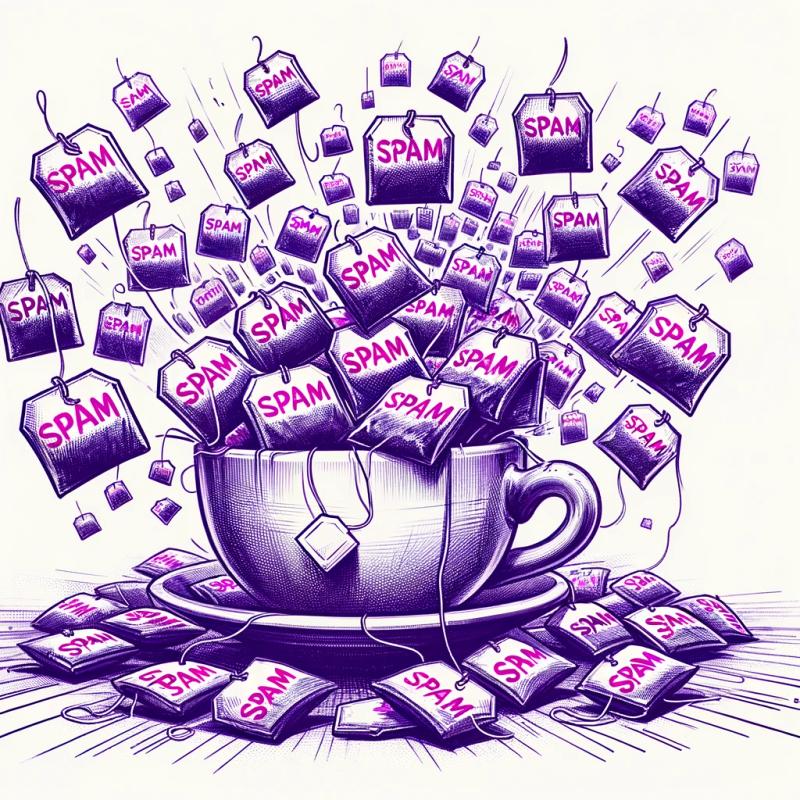
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
redux-websocket-bridge
Advanced tools
Changelog
0.0.1-0 - 2017-12-29
Readme
Inspired by redux-websocket.
This bridge middleware will:
@@websocket/MESSAGE
, payload as-isimport { applyMiddleware, createStore } from 'redux';
import WebSocketActionBridge from 'redux-websocket-bridge';
const createStoreWithMiddleware = applyMiddleware(
WebSocketActionBridge('ws://localhost:4000/', { unfold: true })
)(createStore);
export default createStore(...);
Tips: you can add more than one
WebSocketBridge
to your store by namespacing them withactionPrefix
.
Because we support multiple bridges in a single store, namespace prefix is added to action type, by default, @@websocket/
.
import { OPEN, CLOSE, MESSAGE } from 'redux-websocket-bridge';
function serverConnectivity(state = {}, action) {
switch (action.type) {
case `@@websocket/${ OPEN }`:
state = { ...state, connected: true };
break;
case `@@websocket/${ CLOSE }`:
state = { ...state, connected: false };
break;
case `@@websocket/${ MESSAGE }`:
// Process the raw message here, either string, ArrayBuffer, or Blob
break;
default: break;
}
return state;
}
When an event is received thru the WebSocket, it will be dispatched into the store as one of the actions below:
// When the socket is connected
{
"type": "@@websocket/OPEN"
}
// When the socket is disconnected
{
"type": "@@websocket/CLOSE"
}
// When the socket received a message, the payload is a string
{
"type": "@@websocket/MESSAGE",
"payload": "<string, ArrayBuffer, or Blob>"
}
To send a message, you dispatch an action with the payload and set type
to @@websocket/SEND
. You can only send payload your WebSocket implementation support, e.g. string
, ArrayBuffer
, and Blob
.
import { SEND } from 'redux-websocket-action-bridge';
function fetchServerVersion() {
return { type: `@@websocket/${ SEND }`, payload: '<Raw message here>' };
}
Instead of receiving WebSocket messages as a generic @@websocket/MESSAGE
action, the bridge can automatically unfold the payload if it is a JSON and looks like a Flux Standard Action. For example, the following WebSocket message will be unfolded:
"{\"type\":\"SERVER/ALIVE\",\"payload\":{\"version\":\"1.0.0\"}}"
It will be unfolded into an action:
{
type: 'SERVER/ALIVE',
payload: { version: '1.0.0' }
}
By unfolding Redux actions, you can reduce code and create interesting code pattern. For example, server can send greeting action on connect. The WebSocket message will be directly dispatched into Redux store.
ws.on('connection', socket => {
socket.send(JSON.stringify({
type: 'SERVER/VERSION',
payload: {
version: require('./package.json').version
}
}));
});
Unfold also applies when you are sending out a JavaScript object that looks like an action.
this.props.dispatch({
type: '@@websocket/SEND',
payload: {
type: 'CLIENT/SIGN_IN',
payload: { token: 'my very secret token' }
}
}));
What-if: if you are sending a JavaScript object and it does not pass the FSA test, it will be passed to
WebSocket.send()
as-is without modifications.
In addition to sending over WebSocket, the action will also dispatch locally.
{
type: 'CLIENT/SIGN_IN',
payload: { token: 'my very secret token' }
}
The bridge supports any libraries that adheres to WebSocket standard, for example, SockJS, which provides fallback for browser/proxy that does not support WebSocket.
const createStoreWithMiddleware = applyMiddleware(
WebSocketBridge(
() => new SockJS('http://localhost:3000/ws/')
)
)(createStore);
If you are unsure if your WebSocket implementation will work on the bridge or not, check it against the list of required WebSocket APIs list below:
onopen
onclose
onmessage(event)
event.data
string
send
string
ArrayBuffer
WebSocket standard support sending binary data. But receiving binary data means you might be receiving either ArrayBuffer
or Blob
, depends on your WebSocket implementation.
For your convenience, if you set binaryType
to arrayBuffer
, we will convert all Blob
into ArrayBuffer
before dispatching it to Redux store.
Vice versa, if you set binaryType
to blob
, we will convert all ArrayBuffer
into Blob
.
Name | Description | Default |
---|---|---|
actionPrefix | Action prefix for all system messages | "@@websocket/" |
binaryType | Convert binary to "arrayBuffer" or "blob" | null |
unfold | Unfold messages as actions if they are JSON and look like a Flux Standard Action, and vice versa | false |
Like us? Star us.
Something not right? File us an issue.
FAQs
WebSocket messages to Redux action bridge
The npm package redux-websocket-bridge receives a total of 258 weekly downloads. As such, redux-websocket-bridge popularity was classified as not popular.
We found that redux-websocket-bridge demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.