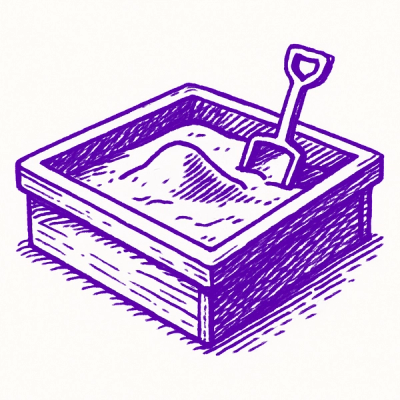
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
remix-auth-oauth2
Advanced tools
A strategy to use and implement OAuth2 framework for authentication with federated services like Google, Facebook, GitHub, etc.
A strategy to use and implement OAuth2 framework for authentication with federated services like Google, Facebook, GitHub, etc.
[!WARNING] This strategy expects the identity provider to strictly follow the OAuth2 specification. If the provider does not follow the specification and diverges from it, this strategy may not work as expected.
Runtime | Has Support |
---|---|
Node.js | ✅ |
Cloudflare | ✅ |
npm add remix-auth-oauth2
You can use this strategy by adding it to your authenticator instance and configuring the correct endpoints.
import { OAuth2Strategy, CodeChallengeMethod } from "remix-auth-oauth2";
export const authenticator = new Authenticator<User>();
authenticator.use(
new OAuth2Strategy(
{
cookie: "oauth2", // Optional, can also be an object with more options
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
authorizationEndpoint: "https://provider.com/oauth2/authorize",
tokenEndpoint: "https://provider.com/oauth2/token",
redirectURI: "https://example.app/auth/callback",
tokenRevocationEndpoint: "https://provider.com/oauth2/revoke", // optional
scopes: ["openid", "email", "profile"], // optional
codeChallengeMethod: CodeChallengeMethod.S256, // optional
},
async ({ tokens, request }) => {
// here you can use the params above to get the user and return it
// what you do inside this and how you find the user is up to you
return await getUser(tokens, request);
}
),
// this is optional, but if you setup more than one OAuth2 instance you will
// need to set a custom name to each one
"provider-name"
);
Then you will need to setup your routes, for the OAuth2 flows you will need to call the authenticate
method twice.
First, you will call the authenticate
method with the provider name you set in the authenticator.
export async function action({ request }: Route.ActionArgs) {
await authenticator.authenticate("provider-name", { request });
}
[!NOTE] This route can be an
action
or aloader
, it depends if you trigger the flow doing a POST or GET request.
This will start the OAuth2 flow and redirect the user to the provider's login page. Once the user logs in and authorizes your application, the provider will redirect the user back to your application redirect URI.
You will now need a route on that URI to handle the callback from the provider.
export async function loader({ request }: Route.LoaderArgs) {
let user = await authenticator.authenticate("provider-name", request);
// now you have the user object with the data you returned in the verify function
}
[!NOTE] This route must be a
loader
as the redirect will trigger aGET
request.
Once you have the user
object returned by your strategy verify function, you can do whatever you want with that information. This can be storing the user in a session, creating a new user in your database, link the account to an existing user in your database, etc.
The strategy exposes a public refreshToken
method that you can use to refresh the access token.
let strategy = new OAuth2Strategy<User>(options, verify);
let tokens = await strategy.refreshToken(refreshToken);
The refresh token is part of the tokens
object the verify function receives. How you store it to call strategy.refreshToken
and what you do with the tokens
object after it is up to you.
The most common approach would be to store the refresh token in the user data and then update the session after refreshing the token.
authenticator.use(
new OAuth2Strategy<User>(
options,
async ({ tokens, request }) => {
let user = await getUser(tokens, request);
return {
...user,
accessToken: tokens.accessToken()
refreshToken: tokens.hasRefreshToken() ? tokens.refreshToken() : null,
}
}
)
);
// later in your code you can use it to get new tokens object
let tokens = await strategy.refreshToken(user.refreshToken);
You can revoke the access token the user has with the provider.
await strategy.revokeToken(user.accessToken);
If you want to discover the provider's endpoints, you can use the discover
static method.
export let authenticator = new Authenticator<User>();
authenticator.use(
await OAuth2Strategy.discover<User>(
"https://provider.com",
{
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
redirectURI: "https://example.app/auth/callback",
scopes: ["openid", "email", "profile"], // optional
},
async ({ tokens, request }) => {
// here you can use the params above to get the user and return it
// what you do inside this and how you find the user is up to you
return await getUser(tokens, request);
}
)
);
This will fetch the provider's configuration endpoint (/.well-known/openid-configuration
) and grab the authorization, token and revocation endpoints from it, it will also grab the code challenge method supported and try to use S256 if it is supported.
Remember this will do a fetch when then strategy is created, this will add a latency to the startup of your application.
It's recommended to use this method only once and then copy the endpoints to your configuration.
You can customize the cookie options by passing an object to the cookie
option.
authenticator.use(
new OAuth2Strategy<User>(
{
cookie: {
name: "oauth2",
maxAge: 60 * 60 * 24 * 7, // 1 week
path: "/auth",
httpOnly: true,
sameSite: "lax",
secure: process.env.NODE_ENV === "production",
},
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
authorizationEndpoint: "https://provider.com/oauth2/authorize",
tokenEndpoint: "https://provider.com/oauth2/token",
redirectURI: "https://example.app/auth/callback",
},
async ({ tokens, request }) => {
return await getUser(tokens, request);
}
)
);
This will set the cookie with the name oauth2
, with a max age of 1 week, only accessible on the /auth
path, http only, same site lax and secure if the application is running in production.
FAQs
A strategy to use and implement OAuth2 framework for authentication with federated services like Google, Facebook, GitHub, etc.
The npm package remix-auth-oauth2 receives a total of 35,914 weekly downloads. As such, remix-auth-oauth2 popularity was classified as popular.
We found that remix-auth-oauth2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.