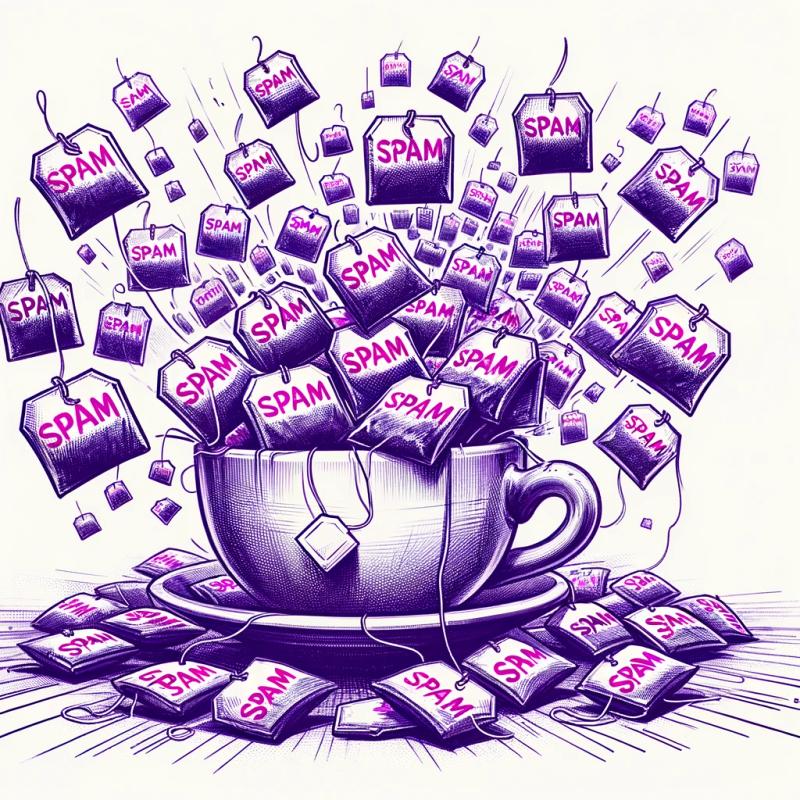
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
resolve-pathname
Advanced tools
Readme
resolve-pathname resolves URL pathnames identical to the way browsers resolve the pathname of an <a href>
value. The goals are:
Using npm:
$ npm install --save resolve-pathname
Then, use as you would anything else:
// using ES6 modules
import resolvePathname from 'resolve-pathname';
// using CommonJS modules
var resolvePathname = require('resolve-pathname');
The UMD build is also available on unpkg:
<script src="https://unpkg.com/resolve-pathname"></script>
You can find the library on window.resolvePathname
.
import resolvePathname from 'resolve-pathname';
// Simply pass the pathname you'd like to resolve. Second
// argument is the path we're coming from, or the current
// pathname. It defaults to "/".
resolvePathname('about', '/company/jobs'); // /company/about
resolvePathname('../jobs', '/company/team/ceo'); // /company/jobs
resolvePathname('about'); // /about
resolvePathname('/about'); // /about
// Index paths (with a trailing slash) are also supported and
// work the same way as browsers.
resolvePathname('about', '/company/info/'); // /company/info/about
// In browsers, it's easy to resolve a URL pathname relative to
// the current page. Just use window.location! e.g. if
// window.location.pathname == '/company/team/ceo' then
resolvePathname('cto', window.location.pathname); // /company/team/cto
resolvePathname('../jobs', window.location.pathname); // /company/jobs
url.resolve
implementation for full URLsFAQs
Resolve URL pathnames using JavaScript
The npm package resolve-pathname receives a total of 3,320,762 weekly downloads. As such, resolve-pathname popularity was classified as popular.
We found that resolve-pathname demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.