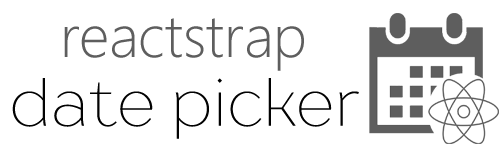

A Reactstrap based, zero dependencies, date picker.
Demo and docs at reactstrap-date-picker.
Table of Contents
- Installation
- Usage
- API Reference
- Deeper customizing
- Inspect this package
- Changelog
Installation
Using npm
:
npm install reactstrap-date-picker
reactstrap-date-picker
works with these peer dependencies:
Check Changelog for more info on other versions.
Usage
import React, {useState, useEffect} from 'react
import {FormGroup, Label, FormText} from 'reactstrap'
import {DatePicker} from 'reactstrap-date-picker'
const App = () => {
const [value, setValue]= useState(new Date().toISOString())
const [fmtValue, setFmtValue]= useState(undefined)
handleChange(value, formattedValue) {
setValue(value)
setFmtValue(formattedValue)
}
useEffect(( )=> {
console.log(`Formatted value is ${fmtValue}`)
}, [fmtValue])
return (
<FormGroup>
<Label>My Date Picker</Label>
<DatePicker id = "example-datepicker"
value = {value}
onChange= {(v,f) => handleChange(v, f)} />
<FormText>Help</FormText>
</FormGroup>
)
}
API Reference
<DatePicker />
reactstrap-date-picker
's public component.
import {DatePicker} from 'reactstrap-date-picker'
const Example = () => {
...
return (
...
<DatePicker {props} />
...
)
}
Global properties
value, defaultValue, id, name, dateFormat, minDate, maxDate, showClearButton, clearButtonElement
value
ISO date string representing the current value. Cannot be set alongside defaultValue
.
- Optional
- Type:
string
. - Example:
"2016-05-19T12:00:00.000Z"
defaultValue
ISO date string representing the default value. Cannot be set alongside value
.
- Optional
- Type:
string
- Example:
"2016-05-19T12:00:00.000Z"
id
HTML identifier for the reactstrap-date-picker
's input (the hidden one). You may
want to use it in case you need to traverse somehow the DOM.
- Optional
- Type:
string
. - Example:
"example-datepicker"
name
HTML name
attribute for the reactstrap-date-picker
's input (the hidden one). You may
need to use it depending on how your handle your Forms.
- Optional
- Type:
string
. - Example:
"date-field"
dateFormat
Date format. Any combination of DD, MM, YYYY and separator.
- Optional
- Type:
string
- Examples:
"MM/DD/YYYY"
, "YYYY/MM/DD"
, "MM-DD-YYYY"
, or "DD MM YYYY"
minDate
ISO date string to set the lowest allowable date value.
- Optional
- Type:
string
- Example:
"2016-05-19T12:00:00.000Z"
maxDate
ISO date string to set the highest allowable date value.
- Optional
- Type:
string
- Example:
"2016-05-19T12:00:00.000Z"
showClearButton
Toggles the visibility of the clearButton
- Optional
- Type:
bool
- Default:
false
clearButtonElement
Character or component to use for the clear button.
- Optional
- Type:
string
or ReactClass
- Default:
"×"
Input properties
autoComplete, autoFocus, disabled, noValidate, placeholder, required, className, style, inputRef, customControl, children
autoComplete
Hint for form autofill feature.
- Optional
- Type:
string
- Default:
on
autoFocus
Whether or not component starts with focus.
- Optional
- Type:
bool
- Default:
false
disabled
Whether or not component is disabled.
- Optional
- Type:
bool
- Default:
false
noValidate
When present, it specifies that the form-data (input) should not be validated when submitted.
- Optional
- Type:
bool
- Default:
false
placeholder
Text that appears in the form control when it has no value set.
- Optional
- Type:
text
- Example:
John Doe
required
boolean
. A value is required or must be check for the form to be submittable
- Optional
- Type:
boolean
- Default:
false
className
Class name passed to the Form Control input element.
- Optional
- Type:
string
- Example:
example-class
style
Style object passed to the Form Control input element.
