() => delete this.collection[toastId], | ||
collection.length * 10 | ||
); | ||
} | ||
@@ -253,4 +291,5 @@ }); | ||
return Object.keys(toastToRender).map(position => { | ||
const disablePointer = toastToRender[position].length === 1 | ||
&& toastToRender[position][0] === null; | ||
const disablePointer = | ||
toastToRender[position].length === 1 && | ||
toastToRender[position][0] === null; | ||
@@ -257,0 +296,0 @@ return ( |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
369246
1628
528
2