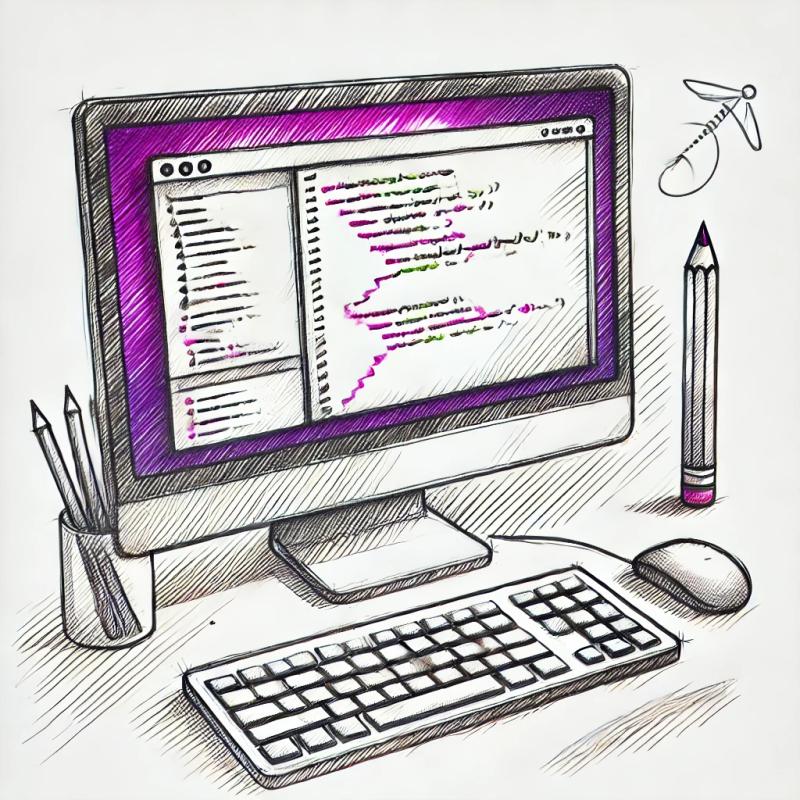
Security News
New axobject-query Maintainer Faces Backlash Over Controversial Decision to Support Legacy Node.js Versions
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.
react-uicomp
Advanced tools
Readme
React UI component for creating complex routes and beautiful UI
npm install react-uicomp --save
Navigation lets you define all the public, private and protected routes. Protected routes are types of public routes but are restricted which means it cannot be accessed after the user has logged into the web application. To use Navigation, wrap entire application with <Navigation.Provider> and provide publicRoutes, privateRoutes and userRoles.
Example
import React from "react";
import { Navigation } from "react-uicomp";
import { Page1, Page2 } from "./Pages";
// Array of object having key, name, path, component and restricted.
const publicPaths = [
{
key: "Public",
name: "Public",
path: "/public",
component: Page1,
restricted: true,
},
];
// Array of object having key, name, path and component.
const privatePaths = [
{
key: "Private",
name: "Private",
path: "/private",
component: Page2,
},
];
// Define user role and provide access routes.
const userRoles = {
user: { access: ["/public"] },
admin:
};
const App = () => {
return (
<Navigation.Provider
publicPaths={publicPaths}
privatePaths={privatePaths}
userRoles={userRoles}
>
// ...
</Navigation.Provider>
);
};
export default App;
Auth lets you authenticate if a user is logged in or not. It has <Auth.Provider> where you define the config prop object with isLoggedIn and userRole. It also has state prop where you can pass any object which will be available in entire application. And to render all the pages you have set up, use <Auth.Screens /> inside <Auth.Provider>.
Example
// import Auth from here
import { Navigation, Auth } from "react-uicomp";
...
const App = () => {
const [config, setConfig] = useState({ isLoggedIn: false, userRole: "user" });
return (
<Navigation.Provider
publicPaths={publicPaths}
privatePaths={privatePaths}
userRoles={userRoles}
>
<Auth.Provider
config={config}
state={{
logout: () => {
setConfig({ isLoggedIn: false, userRole: "user" });
}
}}
>
<Auth.Screens />
</Auth.Provider>
</Navigation.Provider>
);
};
It has useAuth() hook which lets you access state object from any component from entire application.
