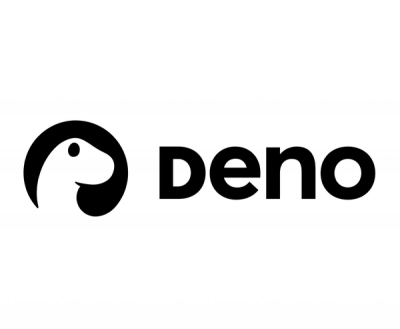
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The 'request' npm package is a simple, yet powerful HTTP client that supports multiple features such as making HTTP calls, handling responses, streaming data, and more. It is designed to be the simplest way possible to make http calls and supports HTTPS and follows redirects by default.
Simple HTTP GET requests
This code performs a simple HTTP GET request to Google's homepage and logs the error, response status code, and the response body.
const request = require('request');
request('http://www.google.com', function (error, response, body) {
console.log('error:', error);
console.log('statusCode:', response && response.statusCode);
console.log('body:', body);
});
Streaming data
This code demonstrates how to stream data from an HTTP request directly to a file, which can be useful for downloading files or handling large amounts of data.
const request = require('request');
const fs = require('fs');
const stream = fs.createWriteStream('file.txt');
request('http://www.google.com').pipe(stream);
Custom HTTP headers
This code shows how to send a custom HTTP header (in this case, the User-Agent header) with a request. This is often required when using certain APIs, like GitHub's.
const request = require('request');
const options = {
url: 'https://api.github.com/repos/request/request',
headers: {
'User-Agent': 'request'
}
};
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
const info = JSON.parse(body);
console.log(info);
}
}
request(options, callback);
Handling POST requests
This code snippet demonstrates how to send a POST request with form data, including how to upload a file as part of that form data.
const request = require('request');
const options = {
method: 'POST',
url: 'http://service.com/upload',
headers: {
'Content-Type': 'multipart/form-data'
},
formData: {
key: 'value',
file: fs.createReadStream('file.txt')
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and is often used as an alternative to 'request' due to its promise support and interceptors for request/response manipulation.
Got is a human-friendly and powerful HTTP request library. It is designed to be a simpler and more performant alternative to 'request', with features like streams support, promise API, and better error handling.
Node-fetch is a light-weight module that brings the Fetch API to Node.js. It is an alternative to 'request' that provides a simpler, promise-based API for making HTTP requests, similar to what is available in modern web browsers.
Superagent is a small progressive client-side HTTP request library, and Node.js module with the same API, sporting many high-level HTTP client features. It compares to 'request' by offering a fluent API and being lightweight.
Request is designed to be the simplest way possible to make http calls. It supports HTTPS and follows redirects by default.
var request = require('request');
request('http://www.google.com', function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body) // Print the google web page.
}
})
You can stream any response to a file stream.
request('http://google.com/doodle.png').pipe(fs.createWriteStream('doodle.png'))
You can also stream a file to a PUT or POST request. This method will also check the file extension against a mapping of file extensions to content-types (in this case application/json
) and use the proper content-type
in the PUT request (if the headers don’t already provide one).
fs.createReadStream('file.json').pipe(request.put('http://mysite.com/obj.json'))
Request can also pipe
to itself. When doing so, content-type
and content-length
are preserved in the PUT headers.
request.get('http://google.com/img.png').pipe(request.put('http://mysite.com/img.png'))
Now let’s get fancy.
http.createServer(function (req, resp) {
if (req.url === '/doodle.png') {
if (req.method === 'PUT') {
req.pipe(request.put('http://mysite.com/doodle.png'))
} else if (req.method === 'GET' || req.method === 'HEAD') {
request.get('http://mysite.com/doodle.png').pipe(resp)
}
}
})
You can also pipe()
from http.ServerRequest
instances, as well as to http.ServerResponse
instances. The HTTP method, headers, and entity-body data will be sent. Which means that, if you don't really care about security, you can do:
http.createServer(function (req, resp) {
if (req.url === '/doodle.png') {
var x = request('http://mysite.com/doodle.png')
req.pipe(x)
x.pipe(resp)
}
})
And since pipe()
returns the destination stream in ≥ Node 0.5.x you can do one line proxying. :)
req.pipe(request('http://mysite.com/doodle.png')).pipe(resp)
Also, none of this new functionality conflicts with requests previous features, it just expands them.
var r = request.defaults({'proxy':'http://localproxy.com'})
http.createServer(function (req, resp) {
if (req.url === '/doodle.png') {
r.get('http://google.com/doodle.png').pipe(resp)
}
})
You can still use intermediate proxies, the requests will still follow HTTP forwards, etc.
request
supports application/x-www-form-urlencoded
and multipart/form-data
form uploads. For multipart/related
refer to the multipart
API.
