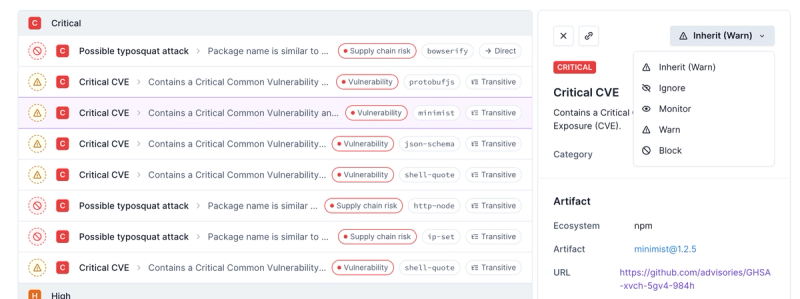
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
request
Advanced tools
Package description
The 'request' npm package is a simple, yet powerful HTTP client that supports multiple features such as making HTTP calls, handling responses, streaming data, and more. It is designed to be the simplest way possible to make http calls and supports HTTPS and follows redirects by default.
Simple HTTP GET requests
This code performs a simple HTTP GET request to Google's homepage and logs the error, response status code, and the response body.
const request = require('request');
request('http://www.google.com', function (error, response, body) {
console.log('error:', error);
console.log('statusCode:', response && response.statusCode);
console.log('body:', body);
});
Streaming data
This code demonstrates how to stream data from an HTTP request directly to a file, which can be useful for downloading files or handling large amounts of data.
const request = require('request');
const fs = require('fs');
const stream = fs.createWriteStream('file.txt');
request('http://www.google.com').pipe(stream);
Custom HTTP headers
This code shows how to send a custom HTTP header (in this case, the User-Agent header) with a request. This is often required when using certain APIs, like GitHub's.
const request = require('request');
const options = {
url: 'https://api.github.com/repos/request/request',
headers: {
'User-Agent': 'request'
}
};
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
const info = JSON.parse(body);
console.log(info);
}
}
request(options, callback);
Handling POST requests
This code snippet demonstrates how to send a POST request with form data, including how to upload a file as part of that form data.
const request = require('request');
const options = {
method: 'POST',
url: 'http://service.com/upload',
headers: {
'Content-Type': 'multipart/form-data'
},
formData: {
key: 'value',
file: fs.createReadStream('file.txt')
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and is often used as an alternative to 'request' due to its promise support and interceptors for request/response manipulation.
Got is a human-friendly and powerful HTTP request library. It is designed to be a simpler and more performant alternative to 'request', with features like streams support, promise API, and better error handling.
Node-fetch is a light-weight module that brings the Fetch API to Node.js. It is an alternative to 'request' that provides a simpler, promise-based API for making HTTP requests, similar to what is available in modern web browsers.
Superagent is a small progressive client-side HTTP request library, and Node.js module with the same API, sporting many high-level HTTP client features. It compares to 'request' by offering a fluent API and being lightweight.
FAQs
Simplified HTTP request client.
The npm package request receives a total of 11,009,507 weekly downloads. As such, request popularity was classified as popular.
We found that request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.