- Optional
- Type:
object
- Example:
{width: "100%"}
inputRef
A React ref to the Form Control input element
customControl
Overwrite the default Form Control component with your own component.
- Optional
- Type:
React.Component
- Example:
<CustomControl />
children
children
elements from the Form Control`
- Optional
- Type:
React.Component
Input Group properties
size, valid, invalid, customInputGroup
size
Size of the input
- Optional
- Type:
string
- Examples:
lg
, sm
, ...
You can also override it completely and pass your own component:
valid
Applies the is-valid
class when true
, does nothing when false
- Optional
- Type:
bool
- Example:
true
invalid
Applies the is-invalid
class when true
, does nothing when false
- Optional
- Type:
bool
- Example:
true
customInputGroup
Overwrite the default InputGroup component with your own component.
- Optional
- Type:
React.Component
- Example:
<CustomInputGroup />
Calendar properties
dayLabels, monthLabels, weekStartsOn, showWeeks, pickMonthElement, previousButtonElement, nextButtonElement, showTodayButton, todayButtonLabel, cellPadding, roundedCorners, calendarPlacement, calendarContainer
dayLabels
Array of day names to use in the calendar. Starting on Sunday.
- Optional
- Type:
array
- Default:
['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat']
monthLabels
Array of month names to use in the calendar.
- Optional
- Type:
array
- Default:
['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']
weekStartsOn
Makes the calendar's week to start on a specified day. 0 = Sunday, 1 = Monday, etc.
- Optional
- Type:
number
- Example:
4
showWeeks
Shows the number of the week in the calendar
- Optional
- Type:
bool
- Default:
false
pickMonthElement
Optional component to use for the calendar's year and month pickers.
- Optional
- Type:
string
or ReactClass
- Default:
undefined
pickMonthElement = undefined
is the same as pickMonthElement = "none"
.
custom pickMonthElement
You can pass a custom React
component, which will receive these properties:
displayDate
minDate
maxDate
onChangeMonth
: a callback receiving an int
parameter (month number)onChangeYear
: a callback receiving an int
parameter (year number)
On the demo
you will find a simple custom element.
default
pickMonthElement
There is a predefined component, consisting of two simple select
elements,
which can be used by passing pickMonthElement = "default"
.
It has a simple styling, which may not fit your needs. Maybe you can tweak it
through the css
classes used by reactstrap-date-picker
:
<div class="rdp-header">
<div class="rdp-header-previous-wrapper"/>
<div class="rdp-header-pick-month-wrapper">
<div class="rdp-header-pick-month-default">
<div class="rdp-header-pick-month-default-month"/>
<div class="rdp-header-pick-month-default-year"/>
</div>
</div>
<div class="rdp-header-next-wrapper"/>
</div>
previousButtonElement
Character or component to use for the calendar's previous button.
- Optional
- Type:
string
or ReactClass
- Default:
"<"
nextButtonElement
Character or component to use for the calendar's next button.
- Optional
- Type:
string
or ReactClass
- Default:
">"
showTodayButton
Toggles the visibility of the today-button.
- Optional
- Type:
boolean
- Default:
false
todayButtonLabel
Label for the today-button
- Optional
- Type:
string
- Default:
"Today"
cellPadding
CSS padding value for calendar date cells.
- Optional
- Type:
string
- Default:
"5px"
roundedCorners
CSS border-radius value for calendar date cells.
- Optional
- Type:
bool
- Default:
false
calendarPlacement
Overlay placement for the popover calendar.
- Optional
- Type:
string
or function
- Default:
"top"
calendarContainer
Overlay container for the popover calendar. When placing the reactstrap-date-picker
in a scrolling container, set this prop to some ancestor of the scrolling container.
- Optional
- Type: A DOM element, a string selector or a
ref
- Example:
document.body
Event properties
onChange, onClear, onFocus, onBlur, onInvalid
onChange
Change callback function.
- Optional
- Type:
function
- Callback Arguments:
value
: ISO date string representing the selected value.
- Type:
String
- Example:
"2016-05-19T12:00:00.000Z"
formattedValue
: String representing the formatted value as defined by the dateFormat
property.