URL-encoded forms are simple.
request.post('http://service.com/upload', {form:{key:'value'}})
// or
request.post('http://service.com/upload').form({key:'value'})
For multipart/form-data
we use the form-data library by @felixge. You don’t need to worry about piping the form object or setting the headers, request
will handle that for you.
var r = request.post('http://service.com/upload')
var form = r.form()
form.append('my_field', 'my_value')
form.append('my_buffer', new Buffer([1, 2, 3]))
form.append('my_file', fs.createReadStream(path.join(__dirname, 'doodle.png'))
form.append('remote_file', request('http://google.com/doodle.png'))
request.get('http://some.server.com/').auth('username', 'password', false);
// or
request.get('http://some.server.com/', {
'auth': {
'user': 'username',
'pass': 'password',
'sendImmediately': false
}
});
If passed as an option, auth
should be a hash containing values user
|| username
, password
|| pass
, and sendImmediately
(optional). The method form takes parameters auth(username, password, sendImmediately)
.
sendImmediately
defaults to true
, which causes a basic authentication header to be sent. If sendImmediately
is false
, then request
will retry with a proper authentication header after receiving a 401
response from the server (which must contain a WWW-Authenticate
header indicating the required authentication method).
Digest authentication is supported, but it only works with sendImmediately
set to false
; otherwise request
will send basic authentication on the initial request, which will probably cause the request to fail.
// Twitter OAuth
var qs = require('querystring')
, oauth =
{ callback: 'http://mysite.com/callback/'
, consumer_key: CONSUMER_KEY
, consumer_secret: CONSUMER_SECRET
}
, url = 'https://api.twitter.com/oauth/request_token'
;
request.post({url:url, oauth:oauth}, function (e, r, body) {
// Ideally, you would take the body in the response
// and construct a URL that a user clicks on (like a sign in button).
// The verifier is only available in the response after a user has
// verified with twitter that they are authorizing your app.
var access_token = qs.parse(body)
, oauth =
{ consumer_key: CONSUMER_KEY
, consumer_secret: CONSUMER_SECRET
, token: access_token.oauth_token
, verifier: access_token.oauth_verifier
}
, url = 'https://api.twitter.com/oauth/access_token'
;
request.post({url:url, oauth:oauth}, function (e, r, body) {
var perm_token = qs.parse(body)
, oauth =
{ consumer_key: CONSUMER_KEY
, consumer_secret: CONSUMER_SECRET
, token: perm_token.oauth_token
, token_secret: perm_token.oauth_token_secret
}
, url = 'https://api.twitter.com/1/users/show.json?'
, params =
{ screen_name: perm_token.screen_name
, user_id: perm_token.user_id
}
;
url += qs.stringify(params)
request.get({url:url, oauth:oauth, json:true}, function (e, r, user) {
console.log(user)
})
})
})
HTTP Headers, such as User-Agent
, can be set in the options
object.
In the example below, we call the github API to find out the number
of stars and forks for the request repository. This requires a
custom User-Agent
header as well as https.
var request = require('request');
var options = {
url: 'https://api.github.com/repos/mikeal/request',
headers: {
'User-Agent': 'request'
}
};
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
var info = JSON.parse(body);
console.log(info.stargazers_count + " Stars");
console.log(info.forks_count + " Forks");
}
}
request(options, callback);
The first argument can be either a url
or an options
object. The only required option is uri
; all others are optional.
