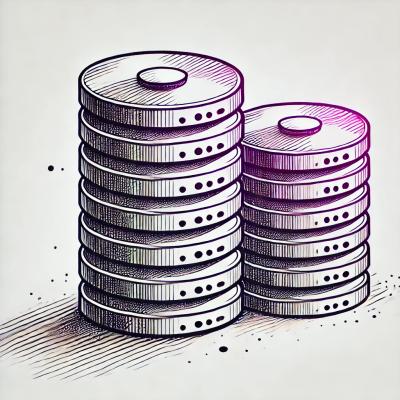
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
redux-form
Advanced tools
redux-form is a library that allows you to manage form state in Redux. It provides a way to keep form state in a Redux store, making it easier to manage complex forms and their validation, submission, and other behaviors.
Form Creation
This code demonstrates how to create a simple form using redux-form. The `Field` component is used to define form fields, and the `reduxForm` higher-order component is used to connect the form to Redux.
import React from 'react';
import { Field, reduxForm } from 'redux-form';
let SimpleForm = props => {
const { handleSubmit } = props;
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" component="input" type="text" />
</div>
<button type="submit">Submit</button>
</form>
);
};
SimpleForm = reduxForm({
form: 'simple'
})(SimpleForm);
export default SimpleForm;
Form Validation
This code demonstrates how to add validation to a form using redux-form. The `validate` function checks for errors and returns an object with error messages, which are then used by redux-form to display validation errors.
import React from 'react';
import { Field, reduxForm } from 'redux-form';
const validate = values => {
const errors = {};
if (!values.firstName) {
errors.firstName = 'Required';
}
return errors;
};
let ValidatedForm = props => {
const { handleSubmit } = props;
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" component="input" type="text" />
</div>
<button type="submit">Submit</button>
</form>
);
};
ValidatedForm = reduxForm({
form: 'validated',
validate
})(ValidatedForm);
export default ValidatedForm;
Form Submission
This code demonstrates how to handle form submission using redux-form. The `handleSubmit` function is passed a `submit` function that processes the form data.
import React from 'react';
import { Field, reduxForm } from 'redux-form';
const submit = values => {
console.log('Form data:', values);
};
let SubmitForm = props => {
const { handleSubmit } = props;
return (
<form onSubmit={handleSubmit(submit)}>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" component="input" type="text" />
</div>
<button type="submit">Submit</button>
</form>
);
};
SubmitForm = reduxForm({
form: 'submit'
})(SubmitForm);
export default SubmitForm;
Formik is a popular form library for React that provides a simpler API compared to redux-form. It focuses on reducing boilerplate and improving performance by not relying on Redux for form state management. Formik is often preferred for its ease of use and flexibility.
React Hook Form is a library that uses React hooks to manage form state and validation. It is known for its minimal re-renders and high performance. Unlike redux-form, it does not require Redux and is often chosen for its simplicity and performance benefits.
Final Form is a framework-agnostic form state management library that can be used with React through the react-final-form package. It offers similar functionality to redux-form but is designed to be more lightweight and flexible, without the need for Redux.
redux-form
works with React Redux to enable an html form in
React to use Redux to store all of its state.
npm install --save redux-form
FAQs
A higher order component decorator for forms using Redux and React
The npm package redux-form receives a total of 271,473 weekly downloads. As such, redux-form popularity was classified as popular.
We found that redux-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.