- Type:
String
- Example:
"05/19/2016"
onClear
Defines what happens when clear button is clicked.
If passed, onChange
event won't be fired when clicking clear button. This way, you will be able to customize
the input behavior, for example:
<DatePicker
onClear = {() => {
const today= new Date()
setValue(today.toISOString())
}}
/>
onFocus
Focus callback function.
- Optional
- Type:
function
- Callback Arguments:
onBlur
Blur callback function.
- Optional
- Type:
function
- Callback Arguments:
onInvalid
Defines what happens when input has not passed the form validation.
Deeper customizing
Customize styling directly trough CSS.
You can also customize reactstrap-date-picker
using CSS
, trough element's id
or class
attributes.
reactstrap-date-picker
renders several elements, all contained within a reactstrap InputGroup.
Such elements will have its unique id
attribute, plus reactstrap-date-picker
custom class
names (prefixed by rdp-*
).
The rendered DOM structure seems like this:
<div class="input-group rdp-input-group" id="rdp-input-group-SUFFIX">
<input class="form-control rdp-form-control" id="props.formControl.id or rdp-form-control-SUFFIX" />
<div class="rdp-overlay">
<div>
<div class="rdp-popover">
<div class="popover">
<div class="popover-inner">
<div class="popover-header">
<div class="rdp-header">
<div class="rdp-header-previous-wrapper"/>
<div class="rdp-header-pick-month-wrapper">
<div class="rdp-header-pick-month-default">
<div class="rdp-header-pick-month-default-month"/>
<div class="rdp-header-pick-month-default-year"/>
</div>
</div>
<div class="rdp-header-next-wrapper"/>
</div>
</div>
<div class="popover-body">
<table class="rdp-calendar">
</table>
</div>
</div>
<span class="arrow">
</div>
</div>
</div>
</div>
<input class="rdp-hidden" id="props.id or rdp-hidden-SUFFIX" />
<div class="input-group-text rdp-addon">
</div>
</div>
This SUFFIX
is:
-
props.name
-
if props.name
is not passed, then use props.id
-
if props.id
is not passed, then take a global counter of active reactstrap-date-picker
instances
So, the idea is, depending on your needs:
-
if you don't need handle id
s at all, reactstrap-date-picker
will render unique id
with no problem
-
if you need a basic id
usage, for example accessing the reactstrap-date-picker
's value from the DOM, then
you just have to pass props.id
and get the value from the element with that id
-
if you will perform more complex operations, then use props.name
or props.id
, and pay attention to the
previous DOM structure and the SUFFIX
presences
Inspect this package
Demo
npm run demo
And visit http://localhost:3010 on your browser
Running Tests
npm run test
Changelog
Originally based on react-bootstrap-date-picker,
reactstrap-date-picker
has evolved. From v1.0 it has been refactored, using React hooks,
with cleaner code and an improved final performance.
Expand
1.0.11
- fixed
calendarContainer
prop causes calendar to close unexpectedly
1.0.10
- fixed
onClear
event: if passed, onChange
is not fired
1.0.9
- fixed blur handle when navigating months
1.0.8
- fixed
inputRef
property to make it work properly when passing callback refs - keep Calendar open when clicking inside the control input
- improve bad format handling on blur
1.0.6
1.0.5
- fix valid props on hidden input
1.0.4
- fix warning on
prop-types
1.0.3
1.0.2
1.0.1
1.0.0
-
Introduction of React Hooks
-
Deep refactor of the source code
-
Supported versions:
· React >= 16.13.1
· Reactstrap >= 8.5.1
· Bootstrap >= 4.5.2
-
Improved performance
-
Smaller bundle sizes
0.0.16
· Version to use if you wanna go Reactstrap 9
· Supported versions:
- React >= 14
- Reactstrap 9.0.1
- Bootstrap 5.1.3
0.0.12
· Fixed issue #15: placeholder will not fallback to dateFormat
· Fixed issue #16. do not allow keyboard input of dates out of minDate/maxDate
· Supported versions:
- React >= 14
- Reactstrap 8.5.1
- Bootstrap 4.5.2