Example
// import useAuth
import { useAuth } from "react-uicomp";
export default function() {
// logout function is available on state prop in <Auth.Provider>
const { logout } = useAuth();
return () {
// ...
}
}
Theming is very essential to every app nowadays. So, we provided theming control in this package. Lets say, if you want to create dark mode and light mode in application. So, lets define both dark and light mode objects.
Example
// Dark theme object variable
const darkTheme = {
dark: true,
// colors cannot have other keys except these...
colors: {
backgroundColor: "#1F1B24",
primaryColor: "#1A6AA7",
secondaryColor: "#989898",
highlightColor: "#FA0404",
textColor: "#FFFFFF",
borderColor: "#353535",
cardColor: "#383838",
}
}
// Light theme object variable
const lightTheme = {
dark: false,
colors: {
backgroundColor: "#F8F8F8",
primaryColor: "#2196F3",
secondaryColor: "#989898",
highlightColor: "#EB4034",
textColor: "#353535",
borderColor: "#E1E1E1",
cardColor: "#FFFFFF",
},
}
Okay now we have set themes for dark and light mode. Lets use it with <Theme.Provider> component which has theme prop object and toggleTheme prop function. Both theme prop and toggleTheme function is available for entire application.
Example
// import Theme from here
import { Navigation, Auth, Theme } from "react-uicomp";
...
const App = () => {
const [ activeTheme, setActiveTheme ] = useState("light");
const theme = activeTheme === "light" ? lightTheme : darkTheme;
const toggleTheme = () => {
setActiveTheme(prev => prev === "light" ? darkTheme : lightTheme);
}
return (
<Navigation.Provider>
<Theme.Provider theme={theme} toggleTheme={toggleTheme}>
<Auth.Provider>
<Auth.Screens />
</Auth.Provider>
</Theme.Provider>
</Navigation.Provider>
)
};
Both theme and toggleTheme can be accessed with useTheme() hook.
Example
// import useTheme
import { useTheme } from "react-uicomp";
export default function() {
// It has theme object and toggleTheme function
const { colors, toggleTheme } = useTheme();
return () {
{/* use it like this which is changed automatically when toggleTheme function is called */}
<div style={{ color: colors.primaryColor }}>
Paragraph Text
</div>
}
}
It has Dropdown component which can be very helpful for you to create dropdown functionality easily.
Example
import { Dropdown } from "react-uicomp";
export default function() {
return() {
<Dropdown triggerElement={() => <button>Click Me</button>}>
<div>Dropdown Content</div>
</Dropdown>
}
}
props
Props | Type | Description | Default |
---|---|---|---|
children | element node | React Node which will be the dropdown content | - |
triggerElement | function | Function which should return the element which will trigger the dropdown | - |
active(optional) | boolean | Sets default state of dropdown, either it is active or not by default | false |
isAnimated(optional) | boolean | Should animate or not while toggling between dropdown | false |
animationType(optional) | "fade" | "expand" | Type of animation for dropdown | "expand" |
dropdownStyles(optional) | style | Style object to style the dropdown | - |
dropdownDirection(optional) | "left" | "right" | Defines the direction of dropdown | "right" |
dismissOnOutsideClick(optional) | boolean | Should dismiss dropdown if we click outside dropdown | true |
toggleOnTriggerElementClick | boolean | Should toggle the dropdown if we click trigger element | false |
MIT © dipeshrai123
FAQs
UI component library for react
The npm package react-uicomp receives a total of 5 weekly downloads. As such, react-uicomp popularity was classified as not popular.
We found that react-uicomp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.
Security News
Results from the 2023 State of JavaScript Survey highlight key trends, including Vite's dominance, rising TypeScript adoption, and the enduring popularity of React. Discover more insights on developer preferences and technology usage.
Security News
The US Justice Department has penalized two consulting firms $11.3 million for failing to meet cybersecurity requirements on federally funded projects, emphasizing strict enforcement to protect sensitive government data.