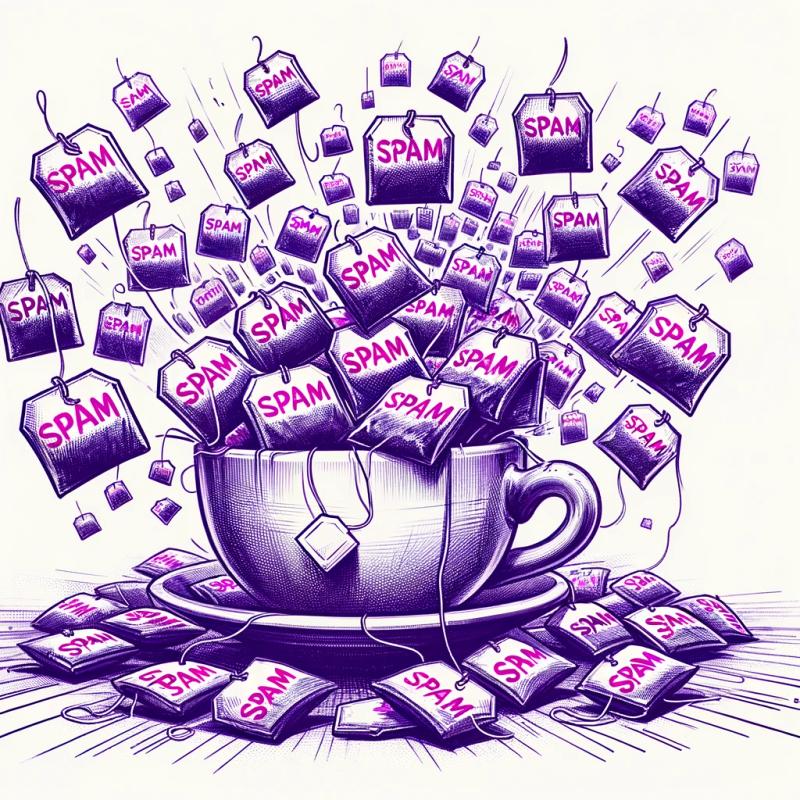
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
rgba-regex
Advanced tools
Readme
Regex for matching RGBA color strings.
npm install --save rgba-regex
var rgbaRegex = require('rgba-regex');
rgbaRegex({ exact: true }).test('rgba(12, 34, 56, .8)'); // => true
rgbaRegex({ exact: true }).test('unicorns'); // -> false
rgbaRegex({ exact: true }).test('rgba(,,,)'); // => false
rgbaRegex().exec('rgba(12, 34, 56, .8)');
// => [
// '12',
// '34',
// '56',
// '.8'
// index: 0,
// input: 'rgba(12,34,56, .8)'
// ]
'rgba(12, 34, 56, .8) cats and dogs'.match(rgbaRegex());
// = ['rgba(12, 34, 56, .8)']
MIT
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)Crafted with <3 by John Otander (@4lpine).
This package was initially generated with yeoman and the p generator.
FAQs
Regex for matching RGBA color strings.
The npm package rgba-regex receives a total of 2,266,467 weekly downloads. As such, rgba-regex popularity was classified as popular.
We found that rgba-regex demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.