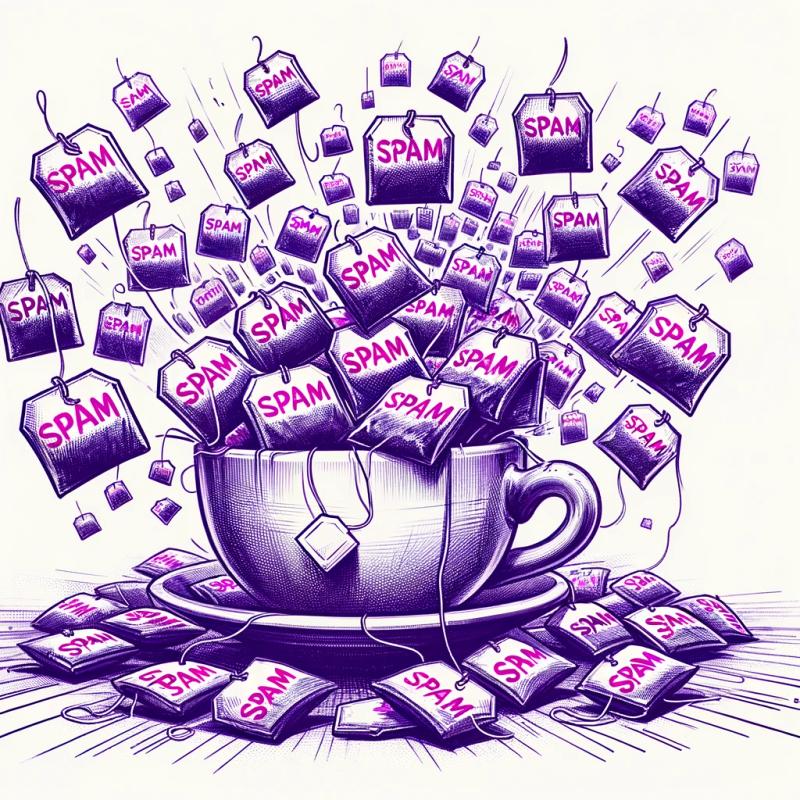
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
rehype-link-processor
Advanced tools
Changelog
1.0.4 Patch release
feat: new 'email' builtin rule fix: force download rule to match against http* protocol
Readme
rehype plugin to process links to
rel
or target
This package helps to decorate links usually in a markdown document where no extra attribute can be set.
Common scenarios:
When you write links in markdown, you're limited with just the url, text and title. You cannot add custom attributes, for example a css class to style that specific link as you want.
This package helps to process and transform links.
This is package is module. So an ESM compatible runtime is required (node 14+, deno, ...)
npm i rehype-link-processor
# or
pnpm add rehype-link-processor
# or
yarn add rehype-link-processor
unified
pipelineInclude rehype-link-processor in the pipeline:
import { unified } from "unified";
import remarkParse from "remark-parse";
import remarkRehype from "remark-rehype";
import rehypeLinkProcessor from "rehype-link-processor";
const file = await unified()
.use(remarkParse)
.use(remarkRehype)
.use(rehypeLinkProcessor)
.process("...")
mdx
compilationInclude rehype-link-processor in the rehype plugins:
import {compile} from "@mdx-js/mdx";
import rehypeLinkProcessor from "rehype-link-processor";
const file = '[download:Get the pdf](/assets/article.pdf)';
await compile(file, { rehypePlugins: [rehypeLinkProcessor] });
Include rehype-link-processor as markdown rehype plugin in the astro.config.ts
:
//file: astro.config.ts
import { defineConfig } from "astro/config";
import rehypeLinkProcessor from "rehype-link-processor";
export default defineConfig({
markdown: {
rehypePlugins: [rehypeLinkProcessor()]
}
);
The processor works with rules. If a rule matches, the action is applied (the link is transformed), and the processing ends.
So the rule order is important, as the first match wins.
