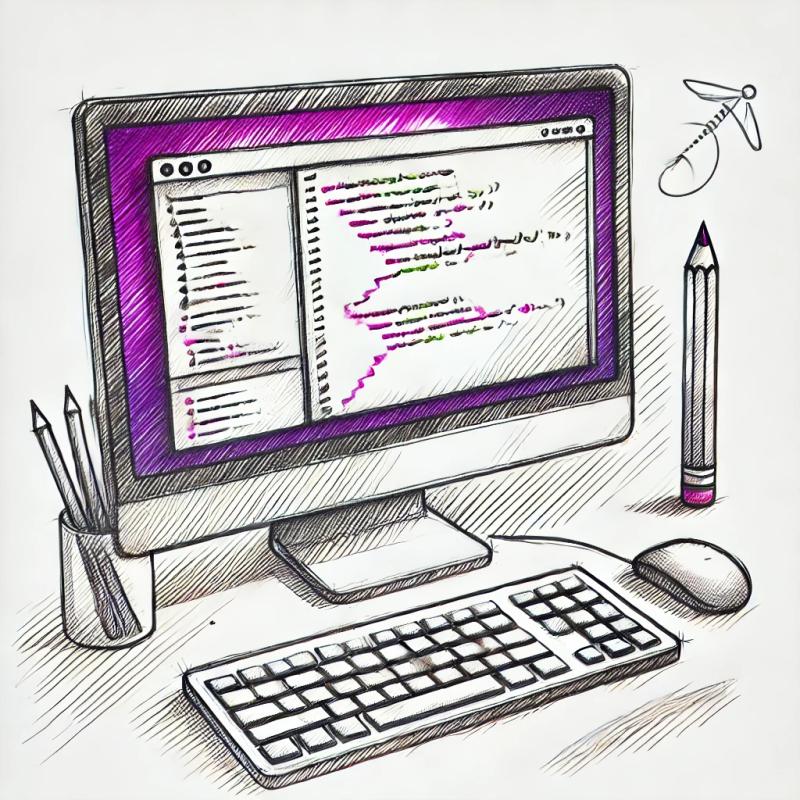
Security News
New axobject-query Maintainer Faces Backlash Over Controversial Decision to Support Legacy Node.js Versions
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.
request
Advanced tools
Package description
The 'request' npm package is a simple, yet powerful HTTP client that supports multiple features such as making HTTP calls, handling responses, streaming data, and more. It is designed to be the simplest way possible to make http calls and supports HTTPS and follows redirects by default.
Simple HTTP GET requests
This code performs a simple HTTP GET request to Google's homepage and logs the error, response status code, and the response body.
const request = require('request');
request('http://www.google.com', function (error, response, body) {
console.log('error:', error);
console.log('statusCode:', response && response.statusCode);
console.log('body:', body);
});
Streaming data
This code demonstrates how to stream data from an HTTP request directly to a file, which can be useful for downloading files or handling large amounts of data.
const request = require('request');
const fs = require('fs');
const stream = fs.createWriteStream('file.txt');
request('http://www.google.com').pipe(stream);
Custom HTTP headers
This code shows how to send a custom HTTP header (in this case, the User-Agent header) with a request. This is often required when using certain APIs, like GitHub's.
const request = require('request');
const options = {
url: 'https://api.github.com/repos/request/request',
headers: {
'User-Agent': 'request'
}
};
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
const info = JSON.parse(body);
console.log(info);
}
}
request(options, callback);
Handling POST requests
This code snippet demonstrates how to send a POST request with form data, including how to upload a file as part of that form data.
const request = require('request');
const options = {
method: 'POST',
url: 'http://service.com/upload',
headers: {
'Content-Type': 'multipart/form-data'
},
formData: {
key: 'value',
file: fs.createReadStream('file.txt')
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and is often used as an alternative to 'request' due to its promise support and interceptors for request/response manipulation.
Got is a human-friendly and powerful HTTP request library. It is designed to be a simpler and more performant alternative to 'request', with features like streams support, promise API, and better error handling.
Node-fetch is a light-weight module that brings the Fetch API to Node.js. It is an alternative to 'request' that provides a simpler, promise-based API for making HTTP requests, similar to what is available in modern web browsers.
Superagent is a small progressive client-side HTTP request library, and Node.js module with the same API, sporting many high-level HTTP client features. It compares to 'request' by offering a fluent API and being lightweight.
Readme
npm install request
Request is designed to be the simplest way possible to make http calls. It support HTTPS and follows redirects by default.
The first argument is an options object. The only required option is uri, all others are optional.
'uri'
- fully qualified uri or a parsed url object from url.parse()'method'
- http method, defaults to GET'headers'
- http headers, defaults to {}'body'
- entity body for POST and PUT requests'client'
- existing http client object (when undefined a new one will be created and assigned to this property so you can keep around a reference to it if you would like use keep-alive on later request)followRedirect
- follow HTTP 3xx responses as redirects. defaults to true.requestBodyStream
- Stream to read request body chunks from.responseBodyStream
- Stream to write body chunks to. When set this option will be passed as the last argument to the callback instead of the entire body.The callback argument gets 3 arguments. The first is an error when applicable (usually from the http.Client option not the http.ClientRequest object). The second in an http.ClientResponse object. The third is the response body buffer.
Example:
var request = require('request'); request({uri:'http://www.google.com'}, function (error, response, body) { if (!error && response.statusCode == 200) { sys.puts(body) // Print the google web page. } })
It's also worth noting that the options argument will mutate. When following a redirect the uri values will change. After setting up a client options it will set the client property.
FAQs
Simplified HTTP request client.
The npm package request receives a total of 10,536,300 weekly downloads. As such, request popularity was classified as popular.
We found that request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.
Security News
Results from the 2023 State of JavaScript Survey highlight key trends, including Vite's dominance, rising TypeScript adoption, and the enduring popularity of React. Discover more insights on developer preferences and technology usage.
Security News
The US Justice Department has penalized two consulting firms $11.3 million for failing to meet cybersecurity requirements on federally funded projects, emphasizing strict enforcement to protect sensitive government data.