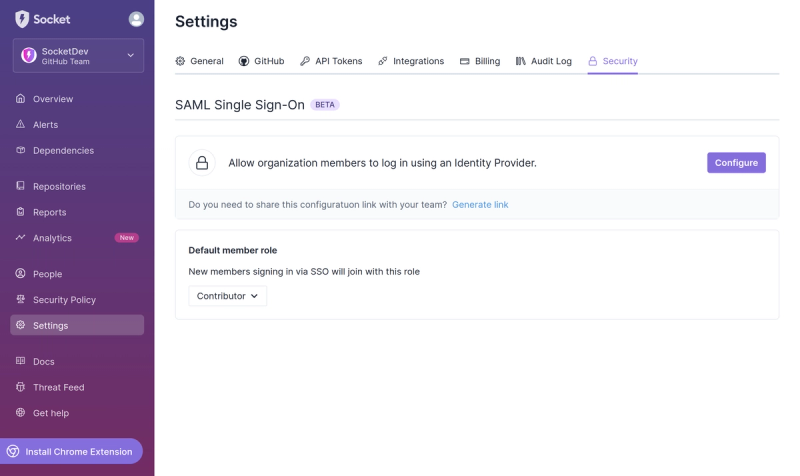
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
redux-fluent
Advanced tools
Readme
Tiny and eloquent way of bringing redux to the next level (~3K, typings included).
Try it out on RunKit (coming soon)
Redux is great, every recent web application has most likely been built on top of it, and we can really make it better!
Action
, Action Type
and Action Creator
could all be implemented in a single entity: Let's introduce Redux Fluent Actions.yarn add redux-fluent redux flux-standard-action
/** todos.actions.js **/
import { createAction } from 'redux-fluent';
export const addTodo = createAction('todos | add');
/** todos.reducer.js **/
import { createReducer, ofType } from 'redux-fluent';
import * as actions from './todos.actions.js';
const addTodo = (state, { payload }) => state.concat(payload);
export const todos = createReducer('todos')
.actions(
ofType(actions.addTodo).map(addTodo),
/* and so on */
)
.default(() => []);
/** application.js **/
import { createStore } from 'redux';
import { combineReducers } from 'redux-fluent';
import * as actions from './todos.actions.js';
import { todos } from './todos.reducer.js';
const rootReducer = combineReducers(todos, ...);
const store = createStore(rootReducer);
console.log(store.getState()); // { todos: [] }
store.dispatch(actions.addTodo({ title: 'Listen for some music' }));
console.log(store.getState()); // { todos: [{ title: 'Listen for some music' }] }
createReducer()
createReducer
is a function combinator whose purpose is to output a redux reducer by combining action handlers and getDefaultState
together.
import { createReducer } from 'redux-fluent';
const reducer = createReducer(name)
.actions(
...handlers: (state, action, config?) => state,
)
.default(
getDefaultState: (state: void, action, config?) => state,
);
createAction()
createAction
is a factory function whose purpose is to output an action creator responsible of shaping your actions. The resulting function holds a field type
giving you access to the action type.
import { createAction } from 'redux-fluent';
createAction(
type: string,
payloadCreator?: (rawPayload, rawMeta, type) => payload,
metaCreator?: (rawPayload, rawMeta, type) => meta,
);
/**
* Action Creator
*
* @param {*|Error} payload
* @param {*} meta
* @returns RFA<'todos | add', *|Error, *> - Flux Standard Action
*/
const addTodo = createAction('todos | add');
console.log(addTodo.type); // 'todos | add'
console.log(addTodo({ id: 1, title: 'have a break' })); // { type: 'todos | add', payload: { id: 1, title: 'have a break' } }
ofType()
As we just said, a reducer is nothing more than a function combinator, it does not contain any business logic. The job of actually mutating the state is left to the Action Handlers. By embracing the Single Responsibility Principle, we can build simple, easy to test, dedicated functions that do only one thing.
import { ofType } from 'redux-fluent';
ofType(action: ReduxFluentActionCreator).map((state: any, action: RFA) => state);
ofType(type: string).map((state: any, action: AnyAction) => state);
ofType(action: { type: string }).map((state: any, action: AnyAction) => state);
// write your action handlers by only focusing on the actual task,
// let `ofType` take care of the orchestration (i.e. intercepting actions).
ofType('todos | add').map(
(state, action) => state.concat(action.payload),
);
withConfig()
Redux Fluent also comes with some additional features to help you write code at scale. Functions that only rely on their arguments are easy to test and reuse, so you can provide an additional argument config: any
to enable dependency injection (multiple config
are shallowly merged).
import * as R from 'ramda';
import { withConfig, createReducer, ofType } from 'redux-fluent';
withConfig(config: any);
const todos = createReducer('todos')
.actions(
ofType('todos | doSomething').map(
(state, action, { doSomething }) => doSomething(state, action),
),
ofType('todos | doSomethingElse').map(
(state, action, { doSomethingElse }) => doSomethingElse(state, action),
),
)
.default(() => []);
export default R.pipe(
withConfig({ doSomething: R.tap(console.log) }),
withConfig({ doSomethingElse: R.tap(console.log) }),
)(todos);
combineReducers()
This api is not strictly needed, you can still use redux#combineReducers
. With Redux Fluent, by the way, both namespace and reducer are defined in the same place so that you don't need to take care of the shape when combining them.
import { createStore } from 'redux';
import { combineReducers } from 'redux-fluent';
import { todosReducer, notesReducer, otherReducer } from './reducers';
combineReducers(...reducers: ReduxFluentReducer[]);
const rootReducer = combineReducers(todosReducer, notesReducer, otherReducer, ...);
const store = createStore(rootReducer);
createReducersMapObject()
This api lets you combine your reducers with redux#combineReducers
, and can be useful when mixing redux fluent reducers with any other reducer.
import { createStore, combineReducers } from 'redux';
import { createReducersMapObject } from 'redux-fluent';
import { todosReducer, notesReducer, otherReducer } from './reducers';
createReducersMapObject(...reducers: ReduxFluentReducer[]);
const reducersMapObject = createReducersMapObject(todosReducer, notesReducer, otherReducer, ...);
const rootReducer = combineReducers({
...reducersMapObject,
anyOtherReducer: (state, action) => 'any other state',
});
const store = createStore(rootReducer);
FAQs
A Practical and Functional utility for redux
The npm package redux-fluent receives a total of 7 weekly downloads. As such, redux-fluent popularity was classified as not popular.
We found that redux-fluent demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.