The rules are set via the options argument:
rehypeLinkProcessor({
rules: [
// add rules here
]
})
This package provides some builtin rules covering common scenarios. You can also add custom rules to fit you needs.
There are three rule types:
object
function
All builtin rules are enabled by default.
Builtin rules can be disabled with useBuiltin
set to false
.
rehypeLinkProcessor({
useBuiltin: false
})
When you disable builtin rules, you can add the ones you like manually:
rehypeLinkProcessor({
rules: [
"external" // <-- enable the external link rule
]
})
or your use the helper R
when you need a configuration:
rehypeLinkProcessor({
rules: [
R.download({
skipExtension: ["html"] // exclude html to be considered a download
})
]
})
The builtin rules are:
external
looks for external links matching when one of:
http:
or https
:external:
external:
if matched, the resulting a
will have the attributes:
class
= "external"target
= "_blank"rel
= "nofollow noopener"Markdown:
[Github](https://github.com)
HTML:
<a href="https://github.com" class="external" target="_blank" rel="nofollow noopener">Github<a>
Markdown:
[external:Discussion on Github](/discussion)
HTML:
<a href="/discussion" class="external" target="_blank" rel="nofollow noopener">Discussion on Github</a>
download
looks for download links matching when one of:
http:
or https:
and the path ends with .<ext>
where ext
is [1-4] chars long (excluded: html
, htm
, md
, mdx
)download:
download:
if matched, the resulting a
will have the attributes:
class
= "download"download
= the filename extracted or true
when detected by the prefixMarkdown:
[Download the pdf](/assets/my-article.pdf)
HTML:
<a download="my-article.pd" href="/assets/my-article.pdf" class="download">Download the pdf<a>
Markdown:
[download:Get the Archive](/directory?format=zip)
HTML:
<a download href="/directory?format=zip" class="download">Get the Archive</a>
email
looks for email links matching when one of:
mailto:
email:
email:
if matched, the resulting a
will have the attributes:
class
= "email"Markdown:
[Contact us](support@domain.com)
HTML:
<a href="mailto:support@domain.com" class="email">Contact us<a>
Markdown:
[email:Send us a mail](info@domain.com)
HTML:
<a href="mailto:info@domain.com" class="email">Send us a mail<a>
same-page
detect navigation within the same page, aka fragment navigation
#
the resulting a
will have the attributes
class
= "same-page"Markdown:
[Chapter 2](#chapter-2)
HTML:
<a href="#chapter-2" class="same-page">Chapter 2<a>
A match rule, works in two steps:
rules: [
{
match: link => link.href.startWith("mailto:"),
action: { className: "email" }
}
]
If the match
returns a falsy value (false
, undefined
, ...). The rule is skipped.
You can specify multiple actions in an Array.
You can use the A
helper witch provides common actions.
// rule to correct GiThuB link casing
rules: [
{
match: link => link.text?.toLowerCase() === "github"
action: [
// add the class: the link can have preexisting class
A.mergeClass("brand-link"),
// another syntax for { text: "GitHub" }
A.set("text", "GitHub")
]
}
]
The match function can also return an object. It will be assigned over the link, overwriting the common fields.
It's useful in a scenario where you want to apply a transformation right away in the matching step.
rules: [
{
match: link => {
if(link.href.startsWith("http:")){
return { href: link.href.replace("http:", "https:") };
}
},
action: [
A.set("target", "_blank"),
]
}
]
With a transform rule you can analyze any like directly. The transform rule provides a function
based syntax to process links.
With the link as input, a transform rule can return:
You can add a transform rule like any other rule:
{
rules: [
link => {
if (link.href?.includes("github.com")) {
return { title: "GitHub: Where this code lives" };
}
}
]
}
This package is built in typescript so it has full typings support.
FAQs
rehype plugin to process links adding custom css class or attributes used by external or download links
The npm package rehype-link-processor receives a total of 4 weekly downloads. As such, rehype-link-processor popularity was classified as not popular.
We found that rehype-link-processor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.