uri
|| url
- fully qualified uri or a parsed url object from url.parse()
qs
- object containing querystring values to be appended to the uri
method
- http method (default: "GET"
)headers
- http headers (default: {}
)body
- entity body for PATCH, POST and PUT requests. Must be a Buffer
or String
.form
- when passed an object, this sets body
to a querystring representation of value, and adds Content-type: application/x-www-form-urlencoded; charset=utf-8
header. When passed no options, a FormData
instance is returned (and is piped to request).auth
- A hash containing values user
|| username
, password
|| pass
, and sendImmediately
(optional). See documentation above.json
- sets body
but to JSON representation of value and adds Content-type: application/json
header. Additionally, parses the response body as JSON.multipart
- (experimental) array of objects which contains their own headers and body
attribute. Sends multipart/related
request. See example below.followRedirect
- follow HTTP 3xx responses as redirects (default: true
)followAllRedirects
- follow non-GET HTTP 3xx responses as redirects (default: false
)maxRedirects
- the maximum number of redirects to follow (default: 10
)encoding
- Encoding to be used on setEncoding
of response data. If null
, the body
is returned as a Buffer
.pool
- A hash object containing the agents for these requests. If omitted, the request will use the global pool (which is set to node's default maxSockets
)pool.maxSockets
- Integer containing the maximum amount of sockets in the pool.timeout
- Integer containing the number of milliseconds to wait for a request to respond before aborting the requestproxy
- An HTTP proxy to be used. Supports proxy Auth with Basic Auth, identical to support for the url
parameter (by embedding the auth info in the uri
)oauth
- Options for OAuth HMAC-SHA1 signing. See documentation above.hawk
- Options for Hawk signing. The credentials
key must contain the necessary signing info, see hawk docs for details.strictSSL
- If true
, requires SSL certificates be valid. Note: to use your own certificate authority, you need to specify an agent that was created with that CA as an option.jar
- If true
, remember cookies for future use (or define your custom cookie jar; see examples section)aws
- object
containing AWS signing information. Should have the properties key
, secret
. Also requires the property bucket
, unless you’re specifying your bucket
as part of the path, or the request doesn’t use a bucket (i.e. GET Services)httpSignature
- Options for the HTTP Signature Scheme using Joyent's library. The keyId
and key
properties must be specified. See the docs for other options.localAddress
- Local interface to bind for network connections.The callback argument gets 3 arguments:
error
when applicable (usually from the http.Client
option, not the http.ClientRequest
object)http.ClientResponse
objectresponse
body (String
or Buffer
)There are also shorthand methods for different HTTP METHODs and some other conveniences.
This method returns a wrapper around the normal request API that defaults to whatever options you pass in to it.
Same as request()
, but defaults to method: "PUT"
.
request.put(url)
Same as request()
, but defaults to method: "PATCH"
.
request.patch(url)
Same as request()
, but defaults to method: "POST"
.
request.post(url)
Same as request() but defaults to method: "HEAD"
.
request.head(url)
Same as request()
, but defaults to method: "DELETE"
.
request.del(url)
Same as request()
(for uniformity).
request.get(url)
Function that creates a new cookie.
request.cookie('cookie_string_here')
Function that creates a new cookie jar.
request.jar()
var request = require('request')
, rand = Math.floor(Math.random()*100000000).toString()
;
request(
{ method: 'PUT'
, uri: 'http://mikeal.iriscouch.com/testjs/' + rand
, multipart:
[ { 'content-type': 'application/json'
, body: JSON.stringify({foo: 'bar', _attachments: {'message.txt': {follows: true, length: 18, 'content_type': 'text/plain' }}})
}
, { body: 'I am an attachment' }
]
}
, function (error, response, body) {
if(response.statusCode == 201){
console.log('document saved as: http://mikeal.iriscouch.com/testjs/'+ rand)
} else {
console.log('error: '+ response.statusCode)
console.log(body)
}
}
)
Cookies are disabled by default (else, they would be used in subsequent requests). To enable cookies, set jar
to true
(either in defaults
or options
).
var request = request.defaults({jar: true})
request('http://www.google.com', function () {
request('http://images.google.com')
})
To use a custom cookie jar (instead request
’s global cookie jar), set jar
to an instance of request.jar()
(either in defaults
or options
)
var j = request.jar()
var request = request.defaults({jar:j})
request('http://www.google.com', function () {
request('http://images.google.com')
})
OR
var j = request.jar()
var cookie = request.cookie('your_cookie_here')
j.add(cookie)
request({url: 'http://www.google.com', jar: j}, function () {
request('http://images.google.com')
})
FAQs
Simplified HTTP request client.
We found that request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.